Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / SpeakInfo.cs / 1 / SpeakInfo.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Each Speak calls is stored in a Speak info object. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Globalization; using System.Speech.Synthesis; using System.Speech.Synthesis.TtsEngine; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56524 // The _voiceSynthesis member is not created in this module and should not be disposed namespace System.Speech.Internal.Synthesis { internal sealed class SpeakInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// Voice synthesizer used /// Default engine to use internal SpeakInfo (VoiceSynthesis voiceSynthesis, TTSVoice ttsVoice) { _voiceSynthesis = voiceSynthesis; _ttsVoice = ttsVoice; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal TTSVoice Voice { get { return _ttsVoice; } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods internal void SetVoice (string name, CultureInfo culture, VoiceGender gender, VoiceAge age, int variant) { TTSVoice ttsVoice = _voiceSynthesis.GetEngine (name, culture, gender, age, variant, false); if (!ttsVoice.Equals (_ttsVoice)) { _ttsVoice = ttsVoice; _fNotInTextSeg = true; } } internal void AddAudio (AudioData audio) { AddNewSeg (null, audio); _fNotInTextSeg = true; } internal void AddText (TTSVoice ttsVoice, TextFragment textFragment) { if (_fNotInTextSeg || ttsVoice != _ttsVoice) { AddNewSeg (ttsVoice, null); _fNotInTextSeg = false; } _lastSeg.AddFrag (textFragment); } #if SPEECHSERVER || PROMPT_ENGINE internal void AddPexml (TTSVoice ttsVoice, TextFragment textFragment) { AddText (ttsVoice, textFragment); _lastSeg.ContainsPrompEngineFragment = true; } #endif internal SpeechSeg RemoveFirst () { SpeechSeg speechSeg = null; if (_listSeg.Count > 0) { speechSeg = _listSeg [0]; _listSeg.RemoveAt (0); } return speechSeg; } #endregion //******************************************************************* // // Private Method // //******************************************************************** #region Private Method private void AddNewSeg (TTSVoice pCurrVoice, AudioData audio) { SpeechSeg pNew = new SpeechSeg (pCurrVoice, audio); _listSeg.Add (pNew); _lastSeg = pNew; } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields // default TTS voice private TTSVoice _ttsVoice; // If true then a new segment is required for the next Add Text private bool _fNotInTextSeg = true; // list of segments (text or audio) private List_listSeg = new List (); // current segment private SpeechSeg _lastSeg; // Reference to the VoiceSynthesizer that created it private VoiceSynthesis _voiceSynthesis; #endregion } //******************************************************************* // // Private Types // //******************************************************************** #region Private Types class AudioData : IDisposable { internal AudioData (Uri uri, ResourceLoader resourceLoader) { _uri = uri; _resourceLoader = resourceLoader; Uri baseAudio; _stream = _resourceLoader.LoadFile (uri, out _mimeType, out baseAudio, out _localFile); } /// /// Needed by IEnumerable!!! /// public void Dispose () { Dispose (true); GC.SuppressFinalize (this); } ~AudioData () { Dispose (false); } internal Uri _uri; internal string _mimeType; internal Stream _stream; protected virtual void Dispose (bool disposing) { if (disposing) { // unload the file from the cache if (_localFile != null) { _resourceLoader.UnloadFile (_localFile); } if (_stream != null) { _stream.Dispose (); _stream = null; _localFile = null; _uri = null; } } } private string _localFile; private ResourceLoader _resourceLoader; } enum VOICEACTIONS { VA_NONE, VA_SPEAK, VA_LOAD_DB, VA_UNLOAD_DB, VA_SET_BACKUP } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Each Speak calls is stored in a Speak info object. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Globalization; using System.Speech.Synthesis; using System.Speech.Synthesis.TtsEngine; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56524 // The _voiceSynthesis member is not created in this module and should not be disposed namespace System.Speech.Internal.Synthesis { internal sealed class SpeakInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// Voice synthesizer used /// Default engine to use internal SpeakInfo (VoiceSynthesis voiceSynthesis, TTSVoice ttsVoice) { _voiceSynthesis = voiceSynthesis; _ttsVoice = ttsVoice; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal TTSVoice Voice { get { return _ttsVoice; } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods internal void SetVoice (string name, CultureInfo culture, VoiceGender gender, VoiceAge age, int variant) { TTSVoice ttsVoice = _voiceSynthesis.GetEngine (name, culture, gender, age, variant, false); if (!ttsVoice.Equals (_ttsVoice)) { _ttsVoice = ttsVoice; _fNotInTextSeg = true; } } internal void AddAudio (AudioData audio) { AddNewSeg (null, audio); _fNotInTextSeg = true; } internal