Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Statements / Rethrow.cs / 1305376 / Rethrow.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Statements { using System.Activities.Runtime; using System.Activities.Validation; using System.Collections.Generic; using System.ComponentModel; public sealed class Rethrow : NativeActivity { public Rethrow() { DelegateInArgumentelement = new DelegateInArgument () { Name = "constraintArg" }; DelegateInArgument validationContext = new DelegateInArgument () { Name = "validationContext" }; base.Constraints.Add(new Constraint { Body = new ActivityAction { Argument1 = element, Argument2 = validationContext, Handler = new RethrowBuildConstraint { ParentChain = new GetParentChain { ValidationContext = validationContext, }, RethrowActivity = element }, } }); } protected override void CacheMetadata(NativeActivityMetadata metadata) { } protected override void Execute(NativeActivityContext context) { FaultContext faultContext = context.Properties.Find(TryCatch.FaultContextId) as FaultContext; if (faultContext == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.FaultContextNotFound(this.DisplayName))); } context.RethrowException(faultContext); } class RethrowBuildConstraint : NativeActivity { [RequiredArgument] [DefaultValue(null)] public InArgument > ParentChain { get; set; } [RequiredArgument] [DefaultValue(null)] public InArgument RethrowActivity { get; set; } protected override void CacheMetadata(NativeActivityMetadata metadata) { RuntimeArgument parentChainArgument = new RuntimeArgument("ParentChain", typeof(IEnumerable ), ArgumentDirection.In, true); metadata.Bind(this.ParentChain, parentChainArgument); metadata.AddArgument(parentChainArgument); RuntimeArgument rethrowActivityArgument = new RuntimeArgument("RethrowActivity", typeof(Rethrow), ArgumentDirection.In, true); metadata.Bind(this.RethrowActivity, rethrowActivityArgument); metadata.AddArgument(rethrowActivityArgument); } protected override void Execute(NativeActivityContext context) { IEnumerable parentChain = this.ParentChain.Get(context); Rethrow rethrowActivity = this.RethrowActivity.Get(context); Activity previousActivity = rethrowActivity; bool privateRethrow = false; // TryCatch with Rethrow is usually authored in the following way: // // TryCatch // { // Try = DoWork // Catch Handler = Sequence // { // ProcessException, // Rethrow // } // } // Notice that the chain of Activities is TryCatch->Sequence->Rethrow // We want to validate that Rethrow is in the catch block of TryCatch // We walk up the parent chain until we find TryCatch. Then we check if one the catch handlers points to Sequence(the previous activity in the tree) foreach (Activity parent in parentChain) { // Rethrow is only allowed under the public children of a TryCatch activity. // If any of the activities in the tree is a private child, report a constraint violation. if (parent.ImplementationChildren.Contains(previousActivity)) { privateRethrow = true; } TryCatch tryCatch = parent as TryCatch; if(tryCatch != null) { if (previousActivity != null) { foreach (Catch catchHandler in tryCatch.Catches) { ActivityDelegate catchAction = catchHandler.GetAction(); if (catchAction != null && catchAction.Handler == previousActivity) { if (privateRethrow) { Constraint.AddValidationError(context, new ValidationError(SR.RethrowMustBeAPublicChild(rethrowActivity.DisplayName), rethrowActivity)); } return; } } } } previousActivity = parent; } Constraint.AddValidationError(context, new ValidationError(SR.RethrowNotInATryCatch(rethrowActivity.DisplayName), rethrowActivity)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
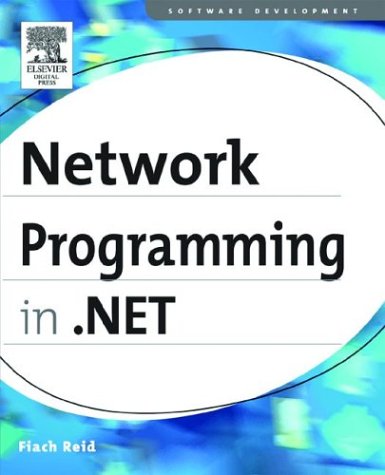
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceAssociationTypeEnd.cs
- WebInvokeAttribute.cs
- Pen.cs
- SwitchElementsCollection.cs
- NativeActivityMetadata.cs
- BuildDependencySet.cs
- BuilderPropertyEntry.cs
- XmlSchemaDatatype.cs
- AnnotationResourceChangedEventArgs.cs
- ExpressionLexer.cs
- PhysicalAddress.cs
- Floater.cs
- LinqDataSourceDisposeEventArgs.cs
- DeploymentSection.cs
- SoapIncludeAttribute.cs
- Button.cs
- PermissionSetEnumerator.cs
- DataQuery.cs
- StyleXamlTreeBuilder.cs
- HyperLinkColumn.cs
- Suspend.cs
- FlowDocumentReaderAutomationPeer.cs
- TreeView.cs
- ClientBuildManagerCallback.cs
- WebPartDeleteVerb.cs
- InvalidCastException.cs
- FontInfo.cs
- MsmqActivation.cs
- FunctionDetailsReader.cs
- QueryOutputWriter.cs
- ListComponentEditor.cs
- ConnectionInterfaceCollection.cs
- SimpleType.cs
- PatternMatchRules.cs
- CompositeKey.cs
- InstanceKey.cs
- Border.cs
- DictionaryContent.cs
- RuntimeConfigurationRecord.cs
- ConditionCollection.cs
- FieldReference.cs
- WsdlImporterElement.cs
- HttpProcessUtility.cs
- EncodingDataItem.cs
- ButtonStandardAdapter.cs
- XmlSchemaObject.cs
- DbXmlEnabledProviderManifest.cs
- WebZone.cs
- SqlDataSourceStatusEventArgs.cs
- ColumnResizeUndoUnit.cs
- Vector3DKeyFrameCollection.cs
- HotSpotCollection.cs
- DuplicateDetector.cs
- EventWaitHandle.cs
- SortedList.cs
- ListMarkerSourceInfo.cs
- TextSpan.cs
- LayoutEngine.cs
- HttpListenerResponse.cs
- DataGridViewRowCancelEventArgs.cs
- TemplatedMailWebEventProvider.cs
- TreeViewBindingsEditor.cs
- PropertyTabChangedEvent.cs
- Encoder.cs
- DataListItemEventArgs.cs
- ImageKeyConverter.cs
- WindowsPen.cs
- AddInController.cs
- ResourceDisplayNameAttribute.cs
- ModelPerspective.cs
- SqlTrackingWorkflowInstance.cs
- EntityDataSourceChangingEventArgs.cs
- SessionStateItemCollection.cs
- GridItemCollection.cs
- HtmlControlDesigner.cs
- MessageQueue.cs
- BuildProviderInstallComponent.cs
- DbConnectionPoolGroupProviderInfo.cs
- PageThemeBuildProvider.cs
- Style.cs
- OpenFileDialog.cs
- ContractSearchPattern.cs
- DurableTimerExtension.cs
- LinkedResourceCollection.cs
- COM2ExtendedTypeConverter.cs
- MethodExpr.cs
- ReferenceConverter.cs
- OutputCacheProviderCollection.cs
- SymbolPair.cs
- SimpleColumnProvider.cs
- TextEditorTyping.cs
- BitConverter.cs
- RepeatBehaviorConverter.cs
- XsltLoader.cs
- SpeakInfo.cs
- ToolStripDesignerUtils.cs
- ValidationService.cs
- FilterableAttribute.cs
- Rfc2898DeriveBytes.cs
- FileChangeNotifier.cs