Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / Literal.cs / 1305376 / Literal.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Expressions { using System; using System.Activities.ExpressionParser; using System.Activities.XamlIntegration; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Linq.Expressions; using System.Runtime; using System.Text.RegularExpressions; using System.Windows.Markup; [ContentProperty("Value")] public sealed class Literal: CodeActivity , IExpressionContainer, IValueSerializableExpression { static Regex ExpressionEscapeRegex = new Regex(@"^(%*\[)"); public Literal() { } public Literal(T value) : this() { this.Value = value; } public T Value { get; set; } Expression IExpressionContainer.Expression { get { return Expression.Lambda >(Expression.Constant(this.Value, typeof(T)), ExpressionUtilities.RuntimeContextParameter); } } protected override void CacheMetadata(CodeActivityMetadata metadata) { Type literalType = typeof(T); if (!literalType.IsValueType && literalType != TypeHelper.StringType) { metadata.AddValidationError(SR.LiteralsMustBeValueTypesOrImmutableTypes(TypeHelper.StringType, literalType)); } } protected override T Execute(CodeActivityContext context) { return ExecuteWithTryGetValue(context); } internal override bool TryGetValue(ActivityContext context, out T value) { value = this.Value; return true; } public override string ToString() { return this.Value == null ? "null" : this.Value.ToString(); } public bool CanConvertToString(IValueSerializerContext context) { Type typeArgument; Type valueType; TypeConverter converter; if (this.Value == null) { return true; } typeArgument = typeof(T); valueType = this.Value.GetType(); if (valueType == TypeHelper.StringType) { string myValue = this.Value as string; if (string.IsNullOrEmpty(myValue)) { return false; } } converter = TypeDescriptor.GetConverter(typeArgument); if (typeArgument == valueType && converter != null && converter.CanConvertTo(TypeHelper.StringType) && converter.CanConvertFrom(TypeHelper.StringType)) { return true; } return false; } [SuppressMessage(FxCop.Category.Globalization, FxCop.Rule.SpecifyIFormatProvider, Justification = "we really do want the string as-is")] public string ConvertToString(IValueSerializerContext context) { Type typeArgument; Type valueType; TypeConverter converter; if (this.Value == null) { return "[Nothing]"; } typeArgument = typeof(T); valueType = this.Value.GetType(); converter = TypeDescriptor.GetConverter(typeArgument); Fx.Assert(typeArgument == valueType && converter != null && converter.CanConvertTo(TypeHelper.StringType) && converter.CanConvertFrom(TypeHelper.StringType), "Literal target type T and the return type mismatch or something wrong with its typeConverter!"); // handle a Literal of "[...]" by inserting escape chararcter '%' at the front if (typeArgument == TypeHelper.StringType) { string originalString = Convert.ToString(this.Value); if (originalString.EndsWith("]", StringComparison.Ordinal) && ExpressionEscapeRegex.IsMatch(originalString)) { return "%" + originalString; } } return converter.ConvertToString(context, this.Value); } [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeValue() { return !object.Equals(this.Value, default(T)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
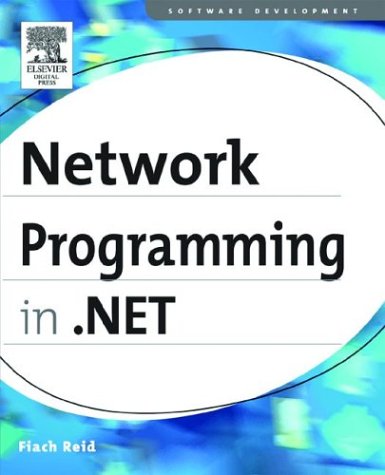
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthStoreRoleProvider.cs
- storepermissionattribute.cs
- ListViewHitTestInfo.cs
- ToolTipAutomationPeer.cs
- MsmqHostedTransportConfiguration.cs
- SmtpNtlmAuthenticationModule.cs
- BulletedListEventArgs.cs
- DLinqDataModelProvider.cs
- ContentPlaceHolder.cs
- documentsequencetextcontainer.cs
- EUCJPEncoding.cs
- X500Name.cs
- CharacterBuffer.cs
- _ListenerResponseStream.cs
- ReadOnlyHierarchicalDataSource.cs
- HMACSHA256.cs
- StatusBarAutomationPeer.cs
- XmlSchemaAttributeGroupRef.cs
- UseLicense.cs
- Stopwatch.cs
- FilterQueryOptionExpression.cs
- BitmapEffectRenderDataResource.cs
- HttpHandlerActionCollection.cs
- StylusPointDescription.cs
- HtmlTextArea.cs
- PropertyCondition.cs
- PerformanceCounterLib.cs
- AsymmetricKeyExchangeFormatter.cs
- Graphics.cs
- RangeValuePatternIdentifiers.cs
- TextBoxView.cs
- SqlCommandBuilder.cs
- NetDataContractSerializer.cs
- WebPartDeleteVerb.cs
- _emptywebproxy.cs
- dtdvalidator.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- Translator.cs
- SponsorHelper.cs
- ServiceSecurityAuditElement.cs
- IntPtr.cs
- PersistencePipeline.cs
- EntityCodeGenerator.cs
- SharedStream.cs
- MimeReturn.cs
- ButtonField.cs
- Ref.cs
- SerialErrors.cs
- ReferentialConstraint.cs
- Helper.cs
- RootBrowserWindow.cs
- LinkLabel.cs
- Parameter.cs
- SafeNativeMethods.cs
- TextChangedEventArgs.cs
- TargetConverter.cs
- QilXmlReader.cs
- FunctionUpdateCommand.cs
- Decoder.cs
- XDRSchema.cs
- ConfigurationElement.cs
- HostProtectionException.cs
- TextFindEngine.cs
- HtmlImage.cs
- RPIdentityRequirement.cs
- XmlElementList.cs
- CompilerInfo.cs
- ComboBoxHelper.cs
- WebHttpSecurityElement.cs
- ContextProperty.cs
- TrimSurroundingWhitespaceAttribute.cs
- OleAutBinder.cs
- OSFeature.cs
- HttpBrowserCapabilitiesBase.cs
- BinaryCommonClasses.cs
- BrushValueSerializer.cs
- TaskbarItemInfo.cs
- TreeViewImageKeyConverter.cs
- ConnectionInterfaceCollection.cs
- EditorZoneBase.cs
- UshortList2.cs
- HandledMouseEvent.cs
- DiscoveryClientReferences.cs
- ExpandCollapseProviderWrapper.cs
- MetaModel.cs
- IFlowDocumentViewer.cs
- EUCJPEncoding.cs
- EarlyBoundInfo.cs
- SqlProfileProvider.cs
- DocComment.cs
- MenuItemBinding.cs
- QuaternionAnimationBase.cs
- TextStore.cs
- DoubleStorage.cs
- SoapReflectionImporter.cs
- IODescriptionAttribute.cs
- DiffuseMaterial.cs
- ToolStrip.cs
- DataGridColumnReorderingEventArgs.cs
- ClientFormsAuthenticationMembershipProvider.cs