Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / interop / ErrorCodes.cs / 1305600 / ErrorCodes.cs
/**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace MS.Internal.Interop { using System; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ////// Wrapper for common Win32 status codes. /// [StructLayout(LayoutKind.Explicit)] internal struct Win32Error { [FieldOffset(0)] private readonly int _value; // NOTE: These public static field declarations are automatically // picked up by (HRESULT's) ToString through reflection. ///The operation completed successfully. public static readonly Win32Error ERROR_SUCCESS = new Win32Error(0); ///Incorrect function. public static readonly Win32Error ERROR_INVALID_FUNCTION = new Win32Error(1); ///The system cannot find the file specified. public static readonly Win32Error ERROR_FILE_NOT_FOUND = new Win32Error(2); ///The system cannot find the path specified. public static readonly Win32Error ERROR_PATH_NOT_FOUND = new Win32Error(3); ///The system cannot open the file. public static readonly Win32Error ERROR_TOO_MANY_OPEN_FILES = new Win32Error(4); ///Access is denied. public static readonly Win32Error ERROR_ACCESS_DENIED = new Win32Error(5); ///The handle is invalid. public static readonly Win32Error ERROR_INVALID_HANDLE = new Win32Error(6); ///Not enough storage is available to complete this operation. public static readonly Win32Error ERROR_OUTOFMEMORY = new Win32Error(14); ///There are no more files. public static readonly Win32Error ERROR_NO_MORE_FILES = new Win32Error(18); ///The process cannot access the file because it is being used by another process. public static readonly Win32Error ERROR_SHARING_VIOLATION = new Win32Error(32); ///The parameter is incorrect. public static readonly Win32Error ERROR_INVALID_PARAMETER = new Win32Error(87); ///The data area passed to a system call is too small. public static readonly Win32Error ERROR_INSUFFICIENT_BUFFER = new Win32Error(122); ///Cannot nest calls to LoadModule. public static readonly Win32Error ERROR_NESTING_NOT_ALLOWED = new Win32Error(215); ///Illegal operation attempted on a registry key that has been marked for deletion. public static readonly Win32Error ERROR_KEY_DELETED = new Win32Error(1018); ///There was no match for the specified key in the index. public static readonly Win32Error ERROR_NO_MATCH = new Win32Error(1169); ///An invalid device was specified. public static readonly Win32Error ERROR_BAD_DEVICE = new Win32Error(1200); ///The operation was canceled by the user. public static readonly Win32Error ERROR_CANCELLED = new Win32Error(1223); ///Invalid window handle. public static readonly Win32Error ERROR_INVALID_WINDOW_HANDLE = new Win32Error(1400); ///This operation returned because the timeout period expired. public static readonly Win32Error ERROR_TIMEOUT = new Win32Error(1460); ///The specified datatype is invalid. public static readonly Win32Error ERROR_INVALID_DATATYPE = new Win32Error(1804); ////// Create a new Win32 error. /// /// The integer value of the error. public Win32Error(int i) { _value = i; } ///Performs HRESULT_FROM_WIN32 conversion. /// The Win32 error being converted to an HRESULT. ///The equivilent HRESULT value. public static explicit operator HRESULT(Win32Error error) { // #define __HRESULT_FROM_WIN32(x) // ((HRESULT)(x) <= 0 ? ((HRESULT)(x)) : ((HRESULT) (((x) & 0x0000FFFF) | (FACILITY_WIN32 << 16) | 0x80000000))) if (error._value <= 0) { return new HRESULT((uint)error._value); } return HRESULT.Make(true, Facility.Win32, error._value & 0x0000FFFF); } // Method version of the cast operator. ///Performs HRESULT_FROM_WIN32 conversion. /// The Win32 error being converted to an