Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / BindingNavigator.cs / 1305376 / BindingNavigator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; using System.Runtime.InteropServices; [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultProperty("BindingSource"), DefaultEvent("RefreshItems"), Designer("System.Windows.Forms.Design.BindingNavigatorDesigner, " + AssemblyRef.SystemDesign), SRDescription(SR.DescriptionBindingNavigator) ] ////// /// Creates an empty BindingNavigator tool strip. /// Call AddStandardItems() to add standard tool strip items. /// [EditorBrowsable(EditorBrowsableState.Never)] public BindingNavigator() : this(false) { } ////// /// Creates a BindingNavigator strip containing standard items, bound to the specified BindingSource. /// public BindingNavigator(BindingSource bindingSource) : this(true) { BindingSource = bindingSource; } ////// /// Creates an empty BindingNavigator tool strip, and adds the strip to the specified container. /// Call AddStandardItems() to add standard tool strip items. /// [EditorBrowsable(EditorBrowsableState.Never)] public BindingNavigator(IContainer container) : this(false) { if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } ////// /// Creates a BindingNavigator strip, optionally containing a set of standard tool strip items. /// public BindingNavigator(bool addStandardItems) { if (addStandardItems) { AddStandardItems(); } } ////// /// ISupportInitialize support. Disables updates to tool strip items during initialization. /// public void BeginInit() { initializing = true; } ////// /// ISupportInitialize support. Enables updates to tool strip items after initialization. /// public void EndInit() { initializing = false; RefreshItemsInternal(); } ////// Unhooks the BindingNavigator from the BindingSource. /// protected override void Dispose(bool disposing) { if (disposing) { BindingSource = null; // ...unwires from events of any prior BindingSource } base.Dispose(disposing); } ////// /// Adds standard set of tool strip items to a BindingNavigator tool strip, for basic /// navigation operations such as Move First, Move Next, Add New, etc. /// /// This method is called by the Windows Form Designer when a new BindingNavigator is /// added to a Form. When creating a BindingNavigator programmatically, this method /// must be called explicitly. /// /// Override this method in derived classes to define additional or alternative standard items. /// To ensure optimal design-time support for your derived class, make sure each item has a /// meaningful value in its Name property. At design time, this will be used to generate a unique /// name for the corresponding member variable. The item's Name property will then be updated /// to match the name given to the member variable. /// /// Note: This method does NOT remove any previous items from the strip, or suspend /// layout while items are being added. Those are responsibilities of the caller. /// public virtual void AddStandardItems() { // // Create items // MoveFirstItem = new System.Windows.Forms.ToolStripButton(); MovePreviousItem = new System.Windows.Forms.ToolStripButton(); MoveNextItem = new System.Windows.Forms.ToolStripButton(); MoveLastItem = new System.Windows.Forms.ToolStripButton(); PositionItem = new System.Windows.Forms.ToolStripTextBox(); CountItem = new System.Windows.Forms.ToolStripLabel(); AddNewItem = new System.Windows.Forms.ToolStripButton(); DeleteItem = new System.Windows.Forms.ToolStripButton(); ToolStripSeparator separator1 = new System.Windows.Forms.ToolStripSeparator(); ToolStripSeparator separator2 = new System.Windows.Forms.ToolStripSeparator(); ToolStripSeparator separator3 = new System.Windows.Forms.ToolStripSeparator(); // // Set up strings // // Hacky workaround for VSWhidbey 407243 // Default to lowercase for null name, because C# dev is more likely to create controls programmatically than // vb dev. char ch = string.IsNullOrEmpty(Name) || char.IsLower(Name[0]) ? 'b' : 'B'; MoveFirstItem.Name = ch + "indingNavigatorMoveFirstItem"; MovePreviousItem.Name = ch + "indingNavigatorMovePreviousItem"; MoveNextItem.Name = ch + "indingNavigatorMoveNextItem"; MoveLastItem.Name = ch + "indingNavigatorMoveLastItem"; PositionItem.Name = ch + "indingNavigatorPositionItem"; CountItem.Name = ch + "indingNavigatorCountItem"; AddNewItem.Name = ch + "indingNavigatorAddNewItem"; DeleteItem.Name = ch + "indingNavigatorDeleteItem"; separator1.Name = ch + "indingNavigatorSeparator"; separator2.Name = ch + "indingNavigatorSeparator"; separator3.Name = ch + "indingNavigatorSeparator"; MoveFirstItem.Text = SR.GetString(SR.BindingNavigatorMoveFirstItemText); MovePreviousItem.Text = SR.GetString(SR.BindingNavigatorMovePreviousItemText); MoveNextItem.Text = SR.GetString(SR.BindingNavigatorMoveNextItemText); MoveLastItem.Text = SR.GetString(SR.BindingNavigatorMoveLastItemText); AddNewItem.Text = SR.GetString(SR.BindingNavigatorAddNewItemText); DeleteItem.Text = SR.GetString(SR.BindingNavigatorDeleteItemText); CountItem.ToolTipText = SR.GetString(SR.BindingNavigatorCountItemTip); PositionItem.ToolTipText = SR.GetString(SR.BindingNavigatorPositionItemTip); CountItem.AutoToolTip = false; PositionItem.AutoToolTip = false; PositionItem.AccessibleName = SR.GetString(SR.BindingNavigatorPositionAccessibleName); // // Set up images // Bitmap moveFirstImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MoveFirst.bmp"); Bitmap movePreviousImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MovePrevious.bmp"); Bitmap moveNextImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MoveNext.bmp"); Bitmap moveLastImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MoveLast.bmp"); Bitmap addNewImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.AddNew.bmp"); Bitmap deleteImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.Delete.bmp"); moveFirstImage.MakeTransparent(System.Drawing.Color.Magenta); movePreviousImage.MakeTransparent(System.Drawing.Color.Magenta); moveNextImage.MakeTransparent(System.Drawing.Color.Magenta); moveLastImage.MakeTransparent(System.Drawing.Color.Magenta); addNewImage.MakeTransparent(System.Drawing.Color.Magenta); deleteImage.MakeTransparent(System.Drawing.Color.Magenta); MoveFirstItem.Image = moveFirstImage; MovePreviousItem.Image = movePreviousImage; MoveNextItem.Image = moveNextImage; MoveLastItem.Image = moveLastImage; AddNewItem.Image = addNewImage; DeleteItem.Image = deleteImage; MoveFirstItem.RightToLeftAutoMirrorImage = true; MovePreviousItem.RightToLeftAutoMirrorImage = true; MoveNextItem.RightToLeftAutoMirrorImage = true; MoveLastItem.RightToLeftAutoMirrorImage = true; AddNewItem.RightToLeftAutoMirrorImage = true; DeleteItem.RightToLeftAutoMirrorImage = true; MoveFirstItem.DisplayStyle = ToolStripItemDisplayStyle.Image; MovePreviousItem.DisplayStyle = ToolStripItemDisplayStyle.Image; MoveNextItem.DisplayStyle = ToolStripItemDisplayStyle.Image; MoveLastItem.DisplayStyle = ToolStripItemDisplayStyle.Image; AddNewItem.DisplayStyle = ToolStripItemDisplayStyle.Image; DeleteItem.DisplayStyle = ToolStripItemDisplayStyle.Image; // // Set other random properties // PositionItem.AutoSize = false; PositionItem.Width = 50; // // Add items to strip // Items.AddRange(new ToolStripItem[] { MoveFirstItem, MovePreviousItem, separator1, PositionItem, CountItem, separator2, MoveNextItem, MoveLastItem, separator3, AddNewItem, DeleteItem, }); } ////// /// The BindingSource who's list we are currently navigating, or null. /// [ DefaultValue(null), SRCategory(SR.CatData), SRDescription(SR.BindingNavigatorBindingSourcePropDescr), TypeConverter(typeof(ReferenceConverter)), SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly") ] public BindingSource BindingSource { get { return bindingSource; } set { WireUpBindingSource(ref bindingSource, value); } } ////// /// The ToolStripItem that triggers the 'Move first' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMoveFirstItemPropDescr) ] public ToolStripItem MoveFirstItem { get { if (moveFirstItem != null && moveFirstItem.IsDisposed) { moveFirstItem = null; } return moveFirstItem; } set { WireUpButton(ref moveFirstItem, value, new EventHandler(OnMoveFirst)); } } ////// /// The ToolStripItem that triggers the 'Move previous' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMovePreviousItemPropDescr) ] public ToolStripItem MovePreviousItem { get { if (movePreviousItem != null && movePreviousItem.IsDisposed) { movePreviousItem = null; } return movePreviousItem; } set { WireUpButton(ref movePreviousItem, value, new EventHandler(OnMovePrevious)); } } ////// /// The ToolStripItem that triggers the 'Move next' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMoveNextItemPropDescr) ] public ToolStripItem MoveNextItem { get { if (moveNextItem != null && moveNextItem.IsDisposed) { moveNextItem = null; } return moveNextItem; } set { WireUpButton(ref moveNextItem, value, new EventHandler(OnMoveNext)); } } ////// /// The ToolStripItem that triggers the 'Move last' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMoveLastItemPropDescr) ] public ToolStripItem MoveLastItem { get { if (moveLastItem != null && moveLastItem.IsDisposed) { moveLastItem = null; } return moveLastItem; } set { WireUpButton(ref moveLastItem, value, new EventHandler(OnMoveLast)); } } ////// /// The ToolStripItem that triggers the 'Add new' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorAddNewItemPropDescr) ] public ToolStripItem AddNewItem { get { if (addNewItem != null && addNewItem.IsDisposed) { addNewItem = null; } return addNewItem; } set { if (addNewItem != value && value != null) { value.InternalEnabledChanged += new System.EventHandler(OnAddNewItemEnabledChanged); addNewItemUserEnabled = value.Enabled; } WireUpButton(ref addNewItem, value, new EventHandler(OnAddNew)); } } ////// /// The ToolStripItem that triggers the 'Delete' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorDeleteItemPropDescr) ] public ToolStripItem DeleteItem { get { if (deleteItem != null && deleteItem.IsDisposed) { deleteItem = null; } return deleteItem; } set { if (deleteItem != value && value != null) { value.InternalEnabledChanged += new System.EventHandler(OnDeleteItemEnabledChanged); deleteItemUserEnabled = value.Enabled; } WireUpButton(ref deleteItem, value, new EventHandler(OnDelete)); } } ////// /// The ToolStripItem that displays the current position, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorPositionItemPropDescr) ] public ToolStripItem PositionItem { get { if (positionItem != null && positionItem.IsDisposed) { positionItem = null; } return positionItem; } set { WireUpTextBox(ref positionItem, value, new KeyEventHandler(OnPositionKey), new EventHandler(OnPositionLostFocus)); } } ////// /// The ToolStripItem that displays the total number of items, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorCountItemPropDescr) ] public ToolStripItem CountItem { get { if (countItem != null && countItem.IsDisposed) { countItem = null; } return countItem; } set { WireUpLabel(ref countItem, value); } } ////// /// Formatting to apply to count displayed in the CountItem tool strip item. /// [ SRCategory(SR.CatAppearance), SRDescription(SR.BindingNavigatorCountItemFormatPropDescr) ] public String CountItemFormat { get { return countItemFormat; } set { if (countItemFormat != value) { countItemFormat = value; RefreshItemsInternal(); } } } ////// /// Event raised when the state of the tool strip items needs to be /// refreshed to reflect the current state of the data. /// [ SRCategory(SR.CatBehavior), SRDescription(SR.BindingNavigatorRefreshItemsEventDescr) ] public event EventHandler RefreshItems { add { onRefreshItems += value; } remove { onRefreshItems -= value; } } ////// /// Refreshes the state of the standard items to reflect the current state of the data. /// [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void RefreshItemsCore() { int count, position; bool allowNew, allowRemove; // Get state info from the binding source (if any) if (bindingSource == null) { count = 0; position = 0; allowNew = false; allowRemove = false; } else { count = bindingSource.Count; position = bindingSource.Position + 1; allowNew = (bindingSource as IBindingList).AllowNew; allowRemove = (bindingSource as IBindingList).AllowRemove; } // Enable or disable items (except when in design mode) if (!DesignMode) { if (MoveFirstItem != null) moveFirstItem.Enabled = (position > 1); if (MovePreviousItem != null) movePreviousItem.Enabled = (position > 1); if (MoveNextItem != null) moveNextItem.Enabled = (position < count); if (MoveLastItem != null) moveLastItem.Enabled = (position < count); if (AddNewItem != null) { System.EventHandler handler = new System.EventHandler(OnAddNewItemEnabledChanged); addNewItem.InternalEnabledChanged -= handler; addNewItem.Enabled = (addNewItemUserEnabled && allowNew); addNewItem.InternalEnabledChanged += handler; } if (DeleteItem != null) { System.EventHandler handler = new System.EventHandler(OnDeleteItemEnabledChanged); deleteItem.InternalEnabledChanged -= handler; deleteItem.Enabled = (deleteItemUserEnabled && allowRemove && count > 0); deleteItem.InternalEnabledChanged += handler; } if (PositionItem != null) positionItem.Enabled = (position > 0 && count > 0); if (CountItem != null) countItem.Enabled = (count > 0); } // Update current position indicator if (positionItem != null) { positionItem.Text = position.ToString(CultureInfo.CurrentCulture); } // Update record count indicator if (countItem != null) { countItem.Text = DesignMode ? CountItemFormat : String.Format(CultureInfo.CurrentCulture, CountItemFormat, count); } } ////// /// Called when the state of the tool strip items needs to be refreshed to reflect the current state of the data. /// Calls protected virtual void OnRefreshItems() { // Refresh all the standard items RefreshItemsCore(); // Raise the public event if (onRefreshItems != null) { onRefreshItems(this, EventArgs.Empty); } } ///to refresh the state of the standard items, then raises the RefreshItems event. /// /// /// Triggers form validation. Used by the BindingNavigator's standard items when clicked. If a validation error occurs /// on the form, focus remains on the active control and the standard item does not perform its standard click action. /// Custom items may also use this method to trigger form validation and check for success before performing an action. /// public bool Validate() { bool validatedControlAllowsFocusChange; return this.ValidateActiveControl(out validatedControlAllowsFocusChange); } ////// Accept new row position entered into PositionItem. /// private void AcceptNewPosition() { // If no position item or binding source, do nothing if (positionItem == null || bindingSource == null) { return; } // Default to old position, in case new position turns out to be garbage int newPosition = bindingSource.Position; try { // Read new position from item text (and subtract one!) newPosition = Convert.ToInt32(positionItem.Text, CultureInfo.CurrentCulture) - 1; } catch (System.FormatException) { // Ignore bad user input } catch (System.OverflowException) { // Ignore bad user input } // If user has managed to enter a valid number, that is not the same as the current position, try // navigating to that position. Let the BindingSource validate the new position to keep it in range. if (newPosition != bindingSource.Position) { bindingSource.Position = newPosition; } // Update state of all items to reflect final position. If the user entered a bad position, // this will effectively reset the Position item back to showing the current position. RefreshItemsInternal(); } ////// Cancel new row position entered into PositionItem. /// private void CancelNewPosition() { // Just refresh state of all items to reflect current position // (causing position item's new value to get blasted away) RefreshItemsInternal(); } ////// Navigates to first item in BindingSource's list when the MoveFirstItem is clicked. /// private void OnMoveFirst(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MoveFirst(); RefreshItemsInternal(); } } } ////// Navigates to previous item in BindingSource's list when the MovePreviousItem is clicked. /// private void OnMovePrevious(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MovePrevious(); RefreshItemsInternal(); } } } ////// Navigates to next item in BindingSource's list when the MoveNextItem is clicked. /// private void OnMoveNext(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MoveNext(); RefreshItemsInternal(); } } } ////// Navigates to last item in BindingSource's list when the MoveLastItem is clicked. /// private void OnMoveLast(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MoveLast(); RefreshItemsInternal(); } } } ////// Adds new item to BindingSource's list when the AddNewItem is clicked. /// private void OnAddNew(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.AddNew(); RefreshItemsInternal(); } } } ////// Deletes current item from BindingSource's list when the DeleteItem is clicked. /// private void OnDelete(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.RemoveCurrent(); RefreshItemsInternal(); } } } ////// Navigates to specific item in BindingSource's