Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / Description / WorkflowServiceBehavior.cs / 1305376 / WorkflowServiceBehavior.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Activities.Description { using System.Activities; using System.ServiceModel; using System.ServiceModel.Activities.Dispatcher; using System.ServiceModel.Description; using System.ServiceModel.Dispatcher; class WorkflowServiceBehavior : IServiceBehavior { public WorkflowServiceBehavior(Activity activity) { this.Activity = activity; } public Activity Activity { get; private set; } public void AddBindingParameters(ServiceDescription serviceDescription, ServiceHostBase serviceHostBase, System.Collections.ObjectModel.Collectionendpoints, System.ServiceModel.Channels.BindingParameterCollection bindingParameters) { } public void ApplyDispatchBehavior(ServiceDescription serviceDescription, ServiceHostBase serviceHostBase) { if (serviceDescription == null) { throw FxTrace.Exception.ArgumentNull("serviceDescription"); } if (serviceHostBase == null) { throw FxTrace.Exception.ArgumentNull("serviceHostBase"); } DurableInstanceContextProvider instanceContextProvider = new DurableInstanceContextProvider(serviceHostBase); DurableInstanceProvider instanceProvider = new DurableInstanceProvider(serviceHostBase); ServiceDebugBehavior serviceDebugBehavior = serviceDescription.Behaviors.Find (); bool includeExceptionDetailInFaults = serviceDebugBehavior != null ? serviceDebugBehavior.IncludeExceptionDetailInFaults : false; foreach (ChannelDispatcherBase channelDispatcherBase in serviceHostBase.ChannelDispatchers) { ChannelDispatcher channelDispatcher = channelDispatcherBase as ChannelDispatcher; if (channelDispatcher != null) { foreach (EndpointDispatcher endPointDispatcher in channelDispatcher.Endpoints) { if (IsWorkflowEndpoint(endPointDispatcher)) { DispatchRuntime dispatchRuntime = endPointDispatcher.DispatchRuntime; dispatchRuntime.AutomaticInputSessionShutdown = true; dispatchRuntime.ConcurrencyMode = ConcurrencyMode.Multiple; // dispatchRuntime.InstanceContextProvider = instanceContextProvider; dispatchRuntime.InstanceProvider = instanceProvider; if (includeExceptionDetailInFaults) { dispatchRuntime.SetDebugFlagInDispatchOperations(includeExceptionDetailInFaults); } } } } } } public void Validate(ServiceDescription serviceDescription, ServiceHostBase serviceHostBase) { if (serviceDescription == null) { throw FxTrace.Exception.ArgumentNull("serviceDescription"); } if (serviceHostBase == null) { throw FxTrace.Exception.ArgumentNull("serviceHostBase"); } } internal static bool IsWorkflowEndpoint(EndpointDispatcher endpointDispatcher) { if (endpointDispatcher.IsSystemEndpoint) { //Check whether the System Endpoint Opted in for WorkflowDispatch ServiceHostBase serviceHost = endpointDispatcher.ChannelDispatcher.Host; ServiceEndpoint serviceEndpoint = null; foreach (ServiceEndpoint endpointToMatch in serviceHost.Description.Endpoints) { if (endpointToMatch.Id == endpointDispatcher.Id) { serviceEndpoint = endpointToMatch; break; } } if (serviceEndpoint != null) { //User defined Std Endpoint with WorkflowContractBehaviorAttribute. return serviceEndpoint is WorkflowHostingEndpoint || serviceEndpoint.Contract.Behaviors.Contains(typeof(WorkflowContractBehaviorAttribute)); } return false; //Some Einstein scenario where EndpointDispatcher is added explicitly without associated ServiceEndpoint. } return true; //Application Endpoint } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
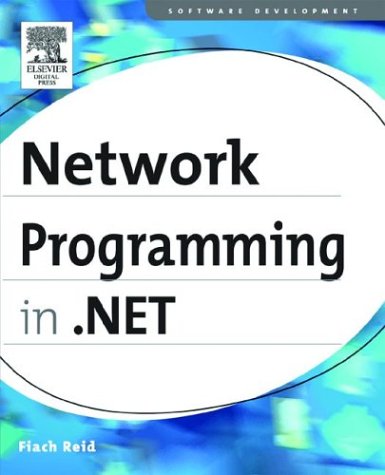
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuntimeTransactionHandle.cs
- DateTimeFormatInfoScanner.cs
- SoapHeaderException.cs
- UpDownEvent.cs
- GenericUriParser.cs
- AncillaryOps.cs
- SoapExtensionReflector.cs
- XmlWriterSettings.cs
- XmlDataLoader.cs
- ContextConfiguration.cs
- OletxCommittableTransaction.cs
- CodeCompileUnit.cs
- Literal.cs
- ContainerFilterService.cs
- WorkItem.cs
- EntityProviderFactory.cs
- DataGridViewIntLinkedList.cs
- SqlCommandAsyncResult.cs
- CalendarTable.cs
- Utilities.cs
- PinnedBufferMemoryStream.cs
- SplashScreenNativeMethods.cs
- FontCacheUtil.cs
- ProcessProtocolHandler.cs
- CroppedBitmap.cs
- CommonEndpointBehaviorElement.cs
- HtmlInputPassword.cs
- DataGridColumnHeader.cs
- TitleStyle.cs
- ClientTargetSection.cs
- DataGridViewAdvancedBorderStyle.cs
- DragEventArgs.cs
- InvariantComparer.cs
- OleDbDataAdapter.cs
- ProxyManager.cs
- XPathNodeList.cs
- KeyValuePair.cs
- FrameSecurityDescriptor.cs
- ZipIOLocalFileHeader.cs
- CollectionConverter.cs
- ExceptionCollection.cs
- SmtpNetworkElement.cs
- BitmapEffectInput.cs
- ListViewItemSelectionChangedEvent.cs
- ISAPIRuntime.cs
- FunctionMappingTranslator.cs
- XPathArrayIterator.cs
- SignatureToken.cs
- ButtonBase.cs
- __Error.cs
- ExpandSegmentCollection.cs
- XPathSingletonIterator.cs
- RegexWriter.cs
- BroadcastEventHelper.cs
- DataGridViewCellValueEventArgs.cs
- SessionIDManager.cs
- DataViewListener.cs
- AliasGenerator.cs
- SecurityTokenSerializer.cs
- TypeRefElement.cs
- Encoding.cs
- wgx_exports.cs
- ZipIOBlockManager.cs
- LocalFileSettingsProvider.cs
- WebServiceErrorEvent.cs
- SQLDoubleStorage.cs
- AxisAngleRotation3D.cs
- PenLineJoinValidation.cs
- DiscoveryExceptionDictionary.cs
- UiaCoreApi.cs
- BlobPersonalizationState.cs
- Splitter.cs
- ParameterToken.cs
- TextDpi.cs
- CodeBinaryOperatorExpression.cs
- CreateUserWizardStep.cs
- WebContext.cs
- returneventsaver.cs
- DisplayInformation.cs
- DocumentEventArgs.cs
- CompModSwitches.cs
- TableLayoutSettingsTypeConverter.cs
- PrePrepareMethodAttribute.cs
- Metafile.cs
- MSAAEventDispatcher.cs
- UnSafeCharBuffer.cs
- Int64.cs
- SafeLocalAllocation.cs
- HtmlSelect.cs
- NameValueSectionHandler.cs
- LocalizedNameDescriptionPair.cs
- ColumnHeaderConverter.cs
- CodeGenerator.cs
- SoapFormatterSinks.cs
- ProtectedProviderSettings.cs
- CompressedStack.cs
- RawStylusInputReport.cs
- PersianCalendar.cs
- ContainerParaClient.cs
- SignatureResourcePool.cs