HRESULT. ///The equivilent HRESULT value. public HRESULT ToHRESULT() { return (HRESULT)this; } ///Performs the equivalent of Win32's GetLastError() ///A Win32Error instance with the result of the native GetLastError public static Win32Error GetLastError() { return new Win32Error(Marshal.GetLastWin32Error()); } public override bool Equals(object obj) { try { return ((Win32Error)obj)._value == _value; } catch (InvalidCastException) { return false; } } public override int GetHashCode() { return _value.GetHashCode(); } ////// Compare two Win32 error codes for equality. /// /// The first error code to compare. /// The second error code to compare. ///Whether the two error codes are the same. public static bool operator ==(Win32Error errLeft, Win32Error errRight) { return errLeft._value == errRight._value; } ////// Compare two Win32 error codes for inequality. /// /// The first error code to compare. /// The second error code to compare. ///Whether the two error codes are not the same. public static bool operator !=(Win32Error errLeft, Win32Error errRight) { return !(errLeft == errRight); } } internal enum Facility { ///FACILITY_NULL Null = 0, ///FACILITY_RPC Rpc = 1, ///FACILITY_DISPATCH Dispatch = 2, ///FACILITY_STORAGE Storage = 3, ///FACILITY_ITF Itf = 4, ///FACILITY_WIN32 Win32 = 7, ///FACILITY_WINDOWS Windows = 8, ///FACILITY_CONTROL Control = 10, ///MSDN doced facility code for ESE errors. Ese = 0xE5E, } ///Wrapper for HRESULT status codes. [StructLayout(LayoutKind.Explicit)] internal struct HRESULT { [FieldOffset(0)] private readonly uint _value; // NOTE: These public static field declarations are automatically // picked up by ToString through reflection. ///S_OK public static readonly HRESULT S_OK = new HRESULT(0x00000000); ///S_FALSE public static readonly HRESULT S_FALSE = new HRESULT(0x00000001); ///E_NOTIMPL public static readonly HRESULT E_NOTIMPL = new HRESULT(0x80004001); ///E_NOINTERFACE public static readonly HRESULT E_NOINTERFACE = new HRESULT(0x80004002); ///E_POINTER public static readonly HRESULT E_POINTER = new HRESULT(0x80004003); ///E_ABORT public static readonly HRESULT E_ABORT = new HRESULT(0x80004004); ///E_FAIL public static readonly HRESULT E_FAIL = new HRESULT(0x80004005); ///E_UNEXPECTED public static readonly HRESULT E_UNEXPECTED = new HRESULT(0x8000FFFF); ///DISP_E_MEMBERNOTFOUND public static readonly HRESULT DISP_E_MEMBERNOTFOUND = new HRESULT(0x80020003); ///DISP_E_TYPEMISMATCH public static readonly HRESULT DISP_E_TYPEMISMATCH = new HRESULT(0x80020005); ///DISP_E_UNKNOWNNAME public static readonly HRESULT DISP_E_UNKNOWNNAME = new HRESULT(0x80020006); ///DISP_E_EXCEPTION public static readonly HRESULT DISP_E_EXCEPTION = new HRESULT(0x80020009); ///DISP_E_OVERFLOW public static readonly HRESULT DISP_E_OVERFLOW = new HRESULT(0x8002000A); ///DISP_E_BADINDEX public static readonly HRESULT DISP_E_BADINDEX = new HRESULT(0x8002000B); ///DISP_E_BADPARAMCOUNT public static readonly HRESULT DISP_E_BADPARAMCOUNT = new HRESULT(0x8002000E); ///DISP_E_PARAMNOTOPTIONAL public static readonly HRESULT DISP_E_PARAMNOTOPTIONAL = new HRESULT(0x8002000F); ///SCRIPT_E_REPORTED public static readonly HRESULT SCRIPT_E_REPORTED = new HRESULT(0x80020101); ///STG_E_INVALIDFUNCTION public static readonly HRESULT STG_E_INVALIDFUNCTION = new HRESULT(0x80030001); ///DESTS_E_NO_MATCHING_ASSOC_HANDLER. ////// Win7 error code for Jump Lists. There is no associated handler for the given item registered by the specified application. /// public static readonly HRESULT DESTS_E_NO_MATCHING_ASSOC_HANDLER = new HRESULT(0x80040F03); ///E_ACCESSDENIED public static readonly HRESULT E_ACCESSDENIED = new HRESULT(0x80070005); ///E_OUTOFMEMORY