Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Animation / KeyTimeConverter.cs / 1 / KeyTimeConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeyTimeConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; namespace System.Windows { ////// /// public class KeyTimeConverter : TypeConverter { #region Data private static char[] _percentCharacter = new char[] { '%' }; #endregion ////// Returns whether or not this class can convert from a given type /// to an instance of a KeyTime. /// public override bool CanConvertFrom( ITypeDescriptorContext typeDescriptorContext, Type type) { if (type == typeof(string)) { return true; } else { return base.CanConvertFrom( typeDescriptorContext, type); } } ////// Returns whether or not this class can convert from an instance of a /// KeyTime to a given type. /// public override bool CanConvertTo( ITypeDescriptorContext typeDescriptorContext, Type type) { if ( type == typeof(InstanceDescriptor) || type == typeof(string)) { return true; } else { return base.CanConvertTo( typeDescriptorContext, type); } } ////// /// public override object ConvertFrom( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Uniform") { return KeyTime.Uniform; } else if (stringValue == "Paced") { return KeyTime.Paced; } else if (stringValue[stringValue.Length - 1] == _percentCharacter[0]) { stringValue = stringValue.TrimEnd(_percentCharacter); double doubleValue = (double)TypeDescriptor.GetConverter( typeof(double)).ConvertFrom( typeDescriptorContext, cultureInfo, stringValue); if (doubleValue == 0.0) { return KeyTime.FromPercent(0.0); } else if (doubleValue == 100.0) { return KeyTime.FromPercent(1.0); } else { return KeyTime.FromPercent(doubleValue / 100.0); } } else { TimeSpan timeSpanValue = (TimeSpan)TypeDescriptor.GetConverter( typeof(TimeSpan)).ConvertFrom( typeDescriptorContext, cultureInfo, stringValue); return KeyTime.FromTimeSpan(timeSpanValue); } } return base.ConvertFrom( typeDescriptorContext, cultureInfo, value); } ////// /// ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeyTime, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if ( value != null && value is KeyTime) { KeyTime keyTime = (KeyTime)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; switch (keyTime.Type) { case KeyTimeType.Percent: mi = typeof(KeyTime).GetMethod("FromPercent", new Type[] { typeof(double) }); return new InstanceDescriptor(mi, new object[] { keyTime.Percent }); case KeyTimeType.TimeSpan: mi = typeof(KeyTime).GetMethod("FromTimeSpan", new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { keyTime.TimeSpan }); case KeyTimeType.Uniform: mi = typeof(KeyTime).GetProperty("Uniform"); return new InstanceDescriptor(mi, null); case KeyTimeType.Paced: mi = typeof(KeyTime).GetProperty("Paced"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(String)) { switch (keyTime.Type) { case KeyTimeType.Uniform: return "Uniform"; case KeyTimeType.Paced: return "Paced"; case KeyTimeType.Percent: string returnValue = (string)TypeDescriptor.GetConverter( typeof(Double)).ConvertTo( typeDescriptorContext, cultureInfo, keyTime.Percent * 100.0, destinationType); return returnValue + _percentCharacter[0].ToString(); case KeyTimeType.TimeSpan: return TypeDescriptor.GetConverter( typeof(TimeSpan)).ConvertTo( typeDescriptorContext, cultureInfo, keyTime.TimeSpan, destinationType); } } } return base.ConvertTo( typeDescriptorContext, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
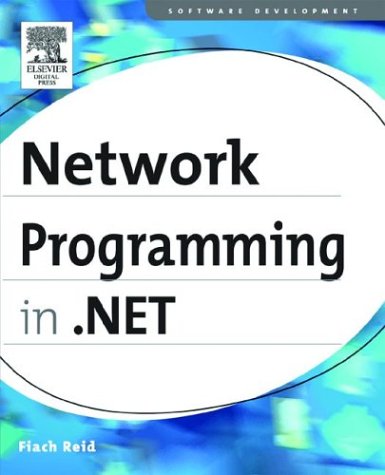
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializerProvider.cs
- MergablePropertyAttribute.cs
- ThreadAttributes.cs
- DecimalConstantAttribute.cs
- DataRecordInternal.cs
- TableCellCollection.cs
- DataGridTablesFactory.cs
- JavaScriptSerializer.cs
- SuppressMergeCheckAttribute.cs
- assemblycache.cs
- ZoomPercentageConverter.cs
- BindingGroup.cs
- Size3D.cs
- SlipBehavior.cs
- AutomationElementCollection.cs
- LocatorBase.cs
- StyleHelper.cs
- SwitchAttribute.cs
- TextEditorContextMenu.cs
- AppSettingsSection.cs
- CaseStatementSlot.cs
- ComponentCommands.cs
- IPGlobalProperties.cs
- FamilyTypeface.cs
- GenericsInstances.cs
- Ppl.cs
- CellLabel.cs
- SemanticAnalyzer.cs
- ListViewItem.cs
- MailBnfHelper.cs
- ToolStripCollectionEditor.cs
- Rect3DConverter.cs
- X509ThumbprintKeyIdentifierClause.cs
- MetadataCacheItem.cs
- MatcherBuilder.cs
- _RequestCacheProtocol.cs
- BindUriHelper.cs
- ProfileParameter.cs
- ToolStripGrip.cs
- httpstaticobjectscollection.cs
- RawAppCommandInputReport.cs
- ControlUtil.cs
- OrderedDictionaryStateHelper.cs
- Size.cs
- AssemblyUtil.cs
- FileDialog.cs
- DataGridView.cs
- ChannelManager.cs
- ObjectManager.cs
- WebResponse.cs
- DesignerSerializationOptionsAttribute.cs
- VisualTreeHelper.cs
- RegexCapture.cs
- ContentElementAutomationPeer.cs
- DoubleLinkListEnumerator.cs
- DispatchRuntime.cs
- SessionPageStateSection.cs
- FrameworkPropertyMetadata.cs
- DependencyProperty.cs
- DataTemplate.cs
- EditingCommands.cs
- UIElementAutomationPeer.cs
- ServerValidateEventArgs.cs
- EntityEntry.cs
- SEHException.cs
- DispatcherTimer.cs
- StorageEntitySetMapping.cs
- MSAAEventDispatcher.cs
- RtfFormatStack.cs
- PropertyItemInternal.cs
- SqlExpressionNullability.cs
- AsyncSerializedWorker.cs
- EncodingNLS.cs
- ApplyHostConfigurationBehavior.cs
- RbTree.cs
- _NetworkingPerfCounters.cs
- Completion.cs
- InvalidPipelineStoreException.cs
- PenThreadPool.cs
- IFlowDocumentViewer.cs
- DocumentSchemaValidator.cs
- TabPage.cs
- ConfigurationSection.cs
- IdentityReference.cs
- ManagedWndProcTracker.cs
- StreamingContext.cs
- TableRow.cs
- BaseServiceProvider.cs
- FactoryGenerator.cs
- SqlTypesSchemaImporter.cs
- COM2TypeInfoProcessor.cs
- PostBackOptions.cs
- SafeUserTokenHandle.cs
- Exception.cs
- BlurEffect.cs
- ClientRolePrincipal.cs
- StubHelpers.cs
- StringCollection.cs
- InputLanguageCollection.cs
- RoutedEvent.cs