Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Documents / ViewManager.cs / 1305376 / ViewManager.cs
namespace System.Activities.Presentation.Documents { using System; using System.Collections.Generic; using System.Diagnostics.CodeAnalysis; using System.Windows; using System.Windows.Media; using System.Activities.Presentation; using System.Activities.Presentation.Model; using System.Activities.Presentation.Services; ////// A ViewManager is a class that manages and provides the view /// for the designer. The view manager is used by MarkupDocumentManager /// to provide the view for the designer. /// abstract class ViewManager : IDisposable { ////// DependencyPropertyKey that allows ViewManagers to set the PropertyRedirections /// attached property. /// // FxCop: these are immutable [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] protected static readonly DependencyPropertyKey PropertyRedirectionsPropertyKey = DependencyProperty.RegisterAttachedReadOnly( "PropertyRedirections", typeof(IEnumerable), typeof(ViewManager), null); /// /// Dependency property for the PropertyRedirections attached property. /// public static readonly DependencyProperty PropertyRedirectionsProperty = PropertyRedirectionsPropertyKey.DependencyProperty; ////// Returns the view for the designer. This will return null until /// Initialize has been called. /// public abstract Visual View { get; } ////// Returns an enumeration of property identifiers indicating properties on the model that should be /// redirected on the view. The view must declare public properties of the same name and /// compatible data type. When a value is set into the model, if its property is being redirected /// the value will be set into the redirected property instead. /// /// /// The view to retrieve the set of property redirections for. /// ////// An enumeration of property redirections, or null if there are none. /// public static IEnumerableGetPropertyRedirections(DependencyObject view) { if (view == null) throw FxTrace.Exception.ArgumentNull("view"); return (IEnumerable )view.GetValue(PropertyRedirectionsProperty); } /// /// Initializes this view manager with the given model tree. /// /// The editing context for the designer. ///If model is null. public abstract void Initialize(EditingContext context); ////// Sets an enumeration of property identifiers indicating properties on the model that /// should be redirected to the view. The view must declare public properties of the same name /// and compatible type. When a value is set into the model, if its property is being redirected /// the value will be set into the redirected property instead. /// /// /// protected static void SetPropertyRedirections(DependencyObject view, IEnumerableredirections) { if (view == null) throw FxTrace.Exception.ArgumentNull("view"); view.SetValue(PropertyRedirectionsPropertyKey, redirections); } /// /// Disposes this view manager. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes this view manager. /// True if this object is being disposed, or false if it is finalizing. /// protected virtual void Dispose(bool disposing) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
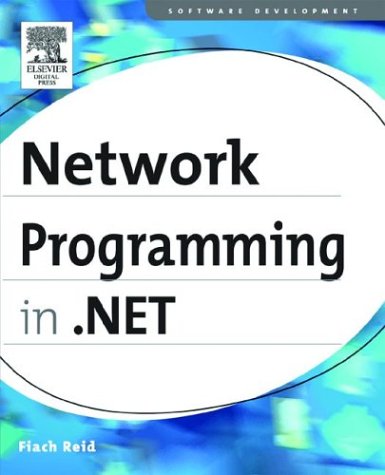
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartDescriptionCollection.cs
- EtwTrackingParticipant.cs
- XmlConvert.cs
- XDeferredAxisSource.cs
- NavigationService.cs
- EffectiveValueEntry.cs
- XPathPatternBuilder.cs
- FamilyMap.cs
- ToolStripRenderer.cs
- TableLayoutStyle.cs
- UIElementCollection.cs
- TemplatedWizardStep.cs
- KeyGesture.cs
- ReaderWriterLockWrapper.cs
- TextReader.cs
- DataGridSortCommandEventArgs.cs
- AspNetSynchronizationContext.cs
- QilFactory.cs
- TreeNodeMouseHoverEvent.cs
- AccessorTable.cs
- ListItemCollection.cs
- LoadWorkflowAsyncResult.cs
- ValueChangedEventManager.cs
- XmlEntityReference.cs
- Directory.cs
- XmlAttributeCollection.cs
- MsiStyleLogWriter.cs
- SymLanguageType.cs
- InstalledFontCollection.cs
- TemplateKeyConverter.cs
- WindowsImpersonationContext.cs
- InstanceOwnerException.cs
- WebServiceHandler.cs
- DataGridViewEditingControlShowingEventArgs.cs
- BitmapMetadataEnumerator.cs
- Message.cs
- DocumentationServerProtocol.cs
- XmlSchemaSimpleContent.cs
- Timeline.cs
- odbcmetadatacollectionnames.cs
- DynamicActivityProperty.cs
- HintTextMaxWidthConverter.cs
- Attribute.cs
- HttpVersion.cs
- DataGridViewButtonColumn.cs
- LinkTarget.cs
- LifetimeServices.cs
- securitycriticaldataformultiplegetandset.cs
- ListControl.cs
- ReflectionServiceProvider.cs
- Error.cs
- InheritablePropertyChangeInfo.cs
- UnsafeNativeMethods.cs
- AppSettingsExpressionBuilder.cs
- XXXOnTypeBuilderInstantiation.cs
- DataSetViewSchema.cs
- FixedDocumentSequencePaginator.cs
- StaticExtension.cs
- TemplateControlBuildProvider.cs
- LicenseManager.cs
- DataGridViewHeaderCell.cs
- RequestCacheValidator.cs
- PerformanceCountersElement.cs
- CompensationDesigner.cs
- RealizedColumnsBlock.cs
- ObjectStateEntry.cs
- InstanceLockLostException.cs
- ContentPosition.cs
- ContainerUIElement3D.cs
- StoragePropertyMapping.cs
- DesignerVerb.cs
- RoutedUICommand.cs
- StreamUpdate.cs
- PathFigureCollectionConverter.cs
- DependencyObjectPropertyDescriptor.cs
- CustomAssemblyResolver.cs
- SubMenuStyleCollection.cs
- SoapMessage.cs
- SqlConnectionFactory.cs
- DiscriminatorMap.cs
- EntitySetDataBindingList.cs
- ManipulationStartingEventArgs.cs
- Attributes.cs
- KeyboardEventArgs.cs
- CodeRegionDirective.cs
- AssociationTypeEmitter.cs
- ParallelTimeline.cs
- XmlSerializerFactory.cs
- UnitySerializationHolder.cs
- Binding.cs
- KeyedCollection.cs
- Delegate.cs
- StrongNameMembershipCondition.cs
- WindowsListView.cs
- XmlCompatibilityReader.cs
- HyperLinkDataBindingHandler.cs
- CustomTypeDescriptor.cs
- Canonicalizers.cs
- userdatakeys.cs
- DbConnectionClosed.cs