Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / IO / Packaging / PackWebRequestFactory.cs / 1 / PackWebRequestFactory.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Creates a PackWebRequest object // // History: // 10/09/2003: BruceMac: Created. // 05/10/2004: BruceMac: Rework for Pack scheme (from CompoundFile scheme) // 05/26/2004: BruceMac: Use ILoader cache instead of PackageCache // 04/26/2005: BruceMac: Use PackUriHelper to obtain Authority for cache lookup // //----------------------------------------------------------------------------- #if DEBUG #define TRACE #endif using System; using System.Net; using System.Diagnostics; // for Assert using MS.Internal.IO.Packaging; // for PackageCache using MS.Internal.PresentationCore; // for ExceptionStringTable using System.Security; using System.Security.Permissions; using MS.Internal; namespace System.IO.Packaging { ////// Invoked by .NET framework when our schema is recognized during a WebRequest /// public sealed class PackWebRequestFactory : IWebRequestCreate { ////// Critical as the BooleanSwitch has a LinkDemand /// TreatAsSafe as this is just a diag switch, Debug-only, no input data is used, /// and the usage is considered safe (tracing). /// [SecurityCritical, SecurityTreatAsSafe] static PackWebRequestFactory() { #if DEBUG _traceSwitch = new BooleanSwitch("PackWebRequest", "PackWebRequest/Response and NetStream trace messages"); #endif } //----------------------------------------------------- // // IWebRequestCreate // //----------------------------------------------------- ////// Create /// /// uri ///PackWebRequest ///Note that this factory may or may not be "registered" with the .NET WebRequest factory as handler /// for "pack" scheme web requests. Because of this, callers should be sure to use the PackUriHelper static class /// to prepare their Uri's. Calling any PackUriHelper method has the side effect of registering /// the "pack" scheme and associating this factory class as its default handler. ////// Critical: Access a package instance from PreloadedPackages. /// TreatAsSafe: because it is a public method and no Package related objects /// are given out from this API other than a part stream /// [SecurityCritical, SecurityTreatAsSafe] WebRequest IWebRequestCreate.Create(Uri uri) { if (uri == null) throw new ArgumentNullException("uri"); // Ensure uri is absolute - if we don't check now, the get_Scheme property will throw // InvalidOperationException which would be misleading to the caller. if (!uri.IsAbsoluteUri) throw new ArgumentException(SR.Get(SRID.UriMustBeAbsolute), "uri"); // Ensure uri is correct scheme because we can be called directly. Case sensitive // is fine because Uri.Scheme contract is to return in lower case only. if (String.Compare(uri.Scheme, PackUriHelper.UriSchemePack, StringComparison.Ordinal) != 0) throw new ArgumentException(SR.Get(SRID.UriSchemeMismatch, PackUriHelper.UriSchemePack), "uri"); #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": " + "PackWebRequestFactory - responding to uri: " + uri); #endif // only inspect cache if part name is present because cache only contains an object, not // the stream it was derived from Uri packageUri; Uri partUri; PackUriHelper.ValidateAndGetPackUriComponents(uri, out packageUri, out partUri); if (partUri != null) { // Note: we look at PreloadedPackages first before we examine the PackageStore // This is to make sure that an app cannot override any predefine packages // match cached object by authority component only - ignore the local path (part name) // inspect local package cache and default to that if possible // All predefined packages such as a package activated by DocumentApplication, // ResourceContainer, and SiteOfOriginContainer are placed in PreloadedPackages bool cachedPackageIsThreadSafe; Package c = PreloadedPackages.GetPackage(packageUri, out cachedPackageIsThreadSafe); // If we don't find anything in the preloaded packages, look into the PackageStore bool cachedPackageIsFromPublicStore = false; if (c == null) { cachedPackageIsThreadSafe = false; // always assume PackageStore packages are not thread-safe cachedPackageIsFromPublicStore = true; // Try to get a package from the package store c = PackageStore.GetPackage(packageUri); } // do we have a package? if (c != null) { #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": " + "PackWebRequestFactory - cache hit - returning CachedPackWebRequest"); #endif // use the cached object return new PackWebRequest(uri, packageUri, partUri, c, cachedPackageIsFromPublicStore, cachedPackageIsThreadSafe); } } #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": " + "PackWebRequestFactory - spawning regular PackWebRequest"); #endif return new PackWebRequest(uri, packageUri, partUri); } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- // CreateWebRequest: Explicitly calls Create on PackWebRequest if uri is pack scheme // Ideally we would want to use RegisterPrefix and WebRequest.Create. // However, these two functions regress 700k working set in System.dll and System.xml.dll // which is mostly for logging and config. // This helper function provides a way to bypass the regression [FriendAccessAllowed] internal static WebRequest CreateWebRequest(Uri uri) { if (String.Compare(uri.Scheme, PackUriHelper.UriSchemePack, StringComparison.Ordinal) == 0) { return ((IWebRequestCreate) _factorySingleton).Create(uri); } else { return WpfWebRequestHelper.CreateRequest(uri); } } #if DEBUG ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing, and it's in debug only /// internal static bool TraceSwitchEnabled { get { return _traceSwitch.Enabled; } [SecurityCritical, SecurityTreatAsSafe] set { _traceSwitch.Enabled = value; } } internal static System.Diagnostics.BooleanSwitch _traceSwitch; #endif //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private static PackWebRequestFactory _factorySingleton = new PackWebRequestFactory(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Creates a PackWebRequest object // // History: // 10/09/2003: BruceMac: Created. // 05/10/2004: BruceMac: Rework for Pack scheme (from CompoundFile scheme) // 05/26/2004: BruceMac: Use ILoader cache instead of PackageCache // 04/26/2005: BruceMac: Use PackUriHelper to obtain Authority for cache lookup // //----------------------------------------------------------------------------- #if DEBUG #define TRACE #endif using System; using System.Net; using System.Diagnostics; // for Assert using MS.Internal.IO.Packaging; // for PackageCache using MS.Internal.PresentationCore; // for ExceptionStringTable using System.Security; using System.Security.Permissions; using MS.Internal; namespace System.IO.Packaging { ////// Invoked by .NET framework when our schema is recognized during a WebRequest /// public sealed class PackWebRequestFactory : IWebRequestCreate { ////// Critical as the BooleanSwitch has a LinkDemand /// TreatAsSafe as this is just a diag switch, Debug-only, no input data is used, /// and the usage is considered safe (tracing). /// [SecurityCritical, SecurityTreatAsSafe] static PackWebRequestFactory() { #if DEBUG _traceSwitch = new BooleanSwitch("PackWebRequest", "PackWebRequest/Response and NetStream trace messages"); #endif } //----------------------------------------------------- // // IWebRequestCreate // //----------------------------------------------------- ////// Create /// /// uri ///PackWebRequest ///Note that this factory may or may not be "registered" with the .NET WebRequest factory as handler /// for "pack" scheme web requests. Because of this, callers should be sure to use the PackUriHelper static class /// to prepare their Uri's. Calling any PackUriHelper method has the side effect of registering /// the "pack" scheme and associating this factory class as its default handler. ////// Critical: Access a package instance from PreloadedPackages. /// TreatAsSafe: because it is a public method and no Package related objects /// are given out from this API other than a part stream /// [SecurityCritical, SecurityTreatAsSafe] WebRequest IWebRequestCreate.Create(Uri uri) { if (uri == null) throw new ArgumentNullException("uri"); // Ensure uri is absolute - if we don't check now, the get_Scheme property will throw // InvalidOperationException which would be misleading to the caller. if (!uri.IsAbsoluteUri) throw new ArgumentException(SR.Get(SRID.UriMustBeAbsolute), "uri"); // Ensure uri is correct scheme because we can be called directly. Case sensitive // is fine because Uri.Scheme contract is to return in lower case only. if (String.Compare(uri.Scheme, PackUriHelper.UriSchemePack, StringComparison.Ordinal) != 0) throw new ArgumentException(SR.Get(SRID.UriSchemeMismatch, PackUriHelper.UriSchemePack), "uri"); #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": " + "PackWebRequestFactory - responding to uri: " + uri); #endif // only inspect cache if part name is present because cache only contains an object, not // the stream it was derived from Uri packageUri; Uri partUri; PackUriHelper.ValidateAndGetPackUriComponents(uri, out packageUri, out partUri); if (partUri != null) { // Note: we look at PreloadedPackages first before we examine the PackageStore // This is to make sure that an app cannot override any predefine packages // match cached object by authority component only - ignore the local path (part name) // inspect local package cache and default to that if possible // All predefined packages such as a package activated by DocumentApplication, // ResourceContainer, and SiteOfOriginContainer are placed in PreloadedPackages bool cachedPackageIsThreadSafe; Package