Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Collections / Generic / Comparer.cs / 1 / Comparer.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Collections; using System.Collections.Generic; namespace System.Collections.Generic { using System.Globalization; using System.Runtime.CompilerServices; [Serializable()] [TypeDependencyAttribute("System.Collections.Generic.GenericComparer`1")] public abstract class Comparer: IComparer, IComparer { static Comparer defaultComparer; public static Comparer Default { get { Comparer comparer = defaultComparer; if (comparer == null) { comparer = CreateComparer(); defaultComparer = comparer; } return comparer; } } private static Comparer CreateComparer() { Type t = typeof(T); // If T implements IComparable return a GenericComparer if (typeof(IComparable ).IsAssignableFrom(t)) { //return (Comparer )Activator.CreateInstance(typeof(GenericComparer<>).MakeGenericType(t)); return (Comparer )(typeof(GenericComparer ).TypeHandle.CreateInstanceForAnotherGenericParameter(t)); } // If T is a Nullable where U implements IComparable return a NullableComparer if (t.IsGenericType && t.GetGenericTypeDefinition() == typeof(Nullable<>)) { Type u = t.GetGenericArguments()[0]; if (typeof(IComparable<>).MakeGenericType(u).IsAssignableFrom(u)) { //return (Comparer )Activator.CreateInstance(typeof(NullableComparer<>).MakeGenericType(u)); return (Comparer )(typeof(NullableComparer ).TypeHandle.CreateInstanceForAnotherGenericParameter(u)); } } // Otherwise return an ObjectComparer return new ObjectComparer (); } public abstract int Compare(T x, T y); int IComparer.Compare(object x, object y) { if (x == null) return y == null ? 0 : -1; if (y == null) return 1; if (x is T && y is T) return Compare((T)x, (T)y); ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_InvalidArgumentForComparison); return 0; } } [Serializable()] internal class GenericComparer : Comparer where T: IComparable { public override int Compare(T x, T y) { if (x != null) { if (y != null) return x.CompareTo(y); return 1; } if (y != null) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ GenericComparer comparer = obj as GenericComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable()] internal class NullableComparer : Comparer > where T : struct, IComparable { public override int Compare(Nullable x, Nullable y) { if (x.HasValue) { if (y.HasValue) return x.value.CompareTo(y.value); return 1; } if (y.HasValue) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ NullableComparer comparer = obj as NullableComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable()] internal class ObjectComparer : Comparer { public override int Compare(T x, T y) { return System.Collections.Comparer.Default.Compare(x, y); } // Equals method for the comparer itself. public override bool Equals(Object obj){ ObjectComparer comparer = obj as ObjectComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } }
Link Menu
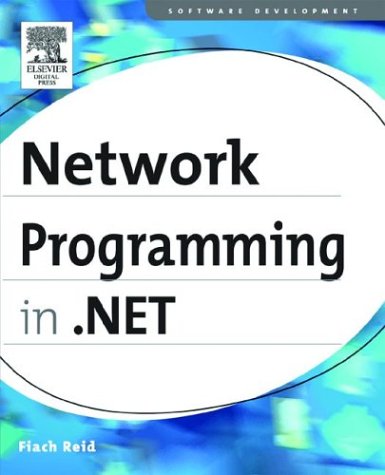
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509CertificateValidator.cs
- IMembershipProvider.cs
- CacheDependency.cs
- NavigatorInput.cs
- filewebresponse.cs
- MultiBinding.cs
- StringValidator.cs
- WebHttpDispatchOperationSelector.cs
- XmlLoader.cs
- ListMarkerLine.cs
- PathTooLongException.cs
- DataGridViewAutoSizeModeEventArgs.cs
- Certificate.cs
- CfgRule.cs
- COAUTHINFO.cs
- CreateUserWizardStep.cs
- HttpFormatExtensions.cs
- ConfigurationElementProperty.cs
- ResourceProperty.cs
- HttpRuntime.cs
- Assembly.cs
- DBCommand.cs
- WorkflowEventArgs.cs
- XamlTreeBuilder.cs
- XmlEntity.cs
- EntitySqlQueryBuilder.cs
- AutoGeneratedField.cs
- HtmlTextArea.cs
- DelegateCompletionCallbackWrapper.cs
- QueryStringHandler.cs
- BindingManagerDataErrorEventArgs.cs
- InfoCardSymmetricCrypto.cs
- DrawingCollection.cs
- WebPartConnectionsCancelEventArgs.cs
- GridViewRow.cs
- AddDataControlFieldDialog.cs
- StreamInfo.cs
- FlowDocumentPaginator.cs
- InvalidCommandTreeException.cs
- Error.cs
- SystemBrushes.cs
- WinEventTracker.cs
- ClientFormsAuthenticationCredentials.cs
- IPEndPoint.cs
- PublisherMembershipCondition.cs
- WorkflowRuntimeServiceElement.cs
- NameSpaceEvent.cs
- PasswordBoxAutomationPeer.cs
- SupportsEventValidationAttribute.cs
- FontFamily.cs
- ThemeDictionaryExtension.cs
- SoapMessage.cs
- HandlerBase.cs
- FontConverter.cs
- KeyManager.cs
- OdbcConnectionPoolProviderInfo.cs
- SchemaTableColumn.cs
- DataGridViewMethods.cs
- TextRunProperties.cs
- StreamInfo.cs
- ZipIOLocalFileBlock.cs
- SingleResultAttribute.cs
- WebRequest.cs
- EDesignUtil.cs
- ActiveDocumentEvent.cs
- Tag.cs
- Margins.cs
- Atom10FormatterFactory.cs
- NotSupportedException.cs
- SecurityTokenContainer.cs
- MultipleViewProviderWrapper.cs
- GrammarBuilderDictation.cs
- ToolbarAUtomationPeer.cs
- DesignerLoader.cs
- IndexedString.cs
- ManagementEventWatcher.cs
- AppDomainFactory.cs
- ExpressionBuilderContext.cs
- CheckPair.cs
- UnescapedXmlDiagnosticData.cs
- TextProperties.cs
- DifferencingCollection.cs
- StreamGeometryContext.cs
- Query.cs
- DataGridParentRows.cs
- CommonGetThemePartSize.cs
- connectionpool.cs
- Simplifier.cs
- ConfigurationLocationCollection.cs
- WebBrowserNavigatedEventHandler.cs
- HtmlEmptyTagControlBuilder.cs
- CutCopyPasteHelper.cs
- SyndicationSerializer.cs
- TextAction.cs
- HtmlTableRowCollection.cs
- OptimalBreakSession.cs
- UnauthorizedAccessException.cs
- Group.cs
- ArgumentOutOfRangeException.cs
- DataGridPreparingCellForEditEventArgs.cs