list when a value is entered into the PositionItem. /// private void OnPositionKey(object sender, KeyEventArgs e) { switch (e.KeyCode) { case Keys.Enter: AcceptNewPosition(); break; case Keys.Escape: CancelNewPosition(); break; } } ////// Navigates to specific item in BindingSource's list when a value is entered into the PositionItem. /// private void OnPositionLostFocus(object sender, EventArgs e) { AcceptNewPosition(); } ////// Refresh tool strip items when something changes in the BindingSource. /// private void OnBindingSourceStateChanged(object sender, EventArgs e) { RefreshItemsInternal(); } ////// Refresh tool strip items when something changes in the BindingSource's list. /// private void OnBindingSourceListChanged(object sender, ListChangedEventArgs e) { RefreshItemsInternal(); } ////// Refresh the state of the items when the state of the data changes. /// private void RefreshItemsInternal() { // Block all updates during initialization if (initializing) { return; } // Call method that updates the items (overridable) OnRefreshItems(); } private void ResetCountItemFormat() { countItemFormat = SR.GetString(SR.BindingNavigatorCountItemFormat); } private bool ShouldSerializeCountItemFormat() { return countItemFormat != SR.GetString(SR.BindingNavigatorCountItemFormat); } private void OnAddNewItemEnabledChanged(object sender, EventArgs e) { if (AddNewItem != null) { addNewItemUserEnabled = addNewItem.Enabled; } } private void OnDeleteItemEnabledChanged(object sender, EventArgs e) { if (DeleteItem != null) { deleteItemUserEnabled = deleteItem.Enabled; } } ////// Wire up some member variable to the specified button item, hooking events /// on the new button and unhooking them from the previous button, if required. /// private void WireUpButton(ref ToolStripItem oldButton, ToolStripItem newButton, EventHandler clickHandler) { if (oldButton == newButton) { return; } if (oldButton != null) { oldButton.Click -= clickHandler; } if (newButton != null) { newButton.Click += clickHandler; } oldButton = newButton; RefreshItemsInternal(); } ////// Wire up some member variable to the specified text box item, hooking events /// on the new text box and unhooking them from the previous text box, if required. /// private void WireUpTextBox(ref ToolStripItem oldTextBox, ToolStripItem newTextBox, KeyEventHandler keyUpHandler, EventHandler lostFocusHandler) { if (oldTextBox != newTextBox) { ToolStripControlHost oldCtrl = oldTextBox as ToolStripControlHost; ToolStripControlHost newCtrl = newTextBox as ToolStripControlHost; if (oldCtrl != null) { oldCtrl.KeyUp -= keyUpHandler; oldCtrl.LostFocus -= lostFocusHandler; } if (newCtrl != null) { newCtrl.KeyUp += keyUpHandler; newCtrl.LostFocus += lostFocusHandler; } oldTextBox = newTextBox; RefreshItemsInternal(); } } ////// Wire up some member variable to the specified label item, hooking events /// on the new label and unhooking them from the previous label, if required. /// private void WireUpLabel(ref ToolStripItem oldLabel, ToolStripItem newLabel) { if (oldLabel != newLabel) { oldLabel = newLabel; RefreshItemsInternal(); } } ////// Wire up some member variable to the specified binding source, hooking events /// on the new binding source and unhooking them from the previous one, if required. /// private void WireUpBindingSource(ref BindingSource oldBindingSource, BindingSource newBindingSource) { if (oldBindingSource != newBindingSource) { if (oldBindingSource != null) { oldBindingSource.PositionChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.CurrentChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.CurrentItemChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.DataSourceChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.DataMemberChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.ListChanged -= new ListChangedEventHandler(OnBindingSourceListChanged); } if (newBindingSource != null) { newBindingSource.PositionChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.CurrentChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.CurrentItemChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.DataSourceChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.DataMemberChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.ListChanged += new ListChangedEventHandler(OnBindingSourceListChanged); } oldBindingSource = newBindingSource; RefreshItemsInternal(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; using System.Runtime.InteropServices; [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultProperty("BindingSource"), DefaultEvent("RefreshItems"), Designer("System.Windows.Forms.Design.BindingNavigatorDesigner, " + AssemblyRef.SystemDesign), SRDescription(SR.DescriptionBindingNavigator) ] ////// /// Creates an empty BindingNavigator tool strip. /// Call AddStandardItems() to add standard tool strip items. /// [EditorBrowsable(EditorBrowsableState.Never)] public BindingNavigator() : this(false) { } ////// /// Creates a BindingNavigator strip containing standard items, bound to the specified BindingSource. /// public BindingNavigator(BindingSource bindingSource) : this(true) { BindingSource = bindingSource; } ////// /// Creates an empty BindingNavigator tool strip, and adds the strip to the specified container. /// Call AddStandardItems() to add standard tool strip items. /// [EditorBrowsable(EditorBrowsableState.Never)] public BindingNavigator(IContainer container) : this(false) { if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } ////// /// Creates a BindingNavigator strip, optionally containing a set of standard tool strip items. /// public BindingNavigator(bool addStandardItems) { if (addStandardItems) { AddStandardItems(); } } ////// /// ISupportInitialize