Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / DelegateArgument.cs / 1305376 / DelegateArgument.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Activities.Runtime; using System.Activities.Validation; using System.Collections.Generic; using System.ComponentModel; using System.Runtime; public abstract class DelegateArgument : LocationReference { ArgumentDirection direction; RuntimeDelegateArgument runtimeArgument; string name; int cacheId; internal DelegateArgument() { this.Id = -1; } [DefaultValue(null)] public new string Name { get { return this.name; } set { this.name = value; } } protected override string NameCore { get { return this.name; } } public ArgumentDirection Direction { get { return this.direction; } internal set { this.direction = value; } } internal Activity Owner { get; private set; } internal bool IsInTree { get { return this.Owner != null; } } internal void ThrowIfNotInTree() { if (!this.IsInTree) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.DelegateArgumentMustBeReferenced(this.Name))); } } internal void Bind(RuntimeDelegateArgument runtimeArgument) { this.runtimeArgument = runtimeArgument; } internal bool InitializeRelationship(Activity parent, ref IListvalidationErrors) { if (this.cacheId == parent.CacheId) { Fx.Assert(this.Owner != null, "must have an owner here"); ValidationError validationError = new ValidationError(SR.DelegateArgumentAlreadyInUseOnActivity(this.Name, parent.DisplayName, this.Owner.DisplayName), this.Owner); ActivityUtilities.Add(ref validationErrors, validationError); // Get out early since we've already initialized this argument. return false; } this.Owner = parent; this.cacheId = parent.CacheId; return true; } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. public object Get(ActivityContext context) { if (context == null) { throw FxTrace.Exception.ArgumentNull("context"); } return context.GetValue
Link Menu
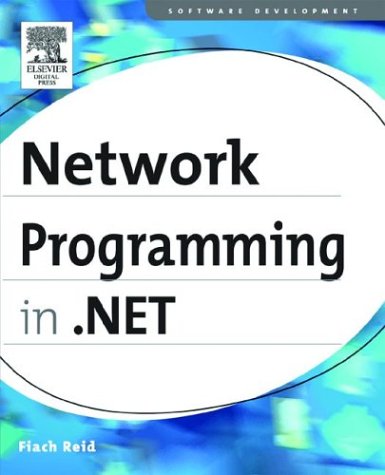
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParamArrayAttribute.cs
- UnsafeCollabNativeMethods.cs
- GPPOINT.cs
- Group.cs
- TextSegment.cs
- TraceLevelStore.cs
- SmtpNegotiateAuthenticationModule.cs
- TextDecorations.cs
- Thickness.cs
- DateTimeOffsetConverter.cs
- SimpleRecyclingCache.cs
- ImageUrlEditor.cs
- LogLogRecord.cs
- BackoffTimeoutHelper.cs
- DataGridRelationshipRow.cs
- HostDesigntimeLicenseContext.cs
- TextServicesManager.cs
- UpdateTracker.cs
- SelectionChangedEventArgs.cs
- SqlConnectionManager.cs
- SafeRightsManagementPubHandle.cs
- TCPClient.cs
- BaseHashHelper.cs
- Attributes.cs
- CallSiteOps.cs
- SchemaTypeEmitter.cs
- PageAsyncTaskManager.cs
- RectConverter.cs
- FixedPage.cs
- ClockGroup.cs
- AdRotator.cs
- RunInstallerAttribute.cs
- XmlSchemaSimpleContentRestriction.cs
- NativeWindow.cs
- SystemFonts.cs
- WaitHandleCannotBeOpenedException.cs
- InkCanvas.cs
- dataprotectionpermission.cs
- ErasingStroke.cs
- UrlPropertyAttribute.cs
- TreeIterator.cs
- BrowserCapabilitiesFactory35.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- MenuItemStyle.cs
- GridViewRowEventArgs.cs
- SoundPlayerAction.cs
- Transform3D.cs
- ProtocolsConfiguration.cs
- XmlMapping.cs
- XhtmlBasicListAdapter.cs
- ClientBuildManagerCallback.cs
- WindowsGraphicsCacheManager.cs
- RootBuilder.cs
- DispatcherHooks.cs
- InvalidAsynchronousStateException.cs
- InstanceKey.cs
- SQLDouble.cs
- HebrewCalendar.cs
- EnumBuilder.cs
- TemplateBaseAction.cs
- PackageDigitalSignature.cs
- RegexCompiler.cs
- MachineKeySection.cs
- WebScriptMetadataMessage.cs
- x509store.cs
- ResolvedKeyFrameEntry.cs
- ToolStripLabel.cs
- XmlQueryOutput.cs
- Parameter.cs
- TdsValueSetter.cs
- LinkDesigner.cs
- Select.cs
- SatelliteContractVersionAttribute.cs
- DataSourceHelper.cs
- GC.cs
- XPathExpr.cs
- SecurityUtils.cs
- FreezableDefaultValueFactory.cs
- ObjectSerializerFactory.cs
- CompiledQuery.cs
- RelatedCurrencyManager.cs
- ClientRequest.cs
- X509ClientCertificateAuthentication.cs
- ExpressionVisitor.cs
- NativeMethods.cs
- DataList.cs
- PropertyPushdownHelper.cs
- FormsAuthentication.cs
- SoapAttributes.cs
- Timer.cs
- EventPropertyMap.cs
- ToolStripItemEventArgs.cs
- InfiniteTimeSpanConverter.cs
- BuildDependencySet.cs
- CodeVariableDeclarationStatement.cs
- WebSysDescriptionAttribute.cs
- ControlCachePolicy.cs
- PropertyCollection.cs
- ConfigurationStrings.cs
- SslStream.cs