Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / EncryptedType.cs / 1 / EncryptedType.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using System.IO; using System.ServiceModel.Channels; using System.ServiceModel; using System.IdentityModel.Tokens; using System.IdentityModel.Selectors; using System.Security.Cryptography; using System.Text; using System.Xml; using DictionaryManager = System.IdentityModel.DictionaryManager; using SignedXml = System.IdentityModel.SignedXml; using ISecurityElement = System.IdentityModel.ISecurityElement; abstract class EncryptedType : ISecurityElement { internal static readonly XmlDictionaryString NamespaceUri = XD.XmlEncryptionDictionary.Namespace; internal static readonly XmlDictionaryString EncodingAttribute = XD.XmlEncryptionDictionary.Encoding; internal static readonly XmlDictionaryString MimeTypeAttribute = XD.XmlEncryptionDictionary.MimeType; internal static readonly XmlDictionaryString TypeAttribute = XD.XmlEncryptionDictionary.Type; internal static readonly XmlDictionaryString CipherDataElementName = XD.XmlEncryptionDictionary.CipherData; internal static readonly XmlDictionaryString CipherValueElementName = XD.XmlEncryptionDictionary.CipherValue; string encoding; EncryptionMethodElement encryptionMethod; string id; string wsuId; SecurityKeyIdentifier keyIdentifier; string mimeType; EncryptionState state; string type; SecurityTokenSerializer tokenSerializer; protected EncryptedType() { this.encryptionMethod.Init(); this.state = EncryptionState.New; this.tokenSerializer = SecurityStandardsManager.DefaultInstance.SecurityTokenSerializer; } public string Encoding { get { return this.encoding; } set { this.encoding = value; } } public string EncryptionMethod { get { return this.encryptionMethod.algorithm; } set { this.encryptionMethod.algorithm = value; } } public XmlDictionaryString EncryptionMethodDictionaryString { get { return this.encryptionMethod.algorithmDictionaryString; } set { this.encryptionMethod.algorithmDictionaryString = value; } } public bool HasId { get { return true; } } public string Id { get { return this.id; } set { this.id = value; } } public string WsuId { get { return this.wsuId; } set { this.wsuId = value; } } public SecurityKeyIdentifier KeyIdentifier { get { return this.keyIdentifier; } set { this.keyIdentifier = value; } } public string MimeType { get { return this.mimeType; } set { this.mimeType = value; } } public string Type { get { return this.type; } set { this.type = value; } } protected abstract XmlDictionaryString OpeningElementName { get; } protected EncryptionState State { get { return this.state; } set { this.state = value; } } public SecurityTokenSerializer SecurityTokenSerializer { get { return this.tokenSerializer; } set { this.tokenSerializer = value ?? WSSecurityTokenSerializer.DefaultInstance; } } protected abstract void ForceEncryption(); protected virtual void ReadAdditionalAttributes(XmlDictionaryReader reader) { } protected virtual void ReadAdditionalElements(XmlDictionaryReader reader) { } protected abstract void ReadCipherData(XmlDictionaryReader reader); protected abstract void ReadCipherData(XmlDictionaryReader reader, long maxBufferSize); public void ReadFrom(XmlReader reader) { ReadFrom(reader, 0); } public void ReadFrom(XmlDictionaryReader reader) { ReadFrom(reader, 0); } public void ReadFrom(XmlReader reader, long maxBufferSize) { ReadFrom(XmlDictionaryReader.CreateDictionaryReader(reader), maxBufferSize); } public void ReadFrom(XmlDictionaryReader reader, long maxBufferSize) { ValidateReadState(); reader.MoveToStartElement(OpeningElementName, NamespaceUri); this.encoding = reader.GetAttribute(EncodingAttribute, null); this.id = reader.GetAttribute(XD.XmlEncryptionDictionary.Id, null) ?? SecurityUniqueId.Create().Value; this.wsuId = reader.GetAttribute(XD.XmlEncryptionDictionary.Id, XD.UtilityDictionary.Namespace) ?? SecurityUniqueId.Create().Value; this.mimeType = reader.GetAttribute(MimeTypeAttribute, null); this.type = reader.GetAttribute(TypeAttribute, null); ReadAdditionalAttributes(reader); reader.Read(); if (reader.IsStartElement(EncryptionMethodElement.ElementName, NamespaceUri)) { this.encryptionMethod.ReadFrom(reader); } if (this.tokenSerializer.CanReadKeyIdentifier(reader)) { this.KeyIdentifier = this.tokenSerializer.ReadKeyIdentifier(reader); } reader.ReadStartElement(CipherDataElementName, EncryptedType.NamespaceUri); reader.ReadStartElement(CipherValueElementName, EncryptedType.NamespaceUri); if (maxBufferSize == 0) ReadCipherData(reader); else ReadCipherData(reader, maxBufferSize); reader.ReadEndElement(); // CipherValue reader.ReadEndElement(); // CipherData ReadAdditionalElements(reader); reader.ReadEndElement(); // OpeningElementName this.State = EncryptionState.Read; } protected virtual void WriteAdditionalAttributes(XmlDictionaryWriter writer) { } protected virtual void WriteAdditionalElements(XmlDictionaryWriter writer) { } protected abstract void WriteCipherData(XmlDictionaryWriter writer); public void WriteTo(XmlDictionaryWriter writer, DictionaryManager dictionaryManager) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } ValidateWriteState(); writer.WriteStartElement(XmlEncryptionStrings.Prefix, this.OpeningElementName, NamespaceUri); if (this.id != null && this.id.Length != 0) { writer.WriteAttributeString(XD.XmlEncryptionDictionary.Id, null, this.Id); } if (this.type != null) { writer.WriteAttributeString(TypeAttribute, null, this.Type); } if (this.mimeType != null) { writer.WriteAttributeString(MimeTypeAttribute, null, this.MimeType); } if (this.encoding != null) { writer.WriteAttributeString(EncodingAttribute, null, this.Encoding); } WriteAdditionalAttributes(writer); if (this.encryptionMethod.algorithm != null) { this.encryptionMethod.WriteTo(writer); } if (this.KeyIdentifier != null) { this.tokenSerializer.WriteKeyIdentifier(writer, this.KeyIdentifier); } writer.WriteStartElement(CipherDataElementName, NamespaceUri); writer.WriteStartElement(CipherValueElementName, NamespaceUri); WriteCipherData(writer); writer.WriteEndElement(); // CipherValue writer.WriteEndElement(); // CipherData WriteAdditionalElements(writer); writer.WriteEndElement(); // OpeningElementName } void ValidateReadState() { if (this.State != EncryptionState.New) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(SR.GetString(SR.BadEncryptionState))); } } void ValidateWriteState() { if (this.State == EncryptionState.EncryptionSetup) { ForceEncryption(); } else if (this.State == EncryptionState.New) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(SR.GetString(SR.BadEncryptionState))); } } protected enum EncryptionState { New, Read, DecryptionSetup, Decrypted, EncryptionSetup, Encrypted } struct EncryptionMethodElement { internal string algorithm; internal XmlDictionaryString algorithmDictionaryString; internal static readonly XmlDictionaryString ElementName = XD.XmlEncryptionDictionary.EncryptionMethod; public void Init() { this.algorithm = null; } public void ReadFrom(XmlDictionaryReader reader) { reader.MoveToStartElement(ElementName, XD.XmlEncryptionDictionary.Namespace); bool isEmptyElement = reader.IsEmptyElement; this.algorithm = reader.GetAttribute(XD.XmlSignatureDictionary.Algorithm, null); if (this.algorithm == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException( SR.GetString(SR.RequiredAttributeMissing, XD.XmlSignatureDictionary.Algorithm.Value, ElementName.Value))); } reader.Read(); if (!isEmptyElement) { while (reader.IsStartElement()) { reader.Skip(); } reader.ReadEndElement(); } } public void WriteTo(XmlDictionaryWriter writer) { writer.WriteStartElement(XmlEncryptionStrings.Prefix, ElementName, XD.XmlEncryptionDictionary.Namespace); if (this.algorithmDictionaryString != null) { writer.WriteStartAttribute(XD.XmlSignatureDictionary.Algorithm, null); writer.WriteString(this.algorithmDictionaryString); writer.WriteEndAttribute(); } else { writer.WriteAttributeString(XD.XmlSignatureDictionary.Algorithm, null, this.algorithm); } if (this.algorithm == XD.SecurityAlgorithmDictionary.RsaOaepKeyWrap.Value) { writer.WriteStartElement(XmlSignatureStrings.Prefix, XD.XmlSignatureDictionary.DigestMethod, XD.XmlSignatureDictionary.Namespace); writer.WriteStartAttribute(XD.XmlSignatureDictionary.Algorithm, null); writer.WriteString(XD.SecurityAlgorithmDictionary.Sha1Digest); writer.WriteEndAttribute(); writer.WriteEndElement(); } writer.WriteEndElement(); // EncryptionMethod } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
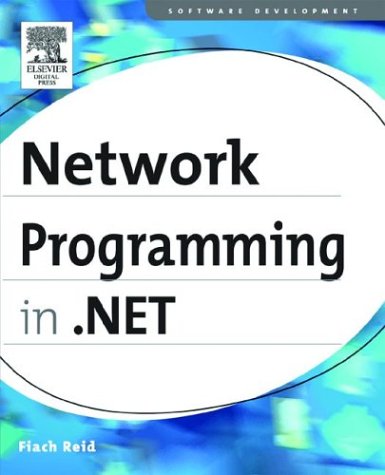
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeConstructor.cs
- NativeBuffer.cs
- SortAction.cs
- GridEntryCollection.cs
- filewebrequest.cs
- PrivateFontCollection.cs
- CounterSetInstance.cs
- DesignerDataColumn.cs
- MarkupExtensionParser.cs
- TypeNameParser.cs
- RightsManagementUser.cs
- TreeNode.cs
- EntitySqlQueryBuilder.cs
- HttpServerUtilityBase.cs
- BooleanSwitch.cs
- GetMemberBinder.cs
- DoWhileDesigner.xaml.cs
- JournalEntryListConverter.cs
- DbProviderServices.cs
- WindowsEditBox.cs
- TrustManager.cs
- SchemaHelper.cs
- DataServiceKeyAttribute.cs
- BitmapFrameEncode.cs
- LicenseManager.cs
- querybuilder.cs
- ConversionContext.cs
- ReadOnlyNameValueCollection.cs
- EntityDataSourceColumn.cs
- Visitors.cs
- BitmapFrameEncode.cs
- StrokeCollection.cs
- BaseTemplatedMobileComponentEditor.cs
- CommandConverter.cs
- StoreContentChangedEventArgs.cs
- WebControl.cs
- ToolStripItemClickedEventArgs.cs
- WarningException.cs
- RequestCacheValidator.cs
- AutomationElement.cs
- TimeIntervalCollection.cs
- Select.cs
- SecuritySessionClientSettings.cs
- FieldAccessException.cs
- TemplatePropertyEntry.cs
- HandleCollector.cs
- Certificate.cs
- StructuralType.cs
- Repeater.cs
- SqlError.cs
- ListChunk.cs
- SapiGrammar.cs
- LocationUpdates.cs
- ActivitySurrogate.cs
- IndexerNameAttribute.cs
- NotifyIcon.cs
- ReferencedAssembly.cs
- TextPattern.cs
- ContainerUIElement3D.cs
- QilSortKey.cs
- GraphicsContainer.cs
- _ConnectOverlappedAsyncResult.cs
- NavigateEvent.cs
- CollectionDataContract.cs
- BlobPersonalizationState.cs
- ToolStripDropDownButton.cs
- JavascriptCallbackMessageInspector.cs
- SmiRequestExecutor.cs
- DynamicField.cs
- SqlProviderUtilities.cs
- ActiveDocumentEvent.cs
- MetadataUtil.cs
- WaitForChangedResult.cs
- WebPartZoneCollection.cs
- SqlProviderManifest.cs
- RestHandlerFactory.cs
- TransactionChannelFactory.cs
- ProviderSettingsCollection.cs
- UnmanagedHandle.cs
- EventPropertyMap.cs
- EntityTypeEmitter.cs
- Menu.cs
- QueryResponse.cs
- PrinterSettings.cs
- BlockUIContainer.cs
- GridViewRowCollection.cs
- DbCommandTree.cs
- StaticContext.cs
- EntityTransaction.cs
- DPCustomTypeDescriptor.cs
- DataControlFieldCell.cs
- ItemPager.cs
- IPCCacheManager.cs
- MatrixKeyFrameCollection.cs
- RemoteWebConfigurationHostServer.cs
- RemotingException.cs
- ProfileInfo.cs
- TemplateControlCodeDomTreeGenerator.cs
- CryptoHandle.cs
- ProfileServiceManager.cs