support. Disables updates to tool strip items during initialization. /// public void BeginInit() { initializing = true; } ////// /// ISupportInitialize support. Enables updates to tool strip items after initialization. /// public void EndInit() { initializing = false; RefreshItemsInternal(); } ////// Unhooks the BindingNavigator from the BindingSource. /// protected override void Dispose(bool disposing) { if (disposing) { BindingSource = null; // ...unwires from events of any prior BindingSource } base.Dispose(disposing); } ////// /// Adds standard set of tool strip items to a BindingNavigator tool strip, for basic /// navigation operations such as Move First, Move Next, Add New, etc. /// /// This method is called by the Windows Form Designer when a new BindingNavigator is /// added to a Form. When creating a BindingNavigator programmatically, this method /// must be called explicitly. /// /// Override this method in derived classes to define additional or alternative standard items. /// To ensure optimal design-time support for your derived class, make sure each item has a /// meaningful value in its Name property. At design time, this will be used to generate a unique /// name for the corresponding member variable. The item's Name property will then be updated /// to match the name given to the member variable. /// /// Note: This method does NOT remove any previous items from the strip, or suspend /// layout while items are being added. Those are responsibilities of the caller. /// public virtual void AddStandardItems() { // // Create items // MoveFirstItem = new System.Windows.Forms.ToolStripButton(); MovePreviousItem = new System.Windows.Forms.ToolStripButton(); MoveNextItem = new System.Windows.Forms.ToolStripButton(); MoveLastItem = new System.Windows.Forms.ToolStripButton(); PositionItem = new System.Windows.Forms.ToolStripTextBox(); CountItem = new System.Windows.Forms.ToolStripLabel(); AddNewItem = new System.Windows.Forms.ToolStripButton(); DeleteItem = new System.Windows.Forms.ToolStripButton(); ToolStripSeparator separator1 = new System.Windows.Forms.ToolStripSeparator(); ToolStripSeparator separator2 = new System.Windows.Forms.ToolStripSeparator(); ToolStripSeparator separator3 = new System.Windows.Forms.ToolStripSeparator(); // // Set up strings // // Hacky workaround for VSWhidbey 407243 // Default to lowercase for null name, because C# dev is more likely to create controls programmatically than // vb dev. char ch = string.IsNullOrEmpty(Name) || char.IsLower(Name[0]) ? 'b' : 'B'; MoveFirstItem.Name = ch + "indingNavigatorMoveFirstItem"; MovePreviousItem.Name = ch + "indingNavigatorMovePreviousItem"; MoveNextItem.Name = ch + "indingNavigatorMoveNextItem"; MoveLastItem.Name = ch + "indingNavigatorMoveLastItem"; PositionItem.Name = ch + "indingNavigatorPositionItem"; CountItem.Name = ch + "indingNavigatorCountItem"; AddNewItem.Name = ch + "indingNavigatorAddNewItem"; DeleteItem.Name = ch + "indingNavigatorDeleteItem"; separator1.Name = ch + "indingNavigatorSeparator"; separator2.Name = ch + "indingNavigatorSeparator"; separator3.Name = ch + "indingNavigatorSeparator"; MoveFirstItem.Text = SR.GetString(SR.BindingNavigatorMoveFirstItemText); MovePreviousItem.Text = SR.GetString(SR.BindingNavigatorMovePreviousItemText); MoveNextItem.Text = SR.GetString(SR.BindingNavigatorMoveNextItemText); MoveLastItem.Text = SR.GetString(SR.BindingNavigatorMoveLastItemText); AddNewItem.Text = SR.GetString(SR.BindingNavigatorAddNewItemText); DeleteItem.Text = SR.GetString(SR.BindingNavigatorDeleteItemText); CountItem.ToolTipText = SR.GetString(SR.BindingNavigatorCountItemTip); PositionItem.ToolTipText = SR.GetString(SR.BindingNavigatorPositionItemTip); CountItem.AutoToolTip = false; PositionItem.AutoToolTip = false; PositionItem.AccessibleName = SR.GetString(SR.BindingNavigatorPositionAccessibleName); // // Set up images // Bitmap moveFirstImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MoveFirst.bmp"); Bitmap movePreviousImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MovePrevious.bmp"); Bitmap moveNextImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MoveNext.bmp"); Bitmap moveLastImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.MoveLast.bmp"); Bitmap addNewImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.AddNew.bmp"); Bitmap deleteImage = new Bitmap(typeof(BindingNavigator), "BindingNavigator.Delete.bmp"); moveFirstImage.MakeTransparent(System.Drawing.Color.Magenta); movePreviousImage.MakeTransparent(System.Drawing.Color.Magenta); moveNextImage.MakeTransparent(System.Drawing.Color.Magenta); moveLastImage.MakeTransparent(System.Drawing.Color.Magenta); addNewImage.MakeTransparent(System.Drawing.Color.Magenta); deleteImage.MakeTransparent(System.Drawing.Color.Magenta); MoveFirstItem.Image = moveFirstImage; MovePreviousItem.Image = movePreviousImage; MoveNextItem.Image = moveNextImage; MoveLastItem.Image = moveLastImage; AddNewItem.Image = addNewImage; DeleteItem.Image = deleteImage; MoveFirstItem.RightToLeftAutoMirrorImage = true; MovePreviousItem.RightToLeftAutoMirrorImage = true; MoveNextItem.RightToLeftAutoMirrorImage = true; MoveLastItem.RightToLeftAutoMirrorImage = true; AddNewItem.RightToLeftAutoMirrorImage = true; DeleteItem.RightToLeftAutoMirrorImage = true; MoveFirstItem.DisplayStyle = ToolStripItemDisplayStyle.Image; MovePreviousItem.DisplayStyle = ToolStripItemDisplayStyle.Image; MoveNextItem.DisplayStyle = ToolStripItemDisplayStyle.Image; MoveLastItem.DisplayStyle = ToolStripItemDisplayStyle.Image; AddNewItem.DisplayStyle = ToolStripItemDisplayStyle.Image; DeleteItem.DisplayStyle = ToolStripItemDisplayStyle.Image; // // Set other random properties // PositionItem.AutoSize = false; PositionItem.Width = 50; // // Add items to strip // Items.AddRange(new ToolStripItem[] { MoveFirstItem, MovePreviousItem, separator1, PositionItem, CountItem, separator2, MoveNextItem, MoveLastItem, separator3, AddNewItem, DeleteItem, }); } ////// /// The BindingSource who's list we are currently navigating, or null. /// [ DefaultValue(null), SRCategory(SR.CatData), SRDescription(SR.BindingNavigatorBindingSourcePropDescr), TypeConverter(typeof(ReferenceConverter)), SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly") ] public BindingSource BindingSource { get { return bindingSource; } set { WireUpBindingSource(ref bindingSource, value); } } ////// /// The ToolStripItem that triggers the 'Move first' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMoveFirstItemPropDescr) ] public ToolStripItem MoveFirstItem { get { if (moveFirstItem != null && moveFirstItem.IsDisposed) { moveFirstItem = null; } return moveFirstItem; } set { WireUpButton(ref moveFirstItem, value, new EventHandler(OnMoveFirst)); } } ////// /// The ToolStripItem that triggers the 'Move previous' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMovePreviousItemPropDescr) ] public ToolStripItem MovePreviousItem { get { if (movePreviousItem != null && movePreviousItem.IsDisposed) { movePreviousItem = null; } return movePreviousItem; } set { WireUpButton(ref movePreviousItem, value, new EventHandler(OnMovePrevious)); } } ////// /// The ToolStripItem that triggers the 'Move next' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMoveNextItemPropDescr) ] public ToolStripItem MoveNextItem { get { if (moveNextItem != null && moveNextItem.IsDisposed) { moveNextItem = null; } return moveNextItem; } set { WireUpButton(ref moveNextItem, value, new EventHandler(OnMoveNext)); } } ////// /// The ToolStripItem that triggers the 'Move last' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorMoveLastItemPropDescr) ] public ToolStripItem MoveLastItem { get { if (moveLastItem != null && moveLastItem.IsDisposed) { moveLastItem = null; } return moveLastItem; } set { WireUpButton(ref moveLastItem, value, new EventHandler(OnMoveLast)); } } ////// /// The ToolStripItem that triggers the 'Add new' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorAddNewItemPropDescr) ] public ToolStripItem AddNewItem { get { if (addNewItem != null && addNewItem.IsDisposed) { addNewItem = null; } return addNewItem; } set { if (addNewItem != value && value != null) { value.InternalEnabledChanged += new System.EventHandler(OnAddNewItemEnabledChanged); addNewItemUserEnabled = value.Enabled; } WireUpButton(ref addNewItem, value, new EventHandler(OnAddNew)); } } ////// /// The ToolStripItem that triggers the 'Delete' action, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorDeleteItemPropDescr) ] public ToolStripItem DeleteItem { get { if (deleteItem != null && deleteItem.IsDisposed) { deleteItem = null; } return deleteItem; } set { if (deleteItem != value && value != null) { value.InternalEnabledChanged += new System.EventHandler(OnDeleteItemEnabledChanged); deleteItemUserEnabled = value.Enabled; } WireUpButton(ref deleteItem, value, new EventHandler(OnDelete)); } } ////// /// The ToolStripItem that displays the current position, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorPositionItemPropDescr) ] public ToolStripItem PositionItem { get { if (positionItem != null && positionItem.IsDisposed) { positionItem = null; } return positionItem; } set { WireUpTextBox(ref positionItem, value, new KeyEventHandler(OnPositionKey), new EventHandler(OnPositionLostFocus)); } } ////// /// The ToolStripItem that displays the total number of items, or null. /// [ TypeConverter(typeof(ReferenceConverter)), SRCategory(SR.CatItems), SRDescription(SR.BindingNavigatorCountItemPropDescr) ] public ToolStripItem CountItem { get { if (countItem != null && countItem.IsDisposed) { countItem = null; } return countItem; } set { WireUpLabel(ref countItem, value); } } ////// /// Formatting to apply to count displayed in the CountItem tool strip item. /// [ SRCategory(SR.CatAppearance), SRDescription(SR.BindingNavigatorCountItemFormatPropDescr) ] public String CountItemFormat { get { return countItemFormat; } set { if (countItemFormat != value) { countItemFormat = value; RefreshItemsInternal(); } } } ////// /// Event raised when the state of the tool strip items needs to be /// refreshed to reflect the current state of the data. /// [ SRCategory(SR.CatBehavior), SRDescription(SR.BindingNavigatorRefreshItemsEventDescr) ] public event EventHandler RefreshItems { add { onRefreshItems += value; } remove { onRefreshItems -= value; } } ////// /// Refreshes the state of the standard items to reflect the current state of the data. /// [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void RefreshItemsCore() { int count, position; bool allowNew, allowRemove; // Get state info from the binding source (if any) if (bindingSource == null) { count = 0; position = 0; allowNew = false; allowRemove = false; } else { count = bindingSource.Count; position = bindingSource.Position + 1; allowNew = (bindingSource as IBindingList).AllowNew; allowRemove = (bindingSource as IBindingList).AllowRemove; } // Enable or disable items (except when in design mode) if (!DesignMode) { if (MoveFirstItem != null) moveFirstItem.Enabled = (position > 1); if (MovePreviousItem != null) movePreviousItem.Enabled = (position > 1); if (MoveNextItem != null) moveNextItem.Enabled = (position < count); if (MoveLastItem != null) moveLastItem.Enabled = (position < count); if (AddNewItem != null) { System.EventHandler handler = new System.EventHandler(OnAddNewItemEnabledChanged); addNewItem.InternalEnabledChanged -= handler; addNewItem.Enabled = (addNewItemUserEnabled && allowNew); addNewItem.InternalEnabledChanged += handler; } if (DeleteItem != null) { System.EventHandler handler = new System.EventHandler(OnDeleteItemEnabledChanged); deleteItem.InternalEnabledChanged -= handler; deleteItem.Enabled = (deleteItemUserEnabled && allowRemove && count > 0); deleteItem.InternalEnabledChanged += handler; } if (PositionItem != null) positionItem.Enabled = (position > 0 && count > 0); if (CountItem != null) countItem.Enabled = (count > 0); } // Update current position indicator if (positionItem != null) { positionItem.Text = position.ToString(CultureInfo.CurrentCulture); } // Update record count indicator if (countItem != null) { countItem.Text = DesignMode ? CountItemFormat : String.Format(CultureInfo.CurrentCulture, CountItemFormat, count); } } ////// /// Called when the state of the tool strip items needs to be refreshed to reflect the current state of the data. /// Calls protected virtual void OnRefreshItems() { // Refresh all the standard items RefreshItemsCore(); // Raise the public event if (onRefreshItems != null) { onRefreshItems(this, EventArgs.Empty); } } ///to refresh the state of the standard items, then raises the RefreshItems event. /// /// /// Triggers form validation. Used by the BindingNavigator's standard items when clicked. If a validation error occurs /// on the form, focus remains on the active control and the standard item does not perform its standard click action. /// Custom items may also use this method to trigger form validation and check for success before performing an action. /// public bool Validate() { bool validatedControlAllowsFocusChange; return this.ValidateActiveControl(out validatedControlAllowsFocusChange); } ////// Accept new row position entered into PositionItem. /// private void AcceptNewPosition() { // If no position item or binding source, do nothing if (positionItem == null || bindingSource == null) { return; } // Default to old position, in case new position turns out to be garbage int newPosition = bindingSource.Position; try { // Read new position from item text (and subtract one!) newPosition = Convert.ToInt32(positionItem.Text, CultureInfo.CurrentCulture) - 1; } catch (System.FormatException) { // Ignore bad user input } catch (System.OverflowException) { // Ignore bad user input } // If user has managed to enter a valid number, that is not the same as the current position, try // navigating to that position. Let the BindingSource validate the new position to keep it in range. if (newPosition != bindingSource.Position) { bindingSource.Position = newPosition; } // Update state of all items to reflect final position. If the user entered a bad position, // this will effectively reset the Position item back to showing the current position. RefreshItemsInternal(); } ////// Cancel new row position entered into PositionItem. /// private void CancelNewPosition() { // Just refresh state of all items to reflect current position // (causing position item's new value to get blasted away) RefreshItemsInternal(); } ////// Navigates to first item in BindingSource's list when the MoveFirstItem is clicked. /// private void OnMoveFirst(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MoveFirst(); RefreshItemsInternal(); } } } ////// Navigates to previous item in BindingSource's list when the MovePreviousItem is clicked. /// private void OnMovePrevious(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MovePrevious(); RefreshItemsInternal(); } } } ////// Navigates to next item in BindingSource's list when the MoveNextItem is clicked. /// private void OnMoveNext(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MoveNext(); RefreshItemsInternal(); } } } ////// Navigates to last item in BindingSource's list when the MoveLastItem is clicked. /// private void OnMoveLast(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.MoveLast(); RefreshItemsInternal(); } } } ////// Adds new item to BindingSource's list when the AddNewItem is clicked. /// private void OnAddNew(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.AddNew(); RefreshItemsInternal(); } } } ////// Deletes current item from BindingSource's list when the DeleteItem is clicked. /// private void OnDelete(object sender, EventArgs e) { if (Validate()) { if (bindingSource != null) { bindingSource.RemoveCurrent(); RefreshItemsInternal(); } } } ////// Navigates to specific item in BindingSource's list when a value is entered into the PositionItem. /// private void OnPositionKey(object sender, KeyEventArgs e) { switch (e.KeyCode) { case Keys.Enter: AcceptNewPosition(); break; case Keys.Escape: CancelNewPosition(); break; } } ////// Navigates to specific item in BindingSource's list when a value is entered into the PositionItem. /// private void OnPositionLostFocus(object sender, EventArgs e) { AcceptNewPosition(); } ////// Refresh tool strip items when something changes in the BindingSource. /// private void OnBindingSourceStateChanged(object sender, EventArgs e) { RefreshItemsInternal(); } ////// Refresh tool strip items when something changes in the BindingSource's list. /// private void OnBindingSourceListChanged(object sender, ListChangedEventArgs e) { RefreshItemsInternal(); } ////// Refresh the state of the items when the state of the data changes. /// private void RefreshItemsInternal() { // Block all updates during initialization if (initializing) { return; } // Call method that updates the items (overridable) OnRefreshItems(); } private void ResetCountItemFormat() { countItemFormat = SR.GetString(SR.BindingNavigatorCountItemFormat); } private bool ShouldSerializeCountItemFormat() { return countItemFormat != SR.GetString(SR.BindingNavigatorCountItemFormat); } private void