Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaFacet.cs / 1305376 / XmlSchemaFacet.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.ComponentModel; using System.Xml.Serialization; internal enum FacetType { None, Length, MinLength, MaxLength, Pattern, Whitespace, Enumeration, MinExclusive, MinInclusive, MaxExclusive, MaxInclusive, TotalDigits, FractionDigits, } ///public abstract class XmlSchemaFacet : XmlSchemaAnnotated { string value; bool isFixed; FacetType facetType; /// [XmlAttribute("value")] public string Value { get { return this.value; } set { this.value = value; } } /// [XmlAttribute("fixed"), DefaultValue(false)] public virtual bool IsFixed { get { return isFixed; } set { if (!(this is XmlSchemaEnumerationFacet) && !(this is XmlSchemaPatternFacet)) { isFixed = value; } } } internal FacetType FacetType { get { return facetType; } set { facetType = value; } } } /// public abstract class XmlSchemaNumericFacet : XmlSchemaFacet { } /// public class XmlSchemaLengthFacet : XmlSchemaNumericFacet { public XmlSchemaLengthFacet() { FacetType = FacetType.Length; } } /// public class XmlSchemaMinLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMinLengthFacet() { FacetType = FacetType.MinLength; } } /// public class XmlSchemaMaxLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMaxLengthFacet() { FacetType = FacetType.MaxLength; } } /// public class XmlSchemaPatternFacet : XmlSchemaFacet { public XmlSchemaPatternFacet() { FacetType = FacetType.Pattern; } } /// public class XmlSchemaEnumerationFacet : XmlSchemaFacet { public XmlSchemaEnumerationFacet() { FacetType = FacetType.Enumeration; } } /// public class XmlSchemaMinExclusiveFacet : XmlSchemaFacet { public XmlSchemaMinExclusiveFacet() { FacetType = FacetType.MinExclusive; } } /// public class XmlSchemaMinInclusiveFacet : XmlSchemaFacet { public XmlSchemaMinInclusiveFacet() { FacetType = FacetType.MinInclusive; } } /// public class XmlSchemaMaxExclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxExclusiveFacet() { FacetType = FacetType.MaxExclusive; } } /// public class XmlSchemaMaxInclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxInclusiveFacet() { FacetType = FacetType.MaxInclusive; } } /// public class XmlSchemaTotalDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaTotalDigitsFacet() { FacetType = FacetType.TotalDigits; } } /// public class XmlSchemaFractionDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaFractionDigitsFacet() { FacetType = FacetType.FractionDigits; } } /// public class XmlSchemaWhiteSpaceFacet : XmlSchemaFacet { public XmlSchemaWhiteSpaceFacet() { FacetType = FacetType.Whitespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.ComponentModel; using System.Xml.Serialization; internal enum FacetType { None, Length, MinLength, MaxLength, Pattern, Whitespace, Enumeration, MinExclusive, MinInclusive, MaxExclusive, MaxInclusive, TotalDigits, FractionDigits, } ///public abstract class XmlSchemaFacet : XmlSchemaAnnotated { string value; bool isFixed; FacetType facetType; /// [XmlAttribute("value")] public string Value { get { return this.value; } set { this.value = value; } } /// [XmlAttribute("fixed"), DefaultValue(false)] public virtual bool IsFixed { get { return isFixed; } set { if (!(this is XmlSchemaEnumerationFacet) && !(this is XmlSchemaPatternFacet)) { isFixed = value; } } } internal FacetType FacetType { get { return facetType; } set { facetType = value; } } } /// public abstract class XmlSchemaNumericFacet : XmlSchemaFacet { } /// public class XmlSchemaLengthFacet : XmlSchemaNumericFacet { public XmlSchemaLengthFacet() { FacetType = FacetType.Length; } } /// public class XmlSchemaMinLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMinLengthFacet() { FacetType = FacetType.MinLength; } } /// public class XmlSchemaMaxLengthFacet : XmlSchemaNumericFacet { public XmlSchemaMaxLengthFacet() { FacetType = FacetType.MaxLength; } } /// public class XmlSchemaPatternFacet : XmlSchemaFacet { public XmlSchemaPatternFacet() { FacetType = FacetType.Pattern; } } /// public class XmlSchemaEnumerationFacet : XmlSchemaFacet { public XmlSchemaEnumerationFacet() { FacetType = FacetType.Enumeration; } } /// public class XmlSchemaMinExclusiveFacet : XmlSchemaFacet { public XmlSchemaMinExclusiveFacet() { FacetType = FacetType.MinExclusive; } } /// public class XmlSchemaMinInclusiveFacet : XmlSchemaFacet { public XmlSchemaMinInclusiveFacet() { FacetType = FacetType.MinInclusive; } } /// public class XmlSchemaMaxExclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxExclusiveFacet() { FacetType = FacetType.MaxExclusive; } } /// public class XmlSchemaMaxInclusiveFacet : XmlSchemaFacet { public XmlSchemaMaxInclusiveFacet() { FacetType = FacetType.MaxInclusive; } } /// public class XmlSchemaTotalDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaTotalDigitsFacet() { FacetType = FacetType.TotalDigits; } } /// public class XmlSchemaFractionDigitsFacet : XmlSchemaNumericFacet { public XmlSchemaFractionDigitsFacet() { FacetType = FacetType.FractionDigits; } } /// public class XmlSchemaWhiteSpaceFacet : XmlSchemaFacet { public XmlSchemaWhiteSpaceFacet() { FacetType = FacetType.Whitespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
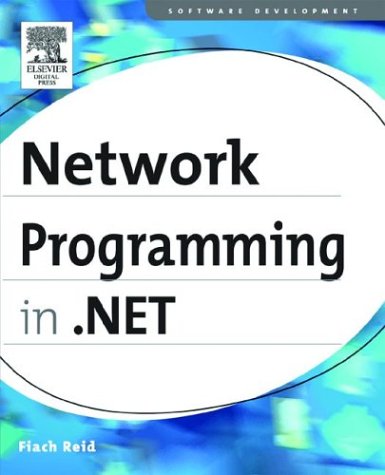
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SspiWrapper.cs
- ToolTip.cs
- counter.cs
- _TLSstream.cs
- PathSegmentCollection.cs
- ObjectDataSourceStatusEventArgs.cs
- StylusButtonCollection.cs
- WebPartHeaderCloseVerb.cs
- XamlClipboardData.cs
- PrintController.cs
- UserControlBuildProvider.cs
- EnumValidator.cs
- EntityDataSourceViewSchema.cs
- MimeFormatExtensions.cs
- DataTransferEventArgs.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- RadioButtonPopupAdapter.cs
- Model3D.cs
- Hashtable.cs
- XPathBuilder.cs
- CellConstantDomain.cs
- FormsAuthenticationCredentials.cs
- HashHelper.cs
- COM2TypeInfoProcessor.cs
- OpacityConverter.cs
- TypeDelegator.cs
- AnonymousIdentificationModule.cs
- ControlIdConverter.cs
- ObjectTag.cs
- GcHandle.cs
- EditorPartChrome.cs
- CustomError.cs
- AccessedThroughPropertyAttribute.cs
- TransportChannelListener.cs
- InputMethodStateChangeEventArgs.cs
- RegexRunner.cs
- TextFormatter.cs
- BrowserDefinition.cs
- EdgeProfileValidation.cs
- ReferenceService.cs
- DbConnectionInternal.cs
- TemplateControlBuildProvider.cs
- EDesignUtil.cs
- InputEventArgs.cs
- ExpressionCopier.cs
- MergablePropertyAttribute.cs
- ProfileManager.cs
- Guid.cs
- KeyEvent.cs
- NonceCache.cs
- HttpDictionary.cs
- MetafileHeader.cs
- DeviceContext2.cs
- NumericUpDownAccelerationCollection.cs
- Module.cs
- TransactionProxy.cs
- DataGridColumnCollection.cs
- IItemProperties.cs
- OrderedDictionaryStateHelper.cs
- SerializationException.cs
- ScriptingWebServicesSectionGroup.cs
- PrincipalPermissionMode.cs
- TextFormatterImp.cs
- JsonStringDataContract.cs
- PageThemeBuildProvider.cs
- ResolvedKeyFrameEntry.cs
- Control.cs
- RepeaterItemEventArgs.cs
- CodeDomConfigurationHandler.cs
- DecoderFallback.cs
- DoubleLink.cs
- XmlILIndex.cs
- WebPartTransformerCollection.cs
- SiteOfOriginContainer.cs
- CharacterShapingProperties.cs
- DrawListViewColumnHeaderEventArgs.cs
- SubclassTypeValidator.cs
- MessageQuerySet.cs
- FixedHyperLink.cs
- _SecureChannel.cs
- RegistrySecurity.cs
- RequiredAttributeAttribute.cs
- ResourceDefaultValueAttribute.cs
- _IPv4Address.cs
- ComponentRenameEvent.cs
- NavigationService.cs
- PrimitiveDataContract.cs
- BindingManagerDataErrorEventArgs.cs
- ConfigurationElement.cs
- InternalControlCollection.cs
- ComponentResourceKey.cs
- ObjectAnimationBase.cs
- CommandField.cs
- ObjectAnimationBase.cs
- IsolationInterop.cs
- OleAutBinder.cs
- WebPartMinimizeVerb.cs
- CultureData.cs
- AuthenticationService.cs
- EditorZone.cs