Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / GroupedContextMenuStrip.cs / 1 / GroupedContextMenuStrip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System; using System.Collections.Generic; using System.Text; using System.Collections.Specialized; using System.Windows.Forms; using System.ComponentModel; /* This is the Custom ContextMenu thathas the notion of groups and groupOrdering. The contrextmenu is divided into groups and the ordering is governed by the groupOrdering. The Groups contain individual menuItems. */ internal class GroupedContextMenuStrip : ContextMenuStrip { private StringCollection groupOrdering; private ContextMenuStripGroupCollection groups; private bool populated = false; public bool Populated { set { populated = value; } } public GroupedContextMenuStrip() { } public ContextMenuStripGroupCollection Groups { get { if (groups == null) { groups = new ContextMenuStripGroupCollection(); } return groups; } } public StringCollection GroupOrdering { get { if (groupOrdering == null) { groupOrdering = new StringCollection(); } return groupOrdering; } } // merges all the items which are currently in the groups into the items collection. public void Populate() { this.Items.Clear(); foreach (string groupName in GroupOrdering) { if (groups.ContainsKey(groupName)) { Listitems = groups[groupName].Items; if (Items.Count > 0 && items.Count > 0) { this.Items.Add(new ToolStripSeparator()); } foreach (ToolStripItem item in items) { this.Items.Add(item); } } } populated = true; } protected override void OnOpening(CancelEventArgs e) { SuspendLayout(); if (!populated) { Populate(); } RefreshItems(); ResumeLayout(true); PerformLayout(); e.Cancel = (Items.Count == 0); base.OnOpening(e); } public virtual void RefreshItems() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
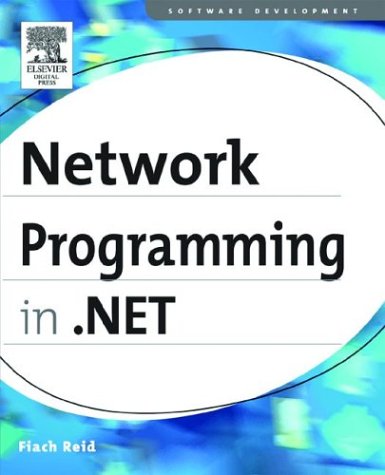
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaSimpleType.cs
- GridViewRowCollection.cs
- ProxyHelper.cs
- QueryExpr.cs
- SqlDataAdapter.cs
- ClientConfigurationSystem.cs
- StringDictionaryWithComparer.cs
- LoginViewDesigner.cs
- CompileLiteralTextParser.cs
- Win32.cs
- FirewallWrapper.cs
- GridViewCancelEditEventArgs.cs
- CodeDOMProvider.cs
- selecteditemcollection.cs
- XhtmlBasicImageAdapter.cs
- RecordConverter.cs
- SynchronizationContext.cs
- UdpChannelListener.cs
- ReplyAdapterChannelListener.cs
- DBCommandBuilder.cs
- ListViewItemSelectionChangedEvent.cs
- EncryptedType.cs
- SurrogateEncoder.cs
- NativeMethods.cs
- FormsAuthenticationModule.cs
- WindowsRichEditRange.cs
- ReadOnlyAttribute.cs
- RtfToXamlLexer.cs
- ObjectStorage.cs
- EventMappingSettings.cs
- ThreadStartException.cs
- PowerStatus.cs
- BinaryParser.cs
- XmlSerializerAssemblyAttribute.cs
- ExtensionSurface.cs
- HtmlInputHidden.cs
- DataObject.cs
- StreamWithDictionary.cs
- TextHintingModeValidation.cs
- UnsettableComboBox.cs
- XmlQueryRuntime.cs
- Scene3D.cs
- WebServiceTypeData.cs
- DateTimeUtil.cs
- XmlChildEnumerator.cs
- RegexCompilationInfo.cs
- SqlMetaData.cs
- CreateSequenceResponse.cs
- InputScopeNameConverter.cs
- WebPartUserCapability.cs
- WindowsRebar.cs
- GPStream.cs
- Win32.cs
- LinkButton.cs
- Soap12ProtocolImporter.cs
- serverconfig.cs
- SizeAnimationUsingKeyFrames.cs
- CryptoStream.cs
- ResourceProperty.cs
- PageTheme.cs
- KeyToListMap.cs
- PropertyFilterAttribute.cs
- TrustSection.cs
- BitmapEditor.cs
- TraceHandlerErrorFormatter.cs
- RecognizedPhrase.cs
- ErasingStroke.cs
- XXXInfos.cs
- Symbol.cs
- DeviceFilterEditorDialog.cs
- CheckBoxPopupAdapter.cs
- IdentityHolder.cs
- MsmqInputSessionChannelListener.cs
- ScrollableControl.cs
- SafeEventLogWriteHandle.cs
- CompoundFileStorageReference.cs
- SourceElementsCollection.cs
- Int16.cs
- NameValueFileSectionHandler.cs
- PrincipalPermission.cs
- StatusBarPanel.cs
- MD5CryptoServiceProvider.cs
- MatrixConverter.cs
- HttpApplicationFactory.cs
- UserPreferenceChangingEventArgs.cs
- Block.cs
- SystemIPv6InterfaceProperties.cs
- XmlCharCheckingWriter.cs
- WasHostedComPlusFactory.cs
- WorkflowInstanceExtensionCollection.cs
- Matrix.cs
- FigureParagraph.cs
- CurrentChangingEventArgs.cs
- EventDrivenDesigner.cs
- Point4DValueSerializer.cs
- PerfCounters.cs
- DataBoundControlHelper.cs
- PenCursorManager.cs
- EffectiveValueEntry.cs
- CdpEqualityComparer.cs