Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Abstractions / HttpServerUtilityWrapper.cs / 1305376 / HttpServerUtilityWrapper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections.Specialized; using System.IO; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class HttpServerUtilityWrapper : HttpServerUtilityBase { private HttpServerUtility _httpServerUtility; public HttpServerUtilityWrapper(HttpServerUtility httpServerUtility) { if (httpServerUtility == null) { throw new ArgumentNullException("httpServerUtility"); } _httpServerUtility = httpServerUtility; } public override Exception GetLastError() { return _httpServerUtility.GetLastError(); } public override string MachineName { get { return _httpServerUtility.MachineName; } } public override int ScriptTimeout { get { return _httpServerUtility.ScriptTimeout; } set { _httpServerUtility.ScriptTimeout = value; } } public override void ClearError() { _httpServerUtility.ClearError(); } public override object CreateObject(string progID) { return _httpServerUtility.CreateObject(progID); } public override object CreateObject(Type type) { return _httpServerUtility.CreateObject(type); } public override object CreateObjectFromClsid(string clsid) { return _httpServerUtility.CreateObjectFromClsid(clsid); } public override void Execute(string path) { _httpServerUtility.Execute(path); } public override void Execute(string path, TextWriter writer) { _httpServerUtility.Execute(path, writer); } public override void Execute(string path, bool preserveForm) { _httpServerUtility.Execute(path, preserveForm); } public override void Execute(string path, TextWriter writer, bool preserveForm) { _httpServerUtility.Execute(path, writer, preserveForm); } public override void Execute(IHttpHandler handler, TextWriter writer, bool preserveForm) { _httpServerUtility.Execute(handler, writer, preserveForm); } public override string HtmlDecode(string s) { return _httpServerUtility.HtmlDecode(s); } public override void HtmlDecode(string s, TextWriter output) { _httpServerUtility.HtmlDecode(s, output); } public override string HtmlEncode(string s) { return _httpServerUtility.HtmlEncode(s); } public override void HtmlEncode(string s, TextWriter output) { _httpServerUtility.HtmlEncode(s, output); } public override string MapPath(string path) { return _httpServerUtility.MapPath(path); } public override void Transfer(string path, bool preserveForm) { _httpServerUtility.Transfer(path, preserveForm); } public override void Transfer(string path) { _httpServerUtility.Transfer(path); } public override void Transfer(IHttpHandler handler, bool preserveForm) { _httpServerUtility.Transfer(handler, preserveForm); } public override void TransferRequest(string path) { _httpServerUtility.TransferRequest(path); } public override void TransferRequest(string path, bool preserveForm) { _httpServerUtility.TransferRequest(path, preserveForm); } public override void TransferRequest(string path, bool preserveForm, string method, NameValueCollection headers) { _httpServerUtility.TransferRequest(path, preserveForm, method, headers); } public override string UrlDecode(string s) { return _httpServerUtility.UrlDecode(s); } public override void UrlDecode(string s, TextWriter output) { _httpServerUtility.UrlDecode(s, output); } public override string UrlEncode(string s) { return _httpServerUtility.UrlEncode(s); } public override void UrlEncode(string s, TextWriter output) { _httpServerUtility.UrlEncode(s, output); } public override string UrlPathEncode(string s) { return _httpServerUtility.UrlPathEncode(s); } public override byte[] UrlTokenDecode(string input) { return HttpServerUtility.UrlTokenDecode(input); } public override string UrlTokenEncode(byte[] input) { return HttpServerUtility.UrlTokenEncode(input); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections.Specialized; using System.IO; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public class HttpServerUtilityWrapper : HttpServerUtilityBase { private HttpServerUtility _httpServerUtility; public HttpServerUtilityWrapper(HttpServerUtility httpServerUtility) { if (httpServerUtility == null) { throw new ArgumentNullException("httpServerUtility"); } _httpServerUtility = httpServerUtility; } public override Exception GetLastError() { return _httpServerUtility.GetLastError(); } public override string MachineName { get { return _httpServerUtility.MachineName; } } public override int ScriptTimeout { get { return _httpServerUtility.ScriptTimeout; } set { _httpServerUtility.ScriptTimeout = value; } } public override void ClearError() { _httpServerUtility.ClearError(); } public override object CreateObject(string progID) { return _httpServerUtility.CreateObject(progID); } public override object CreateObject(Type type) { return _httpServerUtility.CreateObject(type); } public override object CreateObjectFromClsid(string clsid) { return _httpServerUtility.CreateObjectFromClsid(clsid); } public override void Execute(string path) { _httpServerUtility.Execute(path); } public