Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Discovery / System / ServiceModel / Discovery / ProbeRequestResponseAsyncResult.cs / 1305376 / ProbeRequestResponseAsyncResult.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Discovery { using System.Collections.ObjectModel; using System.Diagnostics.CodeAnalysis; using System.Runtime; abstract class ProbeRequestResponseAsyncResult: AsyncResult { readonly IDiscoveryServiceImplementation discoveryServiceImpl; readonly FindRequestResponseContext findRequest; readonly DiscoveryOperationContext context; static AsyncCompletion onOnFindCompletedCallback = new AsyncCompletion(OnOnFindCompleted); bool isFindCompleted; [Fx.Tag.SynchronizationObject] object findCompletedLock; [SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] protected ProbeRequestResponseAsyncResult( TProbeMessage probeMessage, IDiscoveryServiceImplementation discoveryServiceImpl, AsyncCallback callback, object state) : base(callback, state) { Fx.Assert(probeMessage != null, "The probeMessage must be non null."); Fx.Assert(discoveryServiceImpl != null, "The discoveryServiceImpl must be non null."); this.discoveryServiceImpl = discoveryServiceImpl; this.findCompletedLock = new object(); if (!this.Validate(probeMessage)) { this.Complete(true); return; } else { this.context = new DiscoveryOperationContext(OperationContext.Current); this.findRequest = new FindRequestResponseContext(this.GetFindCriteria(probeMessage), this); if (this.ProcessFindRequest()) { this.Complete(true); return; } } } protected virtual bool Validate(TProbeMessage probeMessage) { return (DiscoveryService.EnsureMessageId() && this.ValidateContent(probeMessage) && this.EnsureNotDuplicate()); } protected abstract bool ValidateContent(TProbeMessage probeMessage); protected abstract FindCriteria GetFindCriteria(TProbeMessage probeMessage); protected abstract TResponseMessage GetProbeResponse( DiscoveryMessageSequence discoveryMessageSequence, Collection matchingEndpoints); protected TResponseMessage End() { this.context.AddressRequestResponseMessage(OperationContext.Current); return this.GetProbeResponse( this.discoveryServiceImpl.GetNextMessageSequence(), this.findRequest.MatchingEndpoints); } static bool OnOnFindCompleted(IAsyncResult result) { ProbeRequestResponseAsyncResult thisPtr = (ProbeRequestResponseAsyncResult )result.AsyncState; lock (thisPtr.findCompletedLock) { thisPtr.isFindCompleted = true; } thisPtr.discoveryServiceImpl.EndFind(result); return true; } bool ProcessFindRequest() { IAsyncResult result = this.discoveryServiceImpl.BeginFind( this.findRequest, this.PrepareAsyncCompletion(onOnFindCompletedCallback), this); return (result.CompletedSynchronously && OnOnFindCompleted(result)); } bool EnsureNotDuplicate() { bool isDuplicate = this.discoveryServiceImpl.IsDuplicate(OperationContext.Current.IncomingMessageHeaders.MessageId); if (isDuplicate && TD.DuplicateDiscoveryMessageIsEnabled()) { TD.DuplicateDiscoveryMessage( ProtocolStrings.TracingStrings.Probe, OperationContext.Current.IncomingMessageHeaders.MessageId.ToString()); } return !isDuplicate; } class FindRequestResponseContext : FindRequestContext { Collection matchingEndpoints; readonly ProbeRequestResponseAsyncResult probeRequestResponseAsyncResult; public