Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / Point.cs / 1 / Point.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; using System.Diagnostics.CodeAnalysis; using System.Globalization; ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [ TypeConverterAttribute(typeof(PointConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [SuppressMessage("Microsoft.Usage", "CA2225:OperatorOverloadsHaveNamedAlternates")] public struct Point { ////// /// Creates a new instance of the public static readonly Point Empty = new Point(); private int x; private int y; ///class /// with member data left uninitialized. /// /// /// Initializes a new instance of the public Point(int x, int y) { this.x = x; this.y = y; } ///class /// with the specified coordinates. /// /// /// public Point(Size sz) { this.x = sz.Width; this.y = sz.Height; } ////// Initializes a new instance of the ///class /// from a . /// /// /// Initializes a new instance of the Point class using /// coordinates specified by an integer value. /// public Point(int dw) { this.x = (short)LOWORD(dw); this.y = (short)HIWORD(dw); } ////// /// [Browsable(false)] public bool IsEmpty { get { return x == 0 && y == 0; } } ////// Gets a value indicating whether this ///is empty. /// /// /// Gets the x-coordinate of this public int X { get { return x; } set { x = value; } } ///. /// /// /// public int Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static implicit operator PointF(Point p) { return new PointF(p.X, p.Y); } ////// Creates a ///with the coordinates of the specified /// /// . /// /// /// public static explicit operator Size(Point p) { return new Size(p.X, p.Y); } ////// Creates a ///with the coordinates of the specified . /// /// /// public static Point operator +(Point pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static Point operator -(Point pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(Point left, Point right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(Point left, Point right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static Point Add(Point pt, Size sz) { return new Point(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static Point Subtract(Point pt, Size sz) { return new Point(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// Converts a PointF to a Point by performing a ceiling operation on /// all the coordinates. /// public static Point Ceiling(PointF value) { return new Point((int)Math.Ceiling(value.X), (int)Math.Ceiling(value.Y)); } ////// /// Converts a PointF to a Point by performing a truncate operation on /// all the coordinates. /// public static Point Truncate(PointF value) { return new Point((int)value.X, (int)value.Y); } ////// /// Converts a PointF to a Point by performing a round operation on /// all the coordinates. /// public static Point Round(PointF value) { return new Point((int)Math.Round(value.X), (int)Math.Round(value.Y)); } ////// /// public override bool Equals(object obj) { if (!(obj is Point)) return false; Point comp = (Point)obj; // Note value types can't have derived classes, so we don't need // to check the types of the objects here. -- [....], 2/21/2001 return comp.X == this.X && comp.Y == this.Y; } ////// Specifies whether this ///contains /// the same coordinates as the specified . /// /// /// public override int GetHashCode() { return x ^ y; } /** * Offset the current Point object by the given amount */ ////// Returns a hash code. /// ////// /// Translates this public void Offset(int dx, int dy) { X += dx; Y += dy; } ///by the specified amount. /// /// /// Translates this public void Offset(Point p) { Offset(p.X, p.Y); } ///by the specified amount. /// /// /// public override string ToString() { return "{X=" + X.ToString(CultureInfo.CurrentCulture) + ",Y=" + Y.ToString(CultureInfo.CurrentCulture) + "}"; } private static int HIWORD(int n) { return(n >> 16) & 0xffff; } private static int LOWORD(int n) { return n & 0xffff; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Converts this ////// to a human readable /// string. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; using System.Diagnostics.CodeAnalysis; using System.Globalization; ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [ TypeConverterAttribute(typeof(PointConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [SuppressMessage("Microsoft.Usage", "CA2225:OperatorOverloadsHaveNamedAlternates")] public struct Point { ////// /// Creates a new instance of the public static readonly Point Empty = new Point(); private int x; private int y; ///class /// with member data left uninitialized. /// /// /// Initializes a new instance of the public Point(int x, int y) { this.x = x; this.y = y; } ///class /// with the specified coordinates. /// /// /// public Point(Size sz) { this.x = sz.Width; this.y = sz.Height; } ////// Initializes a new instance of the ///class /// from a . /// /// /// Initializes a new instance of the Point class using /// coordinates specified by an integer value. /// public Point(int dw) { this.x = (short)LOWORD(dw); this.y = (short)HIWORD(dw); } ////// /// [Browsable(false)] public bool IsEmpty { get { return x == 0 && y == 0; } } ////// Gets a value indicating whether this ///is empty. /// /// /// Gets the x-coordinate of this