Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / Button.cs / 1305376 / Button.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.Windows.Forms.ButtonInternal; using System.ComponentModel.Design; using System.ComponentModel; using System.Windows.Forms.Layout; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; ////// /// [ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), SRDescription(SR.DescriptionButton), Designer("System.Windows.Forms.Design.ButtonBaseDesigner, " + AssemblyRef.SystemDesign) ] public class Button : ButtonBase, IButtonControl { ///Represents a /// Windows button. ////// /// The dialog result that will be sent to the parent dialog form when /// we are clicked. /// private DialogResult dialogResult; ////// /// For buttons whose FaltStyle = FlatStyle.Flat, this property specifies the size, in pixels /// of the border around the button. /// private Size systemSize = new Size(Int32.MinValue, Int32.MinValue); ////// /// public Button() : base() { // Buttons shouldn't respond to right clicks, so we need to do all our own click logic SetStyle(ControlStyles.StandardClick | ControlStyles.StandardDoubleClick, false); } ////// Initializes a new instance of the ////// class. /// /// Allows the control to optionally shrink when AutoSize is true. /// [ SRCategory(SR.CatLayout), Browsable(true), DefaultValue(AutoSizeMode.GrowOnly), Localizable(true), SRDescription(SR.ControlAutoSizeModeDescr) ] public AutoSizeMode AutoSizeMode { get { return GetAutoSizeMode(); } set { if (!ClientUtils.IsEnumValid(value, (int)value, (int)AutoSizeMode.GrowAndShrink, (int)AutoSizeMode.GrowOnly)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(AutoSizeMode)); } if (GetAutoSizeMode() != value) { SetAutoSizeMode(value); if(ParentInternal != null) { // DefaultLayout does not keep anchor information until it needs to. When // AutoSize became a common property, we could no longer blindly call into // DefaultLayout, so now we do a special InitLayout just for DefaultLayout. if(ParentInternal.LayoutEngine == DefaultLayout.Instance) { ParentInternal.LayoutEngine.InitLayout(this, BoundsSpecified.Size); } LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.AutoSize); } } } } internal override ButtonBaseAdapter CreateFlatAdapter() { return new ButtonFlatAdapter(this); } internal override ButtonBaseAdapter CreatePopupAdapter() { return new ButtonPopupAdapter(this); } internal override ButtonBaseAdapter CreateStandardAdapter() { return new ButtonStandardAdapter(this); } internal override Size GetPreferredSizeCore(Size proposedConstraints) { if(FlatStyle != FlatStyle.System) { Size prefSize = base.GetPreferredSizeCore(proposedConstraints); return AutoSizeMode == AutoSizeMode.GrowAndShrink ? prefSize : LayoutUtils.UnionSizes(prefSize, Size); } if (systemSize.Width == Int32.MinValue) { Size requiredSize; // Note: The result from the BCM_GETIDEALSIZE message isn't accurate if the font has been // changed, because this method is called before the font is set into the device context. // Commenting this line is the fix for bug VSWhidbey#228843. //if(UnsafeNativeMethods.SendMessage(hWnd, NativeMethods.BCM_GETIDEALSIZE, 0, size) != IntPtr.Zero) { // requiredSize = size.ToSize(); ... requiredSize = TextRenderer.MeasureText(this.Text, this.Font); requiredSize = SizeFromClientSize(requiredSize); // This padding makes FlatStyle.System about the same size as FlatStyle.Standard // with an 8px font. requiredSize.Width += 14; requiredSize.Height += 9; systemSize = requiredSize; } Size paddedSize = systemSize + Padding.Size; return AutoSizeMode == AutoSizeMode.GrowAndShrink ? paddedSize : LayoutUtils.UnionSizes(paddedSize, Size); } ////// /// /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.ClassName = "BUTTON"; if (GetStyle(ControlStyles.UserPaint)) { cp.Style |= NativeMethods.BS_OWNERDRAW; } else { cp.Style |= NativeMethods.BS_PUSHBUTTON; if (IsDefault) { cp.Style |= NativeMethods.BS_DEFPUSHBUTTON; } } return cp; } } ////// This is called when creating a window. Inheriting classes