Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / ProjectedSlot.cs / 1305376 / ProjectedSlot.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Mapping.ViewGeneration.Utils; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.Structures { ////// This class represents the constants or members that that can be referenced in a C or S Cell query /// In addition to fields, may represent constants such as types of fields, booleans, etc. /// internal abstract class ProjectedSlot : InternalBase, IEquatable{ #region Fields // A comparer object for comparing slots internal static readonly IEqualityComparer EqualityComparer = new ProjectedSlotComparer(); #endregion #region Abstract methods // effects: Given a slot and the new mapping, returns the // corresponding nw slot internal virtual ProjectedSlot RemapSlot(Dictionary remap) { Debug.Fail("Should not be called."); return null; // To keep the compiler happy } // effects: Returns true if this is semantically equivalent to s2 protected virtual bool IsEqualTo(ProjectedSlot right) { return base.Equals(right); } protected virtual int GetHash() { return base.GetHashCode(); } public bool Equals(ProjectedSlot right) { return EqualityComparer.Equals(this, right); } public override bool Equals(object obj) { ProjectedSlot right = obj as ProjectedSlot; if (obj == null) { return false; } return Equals(right); } public override int GetHashCode() { return EqualityComparer.GetHashCode(this); } // effects: Converts all "relevant" ProjectedSlots in this (including // this) to aliased slots for CqlBlock "block". A slot type is // relevant if it is not a constant. "outputPath" is the output path // of this slot in the final view internal virtual ProjectedSlot MakeAliasedSlot(CqlBlock block, MemberPath outputPath, int slotNum) { AliasedSlot result = new AliasedSlot(block, this, outputPath, slotNum); return result; } // requires: outputMember is non-null if slot is ConstantSlot (it can be // null otherwise). indentLevel indicates the appropriate // indentation level (method can ignore it) // effects: Given a slot and a tableAlias, generates the Cql // corresponding to it. If slot is an Aliased slot, tableAlias is ignored // Returns the modified builder internal abstract StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias, int indentLevel); #endregion #region Helper Methods for slots and ProjectedSlotMap // effects: Returns the alias corresponding to slot. outputMember is // the member to which this slot is ultimately contributing to, e.g., // CPerson1_pid internal virtual string CqlFieldAlias(MemberPath outputMember) { // Subclasses that don't want the outputMember will override this method return outputMember.CqlFieldAlias; } // effects: Given a slot number, slotNum, returns the output member path // that this slot contributes/corresponds to in the extent view. If // the slot corresponds to one of the boolean variables, returns null internal static MemberPath GetMemberPath(int slotNum, MemberProjectionIndex projectedSlotMap, int numBoolSlots) { MemberPath result = IsBoolSlot(slotNum, projectedSlotMap, numBoolSlots) ? null : projectedSlotMap[slotNum]; return result; } // effects: Given the index of a boolean variable (e.g., of from1), // returns the slot number for that boolean in this internal static int BoolIndexToSlot(int boolIndex, MemberProjectionIndex projectedSlotMap, int numBoolSlots) { // Booleans appear after the regular slot Debug.Assert(boolIndex >= 0 && boolIndex < numBoolSlots, "No such boolean in this node"); return projectedSlotMap.Count + boolIndex; } // effects: Given a slotNum corresponding to a boolean slot, returns // the cel number that the cell corresponds to internal static int SlotToBoolIndex(int slotNum, MemberProjectionIndex projectedSlotMap, int numBoolSlots) { Debug.Assert(slotNum < projectedSlotMap.Count + numBoolSlots && slotNum >= projectedSlotMap.Count, "No such boolean slot"); return slotNum - projectedSlotMap.Count; } // effects: Returns true if slotNum corresponds to a key slot in the // output extent view internal static bool IsKeySlot(int slotNum, MemberProjectionIndex projectedSlotMap, int numBoolSlots) { Debug.Assert(slotNum < projectedSlotMap.Count + numBoolSlots, "No such slot in tree"); return slotNum < projectedSlotMap.Count && projectedSlotMap[slotNum].IsPartOfKey; } // effects: Returns true if slotNum corresponds to a bool slot and // not a regular field internal static bool IsBoolSlot(int slotNum, MemberProjectionIndex projectedSlotMap, int numBoolSlots) { Debug.Assert(slotNum < projectedSlotMap.Count + numBoolSlots, "Boolean slot does not exist in tree"); return slotNum >= projectedSlotMap.Count; } #endregion #region Other Methods // effects: Given fields slots1 