FindRequestResponseContext( FindCriteria criteria, ProbeRequestResponseAsyncResult probeRequestResponseAsyncResult) : base(criteria) { this.matchingEndpoints = new Collection (); this.probeRequestResponseAsyncResult = probeRequestResponseAsyncResult; } public Collection MatchingEndpoints { get { return this.matchingEndpoints; } } protected override void OnAddMatchingEndpoint(EndpointDiscoveryMetadata matchingEndpoint) { lock (this.probeRequestResponseAsyncResult.findCompletedLock) { if (this.probeRequestResponseAsyncResult.isFindCompleted) { throw FxTrace.Exception.AsError( new InvalidOperationException(SR.DiscoveryCannotAddMatchingEndpoint)); } else { this.matchingEndpoints.Add(matchingEndpoint); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
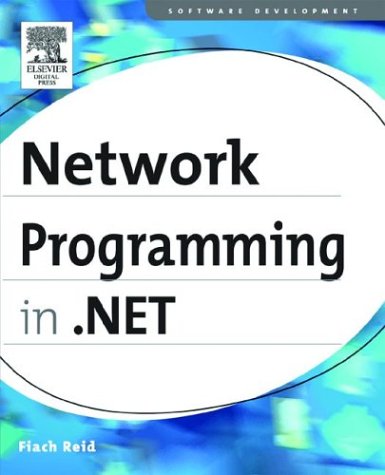
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeGeneratorOptions.cs
- PrintController.cs
- BoundPropertyEntry.cs
- WorkflowServiceHostFactory.cs
- LinkDesigner.cs
- Connector.xaml.cs
- XmlSchemaSimpleTypeRestriction.cs
- URLString.cs
- EnlistmentTraceIdentifier.cs
- FontResourceCache.cs
- X509CertificateInitiatorClientCredential.cs
- InterleavedZipPartStream.cs
- FontDifferentiator.cs
- BufferModesCollection.cs
- RichTextBox.cs
- BooleanKeyFrameCollection.cs
- SafeNativeMethods.cs
- ResourceContainerWrapper.cs
- HtmlFormParameterReader.cs
- RSAPKCS1KeyExchangeFormatter.cs
- XPathDocumentBuilder.cs
- DesignOnlyAttribute.cs
- CompilerGeneratedAttribute.cs
- NativeMethods.cs
- XmlMemberMapping.cs
- ProxyBuilder.cs
- FixedTextBuilder.cs
- NativeMethods.cs
- XmlStreamStore.cs
- RegexStringValidator.cs
- MemoryRecordBuffer.cs
- CodeExporter.cs
- RadioButton.cs
- CodeCompiler.cs
- WindowsScrollBar.cs
- GregorianCalendarHelper.cs
- FormViewDesigner.cs
- QilTypeChecker.cs
- DNS.cs
- Renderer.cs
- RelationshipNavigation.cs
- FlowPosition.cs
- ImplicitInputBrush.cs
- SynchronizationContext.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- WebPartZoneCollection.cs
- ValidateNames.cs
- ErrorFormatter.cs
- CustomAttribute.cs
- DocumentCollection.cs
- OrderedParallelQuery.cs
- InkPresenter.cs
- EnvironmentPermission.cs
- SizeConverter.cs
- DropSource.cs
- SpeechRecognitionEngine.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- WindowsListBox.cs
- Ref.cs
- EntityTransaction.cs
- ContentDisposition.cs
- PropertyRef.cs
- MTConfigUtil.cs
- DeploymentExceptionMapper.cs
- SafeTimerHandle.cs
- HttpListenerException.cs
- MediaScriptCommandRoutedEventArgs.cs
- NullableConverter.cs
- EmissiveMaterial.cs
- BaseProcessProtocolHandler.cs
- DataGridItemCollection.cs
- SoapElementAttribute.cs
- ExitEventArgs.cs
- AutoCompleteStringCollection.cs
- MimeReturn.cs
- PasswordPropertyTextAttribute.cs
- Span.cs
- DynamicPropertyHolder.cs
- ElementProxy.cs
- FunctionImportMapping.cs
- altserialization.cs
- ConfigXmlAttribute.cs
- MyContact.cs
- DesignerForm.cs
- SapiRecognizer.cs
- InstanceDescriptor.cs
- MatrixConverter.cs
- TextElementEditingBehaviorAttribute.cs
- SingleAnimationUsingKeyFrames.cs
- ProxySimple.cs
- Application.cs
- XmlMtomWriter.cs
- ToolStripKeyboardHandlingService.cs
- CodeDelegateCreateExpression.cs
- DataServiceHost.cs
- WebPartActionVerb.cs
- SecurityTokenTypes.cs
- MemberCollection.cs
- ControlBindingsCollection.cs
- DataGridViewCellStyle.cs