can overide /// this to add extra functionality, but should not forget to first call /// base.CreateParams() to make sure the control continues to work /// correctly. /// /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(DialogResult.None), SRDescription(SR.ButtonDialogResultDescr) ] public virtual DialogResult DialogResult { get { return dialogResult; } set { if (!ClientUtils.IsEnumValid(value, (int)value, (int)DialogResult.None, (int) DialogResult.No)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DialogResult)); } dialogResult = value; } } ////// Gets or sets a value that is returned to the /// parent form when the button /// is clicked. /// ////// /// /// protected override void OnMouseEnter(EventArgs e) { base.OnMouseEnter(e); } ////// Raises the ///event. /// /// /// /// protected override void OnMouseLeave(EventArgs e) { base.OnMouseLeave(e); } ////// Raises the ///event. /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced)] public new event EventHandler DoubleClick { add { base.DoubleClick += value; } remove { base.DoubleClick -= value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced)] public new event MouseEventHandler MouseDoubleClick { add { base.MouseDoubleClick += value; } remove { base.MouseDoubleClick -= value; } } /// /// /// public virtual void NotifyDefault(bool value) { if (IsDefault != value) { IsDefault = value; } } ////// Notifies the ////// whether it is the default button so that it can adjust its appearance /// accordingly. /// /// /// /// /// protected override void OnClick(EventArgs e) { Form form = FindFormInternal(); if (form != null) form.DialogResult = dialogResult; // accessibility stuff // AccessibilityNotifyClients(AccessibleEvents.StateChange, -1); AccessibilityNotifyClients(AccessibleEvents.NameChange, -1); base.OnClick(e); } protected override void OnFontChanged(EventArgs e) { systemSize = new Size(Int32.MinValue, Int32.MinValue); base.OnFontChanged(e); } ////// This method actually raises the Click event. Inheriting classes should /// override this if they wish to be notified of a Click event. (This is far /// preferable to actually adding an event handler.) They should not, /// however, forget to call base.onClick(e); before exiting, to ensure that /// other recipients do actually get the event. /// /// ////// /// /// protected override void OnMouseUp(MouseEventArgs mevent) { if (mevent.Button == MouseButtons.Left && MouseIsPressed) { bool isMouseDown = base.MouseIsDown; if (GetStyle(ControlStyles.UserPaint)) { //Paint in raised state... ResetFlagsandPaint(); } if (isMouseDown) { Point pt = PointToScreen(new Point(mevent.X, mevent.Y)); if (UnsafeNativeMethods.WindowFromPoint(pt.X, pt.Y) == Handle && !ValidationCancelled) { if (GetStyle(ControlStyles.UserPaint)) { OnClick(mevent); } OnMouseClick(mevent); } } } base.OnMouseUp(mevent); } protected override void OnTextChanged(EventArgs e) { systemSize = new Size(Int32.MinValue, Int32.MinValue); base.OnTextChanged(e); } ////// Raises the ///event. /// /// /// /// public void PerformClick() { if (CanSelect) { bool validatedControlAllowsFocusChange; bool validate = ValidateActiveControl(out validatedControlAllowsFocusChange); if (!ValidationCancelled && (validate || validatedControlAllowsFocusChange)) { //Paint in raised state... // ResetFlagsandPaint(); OnClick(EventArgs.Empty); } } } ////// Generates a ///event for a /// button. /// /// /// /// [UIPermission(SecurityAction.LinkDemand, Window=UIPermissionWindow.AllWindows)] protected internal override bool ProcessMnemonic(char charCode) { if (UseMnemonic && CanProcessMnemonic() && IsMnemonic(charCode, Text)) { PerformClick(); return true; } return base.ProcessMnemonic(charCode); } ////// Lets a control process mnmemonic characters. Inheriting classes can /// override this to add extra functionality, but should not forget to call /// base.ProcessMnemonic(charCode); to ensure basic functionality /// remains unchanged. /// /// ////// /// /// public override string ToString() { string s = base.ToString(); return