OnAddNewItemEnabledChanged(object sender, EventArgs e) { if (AddNewItem != null) { addNewItemUserEnabled = addNewItem.Enabled; } } private void OnDeleteItemEnabledChanged(object sender, EventArgs e) { if (DeleteItem != null) { deleteItemUserEnabled = deleteItem.Enabled; } } ////// Wire up some member variable to the specified button item, hooking events /// on the new button and unhooking them from the previous button, if required. /// private void WireUpButton(ref ToolStripItem oldButton, ToolStripItem newButton, EventHandler clickHandler) { if (oldButton == newButton) { return; } if (oldButton != null) { oldButton.Click -= clickHandler; } if (newButton != null) { newButton.Click += clickHandler; } oldButton = newButton; RefreshItemsInternal(); } ////// Wire up some member variable to the specified text box item, hooking events /// on the new text box and unhooking them from the previous text box, if required. /// private void WireUpTextBox(ref ToolStripItem oldTextBox, ToolStripItem newTextBox, KeyEventHandler keyUpHandler, EventHandler lostFocusHandler) { if (oldTextBox != newTextBox) { ToolStripControlHost oldCtrl = oldTextBox as ToolStripControlHost; ToolStripControlHost newCtrl = newTextBox as ToolStripControlHost; if (oldCtrl != null) { oldCtrl.KeyUp -= keyUpHandler; oldCtrl.LostFocus -= lostFocusHandler; } if (newCtrl != null) { newCtrl.KeyUp += keyUpHandler; newCtrl.LostFocus += lostFocusHandler; } oldTextBox = newTextBox; RefreshItemsInternal(); } } ////// Wire up some member variable to the specified label item, hooking events /// on the new label and unhooking them from the previous label, if required. /// private void WireUpLabel(ref ToolStripItem oldLabel, ToolStripItem newLabel) { if (oldLabel != newLabel) { oldLabel = newLabel; RefreshItemsInternal(); } } ////// Wire up some member variable to the specified binding source, hooking events /// on the new binding source and unhooking them from the previous one, if required. /// private void WireUpBindingSource(ref BindingSource oldBindingSource, BindingSource newBindingSource) { if (oldBindingSource != newBindingSource) { if (oldBindingSource != null) { oldBindingSource.PositionChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.CurrentChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.CurrentItemChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.DataSourceChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.DataMemberChanged -= new EventHandler(OnBindingSourceStateChanged); oldBindingSource.ListChanged -= new ListChangedEventHandler(OnBindingSourceListChanged); } if (newBindingSource != null) { newBindingSource.PositionChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.CurrentChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.CurrentItemChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.DataSourceChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.DataMemberChanged += new EventHandler(OnBindingSourceStateChanged); newBindingSource.ListChanged += new ListChangedEventHandler(OnBindingSourceListChanged); } oldBindingSource = newBindingSource; RefreshItemsInternal(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
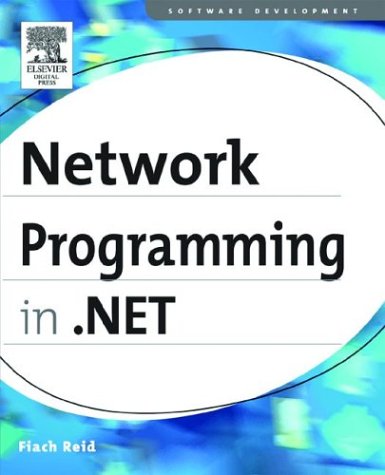
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNamespaceDeclarationsAttribute.cs
- DataGridViewColumnCollectionEditor.cs
- RemoteWebConfigurationHost.cs
- ResourcePool.cs
- QilXmlWriter.cs
- ViewPort3D.cs
- DataShape.cs
- ExclusiveHandleList.cs
- TranslateTransform.cs
- DataGridItemCollection.cs
- HttpChannelListener.cs
- PageThemeParser.cs
- StylusEventArgs.cs
- TemplateApplicationHelper.cs
- AdRotator.cs
- PixelShader.cs
- FragmentQueryKB.cs
- InputScopeConverter.cs
- ScrollBar.cs
- IdentityValidationException.cs
- ResourceAttributes.cs
- XmlILStorageConverter.cs
- SplitContainer.cs
- CharEntityEncoderFallback.cs
- AppSettingsReader.cs
- ButtonPopupAdapter.cs
- ColumnClickEvent.cs
- DataGridViewSelectedColumnCollection.cs
- DataReaderContainer.cs
- KeyMatchBuilder.cs
- DataReaderContainer.cs
- CompilationRelaxations.cs
- DataGridColumnsPage.cs
- EntityCommandCompilationException.cs
- UserPreferenceChangingEventArgs.cs
- SoundPlayer.cs
- ListControlConvertEventArgs.cs
- ArithmeticLiteral.cs
- ModifiableIteratorCollection.cs
- AdornerPresentationContext.cs
- IdentifierCollection.cs
- ExpressionParser.cs
- FakeModelItemImpl.cs
- counter.cs
- DictionaryItemsCollection.cs
- x509store.cs
- DateTimeUtil.cs
- XPathAncestorQuery.cs
- Graphics.cs
- NullRuntimeConfig.cs
- GenericXmlSecurityToken.cs
- NullableLongMinMaxAggregationOperator.cs
- EventProviderWriter.cs
- HMACSHA512.cs
- AssemblyCollection.cs
- AddInDeploymentState.cs
- HttpListenerElement.cs
- CompilerGeneratedAttribute.cs
- RegistryPermission.cs
- IFlowDocumentViewer.cs
- NameValueConfigurationElement.cs
- XmlSchemaExporter.cs
- ImageConverter.cs
- CryptoStream.cs
- GeneralTransform3DCollection.cs
- SmiXetterAccessMap.cs
- LiteralControl.cs
- BuildManager.cs
- MyContact.cs
- Decoder.cs
- NativeWindow.cs
- CookieProtection.cs
- StringToken.cs
- LogRecordSequence.cs
- AddingNewEventArgs.cs
- SqlLiftIndependentRowExpressions.cs
- PointConverter.cs
- ToolStripDropTargetManager.cs
- UpdateException.cs
- AuthorizationRule.cs
- TableLayoutColumnStyleCollection.cs
- ProtocolsConfigurationEntry.cs
- EntryPointNotFoundException.cs
- ExpressionBuilderContext.cs
- XmlSchemaImporter.cs
- EntityParameterCollection.cs
- UnsafeCollabNativeMethods.cs
- Random.cs
- Vector3DConverter.cs
- SecurityTokenRequirement.cs
- List.cs
- ScrollChangedEventArgs.cs
- ScrollProviderWrapper.cs
- DataTrigger.cs
- VectorCollectionConverter.cs
- SwitchElementsCollection.cs
- KeyValueConfigurationCollection.cs
- UIElement.cs
- SQLSingle.cs
- ScanQueryOperator.cs