override void Execute(string path, TextWriter writer) { _httpServerUtility.Execute(path, writer); } public override void Execute(string path, bool preserveForm) { _httpServerUtility.Execute(path, preserveForm); } public override void Execute(string path, TextWriter writer, bool preserveForm) { _httpServerUtility.Execute(path, writer, preserveForm); } public override void Execute(IHttpHandler handler, TextWriter writer, bool preserveForm) { _httpServerUtility.Execute(handler, writer, preserveForm); } public override string HtmlDecode(string s) { return _httpServerUtility.HtmlDecode(s); } public override void HtmlDecode(string s, TextWriter output) { _httpServerUtility.HtmlDecode(s, output); } public override string HtmlEncode(string s) { return _httpServerUtility.HtmlEncode(s); } public override void HtmlEncode(string s, TextWriter output) { _httpServerUtility.HtmlEncode(s, output); } public override string MapPath(string path) { return _httpServerUtility.MapPath(path); } public override void Transfer(string path, bool preserveForm) { _httpServerUtility.Transfer(path, preserveForm); } public override void Transfer(string path) { _httpServerUtility.Transfer(path); } public override void Transfer(IHttpHandler handler, bool preserveForm) { _httpServerUtility.Transfer(handler, preserveForm); } public override void TransferRequest(string path) { _httpServerUtility.TransferRequest(path); } public override void TransferRequest(string path, bool preserveForm) { _httpServerUtility.TransferRequest(path, preserveForm); } public override void TransferRequest(string path, bool preserveForm, string method, NameValueCollection headers) { _httpServerUtility.TransferRequest(path, preserveForm, method, headers); } public override string UrlDecode(string s) { return _httpServerUtility.UrlDecode(s); } public override void UrlDecode(string s, TextWriter output) { _httpServerUtility.UrlDecode(s, output); } public override string UrlEncode(string s) { return _httpServerUtility.UrlEncode(s); } public override void UrlEncode(string s, TextWriter output) { _httpServerUtility.UrlEncode(s, output); } public override string UrlPathEncode(string s) { return _httpServerUtility.UrlPathEncode(s); } public override byte[] UrlTokenDecode(string input) { return HttpServerUtility.UrlTokenDecode(input); } public override string UrlTokenEncode(byte[] input) { return HttpServerUtility.UrlTokenEncode(input); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
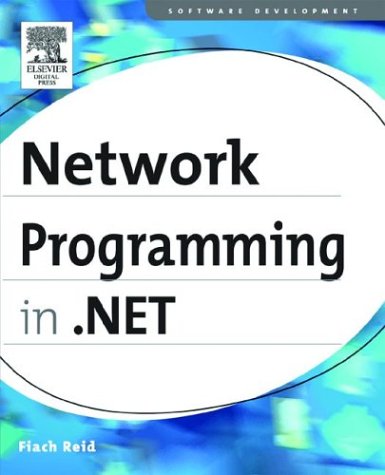
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeMemberField.cs
- XComponentModel.cs
- WindowsListBox.cs
- WpfWebRequestHelper.cs
- _ListenerAsyncResult.cs
- EncoderExceptionFallback.cs
- ScopedKnownTypes.cs
- PropertyEmitterBase.cs
- PageThemeCodeDomTreeGenerator.cs
- StaticSiteMapProvider.cs
- TextSelection.cs
- KeyGestureConverter.cs
- Brush.cs
- LogLogRecordEnumerator.cs
- Panel.cs
- Validator.cs
- SchemaImporterExtensionElementCollection.cs
- CalendarData.cs
- DateTimeFormatInfoScanner.cs
- UndoEngine.cs
- FormClosingEvent.cs
- MatrixCamera.cs
- TrackBar.cs
- DbFunctionCommandTree.cs
- AutomationElementCollection.cs
- DateTimeSerializationSection.cs
- RuntimeEnvironment.cs
- DSACryptoServiceProvider.cs
- GenericPrincipal.cs
- MediaContext.cs
- XmlSerializer.cs
- XhtmlMobileTextWriter.cs
- BrowsableAttribute.cs
- UncommonField.cs
- HttpRawResponse.cs
- DbFunctionCommandTree.cs
- TreeSet.cs
- ReadOnlyCollectionBuilder.cs
- AncestorChangedEventArgs.cs
- DefaultMemberAttribute.cs
- StringPropertyBuilder.cs
- CommandArguments.cs
- FormsAuthenticationModule.cs
- RequestNavigateEventArgs.cs
- TrayIconDesigner.cs
- SupportingTokenParameters.cs
- CmsInterop.cs
- OrderedDictionaryStateHelper.cs
- SendMessageChannelCache.cs
- SecurityValidationBehavior.cs
- ZipIOBlockManager.cs
- TextDecorationCollection.cs
- AuthorizationRuleCollection.cs
- FloatUtil.cs
- SqlError.cs
- FactoryGenerator.cs
- WmlControlAdapter.cs
- ErrorRuntimeConfig.cs
- AnonymousIdentificationSection.cs
- CommonRemoteMemoryBlock.cs
- AnonymousIdentificationSection.cs
- QilPatternFactory.cs
- Signature.cs
- DataSourceControl.cs
- TimeSpanStorage.cs
- DataBinder.cs
- CodeExpressionCollection.cs
- Vector3DAnimationBase.cs
- PathGeometry.cs
- DoubleConverter.cs
- RectangleGeometry.cs
- Matrix3D.cs
- SmiEventSink_DeferedProcessing.cs
- TransformerConfigurationWizardBase.cs
- ComponentChangedEvent.cs
- SessionEndedEventArgs.cs
- _ServiceNameStore.cs
- ADRoleFactory.cs
- LinkButton.cs
- Int32AnimationUsingKeyFrames.cs
- RightNameExpirationInfoPair.cs
- RtfFormatStack.cs
- SafeCryptoHandles.cs
- GridViewAutomationPeer.cs
- DefaultTraceListener.cs
- FixedSOMLineRanges.cs
- Sql8ExpressionRewriter.cs
- RectAnimationBase.cs
- FlowDocumentPage.cs
- LabelAutomationPeer.cs
- XmlRawWriterWrapper.cs
- nulltextcontainer.cs
- Debugger.cs
- FunctionMappingTranslator.cs
- TraceHandlerErrorFormatter.cs
- AnimationClock.cs
- SqlReferenceCollection.cs
- FullTextBreakpoint.cs
- Keyboard.cs
- XmlSchemaFacet.cs