s + ", Text: " + Text; } ////// Provides some interesting information for the Button control in /// String form. /// ////// /// The button's window procedure. Inheriting classes can override this /// to add extra functionality, but should not forget to call /// base.wndProc(m); to ensure the button continues to function properly. /// ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected override void WndProc(ref Message m) { switch (m.Msg) { case NativeMethods.WM_REFLECT + NativeMethods.WM_COMMAND: if (NativeMethods.Util.HIWORD(m.WParam) == NativeMethods.BN_CLICKED) { Debug.Assert(!GetStyle(ControlStyles.UserPaint), "Shouldn't get BN_CLICKED when UserPaint"); if (!ValidationCancelled) { OnClick(EventArgs.Empty); } } break; case NativeMethods.WM_ERASEBKGND: DefWndProc(ref m); break; default: base.WndProc(ref m); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.Windows.Forms.ButtonInternal; using System.ComponentModel.Design; using System.ComponentModel; using System.Windows.Forms.Layout; using System.Drawing; using System.Windows.Forms.Internal; using Microsoft.Win32; ////// /// [ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), SRDescription(SR.DescriptionButton), Designer("System.Windows.Forms.Design.ButtonBaseDesigner, " + AssemblyRef.SystemDesign) ] public class Button : ButtonBase, IButtonControl { ///Represents a /// Windows button. ////// /// The dialog result that will be sent to the parent dialog form when /// we are clicked. /// private DialogResult dialogResult; ////// /// For buttons whose FaltStyle = FlatStyle.Flat, this property specifies the size, in pixels /// of the border around the button. /// private Size systemSize = new Size(Int32.MinValue, Int32.MinValue); ////// /// public Button() : base() { // Buttons shouldn't respond to right clicks, so we need to do all our own click logic SetStyle(ControlStyles.StandardClick | ControlStyles.StandardDoubleClick, false); } ////// Initializes a new instance of the ////// class. /// /// Allows the control to optionally shrink when AutoSize is true. /// [ SRCategory(SR.CatLayout), Browsable(true), DefaultValue(AutoSizeMode.GrowOnly), Localizable(true), SRDescription(SR.ControlAutoSizeModeDescr) ] public AutoSizeMode AutoSizeMode { get { return GetAutoSizeMode(); } set { if (!ClientUtils.IsEnumValid(value, (int)value, (int)AutoSizeMode.GrowAndShrink, (int)AutoSizeMode.GrowOnly)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(AutoSizeMode)); } if (GetAutoSizeMode() != value) { SetAutoSizeMode(value); if(ParentInternal != null) { // DefaultLayout does not keep anchor information until it needs to. When // AutoSize became a common property, we could no longer blindly call into // DefaultLayout, so now we do a special InitLayout just for DefaultLayout. if(ParentInternal.LayoutEngine == DefaultLayout.Instance) { ParentInternal.LayoutEngine.InitLayout(this, BoundsSpecified.Size); } LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.AutoSize); } } } } internal override ButtonBaseAdapter CreateFlatAdapter() { return new ButtonFlatAdapter(this); } internal override ButtonBaseAdapter CreatePopupAdapter() { return new ButtonPopupAdapter(this); } internal override ButtonBaseAdapter CreateStandardAdapter() { return new ButtonStandardAdapter(this); } internal override Size GetPreferredSizeCore(Size proposedConstraints) { if(FlatStyle != FlatStyle.System) { Size prefSize = base.GetPreferredSizeCore(proposedConstraints); return AutoSizeMode == AutoSizeMode.GrowAndShrink ? prefSize : LayoutUtils.UnionSizes(prefSize, Size); } if (systemSize.Width == Int32.MinValue) { Size requiredSize; // Note: The result from the BCM_GETIDEALSIZE message isn't accurate if the font has been // changed, because this method is called before the font is set into the device context. // Commenting this line is the fix for bug VSWhidbey#228843. //if(UnsafeNativeMethods.SendMessage(hWnd, NativeMethods.BCM_GETIDEALSIZE, 0, size) != IntPtr.Zero) { // requiredSize = size.ToSize(); ... requiredSize = TextRenderer.MeasureText(this.Text, this.Font); requiredSize = SizeFromClientSize(requiredSize); // This padding makes FlatStyle.System about the same size as FlatStyle.Standard // with an 8px font. requiredSize.Width += 14; requiredSize.Height += 9; systemSize = requiredSize; } Size paddedSize = systemSize + Padding.Size; return AutoSizeMode == AutoSizeMode.GrowAndShrink ? paddedSize : LayoutUtils.UnionSizes(paddedSize, Size); } ////// /// /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.ClassName = "BUTTON"; if (GetStyle(ControlStyles.UserPaint)) { cp.Style |= NativeMethods.BS_OWNERDRAW; } else { cp.Style |= NativeMethods.BS_PUSHBUTTON; if (IsDefault) { cp.Style |= NativeMethods.BS_DEFPUSHBUTTON; } } return cp; } } ////// This is called when creating a window. Inheriting classes can overide /// this to add extra functionality, but should not forget to first call /// base.CreateParams() to make sure the control continues to work /// correctly. /// /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(DialogResult.None), SRDescription(SR.ButtonDialogResultDescr) ] public virtual DialogResult DialogResult { get { return dialogResult; } set { if (!ClientUtils.IsEnumValid(value, (int)value, (int)DialogResult.None, (int) DialogResult.No)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DialogResult)); } dialogResult = value; } } ////// Gets or sets a value that is returned to the /// parent form when the button /// is clicked. /// ////// /// /// protected override void OnMouseEnter(EventArgs e) { base.OnMouseEnter(e); } ////// Raises the ///event. /// /// /// /// protected override void OnMouseLeave(EventArgs e) { base.OnMouseLeave(e); } ////// Raises the ///event. /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced)] public new event EventHandler DoubleClick { add { base.DoubleClick += value; } remove { base.DoubleClick -= value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Advanced)] public new event MouseEventHandler MouseDoubleClick { add { base.MouseDoubleClick += value; } remove { base.MouseDoubleClick -= value; } } /// /// /// public virtual void NotifyDefault(bool value) { if (IsDefault != value) { IsDefault = value; } } ////// Notifies the ////// whether it is the default button so that it can adjust its appearance /// accordingly. /// /// /// /// /// protected override void OnClick(EventArgs e) { Form form = FindFormInternal(); if (form != null) form.DialogResult = dialogResult; // accessibility stuff // AccessibilityNotifyClients(AccessibleEvents.StateChange, -1); AccessibilityNotifyClients(AccessibleEvents.NameChange, -1); base.OnClick(e); } protected override void OnFontChanged(EventArgs e) { systemSize = new Size(Int32.MinValue, Int32.MinValue); base.OnFontChanged(e); } ////// This method actually raises the Click event. Inheriting classes should /// override this if they wish to be notified of a Click event. (This is far /// preferable to actually adding an event handler.) They should not, /// however, forget to call base.onClick(e); before exiting, to ensure that /// other recipients do actually get the event. /// /// ////// /// /// protected override void OnMouseUp(MouseEventArgs mevent) { if (mevent.Button == MouseButtons.Left && MouseIsPressed) { bool isMouseDown = base.MouseIsDown; if (GetStyle(ControlStyles.UserPaint)) { //Paint in raised state... ResetFlagsandPaint(); } if (isMouseDown) { Point pt = PointToScreen(new Point(mevent.X, mevent.Y)); if (UnsafeNativeMethods.WindowFromPoint(pt.X, pt.Y) == Handle && !ValidationCancelled) { if (GetStyle(ControlStyles.UserPaint)) { OnClick(mevent); } OnMouseClick(mevent); } } } base.OnMouseUp(mevent); } protected override void OnTextChanged(EventArgs e) { systemSize = new Size(Int32.MinValue, Int32.MinValue); base.OnTextChanged(e); } ////// Raises the ///event. /// /// /// /// public void PerformClick() { if (CanSelect) { bool validatedControlAllowsFocusChange; bool validate = ValidateActiveControl(out validatedControlAllowsFocusChange); if (!ValidationCancelled && (validate || validatedControlAllowsFocusChange)) { //Paint