Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebParts / ErrorWebPart.cs / 1 / ErrorWebPart.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Globalization; using System.Security.Permissions; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Util; [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ErrorWebPart : ProxyWebPart, ITrackingPersonalizable { private string _errorMessage; // No constructor that takes a WebPart, since we ony use the ErrorWebPart when the original // WebPart could not be instantiated. public ErrorWebPart(string originalID, string originalTypeName, string originalPath, string genericWebPartID) : base(originalID, originalTypeName, originalPath, genericWebPartID) { } public string ErrorMessage { get { return (_errorMessage != null) ? _errorMessage : String.Empty; } set { _errorMessage = value; } } protected override void AddAttributesToRender(HtmlTextWriter writer) { WebPartZoneBase zone = Zone; if (zone != null && !zone.ErrorStyle.IsEmpty) { zone.ErrorStyle.AddAttributesToRender(writer, this); } base.AddAttributesToRender(writer); } // Can be overridden by derived classes to set properties protected virtual void EndLoadPersonalization() { // We don't really need to set AllowEdit, since EditorPart.Display has // a special case for ErrorWebPart. However, let's set it to false anyway // for consistency. AllowEdit = false; // We want to force the user to see the ErrorWebPart, and we don't want to allow // them to hide or minimize it. ChromeState = PartChromeState.Normal; Hidden = false; AllowHide = false; AllowMinimize = false; // There is no reason to allow exporting an ErrorWebPart. ExportMode = WebPartExportMode.None; // We never call IsAuthorized() on ErrorWebParts, so there is no reason for // AuthorizationFilter to be set. AuthorizationFilter = String.Empty; } protected internal override void RenderContents(HtmlTextWriter writer) { string errorMessage = ErrorMessage; if (!String.IsNullOrEmpty(errorMessage)) { writer.WriteEncodedText(SR.GetString(SR.ErrorWebPart_ErrorText, errorMessage)); } } #region ITrackingPersonalizable implementation // It doesn't really matter what we return from this property, since this codepath will // never be reached for the ErrorWebPart. However, we return true since we will never need // the framework to diff our properties. bool ITrackingPersonalizable.TracksChanges { get { return true; } } void ITrackingPersonalizable.BeginLoad() { } void ITrackingPersonalizable.BeginSave() { } void ITrackingPersonalizable.EndLoad() { EndLoadPersonalization(); } void ITrackingPersonalizable.EndSave() { } #endregion } }
Link Menu
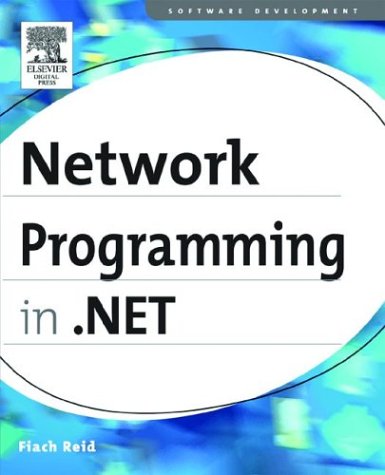
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QilPatternVisitor.cs
- AmbientLight.cs
- DefaultPrintController.cs
- BitmapImage.cs
- GridViewDeleteEventArgs.cs
- Unit.cs
- UndoUnit.cs
- MarginsConverter.cs
- HostedTcpTransportManager.cs
- XmlIlTypeHelper.cs
- ProcessStartInfo.cs
- LinqDataSourceStatusEventArgs.cs
- ExtendedPropertyDescriptor.cs
- WindowsClaimSet.cs
- TextEmbeddedObject.cs
- RegexCompiler.cs
- Deserializer.cs
- CollectionChange.cs
- ImageAnimator.cs
- DataGridPagingPage.cs
- RegexGroupCollection.cs
- Root.cs
- XPathConvert.cs
- Cell.cs
- SamlAuthenticationStatement.cs
- SafeFindHandle.cs
- NumberFunctions.cs
- MailAddress.cs
- EventLogPermission.cs
- ListViewTableRow.cs
- XPathAncestorIterator.cs
- OracleCommand.cs
- ConfigXmlReader.cs
- TextEffect.cs
- SchemaInfo.cs
- ImageListStreamer.cs
- WebDescriptionAttribute.cs
- XamlToRtfParser.cs
- PieceNameHelper.cs
- XPathScanner.cs
- CapabilitiesState.cs
- RemotingHelper.cs
- RayMeshGeometry3DHitTestResult.cs
- ObjectReaderCompiler.cs
- ActivitySurrogateSelector.cs
- ScriptRegistrationManager.cs
- SqlBulkCopy.cs
- SkewTransform.cs
- WindowsListViewItem.cs
- ItemDragEvent.cs
- TextTreeRootNode.cs
- VScrollBar.cs
- Touch.cs
- ListBoxChrome.cs
- RadioButtonBaseAdapter.cs
- EditorBrowsableAttribute.cs
- SymLanguageVendor.cs
- Light.cs
- QuaternionAnimation.cs
- SecurityDescriptor.cs
- CodeDelegateCreateExpression.cs
- DigitalSignature.cs
- TraversalRequest.cs
- DrawingGroup.cs
- EntityViewContainer.cs
- SmiConnection.cs
- SchemaCollectionCompiler.cs
- CodeTypeConstructor.cs
- ExtendedPropertyInfo.cs
- ColumnCollection.cs
- Floater.cs
- CultureSpecificCharacterBufferRange.cs
- DbConvert.cs
- VariableQuery.cs
- DocobjHost.cs
- MetricEntry.cs
- HierarchicalDataBoundControl.cs
- DiscriminatorMap.cs
- SimpleFileLog.cs
- ProfilePropertySettings.cs
- GenericUriParser.cs
- LoginUtil.cs
- DiagnosticStrings.cs
- AttributeCollection.cs
- MsmqHostedTransportConfiguration.cs
- TemplateBindingExpressionConverter.cs
- BamlTreeUpdater.cs
- ScrollProviderWrapper.cs
- EmbeddedMailObjectsCollection.cs
- PropertyChangedEventArgs.cs
- XamlFigureLengthSerializer.cs
- DbCommandDefinition.cs
- OdbcConnectionString.cs
- TogglePatternIdentifiers.cs
- InfoCardCryptoHelper.cs
- ConsoleCancelEventArgs.cs
- DataGridColumnStyleMappingNameEditor.cs
- BooleanConverter.cs
- DeclaredTypeValidatorAttribute.cs
- CheckBoxPopupAdapter.cs