and slot2, remap then using "remap" and merge them internal static bool TryMergeRemapSlots(ProjectedSlot[] slots1, ProjectedSlot[] slots2, out ProjectedSlot[] result) { // First merge them and then remp them ProjectedSlot[] mergedSlots; if (!TryMergeSlots(slots1, slots2, out mergedSlots)) { result = null; return false; } result = mergedSlots; return true; } // effects: Given two lists of slots1 and slot2, merges them and returns // the resulting slots, i.e., empty slots from one are overridden by // the slots from the other private static bool TryMergeSlots(ProjectedSlot[] slots1, ProjectedSlot[] slots2, out ProjectedSlot[] slots) { Debug.Assert(slots1.Length == slots2.Length, "Merged slots of two cells must be same size"); slots = new ProjectedSlot[slots1.Length]; for (int i = 0; i < slots.Length; i++) { ProjectedSlot slot1 = slots1[i]; ProjectedSlot slot2 = slots2[i]; if (slot1 == null) { slots[i] = slot2; } else if (slot2 == null) { slots[i] = slot1; } else { // Both slots are non-null: Either both are the same // members or one of them is a constant // Note: if both are constants (even different constants) // it does not matter which one we pick because the CASE statement will override it MemberProjectedSlot memberSlot1 = slot1 as MemberProjectedSlot; MemberProjectedSlot memberSlot2 = slot2 as MemberProjectedSlot; if (memberSlot1 != null && memberSlot2 != null && false == EqualityComparer.Equals(memberSlot1, memberSlot2)) { // Illegal combination of slots; non-constant fields disagree return false; } // If one of them is a field we have to get the field ProjectedSlot pickedSlot = (memberSlot1 != null) ? slot1 : slot2; slots[i] = pickedSlot; } } return true; } #endregion #region Comparer class // summary: A class that can compare slots basd on their contents internal class ProjectedSlotComparer : IEqualityComparer { // effects: Returns true if left and right are semantically equivalent public bool Equals(ProjectedSlot left, ProjectedSlot right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least. So if the other one is // null, we cannot be equal if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsEqualTo(right); } public int GetHashCode(ProjectedSlot key) { EntityUtil.CheckArgumentNull(key, "key"); return key.GetHash(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
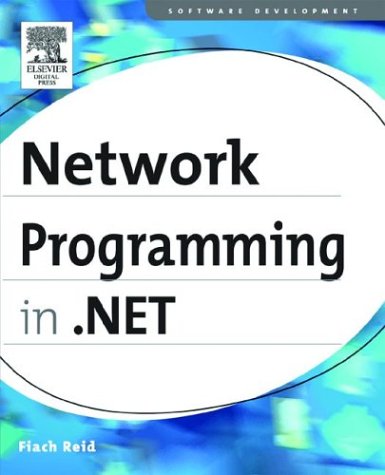
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharacterBufferReference.cs
- ConfigurationLockCollection.cs
- dataprotectionpermissionattribute.cs
- ProofTokenCryptoHandle.cs
- XsdDateTime.cs
- FastPropertyAccessor.cs
- HttpServerVarsCollection.cs
- DocumentGridPage.cs
- BitmapEffectGeneralTransform.cs
- SqlCaseSimplifier.cs
- SimplePropertyEntry.cs
- SocketAddress.cs
- ProxyGenerator.cs
- BrowserDefinitionCollection.cs
- TransformPattern.cs
- AppearanceEditorPart.cs
- UnknownBitmapEncoder.cs
- XmlSchemaSimpleType.cs
- Panel.cs
- Decoder.cs
- UIElement3DAutomationPeer.cs
- EnumBuilder.cs
- ViewGenResults.cs
- graph.cs
- WMIInterop.cs
- XpsPackagingPolicy.cs
- ExpressionBindingCollection.cs
- ToolStripGrip.cs
- CharAnimationUsingKeyFrames.cs
- DragEvent.cs
- User.cs
- EntityCommand.cs
- HealthMonitoringSection.cs
- InvokePatternIdentifiers.cs
- CompiledRegexRunnerFactory.cs
- WebBrowserNavigatingEventHandler.cs
- EdmFunctions.cs
- ZipPackage.cs
- UnsignedPublishLicense.cs
- Mappings.cs
- Pointer.cs
- SamlEvidence.cs
- VisualState.cs
- dataprotectionpermission.cs
- OpCodes.cs
- TextEffectCollection.cs
- IpcServerChannel.cs
- Environment.cs
- QueryAsyncResult.cs
- RowToParametersTransformer.cs
- PointAnimationUsingKeyFrames.cs
- ServiceNotStartedException.cs
- GridViewHeaderRowPresenter.cs
- ObjectManager.cs
- MessageVersion.cs
- DesignerContextDescriptor.cs
- ResourcePool.cs
- PasswordRecovery.cs
- SchemaNames.cs
- ProgramPublisher.cs
- PropertyTab.cs
- WebPartEditorCancelVerb.cs
- XmlQueryStaticData.cs
- RelationshipEndCollection.cs
- Animatable.cs
- CodeDelegateCreateExpression.cs
- COSERVERINFO.cs
- bidPrivateBase.cs
- UnsafeNativeMethodsMilCoreApi.cs
- CodeDomConfigurationHandler.cs
- GlyphRunDrawing.cs
- ValueSerializerAttribute.cs
- RefType.cs
- EdmFunctions.cs
- ResizeGrip.cs
- PropertyMetadata.cs
- TreeChangeInfo.cs
- ContentFilePart.cs
- ServiceControllerDesigner.cs
- oledbmetadatacollectionnames.cs
- ToolboxItemCollection.cs
- RegionData.cs
- FormViewPagerRow.cs
- Composition.cs
- CryptoStream.cs
- MD5.cs
- oledbconnectionstring.cs
- WebPartTracker.cs
- ButtonBaseAutomationPeer.cs
- TableCellAutomationPeer.cs
- TextProperties.cs
- CultureMapper.cs
- CacheAxisQuery.cs
- WindowInteractionStateTracker.cs
- XmlAttributes.cs
- SplayTreeNode.cs
- PageParser.cs
- DocumentSignatureManager.cs
- Point.cs
- CommandHelper.cs