Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / TearOffProxy.cs / 1 / TearOffProxy.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.ServiceModel.Channels; using System.Runtime.InteropServices; using System.Runtime.Remoting.Proxies; using System.Runtime.Remoting.Messaging; using System.Runtime.Remoting.Services; using System.Runtime.Remoting; using System.Runtime.Serialization; using System.ServiceModel; using System.Reflection; using System.Collections.Generic; internal class TearOffProxy : RealProxy, IDisposable { ICreateServiceChannel serviceChannelCreator; DictionarybaseTypeToInterfaceMethod; internal TearOffProxy (ICreateServiceChannel serviceChannelCreator, Type proxiedType) : base (proxiedType) { if (serviceChannelCreator == null) { DiagnosticUtility.DebugAssert("ServiceChannelCreator cannot be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } this.serviceChannelCreator = serviceChannelCreator; baseTypeToInterfaceMethod = new Dictionary (); } public override IMessage Invoke(IMessage message) { RealProxy delegatingProxy = null; IMethodCallMessage msg = message as IMethodCallMessage; try { delegatingProxy = serviceChannelCreator.CreateChannel (); } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; return new ReturnMessage (DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (e.GetBaseException().Message, Marshal.GetHRForException (e.GetBaseException()))), msg); } MethodBase typeMethod = msg.MethodBase; IRemotingTypeInfo typeInfo = delegatingProxy as IRemotingTypeInfo; if (typeInfo == null) { DiagnosticUtility.DebugAssert("Type Info cannot be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } if (typeInfo.CanCastTo (typeMethod.DeclaringType, null)) { IMessage msgReturned = delegatingProxy.Invoke (message); ReturnMessage returnMsg = msgReturned as ReturnMessage; if ((returnMsg == null) || (returnMsg.Exception == null)) return msgReturned; else return new ReturnMessage (DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (returnMsg.Exception.GetBaseException().Message, Marshal.GetHRForException (returnMsg.Exception.GetBaseException()))), msg); } else { return new ReturnMessage (DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (SR.GetString(SR.OperationNotFound, typeMethod.Name), HR.DISP_E_UNKNOWNNAME)), msg); } } void IDisposable.Dispose () { serviceChannelCreator = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
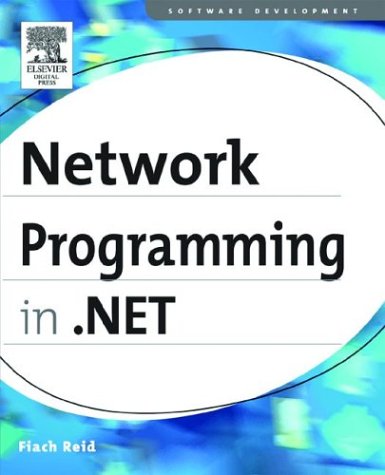
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlColumnizer.cs
- WebPartActionVerb.cs
- PartialTrustHelpers.cs
- WmlCalendarAdapter.cs
- UserCancellationException.cs
- WinInetCache.cs
- ResourceReader.cs
- SqlDataSourceQueryEditorForm.cs
- AngleUtil.cs
- Queue.cs
- CroppedBitmap.cs
- Utils.cs
- GuidelineSet.cs
- SqlLiftWhereClauses.cs
- DataFieldCollectionEditor.cs
- ContainerParagraph.cs
- SocketException.cs
- SecurityTokenResolver.cs
- DetailsViewModeEventArgs.cs
- CustomAttributeSerializer.cs
- RuntimeCompatibilityAttribute.cs
- XmlQueryType.cs
- FixedSOMSemanticBox.cs
- BindingUtils.cs
- CharEnumerator.cs
- SoapReflectionImporter.cs
- NonClientArea.cs
- RectangleF.cs
- ErrorFormatterPage.cs
- ErrorInfoXmlDocument.cs
- XPathException.cs
- CalendarDesigner.cs
- DataBoundControl.cs
- MemberHolder.cs
- InkPresenter.cs
- WindowsProgressbar.cs
- CodeGeneratorOptions.cs
- ResXBuildProvider.cs
- StaticFileHandler.cs
- SortedDictionary.cs
- XmlIlVisitor.cs
- Point4D.cs
- ControlPersister.cs
- SubstitutionResponseElement.cs
- IPEndPoint.cs
- ObjectManager.cs
- XmlNodeChangedEventArgs.cs
- XmlElementCollection.cs
- PassportIdentity.cs
- ModelItemImpl.cs
- SqlBulkCopyColumnMapping.cs
- NativeMethods.cs
- InputBinding.cs
- DictionaryContent.cs
- CompilationLock.cs
- EllipseGeometry.cs
- DocumentXPathNavigator.cs
- Internal.cs
- Brush.cs
- VisualStyleInformation.cs
- Filter.cs
- ICspAsymmetricAlgorithm.cs
- FileRegion.cs
- TypeToTreeConverter.cs
- HashSet.cs
- CompositeDataBoundControl.cs
- Sql8ExpressionRewriter.cs
- Command.cs
- DataGridViewLinkCell.cs
- XmlSchemaSimpleTypeList.cs
- _AcceptOverlappedAsyncResult.cs
- SqlProviderUtilities.cs
- DrawingCollection.cs
- SmiConnection.cs
- HttpCookie.cs
- SymbolEqualComparer.cs
- NameValueConfigurationElement.cs
- TemplateControlParser.cs
- XPathAncestorIterator.cs
- StrongName.cs
- FilePrompt.cs
- AvTrace.cs
- ObjectViewFactory.cs
- NameValuePair.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- XmlBinaryWriterSession.cs
- RelationshipFixer.cs
- ScriptReferenceEventArgs.cs
- TextInfo.cs
- Msmq4PoisonHandler.cs
- ExternalCalls.cs
- StyleTypedPropertyAttribute.cs
- _ScatterGatherBuffers.cs
- ResXDataNode.cs
- XmlHierarchyData.cs
- WebPartCancelEventArgs.cs
- BitSet.cs
- SoapFault.cs
- CancellationToken.cs
- ToolStripContentPanel.cs