public int X { get { return x; } set { x = value; } } ///. /// /// /// public int Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static implicit operator PointF(Point p) { return new PointF(p.X, p.Y); } ////// Creates a ///with the coordinates of the specified /// /// . /// /// /// public static explicit operator Size(Point p) { return new Size(p.X, p.Y); } ////// Creates a ///with the coordinates of the specified . /// /// /// public static Point operator +(Point pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static Point operator -(Point pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(Point left, Point right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(Point left, Point right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static Point Add(Point pt, Size sz) { return new Point(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static Point Subtract(Point pt, Size sz) { return new Point(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// Converts a PointF to a Point by performing a ceiling operation on /// all the coordinates. /// public static Point Ceiling(PointF value) { return new Point((int)Math.Ceiling(value.X), (int)Math.Ceiling(value.Y)); } ////// /// Converts a PointF to a Point by performing a truncate operation on /// all the coordinates. /// public static Point Truncate(PointF value) { return new Point((int)value.X, (int)value.Y); } ////// /// Converts a PointF to a Point by performing a round operation on /// all the coordinates. /// public static Point Round(PointF value) { return new Point((int)Math.Round(value.X), (int)Math.Round(value.Y)); } ////// /// public override bool Equals(object obj) { if (!(obj is Point)) return false; Point comp = (Point)obj; // Note value types can't have derived classes, so we don't need // to check the types of the objects here. -- [....], 2/21/2001 return comp.X == this.X && comp.Y == this.Y; } ////// Specifies whether this ///contains /// the same coordinates as the specified . /// /// /// public override int GetHashCode() { return x ^ y; } /** * Offset the current Point object by the given amount */ ////// Returns a hash code. /// ////// /// Translates this public void Offset(int dx, int dy) { X += dx; Y += dy; } ///by the specified amount. /// /// /// Translates this public void Offset(Point p) { Offset(p.X, p.Y); } ///by the specified amount. /// /// /// public override string ToString() { return "{X=" + X.ToString(CultureInfo.CurrentCulture) + ",Y=" + Y.ToString(CultureInfo.CurrentCulture) + "}"; } private static int HIWORD(int n) { return(n >> 16) & 0xffff; } private static int LOWORD(int n) { return n & 0xffff; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Converts this ////// to a human readable /// string. ///
Link Menu
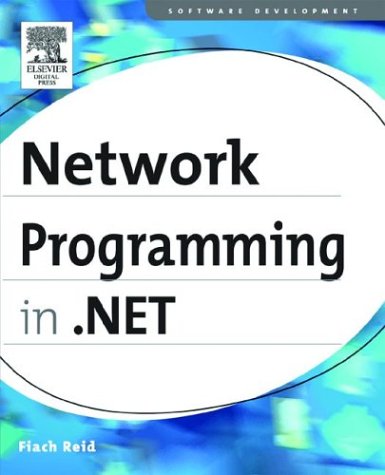
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Debug.cs
- XmlDocumentViewSchema.cs
- XmlReaderDelegator.cs
- XmlRawWriterWrapper.cs
- HttpProfileBase.cs
- SafeRightsManagementSessionHandle.cs
- WinEventHandler.cs
- PopupEventArgs.cs
- DataSourceHelper.cs
- CompositionCommandSet.cs
- TypeResolver.cs
- XmlSchemaInferenceException.cs
- GregorianCalendar.cs
- UnSafeCharBuffer.cs
- StylusShape.cs
- RegexRunner.cs
- DbMetaDataColumnNames.cs
- Base64Decoder.cs
- DataGridPageChangedEventArgs.cs
- EventLogReader.cs
- DiagnosticsConfigurationHandler.cs
- UnauthorizedWebPart.cs
- SafeFileMappingHandle.cs
- C14NUtil.cs
- FileVersionInfo.cs
- WorkflowViewService.cs
- Endpoint.cs
- DataGridClipboardHelper.cs
- AngleUtil.cs
- ViewCellSlot.cs
- MenuEventArgs.cs
- ConnectionManagementElement.cs
- RequestCacheValidator.cs
- SwitchCase.cs
- PersonalizableTypeEntry.cs
- EntityTransaction.cs
- EmbeddedMailObjectsCollection.cs
- SerializeAbsoluteContext.cs
- ParsedRoute.cs
- XPathDocumentNavigator.cs
- InputElement.cs
- EmbossBitmapEffect.cs
- Schema.cs
- DataServiceHostFactory.cs
- ServiceOperation.cs
- MobileControlBuilder.cs
- EnumValAlphaComparer.cs
- Events.cs
- StylusPointPropertyInfo.cs
- ComponentConverter.cs
- BindValidationContext.cs
- MaterialCollection.cs
- AVElementHelper.cs
- SqlFlattener.cs
- CompiledQuery.cs
- SQlBooleanStorage.cs
- X509Utils.cs
- PerformanceCounterManager.cs
- WorkerRequest.cs
- MatrixConverter.cs
- XmlDocumentFragment.cs
- DataGridViewAutoSizeModeEventArgs.cs
- ConnectionPoint.cs
- ContainerParaClient.cs
- QueryLifecycle.cs
- OlePropertyStructs.cs
- ObjectSecurity.cs
- DrawingAttributesDefaultValueFactory.cs
- StrokeNode.cs
- _HeaderInfo.cs
- StaticExtensionConverter.cs
- PackageFilter.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- RijndaelManaged.cs
- EmissiveMaterial.cs
- SatelliteContractVersionAttribute.cs
- GPRECTF.cs
- VirtualDirectoryMappingCollection.cs
- XmlQueryType.cs
- ItemType.cs
- LockedBorderGlyph.cs
- AppDomainFactory.cs
- DrawingContextWalker.cs
- AdditionalEntityFunctions.cs
- DBBindings.cs
- StringDictionaryCodeDomSerializer.cs
- FullTextState.cs
- OpCellTreeNode.cs
- XmlILIndex.cs
- Rule.cs
- ModelUIElement3D.cs
- DoubleConverter.cs
- SafeLibraryHandle.cs
- XmlSchemaSequence.cs
- TextServicesManager.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- MetadataCache.cs
- DataBoundControlAdapter.cs
- SqlDataSourceSelectingEventArgs.cs
- TextRunProperties.cs