Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Hosting / WorkflowRuntimeService.cs / 1305376 / WorkflowRuntimeService.cs
//Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Globalization; using System.Workflow.Runtime; namespace System.Workflow.Runtime.Hosting { public enum WorkflowRuntimeServiceState { Stopped, Starting, Started, Stopping } abstract public class WorkflowRuntimeService { private WorkflowRuntime _runtime; private WorkflowRuntimeServiceState state = WorkflowRuntimeServiceState.Stopped; protected WorkflowRuntime Runtime { get { return _runtime; } } internal void SetRuntime(WorkflowRuntime runtime) { if (runtime == null && _runtime != null) { _runtime.Started -= this.HandleStarted; _runtime.Stopped -= this.HandleStopped; } _runtime = runtime; if (runtime != null) { _runtime.Started += this.HandleStarted; _runtime.Stopped += this.HandleStopped; } } protected void RaiseServicesExceptionNotHandledEvent(Exception exception, Guid instanceId) { Runtime.RaiseServicesExceptionNotHandledEvent(exception, instanceId); } internal void RaiseExceptionNotHandledEvent(Exception exception, Guid instanceId) { Runtime.RaiseServicesExceptionNotHandledEvent(exception, instanceId); } protected WorkflowRuntimeServiceState State { get { return state; } } virtual internal protected void Start() { if (_runtime == null) throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, ExecutionStringManager.ServiceNotAddedToRuntime, this.GetType().Name)); if (state.Equals(WorkflowRuntimeServiceState.Started)) throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, ExecutionStringManager.ServiceAlreadyStarted, this.GetType().Name)); state = WorkflowRuntimeServiceState.Starting; } virtual internal protected void Stop() { if (_runtime == null) throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, ExecutionStringManager.ServiceNotAddedToRuntime, this.GetType().Name)); if (state.Equals(WorkflowRuntimeServiceState.Stopped)) throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, ExecutionStringManager.ServiceNotStarted, this.GetType().Name)); state = WorkflowRuntimeServiceState.Stopping; } virtual protected void OnStarted() {} virtual protected void OnStopped() {} private void HandleStarted(object source, WorkflowRuntimeEventArgs e) { state = WorkflowRuntimeServiceState.Started; this.OnStarted(); } private void HandleStopped(object source, WorkflowRuntimeEventArgs e) { state = WorkflowRuntimeServiceState.Stopped; this.OnStopped(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
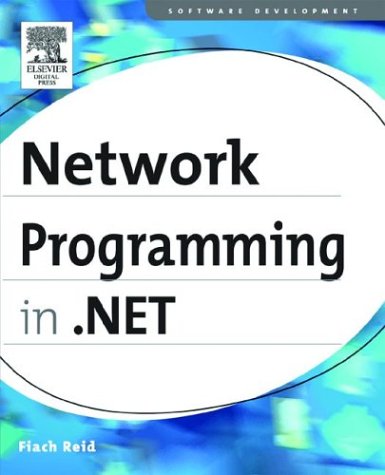
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ControlBindingsCollection.cs
- DateTimeOffsetConverter.cs
- WebServiceClientProxyGenerator.cs
- ConfigXmlElement.cs
- ChannelBuilder.cs
- ToolStripItem.cs
- While.cs
- WebPartZone.cs
- CodeSnippetExpression.cs
- CodeTypeMemberCollection.cs
- NotFiniteNumberException.cs
- VisualStyleTypesAndProperties.cs
- WebPartConnection.cs
- ConfigurationFileMap.cs
- TextBoxAutoCompleteSourceConverter.cs
- StorageComplexPropertyMapping.cs
- Gdiplus.cs
- XmlLinkedNode.cs
- JoinQueryOperator.cs
- CacheDict.cs
- ComboBoxAutomationPeer.cs
- PersistencePipeline.cs
- SmiContextFactory.cs
- SafeReversePInvokeHandle.cs
- SocketException.cs
- WrappedIUnknown.cs
- ApplyTemplatesAction.cs
- MobileCategoryAttribute.cs
- SiteMembershipCondition.cs
- TimeSpanParse.cs
- ServiceChannelManager.cs
- XmlIncludeAttribute.cs
- SmiEventSink_DeferedProcessing.cs
- Error.cs
- HttpConfigurationContext.cs
- Misc.cs
- XhtmlConformanceSection.cs
- EastAsianLunisolarCalendar.cs
- XsltContext.cs
- QueryAccessibilityHelpEvent.cs
- TimelineGroup.cs
- TextBoxBase.cs
- IsolationInterop.cs
- UpnEndpointIdentity.cs
- AutomationIdentifier.cs
- AppLevelCompilationSectionCache.cs
- ImageAnimator.cs
- CatalogPartCollection.cs
- SqlCacheDependency.cs
- AttributeAction.cs
- RegexStringValidator.cs
- OutputCacheSettingsSection.cs
- RangeContentEnumerator.cs
- BigInt.cs
- WebBaseEventKeyComparer.cs
- RecognizedPhrase.cs
- ObjectDataSource.cs
- SystemIPGlobalProperties.cs
- AnnotationHelper.cs
- HtmlAnchor.cs
- CodeTypeDeclaration.cs
- AVElementHelper.cs
- SByte.cs
- Int32Rect.cs
- MenuAutomationPeer.cs
- IIS7UserPrincipal.cs
- SafeEventLogReadHandle.cs
- TypeUsageBuilder.cs
- ContractUtils.cs
- Hex.cs
- StreamWithDictionary.cs
- ContactManager.cs
- SoapClientMessage.cs
- UnknownBitmapEncoder.cs
- MediaElement.cs
- XsltArgumentList.cs
- VariantWrapper.cs
- TextEditorParagraphs.cs
- HttpChannelHelper.cs
- ActivityWithResultWrapper.cs
- DBCSCodePageEncoding.cs
- PreservationFileWriter.cs
- XmlCompatibilityReader.cs
- RepeatInfo.cs
- NameTable.cs
- DataMemberAttribute.cs
- GeometryModel3D.cs
- UserUseLicenseDictionaryLoader.cs
- BulletChrome.cs
- UTF32Encoding.cs
- XPathSelfQuery.cs
- IApplicationTrustManager.cs
- EventWaitHandle.cs
- X509Extension.cs
- MsmqIntegrationMessageProperty.cs
- SqlCacheDependencyDatabase.cs
- TableItemStyle.cs
- TemplateColumn.cs
- RenderingEventArgs.cs
- TokenBasedSetEnumerator.cs