in raised state... // ResetFlagsandPaint(); OnClick(EventArgs.Empty); } } } ////// Generates a ///event for a /// button. /// /// /// /// [UIPermission(SecurityAction.LinkDemand, Window=UIPermissionWindow.AllWindows)] protected internal override bool ProcessMnemonic(char charCode) { if (UseMnemonic && CanProcessMnemonic() && IsMnemonic(charCode, Text)) { PerformClick(); return true; } return base.ProcessMnemonic(charCode); } ////// Lets a control process mnmemonic characters. Inheriting classes can /// override this to add extra functionality, but should not forget to call /// base.ProcessMnemonic(charCode); to ensure basic functionality /// remains unchanged. /// /// ////// /// /// public override string ToString() { string s = base.ToString(); return s + ", Text: " + Text; } ////// Provides some interesting information for the Button control in /// String form. /// ////// /// The button's window procedure. Inheriting classes can override this /// to add extra functionality, but should not forget to call /// base.wndProc(m); to ensure the button continues to function properly. /// ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected override void WndProc(ref Message m) { switch (m.Msg) { case NativeMethods.WM_REFLECT + NativeMethods.WM_COMMAND: if (NativeMethods.Util.HIWORD(m.WParam) == NativeMethods.BN_CLICKED) { Debug.Assert(!GetStyle(ControlStyles.UserPaint), "Shouldn't get BN_CLICKED when UserPaint"); if (!ValidationCancelled) { OnClick(EventArgs.Empty); } } break; case NativeMethods.WM_ERASEBKGND: DefWndProc(ref m); break; default: base.WndProc(ref m); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
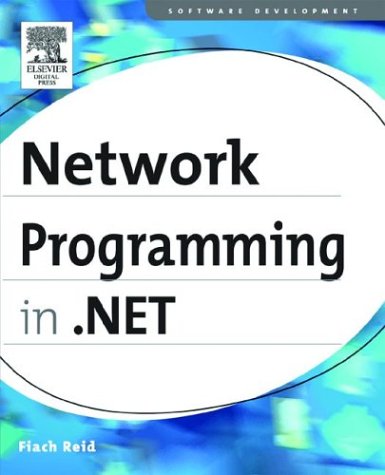
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberHolder.cs
- DataGridHeaderBorder.cs
- XdrBuilder.cs
- CompilerGlobalScopeAttribute.cs
- CalloutQueueItem.cs
- GridViewColumnHeader.cs
- Helpers.cs
- DataViewSetting.cs
- PropertyEmitter.cs
- AliasedExpr.cs
- RelatedEnd.cs
- Pkcs9Attribute.cs
- WebControlsSection.cs
- SplitterPanel.cs
- ListViewTableRow.cs
- ObjectDataSourceFilteringEventArgs.cs
- SoapReflectionImporter.cs
- EntityDataSourceStatementEditorForm.cs
- AnonymousIdentificationSection.cs
- SqlUDTStorage.cs
- ToolStripItemDesigner.cs
- TextBox.cs
- Trace.cs
- TreeView.cs
- PropertyConverter.cs
- WebPartDescriptionCollection.cs
- Stack.cs
- DtdParser.cs
- OverlappedContext.cs
- AudioDeviceOut.cs
- IndexedGlyphRun.cs
- TraceFilter.cs
- XmlSubtreeReader.cs
- XPathParser.cs
- MarkupCompilePass1.cs
- SessionIDManager.cs
- WebBrowser.cs
- Light.cs
- Trace.cs
- ResXResourceSet.cs
- ArgumentOutOfRangeException.cs
- DebugViewWriter.cs
- DataKey.cs
- lengthconverter.cs
- StrokeNodeData.cs
- HtmlTableRow.cs
- Literal.cs
- HtmlImageAdapter.cs
- ExpressionVisitorHelpers.cs
- base64Transforms.cs
- RelationshipDetailsRow.cs
- MouseCaptureWithinProperty.cs
- ConfigXmlSignificantWhitespace.cs
- ToolStripDropDownMenu.cs
- DiscoveryEndpointElement.cs
- SendingRequestEventArgs.cs
- HtmlString.cs
- InitializerFacet.cs
- SemanticBasicElement.cs
- SyndicationPerson.cs
- ListViewGroup.cs
- MenuCommand.cs
- CodePropertyReferenceExpression.cs
- WindowsSlider.cs
- BufferModesCollection.cs
- ElementNotAvailableException.cs
- ResXFileRef.cs
- MessageQueuePermissionEntry.cs
- TextInfo.cs
- Converter.cs
- BasePattern.cs
- Rijndael.cs
- ObjectTag.cs
- PrivilegeNotHeldException.cs
- KeyedHashAlgorithm.cs
- AnimationLayer.cs
- EditorPart.cs
- SoapAttributeAttribute.cs
- StorageMappingItemCollection.cs
- CqlLexer.cs
- SimpleLine.cs
- ToolboxComponentsCreatingEventArgs.cs
- DataRecordObjectView.cs
- DesignerCategoryAttribute.cs
- BitVector32.cs
- EnterpriseServicesHelper.cs
- BmpBitmapEncoder.cs
- Encoder.cs
- SortableBindingList.cs
- arabicshape.cs
- AutomationProperties.cs
- SweepDirectionValidation.cs
- SchemeSettingElement.cs
- DesignerSerializationVisibilityAttribute.cs
- VersionedStream.cs
- ZipIOLocalFileHeader.cs
- RequestSecurityToken.cs
- BaseCodeDomTreeGenerator.cs
- SystemException.cs
- RegexEditorDialog.cs