c = PreloadedPackages.GetPackage(packageUri, out cachedPackageIsThreadSafe); // If we don't find anything in the preloaded packages, look into the PackageStore bool cachedPackageIsFromPublicStore = false; if (c == null) { cachedPackageIsThreadSafe = false; // always assume PackageStore packages are not thread-safe cachedPackageIsFromPublicStore = true; // Try to get a package from the package store c = PackageStore.GetPackage(packageUri); } // do we have a package? if (c != null) { #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": " + "PackWebRequestFactory - cache hit - returning CachedPackWebRequest"); #endif // use the cached object return new PackWebRequest(uri, packageUri, partUri, c, cachedPackageIsFromPublicStore, cachedPackageIsThreadSafe); } } #if DEBUG if (_traceSwitch.Enabled) System.Diagnostics.Trace.TraceInformation( DateTime.Now.ToLongTimeString() + " " + DateTime.Now.Millisecond + " " + System.Threading.Thread.CurrentThread.ManagedThreadId + ": " + "PackWebRequestFactory - spawning regular PackWebRequest"); #endif return new PackWebRequest(uri, packageUri, partUri); } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- // CreateWebRequest: Explicitly calls Create on PackWebRequest if uri is pack scheme // Ideally we would want to use RegisterPrefix and WebRequest.Create. // However, these two functions regress 700k working set in System.dll and System.xml.dll // which is mostly for logging and config. // This helper function provides a way to bypass the regression [FriendAccessAllowed] internal static WebRequest CreateWebRequest(Uri uri) { if (String.Compare(uri.Scheme, PackUriHelper.UriSchemePack, StringComparison.Ordinal) == 0) { return ((IWebRequestCreate) _factorySingleton).Create(uri); } else { return WpfWebRequestHelper.CreateRequest(uri); } } #if DEBUG ////// Critical: sets BooleanSwitch.Enabled which LinkDemands /// TreatAsSafe: ok to enable tracing, and it's in debug only /// internal static bool TraceSwitchEnabled { get { return _traceSwitch.Enabled; } [SecurityCritical, SecurityTreatAsSafe] set { _traceSwitch.Enabled = value; } } internal static System.Diagnostics.BooleanSwitch _traceSwitch; #endif //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private static PackWebRequestFactory _factorySingleton = new PackWebRequestFactory(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
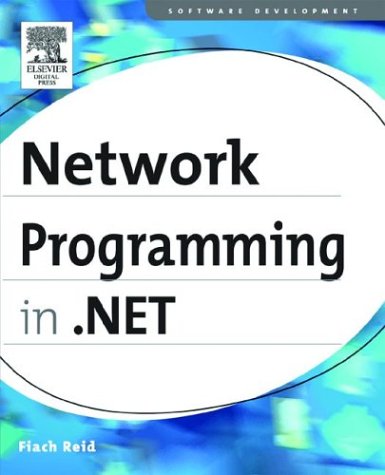
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MatrixTransform.cs
- SecurityCapabilities.cs
- Pen.cs
- ErrorWebPart.cs
- DataSourceSelectArguments.cs
- AssemblyResourceLoader.cs
- ExpandedWrapper.cs
- SeekStoryboard.cs
- SrgsElementFactory.cs
- XmlDataSource.cs
- Int32Converter.cs
- SmtpMail.cs
- ProfessionalColorTable.cs
- WindowsListView.cs
- FrameworkEventSource.cs
- CircleEase.cs
- FolderLevelBuildProviderAppliesToAttribute.cs
- IProvider.cs
- TextEditorLists.cs
- WebPartVerbsEventArgs.cs
- ClientTarget.cs
- ObjectCloneHelper.cs
- ErrorFormatter.cs
- ProjectedSlot.cs
- FormViewDeleteEventArgs.cs
- FixedHighlight.cs
- Control.cs
- SecurityKeyIdentifier.cs
- SimpleApplicationHost.cs
- ChtmlTextWriter.cs
- ControlType.cs
- documentation.cs
- Utilities.cs
- PointLightBase.cs
- StorageComplexTypeMapping.cs
- SpeechUI.cs
- RelOps.cs
- TableFieldsEditor.cs
- MonikerBuilder.cs
- RootBuilder.cs
- DataGridViewRow.cs
- BaseTreeIterator.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- TextServicesManager.cs
- MimeMultiPart.cs
- WebEncodingValidatorAttribute.cs
- MailAddress.cs
- AssemblyFilter.cs
- ShaderEffect.cs
- Triplet.cs
- ChannelAcceptor.cs
- ListViewUpdateEventArgs.cs
- SemanticKeyElement.cs
- BulletedListDesigner.cs
- PermissionSetTriple.cs
- EncodingTable.cs
- ApplicationException.cs
- XmlFormatExtensionPrefixAttribute.cs
- ProvidersHelper.cs
- TextStore.cs
- MergeFilterQuery.cs
- GlobalItem.cs
- PropertyPathConverter.cs
- SatelliteContractVersionAttribute.cs
- safex509handles.cs
- TextRangeEditLists.cs
- RegexCaptureCollection.cs
- WindowsGraphicsCacheManager.cs
- XPathNodeHelper.cs
- CompressionTracing.cs
- CommandDesigner.cs
- TypeResolvingOptionsAttribute.cs
- SqlClientWrapperSmiStreamChars.cs
- CommonXSendMessage.cs
- ClrProviderManifest.cs
- IdentityHolder.cs
- FloatAverageAggregationOperator.cs
- XPathNavigatorKeyComparer.cs
- ValidatingReaderNodeData.cs
- ipaddressinformationcollection.cs
- XmlNodeChangedEventArgs.cs
- ExecutionContext.cs
- XmlException.cs
- IconBitmapDecoder.cs
- Base64Encoder.cs
- SynchronizedPool.cs
- CharConverter.cs
- IntAverageAggregationOperator.cs
- UnmanagedMemoryStream.cs
- ExpressionSelection.cs
- GraphicsPathIterator.cs
- OleDbSchemaGuid.cs
- PropertyPushdownHelper.cs
- SqlDataSourceSelectingEventArgs.cs
- PageContent.cs
- CompatibleComparer.cs
- DashStyle.cs
- FrameworkElement.cs
- TabletDevice.cs
- CodeDirectiveCollection.cs