Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / SetterTriggerConditionValueConverter.cs / 1305600 / SetterTriggerConditionValueConverter.cs
/****************************************************************************\ * * File: SetterTriggerConditionValueConverter.cs * * Class for converting a given value (for Setter/Trigger/Condition) to and from a string * * Copyright (C) 2007 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using MS.Utility; using MS.Internal; using System.Windows; using System.ComponentModel.Design.Serialization; using System.Windows.Documents; using System.Xaml; using System.Xaml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.IO; namespace System.Windows.Markup { ////// Class for converting a given DependencyProperty to and from a string /// public sealed class SetterTriggerConditionValueConverter : TypeConverter { #region Public Methods ////// CanConvertFrom() /// /// ITypeDescriptorContext /// type to convert from ///true if the given type can be converted, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only convert from a string and that too only if we have all the contextual information // Note: Sometimes even the serializer calls CanConvertFrom in order // to determine if it is a valid converter to use for serialization. if (sourceType == typeof(string) || sourceType == typeof(byte[])) { return true; } return false; } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return false; } ////// ConvertFrom() -TypeConverter method override. using the givein name to return DependencyProperty /// /// ITypeDescriptorContext /// CultureInfo /// Object to convert from ///instance of Command public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { return SetterTriggerConditionValueConverter.ResolveValue(context, null, culture, source); } // ////// ConvertTo() - Serialization purposes, returns the string from Command.Name by adding ownerType.FullName /// /// ITypeDescriptorContext /// CultureInfo /// the object to convert from /// the type to convert to ///string object, if the destination type is string public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { throw GetConvertToException(value, destinationType); } #endregion Public Methods internal static object ResolveValue(ITypeDescriptorContext serviceProvider, DependencyProperty property, CultureInfo culture, object source) { if (serviceProvider == null) { throw new ArgumentNullException("serviceProvider"); } if (source == null) { throw new ArgumentNullException("source"); } // Only need to type convert strings and byte[] if (!(source is byte[] || source is String || source is Stream)) { return source; } IXamlSchemaContextProvider ixsc = (serviceProvider.GetService(typeof(IXamlSchemaContextProvider)) as IXamlSchemaContextProvider); if (ixsc == null) { throw new NotSupportedException(SR.Get(SRID.ParserCannotConvertPropertyValue, "Value", typeof(Object).FullName)); } XamlSchemaContext schemaContext = ixsc.SchemaContext; if (property != null) { //Get XamlMember from dp System.Xaml.XamlMember xamlProperty = schemaContext.GetXamlType(property.OwnerType).GetMember(property.Name); if (xamlProperty == null) xamlProperty = schemaContext.GetXamlType(property.OwnerType).GetAttachableMember(property.Name); System.Xaml.Schema.XamlValueConvertertypeConverter = null; if (xamlProperty != null) { // If we have a Baml2006SchemaContext and the property is of type Enum, we already know that the // type converter must be the EnumConverter. if (xamlProperty.Type.UnderlyingType.IsEnum && schemaContext is Baml2006.Baml2006SchemaContext) { typeConverter = XamlReader.BamlSharedSchemaContext.GetTypeConverter(xamlProperty.Type.UnderlyingType); } else { typeConverter = xamlProperty.TypeConverter; if (typeConverter == null) { typeConverter = xamlProperty.Type.TypeConverter; } } } else { typeConverter = schemaContext.GetXamlType(property.PropertyType).TypeConverter; } // No Type converter