Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / SqlClient / SqlGen / Symbol.cs / 2 / Symbol.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// internal class Symbol : ISqlFragment { private Dictionary/// This class represents an extent/nested select statement, /// or a column. /// /// The important fields are Name, Type and NewName. /// NewName starts off the same as Name, and is then modified as necessary. /// /// /// The rest are used by special symbols. /// e.g. NeedsRenaming is used by columns to indicate that a new name must /// be picked for the column in the second phase of translation. /// /// IsUnnest is used by symbols for a collection expression used as a from clause. /// This allows to add the column list /// after the alias. /// /// columns; internal Dictionary Columns { get { if (null == columns) { columns = new Dictionary (StringComparer.OrdinalIgnoreCase); } return columns; } } private bool needsRenaming; internal bool NeedsRenaming { get { return needsRenaming; } set { needsRenaming = value; } } private bool outputColumnsRenamed; internal bool OutputColumnsRenamed { get { return outputColumnsRenamed; } set { outputColumnsRenamed = value; } } private string name; public string Name { get { return name; } } private string newName; public string NewName { get { return newName; } set { newName = value; } } private TypeUsage type; internal TypeUsage Type { get { return type; } set { type = value; } } public Symbol(string name, TypeUsage type) { this.name = name; this.newName = name; this.Type = type; } /// /// Use this constructor the symbol represents a SqlStatement with renamed output columns. /// /// /// /// public Symbol(string name, TypeUsage type, Dictionarycolumns) { this.name = name; this.newName = name; this.Type = type; this.columns = columns; this.OutputColumnsRenamed = true; } #region ISqlFragment Members /// /// Write this symbol out as a string for sql. This is just /// the new name of the symbol (which could be the same as the old name). /// /// We rename columns here if necessary. /// /// /// public void WriteSql(SqlWriter writer, SqlGenerator sqlGenerator) { if (this.NeedsRenaming) { int i; if (sqlGenerator.AllColumnNames.TryGetValue(this.NewName, out i)) { string newNameCandidate; do { ++i; newNameCandidate = this.NewName + i.ToString(System.Globalization.CultureInfo.InvariantCulture); } while (sqlGenerator.AllColumnNames.ContainsKey(newNameCandidate)); sqlGenerator.AllColumnNames[this.NewName] = i; this.NewName = newNameCandidate; } // Add this column name to list of known names so that there are no subsequent // collisions sqlGenerator.AllColumnNames[this.NewName] = 0; // Prevent it from being renamed repeatedly. this.NeedsRenaming = false; } writer.Write(SqlGenerator.QuoteIdentifier(this.NewName)); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// internal class Symbol : ISqlFragment { private Dictionary/// This class represents an extent/nested select statement, /// or a column. /// /// The important fields are Name, Type and NewName. /// NewName starts off the same as Name, and is then modified as necessary. /// /// /// The rest are used by special symbols. /// e.g. NeedsRenaming is used by columns to indicate that a new name must /// be picked for the column in the second phase of translation. /// /// IsUnnest is used by symbols for a collection expression used as a from clause. /// This allows to add the column list /// after the alias. /// /// columns; internal Dictionary Columns { get { if (null == columns) { columns = new Dictionary (StringComparer.OrdinalIgnoreCase); } return columns; } } private bool needsRenaming; internal bool NeedsRenaming { get { return needsRenaming; } set { needsRenaming = value; } } private bool outputColumnsRenamed; internal bool OutputColumnsRenamed { get { return outputColumnsRenamed; } set { outputColumnsRenamed = value; } } private string name; public string Name { get { return name; } } private string newName; public string NewName { get { return newName; } set { newName = value; } } private TypeUsage type; internal TypeUsage Type { get { return type; } set { type = value; } } public Symbol(string name, TypeUsage type) { this.name = name; this.newName = name; this.Type = type; } /// /// Use this constructor the symbol represents a SqlStatement with renamed output columns. /// /// /// /// public Symbol(string name, TypeUsage type, Dictionarycolumns) { this.name = name; this.newName = name; this.Type = type; this.columns = columns; this.OutputColumnsRenamed = true; } #region ISqlFragment Members /// /// Write this symbol out as a string for sql. This is just /// the new name of the symbol (which could be the same as the old name). /// /// We rename columns here if necessary. /// /// /// public void WriteSql(SqlWriter writer, SqlGenerator sqlGenerator) { if (this.NeedsRenaming) { int i; if (sqlGenerator.AllColumnNames.TryGetValue(this.NewName, out i)) { string newNameCandidate; do { ++i; newNameCandidate = this.NewName + i.ToString(System.Globalization.CultureInfo.InvariantCulture); } while (sqlGenerator.AllColumnNames.ContainsKey(newNameCandidate)); sqlGenerator.AllColumnNames[this.NewName] = i; this.NewName = newNameCandidate; } // Add this column name to list of known names so that there are no subsequent // collisions sqlGenerator.AllColumnNames[this.NewName] = 0; // Prevent it from being renamed repeatedly. this.NeedsRenaming = false; } writer.Write(SqlGenerator.QuoteIdentifier(this.NewName)); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
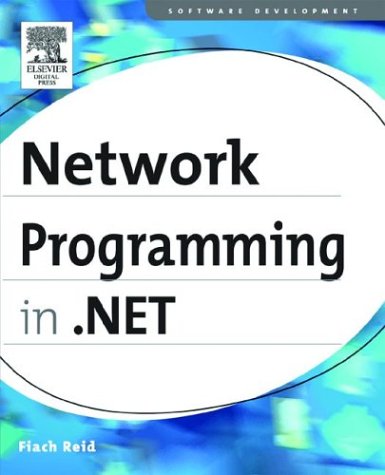
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SharedPersonalizationStateInfo.cs
- DataGridViewHeaderCell.cs
- MenuItemStyleCollectionEditor.cs
- TrackingServices.cs
- SizeConverter.cs
- SerialStream.cs
- VectorCollection.cs
- SecurityDescriptor.cs
- FixedHyperLink.cs
- PointCollectionValueSerializer.cs
- UseManagedPresentationBindingElement.cs
- XmlDataDocument.cs
- SubtreeProcessor.cs
- RequiredFieldValidator.cs
- TextEditorLists.cs
- IUnknownConstantAttribute.cs
- Nodes.cs
- WebPartChrome.cs
- ToolStripSplitStackLayout.cs
- AuthorizationSection.cs
- XamlWriter.cs
- BindMarkupExtensionSerializer.cs
- StructuredTypeInfo.cs
- Util.cs
- BulletedListDesigner.cs
- HtmlImage.cs
- SchemaEntity.cs
- UDPClient.cs
- CreateUserWizardStep.cs
- TdsValueSetter.cs
- ellipse.cs
- ResumeStoryboard.cs
- EdmItemError.cs
- SerializationException.cs
- NotifyInputEventArgs.cs
- PointConverter.cs
- ProvidersHelper.cs
- DataGridViewCheckBoxColumn.cs
- SourceFileInfo.cs
- TokenizerHelper.cs
- ApplyImportsAction.cs
- SmtpDigestAuthenticationModule.cs
- XmlStreamNodeWriter.cs
- HtmlTitle.cs
- WebPartConnectionsDisconnectVerb.cs
- DefaultValueMapping.cs
- ToolStripGrip.cs
- MenuStrip.cs
- OrderedDictionaryStateHelper.cs
- CachedFontFace.cs
- Timer.cs
- TypedReference.cs
- CollectionViewGroup.cs
- FontDriver.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- SmiRequestExecutor.cs
- RowToParametersTransformer.cs
- ComboBoxAutomationPeer.cs
- Helper.cs
- LicenseException.cs
- FileAuthorizationModule.cs
- RegexCharClass.cs
- TextElementCollection.cs
- OracleParameter.cs
- UseLicense.cs
- GroupAggregateExpr.cs
- XmlRootAttribute.cs
- JoinTreeNode.cs
- localization.cs
- ItemsChangedEventArgs.cs
- AvtEvent.cs
- remotingproxy.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- RegularExpressionValidator.cs
- ActiveXSite.cs
- ValueQuery.cs
- SecurityTokenTypes.cs
- ComPlusThreadInitializer.cs
- NegotiationTokenAuthenticator.cs
- CacheForPrimitiveTypes.cs
- AssemblyName.cs
- RuntimeDelegateArgument.cs
- PauseStoryboard.cs
- DataTableExtensions.cs
- TypeForwardedToAttribute.cs
- ApplicationServicesHostFactory.cs
- XmlReader.cs
- RectangleHotSpot.cs
- Attribute.cs
- DataGridViewRowCollection.cs
- ArrayEditor.cs
- DesignerDataColumn.cs
- CookieParameter.cs
- PolicyException.cs
- PropertyToken.cs
- ServiceContractListItemList.cs
- PopupRootAutomationPeer.cs
- IPAddress.cs
- LocalizableAttribute.cs
- WebPartConnectionsCancelEventArgs.cs