public static readonly HRESULT E_OUTOFMEMORY = new HRESULT(0x8007000E); ///E_INVALIDARG public static readonly HRESULT E_INVALIDARG = new HRESULT(0x80070057); ///COR_E_OBJECTDISPOSED public static readonly HRESULT COR_E_OBJECTDISPOSED = new HRESULT(0x80131622); ///WC_E_GREATERTHAN public static readonly HRESULT WC_E_GREATERTHAN = new HRESULT(0xC00CEE23); ///WC_E_SYNTAX public static readonly HRESULT WC_E_SYNTAX = new HRESULT(0xC00CEE2D); ////// Create an HRESULT from an integer value. /// /// public HRESULT(uint i) { _value = i; } public static HRESULT Make(bool severe, Facility facility, int code) { //#define MAKE_HRESULT(sev,fac,code) \ // ((HRESULT) (((unsigned long)(sev)<<31) | ((unsigned long)(fac)<<16) | ((unsigned long)(code))) ) return new HRESULT((uint)((severe ? (1 << 31) : 0) | ((int)facility << 16) | code)); } ////// retrieve HRESULT_FACILITY /// public Facility Facility { get { return GetFacility((int)_value); } } public static Facility GetFacility(int errorCode) { // #define HRESULT_FACILITY(hr) (((hr) >> 16) & 0x1fff) return (Facility)((errorCode >> 16) & 0x1fff); } ////// retrieve HRESULT_CODE /// public int Code { get { return GetCode((int)_value); } } public static int GetCode(int error) { // #define HRESULT_CODE(hr) ((hr) & 0xFFFF) return (int)(error & 0xFFFF); } #region Object class override members ////// Get a string representation of this HRESULT. /// ///public override string ToString() { // Use reflection to try to name this HRESULT. // This is expensive, but if someone's ever printing HRESULT strings then // I think it's a fair guess that they're not in a performance critical area // (e.g. printing exception strings). // This is less error prone than trying to keep the list in the function. // To properly add an HRESULT's name to the ToString table, just add the HRESULT // like all the others above. // // foreach (FieldInfo publicStaticField in typeof(HRESULT).GetFields(BindingFlags.Static | BindingFlags.Public)) { if (publicStaticField.FieldType == typeof(HRESULT)) { var hr = (HRESULT)publicStaticField.GetValue(null); if (hr == this) { return publicStaticField.Name; } } } // Try Win32 error codes also if (Facility == Facility.Win32) { foreach (FieldInfo publicStaticField in typeof(Win32Error).GetFields(BindingFlags.Static | BindingFlags.Public)) { if (publicStaticField.FieldType == typeof(Win32Error)) { var error = (Win32Error)publicStaticField.GetValue(null); if ((HRESULT)error == this) { return "HRESULT_FROM_WIN32(" + publicStaticField.Name + ")"; } } } } // If there's no good name for this HRESULT, // return the string as readable hex (0x########) format. return string.Format(CultureInfo.InvariantCulture, "0x{0:X8}", _value); } public override bool Equals(object obj) { try { return ((HRESULT)obj)._value == _value; } catch (InvalidCastException) { return false; } } public override int GetHashCode() { return _value.GetHashCode(); } #endregion public static bool operator ==(HRESULT hrLeft, HRESULT hrRight) { return hrLeft._value == hrRight._value; } public static bool operator !=(HRESULT hrLeft, HRESULT hrRight) { return !(hrLeft == hrRight); } public bool Succeeded { get { return (int)_value >= 0; } } public bool Failed { get { return (int)_value < 0; } } public void ThrowIfFailed() { ThrowIfFailed(null); } /// /// Critical - Calls critical Marshal method GetExceptionForHR. /// TreatAsSafe - Callers can't gain additional information they didn't already have. /// This is just throwing an exception. /// [SecurityCritical, SecurityTreatAsSafe] public void ThrowIfFailed(string message) { Exception e = GetException(message); if (e != null) { throw e; } } public Exception GetException() { return