Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / SharedTcpTransportManager.cs / 1 / SharedTcpTransportManager.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.ServiceModel.Activation; using System.Collections.Generic; using System.Diagnostics; using System.ServiceModel; using System.Threading; using System.ServiceModel.Diagnostics; class SharedTcpTransportManager : TcpTransportManager, ITransportManagerRegistration { SharedConnectionListener listener; ConnectionDemuxer connectionDemuxer; HostNameComparisonMode hostNameComparisonMode; Uri listenUri; int queueId; Guid token; OnDuplicatedViaDelegate onDuplicatedViaCallback; bool demuxerCreated; public SharedTcpTransportManager(Uri listenUri, TcpChannelListener channelListener) { this.HostNameComparisonMode = channelListener.HostNameComparisonMode; this.listenUri = listenUri; // For port sharing, we apply all of the settings from channel listener to the transport manager. this.ApplyListenerSettings(channelListener); } protected SharedTcpTransportManager(Uri listenUri) { this.listenUri = listenUri; } protected override bool IsCompatible(TcpChannelListener channelListener) { if (channelListener.HostedVirtualPath == null && !channelListener.PortSharingEnabled) { return false; } return base.IsCompatible(channelListener); } public HostNameComparisonMode HostNameComparisonMode { get { return this.hostNameComparisonMode; } set { HostNameComparisonModeHelper.Validate(value); lock (base.ThisLock) { ThrowIfOpen(); this.hostNameComparisonMode = value; } } } public Uri ListenUri { get { return this.listenUri; } } internal override void OnOpen() { OnOpenInternal(0, Guid.Empty); } protected virtual OnViaDelegate GetOnViaCallback() { return null; } // This method is called only for the first via of the current proxy. void OnDuplicatedVia(Uri via, out int connectionBufferSize) { OnViaDelegate onVia = GetOnViaCallback(); if (onVia != null) { onVia(via); } if (!demuxerCreated) { lock (ThisLock) { if (listener == null) { // The listener has been stopped. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new CommunicationObjectAbortedException( SR.GetString(SR.Sharing_ListenerProxyStopped))); } if (!demuxerCreated) { CreateConnectionDemuxer(); demuxerCreated = true; } } } connectionBufferSize = this.ConnectionBufferSize; } void CreateConnectionDemuxer() { IConnectionListener connectionListener = new BufferedConnectionListener(listener, MaxOutputDelay, ConnectionBufferSize); if (DiagnosticUtility.ShouldUseActivity) { connectionListener = new TracingConnectionListener(connectionListener, this.ListenUri); } connectionDemuxer = new ConnectionDemuxer(connectionListener, MaxPendingAccepts, MaxPendingConnections, ChannelInitializationTimeout, IdleTimeout, MaxPooledConnections, OnGetTransportFactorySettings, OnGetSingletonMessageHandler, OnHandleServerSessionPreamble, OnDemuxerError); connectionDemuxer.StartDemuxing(this.GetOnViaCallback()); } internal void OnOpenInternal(int queueId, Guid token) { lock (ThisLock) { this.queueId = queueId; this.token = token; BaseUriWithWildcard path = new BaseUriWithWildcard(this.ListenUri, this.HostNameComparisonMode); if (this.onDuplicatedViaCallback == null) { this.onDuplicatedViaCallback = new OnDuplicatedViaDelegate(OnDuplicatedVia); } listener = new SharedConnectionListener(path, queueId, token, this.onDuplicatedViaCallback); // Delay the creation of the demuxer on the