Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / MsiStyleLogWriter.cs / 1 / MsiStyleLogWriter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using System.Diagnostics; using System.Globalization; using System.IO; class MsiStyleLogWriter { string logEntryPrefix; bool logFailureReported; StreamWriter logWriter; MsiStyleLogWriter(string logEntryPrefix) { this.logEntryPrefix = logEntryPrefix; string tempPath = Path.GetTempPath(); Random random = new Random(); int attempts = 0; const int maxAttempts = 20; Exception lastException = null; do { string logFileName = string.Format(CultureInfo.InvariantCulture, "dd_wcf_retCA{0:X}.txt", random.Next(short.MaxValue)); string logFilePath = Path.Combine(tempPath, logFileName); try { Stream logFileStream = new FileStream(logFilePath, FileMode.CreateNew, FileAccess.Write, FileShare.Read); this.logWriter = new StreamWriter(logFileStream); } catch (DirectoryNotFoundException exception) { EventLogger.LogWarning(SR.GetString(SR.ErrorCreatingMsiStyleLogFile, logFilePath, exception), false); return; } catch (PathTooLongException exception) { EventLogger.LogWarning(SR.GetString(SR.ErrorCreatingMsiStyleLogFile, logFilePath, exception), false); return; } catch (IOException exception) { // The current logFilePath may already exists; try again. lastException = exception; } } while ((this.logWriter == null) && (++attempts < maxAttempts)); if (this.logWriter == null) { EventLogger.LogWarning(SR.GetString(SR.ErrorCreatingMsiStyleLogFile, tempPath, lastException), false); this.logWriter = StreamWriter.Null; } } public static MsiStyleLogWriter CreateWriter() { string mainModuleFilePath = Process.GetCurrentProcess().MainModule.FileName; string logEntryPrefix = Path.GetFileNameWithoutExtension(mainModuleFilePath); MsiStyleLogWriter writer = new MsiStyleLogWriter(logEntryPrefix); DateTime now = DateTime.Now; writer.WriteRaw(SR.GetString(SR.MsiStyleLogBanner, now.ToShortDateString(), now.ToString("HH:mm:ss", CultureInfo.CurrentCulture), mainModuleFilePath)); return writer; } public void WriteEntry(string message) { this.WriteRaw(string.Format(CultureInfo.InvariantCulture, "{0} [{1}]: {2}", this.logEntryPrefix, DateTime.Now.ToString("HH:mm:ss:fff", CultureInfo.CurrentCulture), message)); } public void WriteRaw(string message) { try { this.logWriter.WriteLine(message); this.logWriter.Flush(); } catch (IOException exception) { if (!this.logFailureReported) { EventLogger.WriteLogEntry(SR.GetString(SR.ErrorWritingMsiStyleLogEntry, exception), EventLogEntryType.Warning); this.logFailureReported = true; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
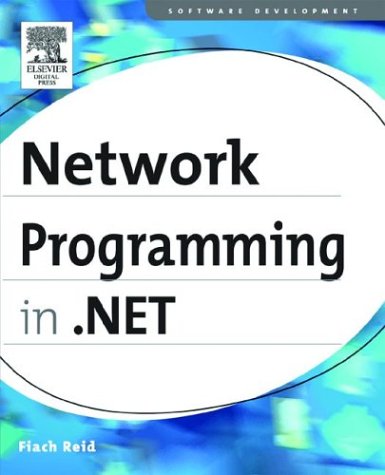
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonPopupAdapter.cs
- XmlNavigatorFilter.cs
- Symbol.cs
- DataGridViewControlCollection.cs
- SqlMethodCallConverter.cs
- DataViewSettingCollection.cs
- Mapping.cs
- ToolTip.cs
- ReadOnlyDictionary.cs
- UnhandledExceptionEventArgs.cs
- StaticContext.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- ClientEventManager.cs
- SerializationSectionGroup.cs
- PropagatorResult.cs
- DataControlPagerLinkButton.cs
- Timeline.cs
- _SslStream.cs
- GACMembershipCondition.cs
- thaishape.cs
- KeyFrames.cs
- ScriptControlDescriptor.cs
- DropDownButton.cs
- ChildTable.cs
- HttpsTransportBindingElement.cs
- SqlCommandBuilder.cs
- MatrixCamera.cs
- TemplateEditingService.cs
- UnaryQueryOperator.cs
- _FtpControlStream.cs
- InputLangChangeEvent.cs
- WebSysDescriptionAttribute.cs
- TypeInfo.cs
- AspProxy.cs
- ToolStripManager.cs
- CodeRegionDirective.cs
- EventlogProvider.cs
- InstanceStore.cs
- WebBrowser.cs
- SqlDataAdapter.cs
- RemotingConfigParser.cs
- CallbackBehaviorAttribute.cs
- XmlComment.cs
- TrackingServices.cs
- ResourcePermissionBase.cs
- RequiredAttributeAttribute.cs
- RolePrincipal.cs
- SafeSecurityHelper.cs
- ActivityDesigner.cs
- AsyncPostBackErrorEventArgs.cs
- Light.cs
- CodeEventReferenceExpression.cs
- DataStreams.cs
- SymbolUsageManager.cs
- ItemsChangedEventArgs.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- SqlSupersetValidator.cs
- SharedConnectionListener.cs
- HybridWebProxyFinder.cs
- Matrix3DValueSerializer.cs
- OleDbPropertySetGuid.cs
- ExtentCqlBlock.cs
- NextPreviousPagerField.cs
- CreateRefExpr.cs
- MulticastDelegate.cs
- Brush.cs
- RoleManagerSection.cs
- ChtmlMobileTextWriter.cs
- WebControl.cs
- ScriptManager.cs
- VectorAnimationUsingKeyFrames.cs
- XmlNamespaceDeclarationsAttribute.cs
- RegexTree.cs
- SQLGuidStorage.cs
- BaseTreeIterator.cs
- AssemblyHelper.cs
- ToolStripDesignerUtils.cs
- ServicePointManagerElement.cs
- OptimalBreakSession.cs
- ExtendedPropertyCollection.cs
- AsyncDataRequest.cs
- PersonalizationEntry.cs
- InternalDispatchObject.cs
- Pkcs7Recipient.cs
- StreamResourceInfo.cs
- ParentQuery.cs
- BuildProviderAppliesToAttribute.cs
- ConnectorSelectionGlyph.cs
- BufferBuilder.cs
- FontFamilyIdentifier.cs
- BitVector32.cs
- FlatButtonAppearance.cs
- TemplateBindingExpression.cs
- DataGridToolTip.cs
- XmlReflectionMember.cs
- HttpPostedFile.cs
- TextEditorTyping.cs
- DataServiceClientException.cs
- OletxDependentTransaction.cs
- WebPartZoneDesigner.cs