GetException(null); } ////// Critical - Calls critical Marshal method GetExceptionForHR. /// TreatAsSafe - Callers can't gain additional information they didn't already have. /// This is just getting an exception object. /// [SecurityCritical, SecurityTreatAsSafe] public Exception GetException(string message) { if (!Failed) { return null; } // If you're throwing an exception I assume it's OK for me to take some time to give it back. // I want to convert the HRESULT to a more appropriate exception type than COMException. // Marshal.ThrowExceptionForHR does this for me, but the general call uses GetErrorInfo // if it's set, and then ignores the HRESULT that I've provided. This makes it so this // call works the first time but you get burned on the second. To avoid this, I use // the overload that explicitly ignores the IErrorInfo. // In addition, the function doesn't allow me to set the Message unless I go through // the process of implementing an IErrorInfo and then use that. There's no stock // implementations of IErrorInfo available and I don't think it's worth the maintenance // overhead of doing it, nor would it have significant value over this approach. Exception e = Marshal.GetExceptionForHR((int)_value, new IntPtr(-1)); Debug.Assert(e != null); // ArgumentNullException doesn't have the right constructor parameters, // (nor does Win32Exception...) // but E_POINTER gets mapped to NullReferenceException, // so I don't think it will ever matter. Debug.Assert(!(e is ArgumentNullException)); // If we're not getting anything better than a COMException from Marshal, // then at least check the facility and attempt to do better ourselves. if (e.GetType() == typeof(COMException)) { switch (Facility) { case Facility.Win32: // Win32Exception generates default messages based on the error type. // Don't override this behavior if the caller didn't explicitly // specify something more appropriate. if (string.IsNullOrEmpty(message)) { e = new Win32Exception(Code); } else { e = new Win32Exception(Code, message); } break; default: e = new COMException(message ?? e.Message, (int)_value); break; } } else { // Replace the message if we have something better. if (!string.IsNullOrEmpty(message)) { ConstructorInfo cons = e.GetType().GetConstructor(new[] { typeof(string) }); if (null != cons) { e = cons.Invoke(new object[] { message }) as Exception; Debug.Assert(e != null); } } } return e; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace MS.Internal.Interop { using System; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ////// Wrapper for common Win32 status codes. /// [StructLayout(LayoutKind.Explicit)] internal struct Win32Error { [FieldOffset(0)] private readonly int _value; // NOTE: These public static field declarations are automatically // picked up by (HRESULT's) ToString through reflection. ///The operation completed successfully. public static readonly Win32Error ERROR_SUCCESS = new Win32Error(0); ///Incorrect function. public static readonly Win32Error ERROR_INVALID_FUNCTION = new Win32Error(1); ///The system cannot find the file specified. public static readonly Win32Error ERROR_FILE_NOT_FOUND = new Win32Error(2); ///The system cannot find the path specified. public static readonly Win32Error ERROR_PATH_NOT_FOUND = new Win32Error(3); ///The system cannot open the file. public static readonly Win32Error ERROR_TOO_MANY_OPEN_FILES = new Win32Error(4); ///Access is denied. public static readonly Win32Error ERROR_ACCESS_DENIED = new Win32Error(5); ///The handle is invalid. public static readonly Win32Error ERROR_INVALID_HANDLE = new Win32Error(6); ///Not enough storage is available to complete this operation. public