first request. } } protected void CleanUp() { lock (ThisLock) { if (listener != null) { listener.Stop(); // The listener will be closed by the demuxer. listener = null; } if (connectionDemuxer != null) { connectionDemuxer.Dispose(); } demuxerCreated = false; } } internal override void OnClose() { CleanUp(); TcpChannelListener.StaticTransportManagerTable.UnregisterUri(this.ListenUri, this.HostNameComparisonMode); } protected virtual void OnSelecting(TcpChannelListener channelListener) { } IListITransportManagerRegistration.Select(TransportChannelListener channelListener) { if (!channelListener.IsScopeIdCompatible(this.hostNameComparisonMode, this.listenUri)) { return null; } OnSelecting((TcpChannelListener)channelListener); IList result = null; if (this.IsCompatible((TcpChannelListener)channelListener)) { result = new List (); result.Add(this); } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
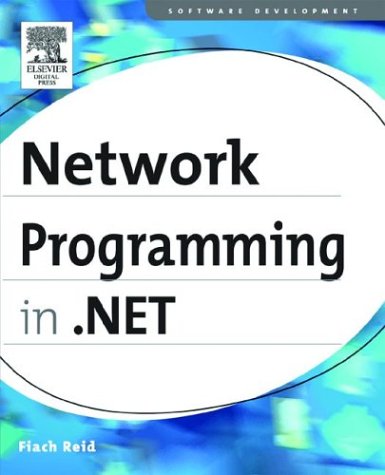
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripItemImageRenderEventArgs.cs
- X509Certificate.cs
- BinaryNode.cs
- NativeCppClassAttribute.cs
- XslVisitor.cs
- EqualityArray.cs
- httpapplicationstate.cs
- Source.cs
- SoapObjectReader.cs
- TraceListeners.cs
- FocusWithinProperty.cs
- SQLInt64.cs
- ECDsaCng.cs
- ScriptBehaviorDescriptor.cs
- PersonalizationStateInfo.cs
- MembershipPasswordException.cs
- DataBindingExpressionBuilder.cs
- WindowsListViewItemStartMenu.cs
- DynamicDataResources.Designer.cs
- AdPostCacheSubstitution.cs
- GroupedContextMenuStrip.cs
- AssociationTypeEmitter.cs
- Label.cs
- OleStrCAMarshaler.cs
- HtmlShimManager.cs
- EventLogPermissionEntryCollection.cs
- CodeMemberEvent.cs
- XmlReflectionImporter.cs
- DocumentScope.cs
- ServiceNotStartedException.cs
- FlowDocument.cs
- InputLangChangeRequestEvent.cs
- TimeSpanOrInfiniteValidator.cs
- Comparer.cs
- SQLResource.cs
- ControlCommandSet.cs
- DocumentStream.cs
- ListViewItem.cs
- DictionaryManager.cs
- ErrorRuntimeConfig.cs
- XmlQuerySequence.cs
- InkSerializer.cs
- ScrollBar.cs
- ConsumerConnectionPoint.cs
- Ticks.cs
- HandoffBehavior.cs
- TaskExtensions.cs
- PrimaryKeyTypeConverter.cs
- OperationAbortedException.cs
- ServerValidateEventArgs.cs
- Symbol.cs
- DataServiceHostFactory.cs
- RegistrationServices.cs
- ApplicationServiceHelper.cs
- CultureSpecificStringDictionary.cs
- ViewSimplifier.cs
- HttpCachePolicyElement.cs
- DatatypeImplementation.cs
- SchemaComplexType.cs
- Int16Animation.cs
- CookieProtection.cs
- PeerServiceMessageContracts.cs
- RemoteHelper.cs
- AsymmetricKeyExchangeDeformatter.cs
- TimeSpanValidatorAttribute.cs
- JobCollate.cs
- PrintDialog.cs
- MemberCollection.cs
- ResourcePart.cs
- ItemsChangedEventArgs.cs
- DocumentPageView.cs
- DispatcherExceptionEventArgs.cs
- CurrentChangingEventManager.cs
- MetadataArtifactLoader.cs
- HostedNamedPipeTransportManager.cs
- Grant.cs
- MessageRpc.cs
- _SpnDictionary.cs
- SymbolMethod.cs
- RegionIterator.cs
- OneToOneMappingSerializer.cs
- ClassicBorderDecorator.cs
- glyphs.cs
- RemoteWebConfigurationHostStream.cs
- BinaryNode.cs
- CharacterHit.cs
- DataTableExtensions.cs
- HashRepartitionStream.cs
- QueryResponse.cs
- FieldToken.cs
- TableCellCollection.cs
- MultipleViewProviderWrapper.cs
- UntrustedRecipientException.cs
- basecomparevalidator.cs
- TextParagraphView.cs
- SemaphoreFullException.cs
- EncoderNLS.cs
- MultiBinding.cs
- ConstructorBuilder.cs
- TextParagraphView.cs