Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Debugger / InstrumentationTracker.cs / 1305376 / InstrumentationTracker.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Debugger { using System; using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Linq.Expressions; using System.IO; using System.Runtime; // Keep track of instrumentation information. // - which subroot has source file but not yet instrumented. // - which subroots share the same source file // SubRoot is defined as an activity that has a source file // (Custom Activity). class InstrumentationTracker { // Root of the workflow to keep track. Activity root; // Mapping of subroots to their source files. DictionaryuninstrumentedSubRoots; Dictionary UninstrumentedSubRoots { get { if (this.uninstrumentedSubRoots == null) { InitializeUninstrumentedSubRoots(); } return this.uninstrumentedSubRoots; } } public InstrumentationTracker(Activity root) { this.root = root; } // Initialize UninstrumentedSubRoots by traversing the workflow. void InitializeUninstrumentedSubRoots() { this.uninstrumentedSubRoots = new Dictionary (); Queue activitiesRemaining = new Queue (); CollectSubRoot(this.root); activitiesRemaining.Enqueue(this.root); while (activitiesRemaining.Count > 0) { Activity toProcess = activitiesRemaining.Dequeue(); foreach (Activity activity in WorkflowInspectionServices.GetActivities(toProcess)) { CollectSubRoot(activity); activitiesRemaining.Enqueue(activity); } } } // Collect subroot as uninstrumented activity. void CollectSubRoot(Activity activity) { string sourcePath = XamlDebuggerXmlReader.GetFileName(activity) as string; if (!string.IsNullOrEmpty(sourcePath)) { this.uninstrumentedSubRoots.Add(activity, sourcePath); } } // Whether this is unistrumented sub root. public bool IsUninstrumentedSubRoot(Activity subRoot) { return this.UninstrumentedSubRoots.ContainsKey(subRoot); } // Returns Activities that have the same source as the given subRoot. // This will return other instantiation of the same custom activity. // Needed to avoid re-instrumentation of the same file. public List GetSameSourceSubRoots(Activity subRoot) { string sourcePath; List sameSourceSubRoots = new List (); if (this.UninstrumentedSubRoots.TryGetValue(subRoot, out sourcePath)) { foreach (KeyValuePair entry in this.UninstrumentedSubRoots) { if (entry.Value == sourcePath && entry.Key != subRoot) { sameSourceSubRoots.Add(entry.Key); } } } return sameSourceSubRoots; } // Mark this sub root as instrumented. public void MarkInstrumented(Activity subRoot) { this.UninstrumentedSubRoots.Remove(subRoot); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
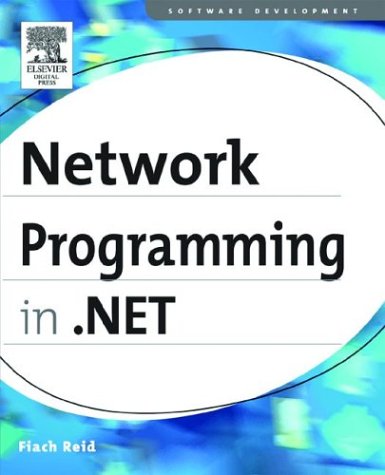
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackWebRequestFactory.cs
- CodeObjectCreateExpression.cs
- StrokeNodeEnumerator.cs
- MasterPageBuildProvider.cs
- IPAddressCollection.cs
- XmlBoundElement.cs
- VirtualDirectoryMappingCollection.cs
- VersionedStreamOwner.cs
- CategoryValueConverter.cs
- BindingList.cs
- CqlLexer.cs
- ComplexLine.cs
- SmiRecordBuffer.cs
- Geometry.cs
- EndpointDiscoveryBehavior.cs
- RequestTimeoutManager.cs
- ColorPalette.cs
- DbParameterCollection.cs
- DocumentReference.cs
- ToolStripDropDownClosedEventArgs.cs
- WizardStepCollectionEditor.cs
- DocumentViewerBaseAutomationPeer.cs
- StorageRoot.cs
- HttpConfigurationSystem.cs
- SelectionRangeConverter.cs
- CellParagraph.cs
- TargetConverter.cs
- CookielessHelper.cs
- CodeVariableReferenceExpression.cs
- AttributeSetAction.cs
- SlotInfo.cs
- AsyncContentLoadedEventArgs.cs
- SerializableAttribute.cs
- FunctionQuery.cs
- InputReportEventArgs.cs
- InheritanceRules.cs
- DataGridViewColumnDesigner.cs
- ContextMarshalException.cs
- ControlIdConverter.cs
- RectKeyFrameCollection.cs
- AutomationElement.cs
- MappedMetaModel.cs
- Wildcard.cs
- RuntimeHandles.cs
- WinInetCache.cs
- SqlNodeTypeOperators.cs
- basecomparevalidator.cs
- LinkButton.cs
- DiscoveryReferences.cs
- DiscreteKeyFrames.cs
- _RegBlobWebProxyDataBuilder.cs
- TdsParameterSetter.cs
- Operators.cs
- ForEachDesigner.xaml.cs
- Cursors.cs
- InfoCardSymmetricCrypto.cs
- Validator.cs
- MenuItemStyle.cs
- ContextMarshalException.cs
- Win32SafeHandles.cs
- IconBitmapDecoder.cs
- TransformerTypeCollection.cs
- Gdiplus.cs
- RegistrationServices.cs
- PeerPresenceInfo.cs
- Pens.cs
- LOSFormatter.cs
- TimelineClockCollection.cs
- WebPartAuthorizationEventArgs.cs
- exports.cs
- ProfileGroupSettings.cs
- login.cs
- TriState.cs
- RoutedUICommand.cs
- TaskFactory.cs
- DatatypeImplementation.cs
- RegisteredArrayDeclaration.cs
- StringStorage.cs
- SoapCodeExporter.cs
- DataColumnChangeEvent.cs
- ShadowGlyph.cs
- TabControl.cs
- LayoutEditorPart.cs
- SchemaHelper.cs
- XmlRootAttribute.cs
- ProfileProvider.cs
- RequestCacheEntry.cs
- FixedHyperLink.cs
- InternalConfigEventArgs.cs
- AuthorizationRule.cs
- SqlXmlStorage.cs
- ErrorEventArgs.cs
- _SSPIWrapper.cs
- BinaryExpression.cs
- RuntimeArgumentHandle.cs
- _ListenerAsyncResult.cs
- WebPartVerb.cs
- DataSetFieldSchema.cs
- ResourceSetExpression.cs
- PeerNameResolver.cs