static readonly Win32Error ERROR_OUTOFMEMORY = new Win32Error(14); ///There are no more files. public static readonly Win32Error ERROR_NO_MORE_FILES = new Win32Error(18); ///The process cannot access the file because it is being used by another process. public static readonly Win32Error ERROR_SHARING_VIOLATION = new Win32Error(32); ///The parameter is incorrect. public static readonly Win32Error ERROR_INVALID_PARAMETER = new Win32Error(87); ///The data area passed to a system call is too small. public static readonly Win32Error ERROR_INSUFFICIENT_BUFFER = new Win32Error(122); ///Cannot nest calls to LoadModule. public static readonly Win32Error ERROR_NESTING_NOT_ALLOWED = new Win32Error(215); ///Illegal operation attempted on a registry key that has been marked for deletion. public static readonly Win32Error ERROR_KEY_DELETED = new Win32Error(1018); ///There was no match for the specified key in the index. public static readonly Win32Error ERROR_NO_MATCH = new Win32Error(1169); ///An invalid device was specified. public static readonly Win32Error ERROR_BAD_DEVICE = new Win32Error(1200); ///The operation was canceled by the user. public static readonly Win32Error ERROR_CANCELLED = new Win32Error(1223); ///Invalid window handle. public static readonly Win32Error ERROR_INVALID_WINDOW_HANDLE = new Win32Error(1400); ///This operation returned because the timeout period expired. public static readonly Win32Error ERROR_TIMEOUT = new Win32Error(1460); ///The specified datatype is invalid. public static readonly Win32Error ERROR_INVALID_DATATYPE = new Win32Error(1804); ////// Create a new Win32 error. /// /// The integer value of the error. public Win32Error(int i) { _value = i; } ///Performs HRESULT_FROM_WIN32 conversion. /// The Win32 error being converted to an HRESULT. ///The equivilent HRESULT value. public static explicit operator HRESULT(Win32Error error) { // #define __HRESULT_FROM_WIN32(x) // ((HRESULT)(x) <= 0 ? ((HRESULT)(x)) : ((HRESULT) (((x) & 0x0000FFFF) | (FACILITY_WIN32 << 16) | 0x80000000))) if (error._value <= 0) { return new HRESULT((uint)error._value); } return HRESULT.Make(true, Facility.Win32, error._value & 0x0000FFFF); } // Method version of the cast operator. ///Performs HRESULT_FROM_WIN32 conversion. /// The Win32 error being converted to an HRESULT. ///The equivilent HRESULT value. public HRESULT ToHRESULT() { return (HRESULT)this; } ///Performs the equivalent of Win32's GetLastError() ///A Win32Error instance with the result of the native GetLastError public static Win32Error GetLastError() { return new Win32Error(Marshal.GetLastWin32Error()); } public override bool Equals(object obj) { try { return ((Win32Error)obj)._value == _value; } catch (InvalidCastException) { return false; } } public override int GetHashCode() { return _value.GetHashCode(); } ////// Compare two Win32 error codes for equality. /// /// The first error code to compare. /// The second error code to compare. ///Whether the two error codes are the same. public static bool operator ==(Win32Error errLeft, Win32Error errRight) { return errLeft._value == errRight._value; } ////// Compare two Win32 error codes for inequality. /// /// The first error code to compare. /// The second error code to compare. ///Whether the two error codes are not the same. public static bool operator !=(Win32Error errLeft, Win32Error errRight) { return !