Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Ink / ApplicationGesture.cs / 1 / ApplicationGesture.cs
//---------------------------------------------------------------------------- // // File: ApplicationGesture.cs // // Description: // The definition of ApplicationGesture enum type // // Features: // // History: // 01/14/2005 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; namespace System.Windows.Ink { ////// ApplicationGesture /// public enum ApplicationGesture { ////// AllGestures /// AllGestures = 0, ////// ArrowDown /// ArrowDown = 61497, ////// ArrowLeft /// ArrowLeft = 61498, ////// ArrowRight /// ArrowRight = 61499, ////// ArrowUp /// ArrowUp = 61496, ////// Check /// Check = 61445, ////// ChevronDown /// ChevronDown = 61489, ////// ChevronLeft /// ChevronLeft = 61490, ////// ChevronRight /// ChevronRight = 61491, ////// ChevronUp /// ChevronUp = 61488, ////// Circle /// Circle = 61472, ////// Curlicue /// Curlicue = 61456, ////// DoubleCircle /// DoubleCircle = 61473, ////// DoubleCurlicue /// DoubleCurlicue = 61457, ////// DoubleTap /// DoubleTap = 61681, ////// Down /// Down = 61529, ////// DownLeft /// DownLeft = 61546, ////// DownLeftLong /// DownLeftLong = 61542, ////// DownRight /// DownRight = 61547, ////// DownRightLong /// DownRightLong = 61543, ////// DownUp /// DownUp = 61537, ////// Exclamation /// Exclamation = 61604, ////// Left /// Left = 61530, ////// LeftDown /// LeftDown = 61549, ////// LeftRight /// LeftRight = 61538, ////// LeftUp /// LeftUp = 61548, ////// NoGesture /// NoGesture = 61440, ////// Right /// Right = 61531, ////// RightDown /// RightDown = 61551, ////// RightLeft /// RightLeft = 61539, ////// RightUp /// RightUp = 61550, ////// ScratchOut /// ScratchOut = 61441, ////// SemicircleLeft /// SemicircleLeft = 61480, ////// SemicircleRight /// SemicircleRight = 61481, ////// Square /// Square = 61443, ////// Star /// Star = 61444, ////// Tap /// Tap = 61680, ////// Triangle /// Triangle = 61442, ////// Up /// Up = 61528, ////// UpDown /// UpDown = 61536, ////// UpLeft /// UpLeft = 61544, ////// UpLeftLong /// UpLeftLong = 61540, ////// UpRight /// UpRight = 61545, ////// UpRightLong /// UpRightLong = 61541 } // Whenever the ApplicationGesture is modified, please update this ApplicationGestureHelper.IsDefined. internal static class ApplicationGestureHelper { // the number of enums defined, used by NativeRecognizer // to limit input internal static readonly int CountOfValues = 44; // Helper like Enum.IsDefined, for ApplicationGesture. It is an fxcop violation // to use Enum.IsDefined (for perf reasons) internal static bool IsDefined(ApplicationGesture applicationGesture) { //note that we can't just check the upper and lower bounds since the app gesture //values are not contiguous switch(applicationGesture) { case ApplicationGesture.AllGestures: case ApplicationGesture.ArrowDown: case ApplicationGesture.ArrowLeft: case ApplicationGesture.ArrowRight: case ApplicationGesture.ArrowUp: case ApplicationGesture.Check: case ApplicationGesture.ChevronDown: case ApplicationGesture.ChevronLeft: case ApplicationGesture.ChevronRight: case ApplicationGesture.ChevronUp: case ApplicationGesture.Circle: case ApplicationGesture.Curlicue: case ApplicationGesture.DoubleCircle: case ApplicationGesture.DoubleCurlicue: case ApplicationGesture.DoubleTap: case ApplicationGesture.Down: case ApplicationGesture.DownLeft: case ApplicationGesture.DownLeftLong: case ApplicationGesture.DownRight: case ApplicationGesture.DownRightLong: case ApplicationGesture.DownUp: case ApplicationGesture.Exclamation: case ApplicationGesture.Left: case ApplicationGesture.LeftDown: case ApplicationGesture.LeftRight: case ApplicationGesture.LeftUp: case ApplicationGesture.NoGesture: case ApplicationGesture.Right: case ApplicationGesture.RightDown: case ApplicationGesture.RightLeft: case ApplicationGesture.RightUp: case ApplicationGesture.ScratchOut: case ApplicationGesture.SemicircleLeft: case ApplicationGesture.SemicircleRight: case ApplicationGesture.Square: case ApplicationGesture.Star: case ApplicationGesture.Tap: case ApplicationGesture.Triangle: case ApplicationGesture.Up: case ApplicationGesture.UpDown: case ApplicationGesture.UpLeft: case ApplicationGesture.UpLeftLong: case ApplicationGesture.UpRight: case ApplicationGesture.UpRightLong: { return true; } default: { return false; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ApplicationGesture.cs // // Description: // The definition of ApplicationGesture enum type // // Features: // // History: // 01/14/2005 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; namespace System.Windows.Ink { ////// ApplicationGesture /// public enum ApplicationGesture { ////// AllGestures /// AllGestures = 0, ////// ArrowDown /// ArrowDown = 61497, ////// ArrowLeft /// ArrowLeft = 61498, ////// ArrowRight /// ArrowRight = 61499, ////// ArrowUp /// ArrowUp = 61496, ////// Check /// Check = 61445, ////// ChevronDown /// ChevronDown = 61489, ////// ChevronLeft /// ChevronLeft = 61490, ////// ChevronRight /// ChevronRight = 61491, ////// ChevronUp /// ChevronUp = 61488, ////// Circle /// Circle = 61472, ////// Curlicue /// Curlicue = 61456, ////// DoubleCircle /// DoubleCircle = 61473, ////// DoubleCurlicue /// DoubleCurlicue = 61457, ////// DoubleTap /// DoubleTap = 61681, ////// Down /// Down = 61529, ////// DownLeft /// DownLeft = 61546, ////// DownLeftLong /// DownLeftLong = 61542, ////// DownRight /// DownRight = 61547, ////// DownRightLong /// DownRightLong = 61543, ////// DownUp /// DownUp = 61537, ////// Exclamation /// Exclamation = 61604, ////// Left /// Left = 61530, ////// LeftDown /// LeftDown = 61549, ////// LeftRight /// LeftRight = 61538, ////// LeftUp /// LeftUp = 61548, ////// NoGesture /// NoGesture = 61440, ////// Right /// Right = 61531, ////// RightDown /// RightDown = 61551, ////// RightLeft /// RightLeft = 61539, ////// RightUp /// RightUp = 61550, ////// ScratchOut /// ScratchOut = 61441, ////// SemicircleLeft /// SemicircleLeft = 61480, ////// SemicircleRight /// SemicircleRight = 61481, ////// Square /// Square = 61443, ////// Star /// Star = 61444, ////// Tap /// Tap = 61680, ////// Triangle /// Triangle = 61442, ////// Up /// Up = 61528, ////// UpDown /// UpDown = 61536, ////// UpLeft /// UpLeft = 61544, ////// UpLeftLong /// UpLeftLong = 61540, ////// UpRight /// UpRight = 61545, ////// UpRightLong /// UpRightLong = 61541 } // Whenever the ApplicationGesture is modified, please update this ApplicationGestureHelper.IsDefined. internal static class ApplicationGestureHelper { // the number of enums defined, used by NativeRecognizer // to limit input internal static readonly int CountOfValues = 44; // Helper like Enum.IsDefined, for ApplicationGesture. It is an fxcop violation // to use Enum.IsDefined (for perf reasons) internal static bool IsDefined(ApplicationGesture applicationGesture) { //note that we can't just check the upper and lower bounds since the app gesture //values are not contiguous switch(applicationGesture) { case ApplicationGesture.AllGestures: case ApplicationGesture.ArrowDown: case ApplicationGesture.ArrowLeft: case ApplicationGesture.ArrowRight: case ApplicationGesture.ArrowUp: case ApplicationGesture.Check: case ApplicationGesture.ChevronDown: case ApplicationGesture.ChevronLeft: case ApplicationGesture.ChevronRight: