Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / EditCommandColumn.cs / 1305376 / EditCommandColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; ////// public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Initializes a cell within the column. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; ////// public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Initializes a cell within the column. ///
Link Menu
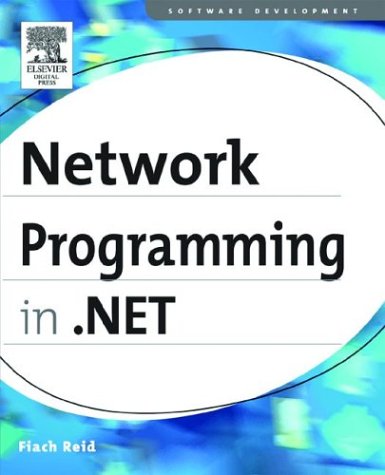
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinkedResourceCollection.cs
- ComEventsHelper.cs
- assemblycache.cs
- IPGlobalProperties.cs
- SoapProtocolReflector.cs
- WebPartConnectionsEventArgs.cs
- ControlValuePropertyAttribute.cs
- XmlAttributeProperties.cs
- AsyncPostBackTrigger.cs
- DataProtection.cs
- NavigateEvent.cs
- TreeNodeCollectionEditor.cs
- Visual3DCollection.cs
- MLangCodePageEncoding.cs
- InputScopeConverter.cs
- CommentAction.cs
- LinkConverter.cs
- HtmlTableRow.cs
- WSTrustDec2005.cs
- TargetInvocationException.cs
- XXXInfos.cs
- unsafeIndexingFilterStream.cs
- PixelShader.cs
- EventLogPermissionAttribute.cs
- InfoCardMetadataExchangeClient.cs
- InfoCardPolicy.cs
- DomainConstraint.cs
- DataGridViewCellStyleConverter.cs
- RouteParameter.cs
- GeometryHitTestResult.cs
- SmtpClient.cs
- ImmutableObjectAttribute.cs
- ReadOnlyDataSourceView.cs
- SQLChars.cs
- CompositeCollection.cs
- CustomGrammar.cs
- InvalidDataException.cs
- ApplicationDirectory.cs
- ComboBoxItem.cs
- FormViewDeletedEventArgs.cs
- EditingScopeUndoUnit.cs
- IPEndPointCollection.cs
- CalendarAutomationPeer.cs
- Line.cs
- QueryOutputWriterV1.cs
- Overlapped.cs
- XmlWellformedWriter.cs
- ComponentChangedEvent.cs
- ColorConverter.cs
- BrowserCapabilitiesCodeGenerator.cs
- ExceptionUtility.cs
- RemoteWebConfigurationHostStream.cs
- TransactionBridgeSection.cs
- PropertyGrid.cs
- RotateTransform.cs
- TdsParserHelperClasses.cs
- Error.cs
- SmtpFailedRecipientException.cs
- DBBindings.cs
- DelegatedStream.cs
- FigureParaClient.cs
- Queue.cs
- TempFiles.cs
- XmlUrlResolver.cs
- ObjectStorage.cs
- WorkflowQueuingService.cs
- Size.cs
- InstanceDescriptor.cs
- JumpList.cs
- CodeTypeDeclarationCollection.cs
- RectConverter.cs
- ApplicationSecurityManager.cs
- AddInActivator.cs
- EventDescriptor.cs
- EmbossBitmapEffect.cs
- QueryStatement.cs
- ControlAdapter.cs
- FormsAuthenticationModule.cs
- ToolStripSeparator.cs
- InvalidComObjectException.cs
- LOSFormatter.cs
- WebServiceParameterData.cs
- TypeConverterAttribute.cs
- HostingEnvironment.cs
- TableLayoutPanelCellPosition.cs
- _PooledStream.cs
- X509SubjectKeyIdentifierClause.cs
- TaskFileService.cs
- HtmlEncodedRawTextWriter.cs
- DesignColumnCollection.cs
- SafeNativeMethods.cs
- CqlErrorHelper.cs
- RadialGradientBrush.cs
- InvalidPropValue.cs
- DbMetaDataCollectionNames.cs
- ByteKeyFrameCollection.cs
- InputProcessorProfilesLoader.cs
- ObjectSpanRewriter.cs
- ActivityExecutionContext.cs
- UpdateDelegates.Generated.cs