Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / DeflateStreamAsyncResult.cs / 1305376 / DeflateStreamAsyncResult.cs
namespace System.IO.Compression { using System.Threading; internal class DeflateStreamAsyncResult : IAsyncResult { public byte[] buffer; public int offset; public int count; // disable csharp compiler warning #0414: field assigned unused value #pragma warning disable 0414 public bool isWrite; #pragma warning restore 0414 private object m_AsyncObject; // Caller's async object. private object m_AsyncState; // Caller's state object. private AsyncCallback m_AsyncCallback; // Caller's callback method. private object m_Result; // Final IO result to be returned byt the End*() method. internal bool m_CompletedSynchronously; // true if the operation completed synchronously. private int m_InvokedCallback; // 0 is callback is not called private int m_Completed; // 0 if not completed >0 otherwise. private object m_Event; // lazy allocated event to be returned in the IAsyncResult for the client to wait on public DeflateStreamAsyncResult(object asyncObject, object asyncState, AsyncCallback asyncCallback, byte[] buffer, int offset, int count) { this.buffer = buffer; this.offset = offset; this.count = count; m_CompletedSynchronously = true; m_AsyncObject = asyncObject; m_AsyncState = asyncState; m_AsyncCallback = asyncCallback; } // Interface method to return the caller's state object. public object AsyncState { get { return m_AsyncState; } } // Interface property to return a WaitHandle that can be waited on for I/O completion. // This property implements lazy event creation. // the event object is only created when this property is accessed, // since we're internally only using callbacks, as long as the user is using // callbacks as well we will not create an event at all. public WaitHandle AsyncWaitHandle { get { // save a copy of the completion status int savedCompleted = m_Completed; if (m_Event == null) { // lazy allocation of the event: // if this property is never accessed this object is never created Interlocked.CompareExchange(ref m_Event, new ManualResetEvent(savedCompleted != 0), null); } ManualResetEvent castedEvent = (ManualResetEvent)m_Event; if (savedCompleted == 0 && m_Completed != 0) { // if, while the event was created in the reset state, // the IO operation completed, set the event here. castedEvent.Set(); } return castedEvent; } } // Interface property, returning synchronous completion status. public bool CompletedSynchronously { get { return m_CompletedSynchronously; } } // Interface property, returning completion status. public bool IsCompleted { get { return m_Completed != 0; } } // Internal property for setting the IO result. internal object Result { get { return m_Result; } } internal void Close() { if (m_Event != null) { ((ManualResetEvent)m_Event).Close(); } } internal void InvokeCallback(bool completedSynchronously, object result) { Complete(completedSynchronously, result); } internal void InvokeCallback(object result) { Complete(result); } // Internal method for setting completion. // As a side effect, we'll signal the WaitHandle event and clean up. private void Complete(bool completedSynchronously, object result) { m_CompletedSynchronously = completedSynchronously; Complete(result); } private void Complete(object result) { m_Result = result; // Set IsCompleted and the event only after the usercallback method. Interlocked.Increment(ref m_Completed); if (m_Event != null) { ((ManualResetEvent)m_Event).Set(); } if (Interlocked.Increment(ref m_InvokedCallback) == 1) { if (m_AsyncCallback != null) { m_AsyncCallback(this); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.IO.Compression { using System.Threading; internal class DeflateStreamAsyncResult : IAsyncResult { public byte[] buffer; public int offset; public int count; // disable csharp compiler warning #0414: field assigned unused value #pragma warning disable 0414 public bool isWrite; #pragma warning restore 0414 private object m_AsyncObject; // Caller's async object. private object m_AsyncState; // Caller's state object. private AsyncCallback m_AsyncCallback; // Caller's callback method. private object m_Result; // Final IO result to be returned byt the End*() method. internal bool m_CompletedSynchronously; // true if the operation completed synchronously. private int m_InvokedCallback; // 0 is callback is not called private int m_Completed; // 0 if not completed >0 otherwise. private object m_Event; // lazy allocated event to be returned in the IAsyncResult for the client to wait on public DeflateStreamAsyncResult(object asyncObject, object asyncState, AsyncCallback asyncCallback, byte[] buffer, int offset, int count) { this.buffer = buffer; this.offset = offset; this.count = count; m_CompletedSynchronously = true; m_AsyncObject = asyncObject; m_AsyncState = asyncState; m_AsyncCallback = asyncCallback; } // Interface method to return the caller's state object. public object AsyncState { get { return m_AsyncState; } } // Interface property to return a WaitHandle that can be waited on for I/O completion. // This property implements lazy event creation. // the event object is only created when this property is accessed, // since we're internally only using callbacks, as long as the user is using // callbacks as well we will not create an event at all. public WaitHandle AsyncWaitHandle { get { // save a copy of the completion status int savedCompleted = m_Completed; if (m_Event == null) { // lazy allocation of the event: // if this property is never accessed this object is never created Interlocked.CompareExchange(ref m_Event, new ManualResetEvent(savedCompleted != 0), null); } ManualResetEvent castedEvent = (ManualResetEvent)m_Event; if (savedCompleted == 0 && m_Completed != 0) { // if, while the event was created in the reset state, // the IO operation completed, set the event here. castedEvent.Set(); } return castedEvent; } } // Interface property, returning synchronous completion status. public bool CompletedSynchronously { get { return m_CompletedSynchronously; } } // Interface property, returning completion status. public bool IsCompleted { get { return m_Completed != 0; } } // Internal property for setting the IO result. internal object Result { get { return m_Result; } } internal void Close() { if (m_Event != null) { ((ManualResetEvent)m_Event).Close(); } } internal void InvokeCallback(bool completedSynchronously, object result) { Complete(completedSynchronously, result); } internal void InvokeCallback(object result) { Complete(result); } // Internal method for setting completion. // As a side effect, we'll signal the WaitHandle event and clean up. private void Complete(bool completedSynchronously, object result) { m_CompletedSynchronously = completedSynchronously; Complete(result); } private void Complete(object result) { m_Result = result; // Set IsCompleted and the event only after the usercallback method. Interlocked.Increment(ref m_Completed); if (m_Event != null) { ((ManualResetEvent)m_Event).Set(); } if (Interlocked.Increment(ref m_InvokedCallback) == 1) { if (m_AsyncCallback != null) { m_AsyncCallback(this); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
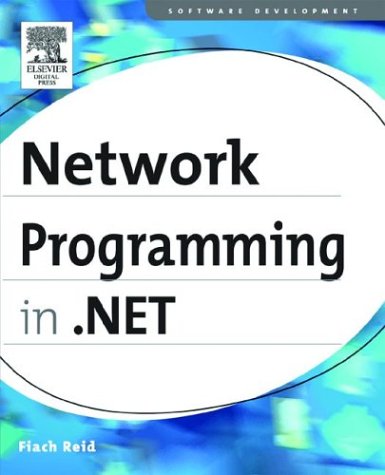
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProcessModuleCollection.cs
- Validator.cs
- Int32.cs
- HtmlTable.cs
- DataGridViewRowEventArgs.cs
- OdbcDataAdapter.cs
- ErrorEventArgs.cs
- WindowsTitleBar.cs
- CodeDirectoryCompiler.cs
- PasswordBox.cs
- AlternateViewCollection.cs
- BitmapMetadata.cs
- dsa.cs
- NamedPipeAppDomainProtocolHandler.cs
- RoutedUICommand.cs
- AssociationTypeEmitter.cs
- MsmqProcessProtocolHandler.cs
- ToolStripDropDownClosedEventArgs.cs
- IChannel.cs
- DataGridViewTextBoxEditingControl.cs
- SafeFileHandle.cs
- SelectionListDesigner.cs
- ClientRuntimeConfig.cs
- CodeNamespaceImportCollection.cs
- SortFieldComparer.cs
- SQLBytesStorage.cs
- EncryptedXml.cs
- DashStyle.cs
- ActivityExecutorOperation.cs
- ToolTip.cs
- DesignColumn.cs
- SynchronizedMessageSource.cs
- FormViewDeletedEventArgs.cs
- ChangeInterceptorAttribute.cs
- printdlgexmarshaler.cs
- BrowserCapabilitiesFactoryBase.cs
- InstanceOwner.cs
- FieldCollectionEditor.cs
- MenuItemBinding.cs
- MediaElement.cs
- SymLanguageType.cs
- Mutex.cs
- ResourcePool.cs
- OleDbRowUpdatedEvent.cs
- EntityDataSourceSelectedEventArgs.cs
- ItemCollection.cs
- HelpProvider.cs
- ServiceEndpointAssociationProvider.cs
- ContextActivityUtils.cs
- SocketInformation.cs
- ExpandSegment.cs
- SmtpException.cs
- _OverlappedAsyncResult.cs
- CalendarAutoFormatDialog.cs
- QueueProcessor.cs
- ExtentCqlBlock.cs
- TearOffProxy.cs
- FormViewRow.cs
- StrokeNodeOperations2.cs
- FacetDescriptionElement.cs
- ConnectionModeReader.cs
- ComboBoxAutomationPeer.cs
- Button.cs
- Match.cs
- LabelInfo.cs
- CodeArgumentReferenceExpression.cs
- ResourcePart.cs
- TimelineCollection.cs
- BamlLocalizabilityResolver.cs
- EventMappingSettings.cs
- NoneExcludedImageIndexConverter.cs
- SafeArrayRankMismatchException.cs
- Models.cs
- DebugInfoExpression.cs
- StorageTypeMapping.cs
- wmiprovider.cs
- MarkupExtensionReturnTypeAttribute.cs
- SecurityProtocolFactory.cs
- AsyncResult.cs
- WebPartManagerInternals.cs
- GeometryDrawing.cs
- Buffer.cs
- TripleDESCryptoServiceProvider.cs
- PinnedBufferMemoryStream.cs
- AmbientProperties.cs
- WebControl.cs
- ComboBoxRenderer.cs
- DefinitionUpdate.cs
- TreeNodeStyle.cs
- PackageRelationshipCollection.cs
- ColorDialog.cs
- WindowsListViewItemStartMenu.cs
- DataGridParentRows.cs
- Vector3DAnimationUsingKeyFrames.cs
- TransformGroup.cs
- SoapProtocolImporter.cs
- Dictionary.cs
- ConstrainedDataObject.cs
- ListViewDataItem.cs
- RijndaelManaged.cs