(errLeft == errRight); } } internal enum Facility { ///FACILITY_NULL Null = 0, ///FACILITY_RPC Rpc = 1, ///FACILITY_DISPATCH Dispatch = 2, ///FACILITY_STORAGE Storage = 3, ///FACILITY_ITF Itf = 4, ///FACILITY_WIN32 Win32 = 7, ///FACILITY_WINDOWS Windows = 8, ///FACILITY_CONTROL Control = 10, ///MSDN doced facility code for ESE errors. Ese = 0xE5E, } ///Wrapper for HRESULT status codes. [StructLayout(LayoutKind.Explicit)] internal struct HRESULT { [FieldOffset(0)] private readonly uint _value; // NOTE: These public static field declarations are automatically // picked up by ToString through reflection. ///S_OK public static readonly HRESULT S_OK = new HRESULT(0x00000000); ///S_FALSE public static readonly HRESULT S_FALSE = new HRESULT(0x00000001); ///E_NOTIMPL public static readonly HRESULT E_NOTIMPL = new HRESULT(0x80004001); ///E_NOINTERFACE public static readonly HRESULT E_NOINTERFACE = new HRESULT(0x80004002); ///E_POINTER public static readonly HRESULT E_POINTER = new HRESULT(0x80004003); ///E_ABORT public static readonly HRESULT E_ABORT = new HRESULT(0x80004004); ///E_FAIL public static readonly HRESULT E_FAIL = new HRESULT(0x80004005); ///E_UNEXPECTED public static readonly HRESULT E_UNEXPECTED = new HRESULT(0x8000FFFF); ///DISP_E_MEMBERNOTFOUND public static readonly HRESULT DISP_E_MEMBERNOTFOUND = new HRESULT(0x80020003); ///DISP_E_TYPEMISMATCH public static readonly HRESULT DISP_E_TYPEMISMATCH = new HRESULT(0x80020005); ///DISP_E_UNKNOWNNAME public static readonly HRESULT DISP_E_UNKNOWNNAME = new HRESULT(0x80020006); ///DISP_E_EXCEPTION public static readonly HRESULT DISP_E_EXCEPTION = new HRESULT(0x80020009); ///DISP_E_OVERFLOW public static readonly HRESULT DISP_E_OVERFLOW = new HRESULT(0x8002000A); ///DISP_E_BADINDEX public static readonly HRESULT DISP_E_BADINDEX = new HRESULT(0x8002000B); ///DISP_E_BADPARAMCOUNT public static readonly HRESULT DISP_E_BADPARAMCOUNT = new HRESULT(0x8002000E); ///DISP_E_PARAMNOTOPTIONAL public static readonly HRESULT DISP_E_PARAMNOTOPTIONAL = new HRESULT(0x8002000F); ///SCRIPT_E_REPORTED public static readonly HRESULT SCRIPT_E_REPORTED = new HRESULT(0x80020101); ///STG_E_INVALIDFUNCTION public static readonly HRESULT STG_E_INVALIDFUNCTION = new HRESULT(0x80030001); ///DESTS_E_NO_MATCHING_ASSOC_HANDLER. ////// Win7 error code for Jump Lists. There is no associated handler for the given item registered by the specified application. /// public static readonly HRESULT DESTS_E_NO_MATCHING_ASSOC_HANDLER = new HRESULT(0x80040F03); ///E_ACCESSDENIED public static readonly HRESULT E_ACCESSDENIED = new HRESULT(0x80070005); ///E_OUTOFMEMORY public static readonly HRESULT E_OUTOFMEMORY = new HRESULT(0x8007000E); ///E_INVALIDARG public static readonly HRESULT E_INVALIDARG = new HRESULT(0x80070057); ///COR_E_OBJECTDISPOSED public static readonly HRESULT COR_E_OBJECTDISPOSED = new HRESULT(0x80131622); ///WC_E_GREATERTHAN public static readonly HRESULT WC_E_GREATERTHAN = new HRESULT(0xC00CEE23); ///WC_E_SYNTAX public static readonly HRESULT WC_E_SYNTAX = new HRESULT(0xC00CEE2D); ////// Create an HRESULT from an integer value. /// /// public HRESULT(uint i) { _value = i; } public static HRESULT Make(bool severe, Facility facility, int code) { //#define MAKE_HRESULT(sev,fac,code) \ // ((HRESULT) (((unsigned long)(sev)<<31) | ((unsigned long)(fac)<<16) | ((unsigned long)(code))) ) return new HRESULT((uint)((severe ? (1 << 31) : 0) | ((int)facility << 16) | code)); } ////// retrieve HRESULT_FACILITY /// public Facility Facility { get { return GetFacility((int)_value); } } public static Facility GetFacility(int errorCode) { // #define HRESULT_FACILITY(hr) (((hr) >> 16) & 0x1fff) return (Facility)((errorCode >> 16) & 0x1fff); } ////// retrieve HRESULT_CODE /// public int Code { get { return GetCode((int)_value); } } public static int GetCode(int error) { // #define HRESULT_CODE(hr) ((hr) & 0xFFFF) return (int)(error & 0xFFFF); } #region Object class override members ////// Get a string representation