Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / System.Runtime.DurableInstancing / System / Runtime / Collections / OrderedDictionary.cs / 1305376 / OrderedDictionary.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime.Collections { using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; // System.Collections.Specialized.OrderedDictionary is NOT generic. // This class is essentially a generic wrapper for OrderedDictionary. class OrderedDictionary: IDictionary , ICollection >, IEnumerable > { OrderedDictionary privateDictionary; public OrderedDictionary() { this.privateDictionary = new OrderedDictionary(); } public OrderedDictionary(IDictionary dictionary) { if (dictionary != null) { this.privateDictionary = new OrderedDictionary(); foreach (KeyValuePair pair in dictionary) { this.privateDictionary.Add(pair.Key, pair.Value); } } } public int Count { get { return this.privateDictionary.Count; } } public bool IsReadOnly { get { return false; } } public TValue this[TKey key] { get { if (key == null) { throw Fx.Exception.ArgumentNull("key"); } if (this.privateDictionary.Contains(key)) { return (TValue)this.privateDictionary[(object)key]; } else { throw Fx.Exception.AsError(new KeyNotFoundException(SRCore.KeyNotFoundInDictionary)); } } set { if (key == null) { throw Fx.Exception.ArgumentNull("key"); } this.privateDictionary[(object)key] = value; } } public ICollection Keys { get { List keys = new List (this.privateDictionary.Count); foreach (TKey key in this.privateDictionary.Keys) { keys.Add(key); } // Keys should be put in a ReadOnlyCollection, // but since this is an internal class, for performance reasons, // we choose to avoid creating yet another collection. return keys; } } public ICollection Values { get { List values = new List (this.privateDictionary.Count); foreach (TValue value in this.privateDictionary.Values) { values.Add(value); } // Values should be put in a ReadOnlyCollection, // but since this is an internal class, for performance reasons, // we choose to avoid creating yet another collection. return values; } } public void Add(KeyValuePair item) { Add(item.Key, item.Value); } public void Add(TKey key, TValue value) { if (key == null) { throw Fx.Exception.ArgumentNull("key"); } this.privateDictionary.Add(key, value); } public void Clear() { this.privateDictionary.Clear(); } public bool Contains(KeyValuePair item) { if (item.Key == null || !this.privateDictionary.Contains(item.Key)) { return false; } else { return this.privateDictionary[(object)item.Key].Equals(item.Value); } } public bool ContainsKey(TKey key) { if (key == null) { throw Fx.Exception.ArgumentNull("key"); } return this.privateDictionary.Contains(key); } public void CopyTo(KeyValuePair [] array, int arrayIndex) { if (array == null) { throw Fx.Exception.ArgumentNull("array"); } if (arrayIndex < 0) { throw Fx.Exception.AsError(new ArgumentOutOfRangeException("arrayIndex")); } if (array.Rank > 1 || arrayIndex >= array.Length || array.Length - arrayIndex < this.privateDictionary.Count) { throw Fx.Exception.Argument("array", SRCore.BadCopyToArray); } int index = arrayIndex; foreach (DictionaryEntry entry in this.privateDictionary) { array[index] = new KeyValuePair ((TKey)entry.Key, (TValue)entry.Value); index++; } } public IEnumerator > GetEnumerator() { foreach (DictionaryEntry entry in this.privateDictionary) { yield return new KeyValuePair ((TKey)entry.Key, (TValue)entry.Value); } } IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } public bool Remove(KeyValuePair item) { if (Contains(item)) { this.privateDictionary.Remove(item.Key); return true; } else { return false; } } public bool Remove(TKey key) { if (key == null) { throw Fx.Exception.ArgumentNull("key"); } if (this.privateDictionary.Contains(key)) { this.privateDictionary.Remove(key); return true; } else { return false; } } public bool TryGetValue(TKey key, out TValue value) { if (key == null) { throw Fx.Exception.ArgumentNull("key"); } bool keyExists = this.privateDictionary.Contains(key); value = keyExists ? (TValue)this.privateDictionary[(object)key] : default(TValue); return keyExists; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
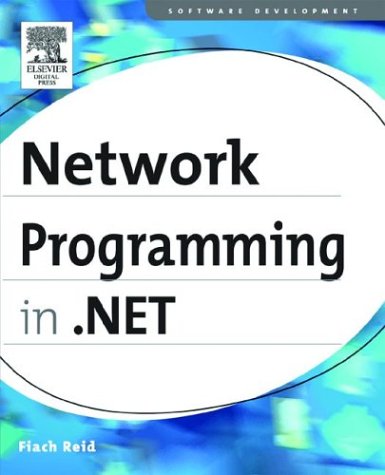
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FieldAccessException.cs
- ChannelManager.cs
- MeshGeometry3D.cs
- BindingNavigator.cs
- ManipulationInertiaStartingEventArgs.cs
- XmlAnyAttributeAttribute.cs
- ConfigurationErrorsException.cs
- EventData.cs
- SvcMapFileLoader.cs
- wgx_exports.cs
- DisposableCollectionWrapper.cs
- CultureInfoConverter.cs
- SoapFault.cs
- AttributeEmitter.cs
- GeometryConverter.cs
- DataGridPagerStyle.cs
- ColorMap.cs
- RemotingClientProxy.cs
- StylusCollection.cs
- DataBindingList.cs
- RequestStatusBarUpdateEventArgs.cs
- DetailsViewDeletedEventArgs.cs
- MailDefinition.cs
- QuaternionAnimationBase.cs
- EncodingTable.cs
- InputMethod.cs
- DetailsViewUpdateEventArgs.cs
- MetadataHelper.cs
- ScriptingProfileServiceSection.cs
- RequiredArgumentAttribute.cs
- COM2Enum.cs
- ResourceCategoryAttribute.cs
- DataBindingHandlerAttribute.cs
- Transform.cs
- InheritanceAttribute.cs
- BmpBitmapEncoder.cs
- PropertyItemInternal.cs
- ReaderWriterLockSlim.cs
- Journal.cs
- EditCommandColumn.cs
- XpsResourceDictionary.cs
- EFTableProvider.cs
- ThumbAutomationPeer.cs
- Typography.cs
- AttachedPropertiesService.cs
- LogExtent.cs
- contentDescriptor.cs
- PanelDesigner.cs
- ErrorRuntimeConfig.cs
- Vector.cs
- SerializationStore.cs
- ToolstripProfessionalRenderer.cs
- ContextMenu.cs
- XPathParser.cs
- Faults.cs
- TrackingAnnotationCollection.cs
- DataBoundControl.cs
- SQLInt64Storage.cs
- Binding.cs
- HtmlTableRow.cs
- TemplatePropertyEntry.cs
- Pkcs7Recipient.cs
- MDIControlStrip.cs
- ArgumentOutOfRangeException.cs
- EmptyImpersonationContext.cs
- FileUtil.cs
- FrameworkReadOnlyPropertyMetadata.cs
- WindowPattern.cs
- TextDecorations.cs
- WebPartConnectionsEventArgs.cs
- ScriptingProfileServiceSection.cs
- XmlAnyAttributeAttribute.cs
- GPRECT.cs
- DiagnosticSection.cs
- PanelDesigner.cs
- GenericRootAutomationPeer.cs
- TextBoxView.cs
- Ipv6Element.cs
- WebBrowserUriTypeConverter.cs
- XamlTreeBuilder.cs
- BrowserTree.cs
- Rect3DValueSerializer.cs
- TableRowGroup.cs
- StringKeyFrameCollection.cs
- WebBrowserHelper.cs
- RuntimeConfigLKG.cs
- TempEnvironment.cs
- PictureBox.cs
- Utility.cs
- InertiaRotationBehavior.cs
- CaretElement.cs
- TextReader.cs
- ActivityBindForm.cs
- ComplusTypeValidator.cs
- HtmlFormParameterReader.cs
- StaticExtensionConverter.cs
- ActiveXContainer.cs
- GatewayDefinition.cs
- RemoteDebugger.cs
- HttpFileCollection.cs