void AddText (TTSVoice ttsVoice, TextFragment textFragment) { if (_fNotInTextSeg || ttsVoice != _ttsVoice) { AddNewSeg (ttsVoice, null); _fNotInTextSeg = false; } _lastSeg.AddFrag (textFragment); } #if SPEECHSERVER || PROMPT_ENGINE internal void AddPexml (TTSVoice ttsVoice, TextFragment textFragment) { AddText (ttsVoice, textFragment); _lastSeg.ContainsPrompEngineFragment = true; } #endif internal SpeechSeg RemoveFirst () { SpeechSeg speechSeg = null; if (_listSeg.Count > 0) { speechSeg = _listSeg [0]; _listSeg.RemoveAt (0); } return speechSeg; } #endregion //******************************************************************* // // Private Method // //******************************************************************** #region Private Method private void AddNewSeg (TTSVoice pCurrVoice, AudioData audio) { SpeechSeg pNew = new SpeechSeg (pCurrVoice, audio); _listSeg.Add (pNew); _lastSeg = pNew; } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields // default TTS voice private TTSVoice _ttsVoice; // If true then a new segment is required for the next Add Text private bool _fNotInTextSeg = true; // list of segments (text or audio) private List_listSeg = new List (); // current segment private SpeechSeg _lastSeg; // Reference to the VoiceSynthesizer that created it private VoiceSynthesis _voiceSynthesis; #endregion } //******************************************************************* // // Private Types // //******************************************************************** #region Private Types class AudioData : IDisposable { internal AudioData (Uri uri, ResourceLoader resourceLoader) { _uri = uri; _resourceLoader = resourceLoader; Uri baseAudio; _stream = _resourceLoader.LoadFile (uri, out _mimeType, out baseAudio, out _localFile); } /// /// Needed by IEnumerable!!! /// public void Dispose () { Dispose (true); GC.SuppressFinalize (this); } ~AudioData () { Dispose (false); } internal Uri _uri; internal string _mimeType; internal Stream _stream; protected virtual void Dispose (bool disposing) { if (disposing) { // unload the file from the cache if (_localFile != null) { _resourceLoader.UnloadFile (_localFile); } if (_stream != null) { _stream.Dispose (); _stream = null; _localFile = null; _uri = null; } } } private string _localFile; private ResourceLoader _resourceLoader; } enum VOICEACTIONS { VA_NONE, VA_SPEAK, VA_LOAD_DB, VA_UNLOAD_DB, VA_SET_BACKUP } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
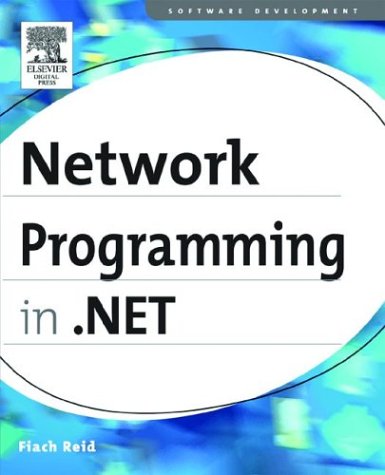
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Context.cs
- OleTxTransaction.cs
- SqlTransaction.cs
- ProtectedUri.cs
- AmbientLight.cs
- CursorConverter.cs
- SizeFConverter.cs
- CancelEventArgs.cs
- TypeNameHelper.cs
- ColumnHeaderConverter.cs
- ServiceBuildProvider.cs
- StdValidatorsAndConverters.cs
- AutoCompleteStringCollection.cs
- Pointer.cs
- TableLayoutStyleCollection.cs
- httpserverutility.cs
- GraphicsState.cs
- SqlConnectionHelper.cs
- GenericParameterDataContract.cs
- QueryCacheManager.cs
- Triplet.cs
- RootDesignerSerializerAttribute.cs
- ConfigurationLockCollection.cs
- AsymmetricSignatureFormatter.cs
- TextTreeInsertElementUndoUnit.cs
- EntityTransaction.cs
- ValidationError.cs
- SocketElement.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- HttpCookieCollection.cs
- Module.cs
- SoapCommonClasses.cs
- XmlExpressionDumper.cs
- FormsAuthenticationConfiguration.cs
- ServiceChannelFactory.cs
- QilInvoke.cs
- ContainerControlDesigner.cs
- Font.cs
- EventLogEntryCollection.cs
- ServiceContractAttribute.cs
- ContainerParaClient.cs
- HtmlControlPersistable.cs
- MruCache.cs
- GenericTypeParameterBuilder.cs
- ImageAutomationPeer.cs
- SingleAnimation.cs
- __ConsoleStream.cs
- securitycriticaldataformultiplegetandset.cs
- SecurityPolicyVersion.cs
- SchemaNotation.cs
- DbConnectionHelper.cs
- ElapsedEventArgs.cs
- Divide.cs
- RuntimeResourceSet.cs
- RegexInterpreter.cs
- IResourceProvider.cs
- BordersPage.cs
- TraceHandler.cs
- SerializationSectionGroup.cs
- As.cs
- Point3DCollectionConverter.cs
- ApplicationGesture.cs
- IPEndPointCollection.cs
- _NTAuthentication.cs
- AttachedAnnotationChangedEventArgs.cs
- DashStyles.cs
- DBDataPermission.cs
- XhtmlStyleClass.cs
- ListSourceHelper.cs
- IgnoreFlushAndCloseStream.cs
- PtsHost.cs
- CoreChannel.cs
- SafeArrayTypeMismatchException.cs
- MessageHeaders.cs
- InvariantComparer.cs
- DataGridAddNewRow.cs
- XmlProcessingInstruction.cs
- StreamSecurityUpgradeInitiatorBase.cs
- MetafileHeader.cs
- DelayedRegex.cs
- StructuredTypeInfo.cs
- BamlRecordWriter.cs
- MemoryRecordBuffer.cs
- TraceFilter.cs
- DataBinding.cs
- ServicePointManager.cs
- BamlTreeMap.cs
- BindingContext.cs
- CustomValidator.cs
- SizeF.cs
- AdornerDecorator.cs
- StringStorage.cs
- SvcMapFile.cs
- TrailingSpaceComparer.cs
- RtfToken.cs
- NullableIntSumAggregationOperator.cs
- XPathNodeList.cs
- CommandValueSerializer.cs
- BuildProviderCollection.cs
- PackageRelationshipCollection.cs