Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / SendMessageContent.cs / 1305376 / SendMessageContent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activities { using System.Activities; using System.ComponentModel; using System.Runtime; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.Windows.Markup; [ContentProperty("Message")] public sealed class SendMessageContent : SendContent { public SendMessageContent() : base() { } public SendMessageContent(InArgument message) : this() { this.Message = message; } public SendMessageContent(InArgument message, Type declaredMessageType) : this(message) { this.DeclaredMessageType = declaredMessageType; } // The value that is sent as the body of the message. // The type is derived from Message.Expression.ResultType. If the optional // DeclaredMessageType property is also specified, it is validated against // Message.Expression.ResultType. [DefaultValue(null)] public InArgument Message { get; set; } // Allows the type of the variable specified for Message to be a derived type from the type // on the message contract. This type specifies what the type is one the message contract. // If DeclaredMessageType is not specified, the type from the Message InArgument is used. // The DeclaredMessageType must either be the same as the type of the InArgument Message, // or it must be a base type of Message. [DefaultValue(null)] public Type DeclaredMessageType { get; set; } internal Type InternalDeclaredMessageType { get { if (this.DeclaredMessageType == null && this.Message != null) { return this.Message.ArgumentType; } else { return this.DeclaredMessageType; } } } internal override bool IsFault { get { if (this.InternalDeclaredMessageType != null) { return ContractInferenceHelper.ExceptionType.IsAssignableFrom(this.InternalDeclaredMessageType); } else { return false; } } } [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeDeclaredMessageType() { // don't serialize null if (this.DeclaredMessageType == null) { return false; } // if the expression type of Message matches the declared message type, no need to serialize duplicative information if (this.Message != null && this.DeclaredMessageType == this.Message.ArgumentType) { return false; } return true; } internal override void CacheMetadata(ActivityMetadata metadata, Activity owner, string operationName) { MessagingActivityHelper.FixMessageArgument(this.Message, ArgumentDirection.In, metadata); if (this.DeclaredMessageType != null) { if (this.Message == null && this.DeclaredMessageType != TypeHelper.VoidType) { string errorOperationName = ContractValidationHelper.GetErrorMessageOperationName(operationName); metadata.AddValidationError(SR.ValueCannotBeNull(owner.DisplayName, errorOperationName)); } else if (this.Message != null && !this.DeclaredMessageType.IsAssignableFrom(this.Message.ArgumentType)) { string errorOperationName = ContractValidationHelper.GetErrorMessageOperationName(operationName); metadata.AddValidationError(SR.ValueArgumentTypeNotDerivedFromValueType(owner.DisplayName, errorOperationName)); } } } internal override void ConfigureInternalSend(InternalSendMessage internalSendMessage, out ToRequest requestFormatter) { if (this.InternalDeclaredMessageType == MessageDescription.TypeOfUntypedMessage) { // Value is a Message, do not use the formatter but directly pass it to InternalSendMessage internalSendMessage.Message = new InArgument(context => ((InArgument )this.Message).Get(context)); requestFormatter = null; } else { requestFormatter = new ToRequest(); if (this.Message != null) { requestFormatter.Parameters.Add(InArgument.CreateReference(this.Message, "Message")); } } } internal override void ConfigureInternalSendReply(InternalSendMessage internalSendMessage, out ToReply responseFormatter) { if (this.InternalDeclaredMessageType == MessageDescription.TypeOfUntypedMessage) { internalSendMessage.Message = new InArgument (context => ((InArgument )this.Message).Get(context)); responseFormatter = null; } else { responseFormatter = new ToReply(); // WCF rule dictates that MessageContract must be bound to ReturnValue, not Parameters if (MessageBuilder.IsMessageContract(this.InternalDeclaredMessageType)) { responseFormatter.Result = InArgument.CreateReference(this.Message, "Message"); } else if (this.Message != null) { responseFormatter.Parameters.Add(InArgument.CreateReference(this.Message, "Message")); } } } internal override void InferMessageDescription(OperationDescription operation, object owner, MessageDirection direction) { ContractInferenceHelper.CheckForDisposableParameters(operation, this.InternalDeclaredMessageType); string overridingAction = null; SerializerOption serializerOption = SerializerOption.DataContractSerializer; Send send = owner as Send; if (send != null) { overridingAction = send.Action; serializerOption = send.SerializerOption; } else { SendReply sendReply = owner as SendReply; Fx.Assert(sendReply != null, "The owner of SendMessageContent can only be Send or SendReply!"); overridingAction = sendReply.Action; serializerOption = sendReply.Request.SerializerOption; } if (direction == MessageDirection.Input) { ContractInferenceHelper.AddInputMessage(operation, overridingAction, this.InternalDeclaredMessageType, serializerOption); } else { ContractInferenceHelper.AddOutputMessage(operation, overridingAction, this.InternalDeclaredMessageType, serializerOption); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
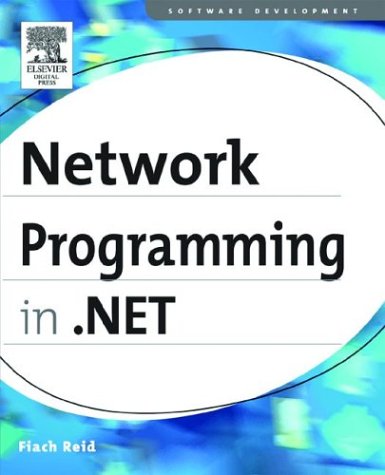
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryObjectWriter.cs
- PackageRelationship.cs
- PropertyRecord.cs
- PointUtil.cs
- WebPartDisplayModeEventArgs.cs
- BitmapImage.cs
- XmlCodeExporter.cs
- ExpressionCopier.cs
- QueryContinueDragEvent.cs
- FilterException.cs
- LinearQuaternionKeyFrame.cs
- ManagementEventWatcher.cs
- ReferencedType.cs
- InputReport.cs
- WebBrowserContainer.cs
- EventArgs.cs
- CodeMethodReturnStatement.cs
- EventProperty.cs
- ApplicationFileParser.cs
- codemethodreferenceexpression.cs
- sqlmetadatafactory.cs
- FloatMinMaxAggregationOperator.cs
- DataGridViewCell.cs
- ReversePositionQuery.cs
- StateMachineDesignerPaint.cs
- WebHttpBindingCollectionElement.cs
- GeneralTransform3DGroup.cs
- SystemSounds.cs
- Highlights.cs
- SafeSystemMetrics.cs
- XamlDesignerSerializationManager.cs
- FunctionDetailsReader.cs
- ActivityDelegate.cs
- XslException.cs
- MessageQueueKey.cs
- SmiEventSink.cs
- ApplicationServiceManager.cs
- EditorPart.cs
- GAC.cs
- BinaryFormatterWriter.cs
- EventLogLink.cs
- HttpCookie.cs
- XmlCharType.cs
- UnitySerializationHolder.cs
- ParseChildrenAsPropertiesAttribute.cs
- CLRBindingWorker.cs
- KeyInfo.cs
- Stroke2.cs
- DocumentCollection.cs
- SamlAttributeStatement.cs
- BitmapVisualManager.cs
- TcpProcessProtocolHandler.cs
- UIInitializationException.cs
- SettingsPropertyWrongTypeException.cs
- WebPartPersonalization.cs
- XPathParser.cs
- BamlLocalizableResourceKey.cs
- SafeRegistryHandle.cs
- PageAsyncTaskManager.cs
- XsltQilFactory.cs
- XmlHierarchyData.cs
- SQLResource.cs
- TreeViewCancelEvent.cs
- DesignTimeXamlWriter.cs
- RectIndependentAnimationStorage.cs
- OleDbEnumerator.cs
- MobileRedirect.cs
- Pkcs7Recipient.cs
- SettingsSection.cs
- OperationDescriptionCollection.cs
- TreeWalker.cs
- ExtendedPropertyCollection.cs
- TdsParserStaticMethods.cs
- ActivityTypeCodeDomSerializer.cs
- NavigationEventArgs.cs
- ThreadExceptionDialog.cs
- ReadingWritingEntityEventArgs.cs
- BufferedGraphicsManager.cs
- CodeValidator.cs
- SchemaLookupTable.cs
- TextElement.cs
- XsltArgumentList.cs
- QilNode.cs
- ObjectConverter.cs
- PersistenceProviderFactory.cs
- InvalidPrinterException.cs
- ProjectedSlot.cs
- ParamArrayAttribute.cs
- FileDialogPermission.cs
- DockPanel.cs
- DataControlFieldHeaderCell.cs
- SQLMembershipProvider.cs
- FormatPage.cs
- OracleRowUpdatingEventArgs.cs
- DetailsViewDeleteEventArgs.cs
- ExpressionBinding.cs
- ArrayConverter.cs
- FormatterConverter.cs
- CustomCategoryAttribute.cs
- GridErrorDlg.cs