of this HRESULT. /// ///public override string ToString() { // Use reflection to try to name this HRESULT. // This is expensive, but if someone's ever printing HRESULT strings then // I think it's a fair guess that they're not in a performance critical area // (e.g. printing exception strings). // This is less error prone than trying to keep the list in the function. // To properly add an HRESULT's name to the ToString table, just add the HRESULT // like all the others above. // // foreach (FieldInfo publicStaticField in typeof(HRESULT).GetFields(BindingFlags.Static | BindingFlags.Public)) { if (publicStaticField.FieldType == typeof(HRESULT)) { var hr = (HRESULT)publicStaticField.GetValue(null); if (hr == this) { return publicStaticField.Name; } } } // Try Win32 error codes also if (Facility == Facility.Win32) { foreach (FieldInfo publicStaticField in typeof(Win32Error).GetFields(BindingFlags.Static | BindingFlags.Public)) { if (publicStaticField.FieldType == typeof(Win32Error)) { var error = (Win32Error)publicStaticField.GetValue(null); if ((HRESULT)error == this) { return "HRESULT_FROM_WIN32(" + publicStaticField.Name + ")"; } } } } // If there's no good name for this HRESULT, // return the string as readable hex (0x########) format. return string.Format(CultureInfo.InvariantCulture, "0x{0:X8}", _value); } public override bool Equals(object obj) { try { return ((HRESULT)obj)._value == _value; } catch (InvalidCastException) { return false; } } public override int GetHashCode() { return _value.GetHashCode(); } #endregion public static bool operator ==(HRESULT hrLeft, HRESULT hrRight) { return hrLeft._value == hrRight._value; } public static bool operator !=(HRESULT hrLeft, HRESULT hrRight) { return !(hrLeft == hrRight); } public bool Succeeded { get { return (int)_value >= 0; } } public bool Failed { get { return (int)_value < 0; } } public void ThrowIfFailed() { ThrowIfFailed(null); } /// /// Critical - Calls critical Marshal method GetExceptionForHR. /// TreatAsSafe - Callers can't gain additional information they didn't already have. /// This is just throwing an exception. /// [SecurityCritical, SecurityTreatAsSafe] public void ThrowIfFailed(string message) { Exception e = GetException(message); if (e != null) { throw e; } } public Exception GetException() { return GetException(null); } ////// Critical - Calls critical Marshal method GetExceptionForHR. /// TreatAsSafe - Callers can't gain additional information they didn't already have. /// This is just getting an exception object. /// [SecurityCritical, SecurityTreatAsSafe] public Exception GetException(string message) { if (!Failed) { return null; } // If you're throwing an exception I assume it's OK for me to take some time to give it back. // I want to convert the HRESULT to a more appropriate exception type than COMException. // Marshal.ThrowExceptionForHR does this for me, but the general call uses GetErrorInfo // if it's set, and then ignores the HRESULT that I've provided. This makes it so this // call works the first time but you get burned on the second. To avoid this, I use // the overload that explicitly ignores the IErrorInfo. // In addition, the function doesn't allow me to set the Message unless I go through // the process of implementing an IErrorInfo and then use that. There's no stock // implementations of IErrorInfo available and I don't think it's worth the maintenance // overhead of doing it, nor would it have significant value over this approach. Exception e = Marshal.GetExceptionForHR((int)_value, new IntPtr(-1)); Debug.Assert(e != null); // ArgumentNullException doesn't have the right constructor parameters, // (nor does Win32Exception...) // but E_POINTER gets mapped to NullReferenceException, // so I don't think it will ever matter. Debug.Assert(!