case... if (typeConverter.ConverterType == null) { return source; } TypeConverter converter = null; if (xamlProperty != null && xamlProperty.Type.UnderlyingType == typeof(Boolean)) { if (source is String) { converter = new BooleanConverter(); } else if (source is byte[]) { byte[] bytes = source as byte[]; if (bytes != null && bytes.Length == 1) { return (bytes[0] != 0); } else { throw new NotSupportedException(SR.Get(SRID.ParserCannotConvertPropertyValue, "Value", typeof(Object).FullName)); } } else { throw new NotSupportedException(SR.Get(SRID.ParserCannotConvertPropertyValue, "Value", typeof(Object).FullName)); } } else { converter = (TypeConverter)typeConverter.ConverterInstance; } return converter.ConvertFrom(serviceProvider, culture, source); } else { return source; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: SetterTriggerConditionValueConverter.cs * * Class for converting a given value (for Setter/Trigger/Condition) to and from a string * * Copyright (C) 2007 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using MS.Utility; using MS.Internal; using System.Windows; using System.ComponentModel.Design.Serialization; using System.Windows.Documents; using System.Xaml; using System.Xaml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.IO; namespace System.Windows.Markup { /// /// Class for converting a given DependencyProperty to and from a string /// public sealed class SetterTriggerConditionValueConverter : TypeConverter { #region Public Methods ////// CanConvertFrom() /// /// ITypeDescriptorContext /// type to convert from ///true if the given type can be converted, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only convert from a string and that too only if we have all the contextual information // Note: Sometimes even the serializer calls CanConvertFrom in order // to determine if it is a valid converter to use for serialization. if (sourceType == typeof(string) || sourceType == typeof(byte[])) { return true; } return false; } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return false; } ////// ConvertFrom() -TypeConverter method override. using the givein name to return DependencyProperty /// /// ITypeDescriptorContext /// CultureInfo /// Object to convert from ///instance of Command public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { return SetterTriggerConditionValueConverter.ResolveValue(context, null, culture, source); } // ////// ConvertTo() - Serialization purposes, returns the string from Command.Name by adding ownerType.FullName /// /// ITypeDescriptorContext /// CultureInfo /// the object to convert from /// the type to convert to ///string object, if the destination type is string public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { throw GetConvertToException(value, destinationType); } #endregion Public Methods internal static object ResolveValue(ITypeDescriptorContext serviceProvider, DependencyProperty property, CultureInfo culture, object source) { if (serviceProvider == null) { throw new ArgumentNullException("serviceProvider"); } if (source == null) { throw new ArgumentNullException("source"); } // Only need to type convert strings and byte[] if (!(source is byte[] || source is String || source is Stream)) { return source; } IXamlSchemaContextProvider ixsc = (serviceProvider.GetService(typeof(IXamlSchemaContextProvider)) as IXamlSchemaContextProvider); if (ixsc == null) { throw new NotSupportedException(SR.Get(SRID.ParserCannotConvertPropertyValue, "Value", typeof(Object).FullName)); } XamlSchemaContext schemaContext = ixsc.SchemaContext; if (property != null) { //Get XamlMember from dp System.Xaml.XamlMember xamlProperty = schemaContext.GetXamlType(property.OwnerType).GetMember(property.Name); if (xamlProperty == null) xamlProperty = schemaContext.GetXamlType(property.OwnerType).GetAttachableMember(property.Name); System.Xaml.Schema.XamlValueConvertertypeConverter = null; if (xamlProperty != null) { // If we have a Baml2006SchemaContext and the property is of type Enum, we already know that the // type converter must be the EnumConverter. if (xamlProperty.Type.UnderlyingType.IsEnum && schemaContext is Baml2006.Baml2006SchemaContext) { typeConverter = XamlReader.BamlSharedSchemaContext.GetTypeConverter(xamlProperty.Type.UnderlyingType); } else { typeConverter = xamlProperty.TypeConverter; if (typeConverter == null) { typeConverter = xamlProperty.Type.TypeConverter; } } } else { typeConverter = schemaContext.GetXamlType(property.PropertyType).TypeConverter; } // No Type converter case... if (typeConverter.ConverterType == null) { return source; } TypeConverter converter = null; if (xamlProperty != null && xamlProperty.Type.UnderlyingType == typeof(Boolean)) { if (source is String) { converter = new BooleanConverter(); } else if (source is byte[]) { byte[] bytes = source as byte[]; if (bytes != null && bytes.Length == 1) { return (bytes[0] != 0); } else { throw new NotSupportedException(SR.Get(SRID.ParserCannotConvertPropertyValue, "Value", typeof(Object).FullName)); } } else { throw new NotSupportedException(SR.Get(SRID.ParserCannotConvertPropertyValue, "Value", typeof(Object).FullName)); } } else { converter = (TypeConverter)typeConverter.ConverterInstance; } return converter.ConvertFrom(serviceProvider, culture, source); } else { return source; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
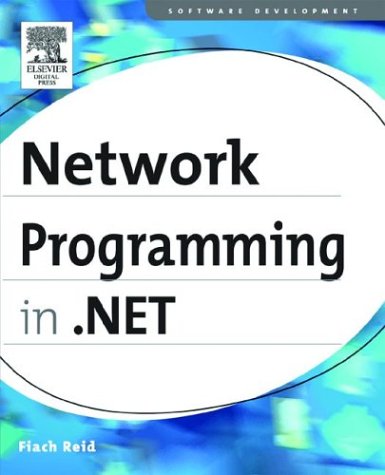
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MultiBindingExpression.cs
- ShowExpandedMultiValueConverter.cs
- UdpUtility.cs
- HtmlObjectListAdapter.cs
- ConnectionsZone.cs
- DashStyles.cs
- DataContractSerializer.cs
- ListViewPagedDataSource.cs
- PointLightBase.cs
- SafeHandles.cs
- TrueReadOnlyCollection.cs
- XsdBuildProvider.cs
- _Semaphore.cs
- SimpleWebHandlerParser.cs
- HierarchicalDataBoundControl.cs
- PolyLineSegment.cs
- SiblingIterators.cs
- ExpressionEvaluator.cs
- DependencyObjectPropertyDescriptor.cs
- StructuredProperty.cs
- PeerDefaultCustomResolverClient.cs
- Timer.cs
- AsyncResult.cs
- Part.cs
- ChangesetResponse.cs
- ComplexType.cs
- PartialTrustHelpers.cs
- TransformationRules.cs
- LeafCellTreeNode.cs
- StateMachineExecutionState.cs
- Wildcard.cs
- ConstructorBuilder.cs
- StrokeCollection2.cs
- DelegatingTypeDescriptionProvider.cs
- BamlRecordWriter.cs
- OracleInfoMessageEventArgs.cs
- WebPartHeaderCloseVerb.cs
- HttpStaticObjectsCollectionWrapper.cs
- newitemfactory.cs
- NavigateEvent.cs
- ResumeStoryboard.cs
- GPPOINT.cs
- SizeFConverter.cs
- LinqDataSourceSelectEventArgs.cs
- CDSCollectionETWBCLProvider.cs
- BamlResourceSerializer.cs
- cache.cs
- ServiceBehaviorElementCollection.cs
- DataSourceView.cs
- WebResourceUtil.cs
- FacetChecker.cs
- EarlyBoundInfo.cs
- ActivationServices.cs
- HebrewNumber.cs
- GenerateScriptTypeAttribute.cs
- ServiceModelStringsVersion1.cs
- Rule.cs
- ColumnResizeAdorner.cs
- IndexedDataBuffer.cs
- CodeNamespaceImport.cs
- ChameleonKey.cs
- LinkLabelLinkClickedEvent.cs
- HtmlHead.cs
- ISessionStateStore.cs
- WebBodyFormatMessageProperty.cs
- LinqDataSourceValidationException.cs
- ValidationException.cs
- CroppedBitmap.cs
- xml.cs
- MetafileHeader.cs
- QilVisitor.cs
- IgnorePropertiesAttribute.cs
- ContextMenuStripActionList.cs
- DataGrid.cs
- MSAANativeProvider.cs
- SrgsRule.cs
- SessionEndingEventArgs.cs
- DataGridViewColumnTypePicker.cs
- XmlDesignerDataSourceView.cs
- DataGridTable.cs
- Size.cs
- GridItemPattern.cs
- MatrixConverter.cs
- ProfileGroupSettings.cs
- ProxyAttribute.cs
- DefaultValueMapping.cs
- SerializableAttribute.cs
- WebPartDisplayModeCollection.cs
- NativeCppClassAttribute.cs
- InkSerializer.cs
- ObjectDataProvider.cs
- CodeAttributeArgument.cs
- AttributeAction.cs
- SqlConnectionPoolProviderInfo.cs
- PathSegmentCollection.cs
- ConditionCollection.cs
- TypeNameConverter.cs
- WebConfigurationManager.cs
- ScriptControlManager.cs
- Ref.cs