Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / Activities / Presentation / TypeCollectionDesigner.xaml.cs / 1586724 / TypeCollectionDesigner.xaml.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System; using System.Activities.Presentation.Model; using System.Activities.Presentation.View; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Linq; using System.Runtime; using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.ComponentModel; using System.Windows.Threading; using System.Windows.Data; [Fx.Tag.XamlVisible(false)] partial class TypeCollectionDesigner { public static readonly DependencyProperty ContextProperty = DependencyProperty.Register( "Context", typeof(EditingContext), typeof(TypeCollectionDesigner), new UIPropertyMetadata(null)); [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes", Justification = "Type RoutedCommand is immutable.")] public static readonly ICommand AddNewTypeCommand = new RoutedCommand("AddNewType", typeof(TypeCollectionDesigner)); DataGridHelper dgHelper; ObservableCollectionwrapperCollection; public TypeCollectionDesigner() { InitializeComponent(); } // The collection of Type objects to display when type collection designer is opened. internal IEnumerable InitialTypeCollection { set { this.wrapperCollection = new ObservableCollection (value.Select(type => new TypeWrapper(type))); this.typesDataGrid.ItemsSource = this.wrapperCollection; } } // The collction of Type objects in the type collection designer when user clicks OK. public IEnumerable UpdatedTypeCollection { get { return wrapperCollection.Select(wrapper => wrapper.Type); } } public EditingContext Context { get { return (EditingContext)GetValue(ContextProperty); } set { SetValue(ContextProperty, value); } } public bool AllowDuplicate { get; set; } internal WorkflowElementDialog ParentDialog { get; set; } internal bool OnOK() { if (!this.AllowDuplicate) { List list = new List (); foreach (TypeWrapper tw in this.wrapperCollection) { if (list.Any (entry => Type.Equals(entry.Type, tw.Type))) { ErrorReporting.ShowErrorMessage(string.Format(CultureInfo.CurrentCulture, (string)this.FindResource("duplicateEntryErrorMessage"), TypeNameHelper.GetDisplayName(tw.Type, true))); return false; } list.Add(tw); } } return true; } // The DataGrid does not bubble up KeyDown event and we expect the upper window to be closed when ESC key is down. // Thus we added an event handler in DataGrid to handle ESC key and closes the uppper window. void OnTypesDataGridRowKeyDown(object sender, KeyEventArgs args) { DataGridRow row = (DataGridRow)sender; if (args.Key == Key.Escape && !row.IsEditing && this.ParentDialog != null) { this.ParentDialog.CloseDialog(false); } } protected override void OnInitialized(EventArgs e) { this.dgHelper = new DataGridHelper(this.typesDataGrid, this); this.dgHelper.AddNewRowContent = this.FindResource("addNewRowLabel"); this.dgHelper.AddNewRowCommand = AddNewTypeCommand; base.OnInitialized(e); } void OnAddTypeExecuted(object sender, ExecutedRoutedEventArgs e) { var newEntry = new TypeWrapper(typeof(Object)); this.wrapperCollection.Add(newEntry); this.dgHelper.BeginRowEdit(newEntry); e.Handled = true; } sealed class TypeWrapper : DependencyObject { public static readonly DependencyProperty TypeProperty = DependencyProperty.Register("Type", typeof(Type), typeof(TypeWrapper)); //Default constructor is required by DataGrid to load NewItemPlaceHolder row and this constructor will never be called. //Since we've already customized the new row template and hooked over creating new object event. public TypeWrapper() { throw FxTrace.Exception.AsError(new NotSupportedException()); } public TypeWrapper(Type type) { this.Type = type; } public Type Type { get { return (Type)GetValue(TypeProperty); } set { SetValue(TypeProperty, value); } } // For screen reader to read the DataGrid row. public override string ToString() { if (this.Type != null && !string.IsNullOrEmpty(this.Type.Name)) { return this.Type.Name; } return "null"; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
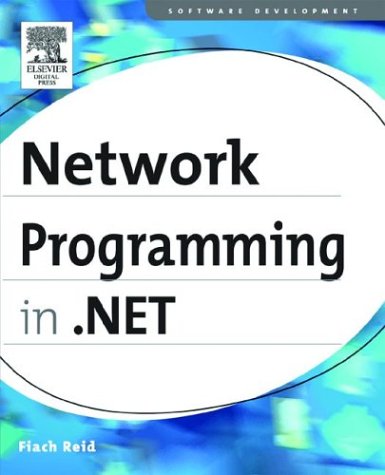
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListItem.cs
- XmlQualifiedName.cs
- GridViewSelectEventArgs.cs
- SafeReversePInvokeHandle.cs
- DbgCompiler.cs
- MergePropertyDescriptor.cs
- ConnectionStringSettingsCollection.cs
- Helpers.cs
- InkCanvas.cs
- VirtualizingPanel.cs
- StylusPointPropertyInfo.cs
- StringBlob.cs
- Executor.cs
- _NestedSingleAsyncResult.cs
- ConfigurationManagerHelperFactory.cs
- Point.cs
- MailMessage.cs
- UpdatableWrapper.cs
- NetworkAddressChange.cs
- FontUnit.cs
- OutputScopeManager.cs
- TemplatePagerField.cs
- EmptyReadOnlyDictionaryInternal.cs
- figurelength.cs
- ElapsedEventArgs.cs
- WebPartConnectionsConnectVerb.cs
- UnsafeNativeMethods.cs
- BaseDataBoundControl.cs
- SrgsGrammarCompiler.cs
- SizeF.cs
- CodeDirectoryCompiler.cs
- SqlReorderer.cs
- WebSysDisplayNameAttribute.cs
- SortDescription.cs
- ActivityInstanceReference.cs
- BooleanSwitch.cs
- DecoderExceptionFallback.cs
- DataGridViewTextBoxCell.cs
- JsonDataContract.cs
- CancellationTokenRegistration.cs
- Atom10FormatterFactory.cs
- SerializationStore.cs
- EdmSchemaError.cs
- SyndicationCategory.cs
- DataGridViewImageColumn.cs
- SqlBuffer.cs
- BCLDebug.cs
- QilTargetType.cs
- StatusBarDrawItemEvent.cs
- Grid.cs
- ConnectionsZone.cs
- ControlCodeDomSerializer.cs
- FormViewUpdatedEventArgs.cs
- PreviewPageInfo.cs
- WindowsListViewItemStartMenu.cs
- CollectionViewGroupRoot.cs
- XmlMessageFormatter.cs
- InputGestureCollection.cs
- PanelDesigner.cs
- SchemaCollectionPreprocessor.cs
- PropertyTabChangedEvent.cs
- TableLayoutStyleCollection.cs
- DesignerVerb.cs
- LocalizableResourceBuilder.cs
- DiscardableAttribute.cs
- ButtonBase.cs
- InputScopeConverter.cs
- SettingsPropertyNotFoundException.cs
- MethodCallTranslator.cs
- GeometryModel3D.cs
- GuidelineCollection.cs
- AccessDataSourceView.cs
- MetadataSerializer.cs
- HtmlTableRow.cs
- NumberFormatInfo.cs
- XPathNode.cs
- SmtpMail.cs
- WindowsScroll.cs
- DbDataReader.cs
- DBConnectionString.cs
- ContextStack.cs
- FilterFactory.cs
- ListViewGroupConverter.cs
- DbProviderSpecificTypePropertyAttribute.cs
- DocumentReference.cs
- CatalogPartCollection.cs
- FtpCachePolicyElement.cs
- AssemblySettingAttributes.cs
- ColorConvertedBitmapExtension.cs
- DocComment.cs
- RotateTransform.cs
- DataGridItemEventArgs.cs
- MoveSizeWinEventHandler.cs
- DBNull.cs
- CompoundFileDeflateTransform.cs
- TextReader.cs
- IndexingContentUnit.cs
- TypeRefElement.cs
- XMLSyntaxException.cs
- KeySpline.cs