(e is ArgumentNullException)); // If we're not getting anything better than a COMException from Marshal, // then at least check the facility and attempt to do better ourselves. if (e.GetType() == typeof(COMException)) { switch (Facility) { case Facility.Win32: // Win32Exception generates default messages based on the error type. // Don't override this behavior if the caller didn't explicitly // specify something more appropriate. if (string.IsNullOrEmpty(message)) { e = new Win32Exception(Code); } else { e = new Win32Exception(Code, message); } break; default: e = new COMException(message ?? e.Message, (int)_value); break; } } else { // Replace the message if we have something better. if (!string.IsNullOrEmpty(message)) { ConstructorInfo cons = e.GetType().GetConstructor(new[] { typeof(string) }); if (null != cons) { e = cons.Invoke(new object[] { message }) as Exception; Debug.Assert(e != null); } } } return e; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
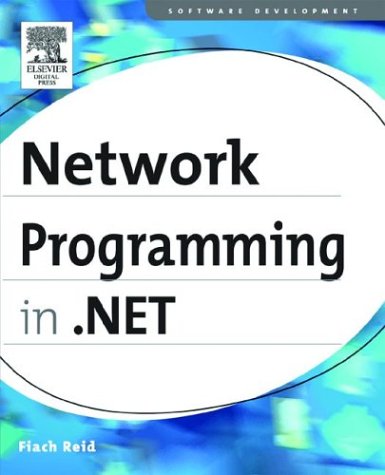
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BlockingCollection.cs
- ReservationCollection.cs
- DPAPIProtectedConfigurationProvider.cs
- URIFormatException.cs
- MulticastIPAddressInformationCollection.cs
- HasCopySemanticsAttribute.cs
- UndirectedGraph.cs
- SqlConnectionString.cs
- StateFinalizationActivity.cs
- JsonGlobals.cs
- UIElementAutomationPeer.cs
- WriteFileContext.cs
- InputScope.cs
- SigningCredentials.cs
- returneventsaver.cs
- DbXmlEnabledProviderManifest.cs
- HwndProxyElementProvider.cs
- WmpBitmapEncoder.cs
- EncoderExceptionFallback.cs
- XamlSerializerUtil.cs
- ConnectionInterfaceCollection.cs
- DataSpaceManager.cs
- ProfileProvider.cs
- _IPv6Address.cs
- ServicePointManager.cs
- GestureRecognizer.cs
- SchemaTableColumn.cs
- WorkflowItemPresenter.cs
- WindowsGraphicsCacheManager.cs
- GeneralTransform3DGroup.cs
- ComponentCollection.cs
- XmlTypeAttribute.cs
- SyndicationElementExtension.cs
- xml.cs
- TypeExtensionSerializer.cs
- WizardForm.cs
- Label.cs
- XhtmlConformanceSection.cs
- TextTreeDeleteContentUndoUnit.cs
- EditorZoneBase.cs
- CellNormalizer.cs
- _AutoWebProxyScriptWrapper.cs
- TabControlAutomationPeer.cs
- TreeNode.cs
- GroupBox.cs
- SafeNativeMethods.cs
- WorkflowMarkupSerializationManager.cs
- RootBrowserWindow.cs
- SatelliteContractVersionAttribute.cs
- BaseDataList.cs
- _NegoState.cs
- TextServicesCompartmentEventSink.cs
- ValidatingCollection.cs
- StrongNamePublicKeyBlob.cs
- DataTableMappingCollection.cs
- DoubleKeyFrameCollection.cs
- InternalBufferOverflowException.cs
- SendMailErrorEventArgs.cs
- PromptEventArgs.cs
- WebPartDisplayModeCancelEventArgs.cs
- ContainerFilterService.cs
- DesignParameter.cs
- Padding.cs
- ScriptModule.cs
- LayoutSettings.cs
- RegexMatch.cs
- TraceInternal.cs
- Light.cs
- HttpProfileBase.cs
- WindowProviderWrapper.cs
- MenuItemStyle.cs
- SimpleType.cs
- PropertyDescriptorComparer.cs
- MoveSizeWinEventHandler.cs
- MaskedTextBoxTextEditorDropDown.cs
- XmlDownloadManager.cs
- HttpListener.cs
- WinEventWrap.cs
- BamlLocalizer.cs
- DynamicObjectAccessor.cs
- XmlDesigner.cs
- DesignerActionUIStateChangeEventArgs.cs
- DefaultTextStore.cs
- FlowSwitchDesigner.xaml.cs
- TextPointerBase.cs
- WorkflowLayouts.cs
- DefaultSerializationProviderAttribute.cs
- ChtmlTextWriter.cs
- LogAppendAsyncResult.cs
- DefaultPropertyAttribute.cs
- XPathEmptyIterator.cs
- TextBox.cs
- AvTraceFormat.cs
- DataGridClipboardCellContent.cs
- Underline.cs
- CellTreeNode.cs
- _FixedSizeReader.cs
- ChtmlCalendarAdapter.cs
- WinEventTracker.cs
- HwndSourceParameters.cs