case ApplicationGesture.ChevronUp: case ApplicationGesture.Circle: case ApplicationGesture.Curlicue: case ApplicationGesture.DoubleCircle: case ApplicationGesture.DoubleCurlicue: case ApplicationGesture.DoubleTap: case ApplicationGesture.Down: case ApplicationGesture.DownLeft: case ApplicationGesture.DownLeftLong: case ApplicationGesture.DownRight: case ApplicationGesture.DownRightLong: case ApplicationGesture.DownUp: case ApplicationGesture.Exclamation: case ApplicationGesture.Left: case ApplicationGesture.LeftDown: case ApplicationGesture.LeftRight: case ApplicationGesture.LeftUp: case ApplicationGesture.NoGesture: case ApplicationGesture.Right: case ApplicationGesture.RightDown: case ApplicationGesture.RightLeft: case ApplicationGesture.RightUp: case ApplicationGesture.ScratchOut: case ApplicationGesture.SemicircleLeft: case ApplicationGesture.SemicircleRight: case ApplicationGesture.Square: case ApplicationGesture.Star: case ApplicationGesture.Tap: case ApplicationGesture.Triangle: case ApplicationGesture.Up: case ApplicationGesture.UpDown: case ApplicationGesture.UpLeft: case ApplicationGesture.UpLeftLong: case ApplicationGesture.UpRight: case ApplicationGesture.UpRightLong: { return true; } default: { return false; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
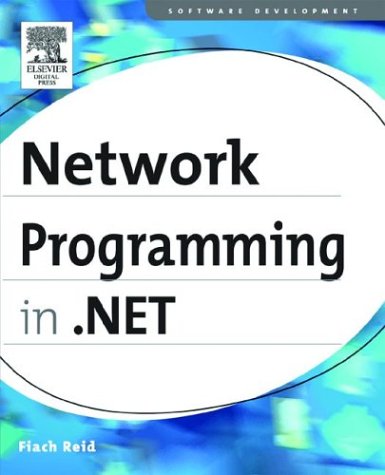
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceManager.cs
- ToolTipService.cs
- ShaderEffect.cs
- DataTableNewRowEvent.cs
- BitmapSource.cs
- CellParagraph.cs
- _LocalDataStoreMgr.cs
- GenericUriParser.cs
- FormatConvertedBitmap.cs
- MetadataSet.cs
- SqlTypesSchemaImporter.cs
- SchemaComplexType.cs
- ObjectToIdCache.cs
- MetroSerializationManager.cs
- ButtonPopupAdapter.cs
- GridProviderWrapper.cs
- IMembershipProvider.cs
- BadImageFormatException.cs
- UIElement3D.cs
- ScrollEventArgs.cs
- validation.cs
- SqlBulkCopy.cs
- MatrixCamera.cs
- Transactions.cs
- BadImageFormatException.cs
- WebPartConnectionsCancelVerb.cs
- HtmlImage.cs
- SettingsBase.cs
- Wizard.cs
- DataServiceRequestArgs.cs
- PixelFormatConverter.cs
- GeneralTransform3DTo2DTo3D.cs
- entityreference_tresulttype.cs
- WindowsGraphicsCacheManager.cs
- FormsAuthenticationConfiguration.cs
- XmlSchemas.cs
- MessageBuffer.cs
- CacheMode.cs
- NetWebProxyFinder.cs
- _SslState.cs
- LifetimeServices.cs
- JsonFormatWriterGenerator.cs
- TableSectionStyle.cs
- InfoCardService.cs
- ResourceAttributes.cs
- InstanceLockedException.cs
- CapiSafeHandles.cs
- BulletChrome.cs
- Pts.cs
- MenuAdapter.cs
- SqlUtil.cs
- XmlSchemaType.cs
- ExpandoObject.cs
- _BasicClient.cs
- GatewayIPAddressInformationCollection.cs
- FormViewDeleteEventArgs.cs
- GenericTextProperties.cs
- Preprocessor.cs
- EndpointDispatcherTable.cs
- _IPv6Address.cs
- ResolveMatches11.cs
- SingleAnimationUsingKeyFrames.cs
- MdiWindowListStrip.cs
- WriteFileContext.cs
- NetworkInformationPermission.cs
- NumericUpDownAcceleration.cs
- BindingNavigator.cs
- SHA1Cng.cs
- Win32SafeHandles.cs
- HttpRequest.cs
- EntityContainerEmitter.cs
- Relationship.cs
- ViewGenerator.cs
- Geometry.cs
- NavigationPropertyEmitter.cs
- XhtmlConformanceSection.cs
- GeneralTransform3D.cs
- TimeManager.cs
- Int16Animation.cs
- StrokeNode.cs
- FixedSchema.cs
- InputLanguageProfileNotifySink.cs
- TextServicesDisplayAttributePropertyRanges.cs
- Line.cs
- DirectionalAction.cs
- MouseDevice.cs
- CodeCastExpression.cs
- RoutingExtension.cs
- StreamAsIStream.cs
- LabelDesigner.cs
- HostingEnvironmentSection.cs
- SqlConnectionFactory.cs
- HtmlTitle.cs
- CollectionBase.cs
- LockRenewalTask.cs
- TimeSpanOrInfiniteConverter.cs
- HttpHandlersSection.cs
- X509Chain.cs
- PropertyFilterAttribute.cs
- InkCanvasInnerCanvas.cs