Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Runtime / Versioning / MultitargetingHelpers.cs / 1305376 / MultitargetingHelpers.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: MultitargetingHelpers ** **[....] ** ** ** Purpose: Central repository for helpers supporting ** multitargeting, such as emitting the correct version numbers ** and assembly names. ** ** ===========================================================*/ namespace System.Runtime.Versioning { using System; using System.IO; using System.Text; using System.Diagnostics.Contracts; internal static class MultitargetingHelpers { // default type converter private static FuncdefaultConverter = (t) => t.AssemblyQualifiedName; // This method gets assembly info for the corresponding type. If the typeConverter // is provided it is used to get this information. internal static string GetAssemblyQualifiedName(Type type, Func converter) { string assemblyFullName = null; if (type != null) { if (converter != null) { try { assemblyFullName = converter(type); // } catch (Exception e) { if (IsSecurityOrCriticalException(e)) { throw; } } } if (assemblyFullName == null) { assemblyFullName = defaultConverter(type); } } return assemblyFullName; } private static bool IsCriticalException(Exception ex) { return ex is NullReferenceException || ex is StackOverflowException || ex is OutOfMemoryException || ex is System.Threading.ThreadAbortException || ex is IndexOutOfRangeException || ex is AccessViolationException; } private static bool IsSecurityOrCriticalException(Exception ex) { return (ex is System.Security.SecurityException) || IsCriticalException(ex); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
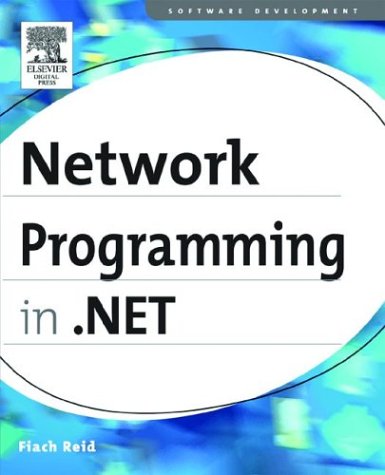
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripPanel.cs
- NamespaceQuery.cs
- WebPartCloseVerb.cs
- RemotingException.cs
- BitmapEffectrendercontext.cs
- EndPoint.cs
- SmiRecordBuffer.cs
- XPathAncestorIterator.cs
- DecoderNLS.cs
- DurableRuntimeValidator.cs
- SecurityState.cs
- AudioFormatConverter.cs
- EventProxy.cs
- UnmanagedHandle.cs
- MenuBase.cs
- TableProviderWrapper.cs
- ListenerConnectionDemuxer.cs
- Quaternion.cs
- EncryptionUtility.cs
- CopyNodeSetAction.cs
- SQLBinaryStorage.cs
- CancellationTokenSource.cs
- DependencyPropertyAttribute.cs
- DetailsViewModeEventArgs.cs
- RootBuilder.cs
- PackageProperties.cs
- StackBuilderSink.cs
- LicenseProviderAttribute.cs
- _StreamFramer.cs
- ListViewGroup.cs
- SignedXml.cs
- ResizeGrip.cs
- ExpressionBindings.cs
- ObjectNavigationPropertyMapping.cs
- UserNameSecurityTokenAuthenticator.cs
- PEFileEvidenceFactory.cs
- ZoneMembershipCondition.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- QilTypeChecker.cs
- CqlErrorHelper.cs
- MarshalDirectiveException.cs
- ProtectedConfiguration.cs
- sqlnorm.cs
- OuterGlowBitmapEffect.cs
- QualifiedId.cs
- PackageDigitalSignatureManager.cs
- HttpAsyncResult.cs
- DataGridHeaderBorder.cs
- ParserContext.cs
- NegatedCellConstant.cs
- keycontainerpermission.cs
- DeclarationUpdate.cs
- ISAPIWorkerRequest.cs
- _HeaderInfo.cs
- OrderablePartitioner.cs
- MediaContextNotificationWindow.cs
- StateChangeEvent.cs
- TextOptions.cs
- SQLBytesStorage.cs
- HeaderedItemsControl.cs
- _emptywebproxy.cs
- GC.cs
- ScriptingJsonSerializationSection.cs
- WebServiceParameterData.cs
- UInt32Storage.cs
- DataGridTextBox.cs
- AlphabetConverter.cs
- SettingsPropertyCollection.cs
- _AutoWebProxyScriptWrapper.cs
- SizeFConverter.cs
- SwitchElementsCollection.cs
- ToolStripScrollButton.cs
- ZipIOExtraFieldElement.cs
- Property.cs
- Polygon.cs
- BoolExpr.cs
- SettingsPropertyValueCollection.cs
- FileDetails.cs
- DataComponentMethodGenerator.cs
- ReflectionUtil.cs
- XmlNode.cs
- ShapeTypeface.cs
- HostnameComparisonMode.cs
- LookupNode.cs
- SQLBinaryStorage.cs
- BaseParagraph.cs
- BaseProcessor.cs
- WizardSideBarListControlItemEventArgs.cs
- TrailingSpaceComparer.cs
- WindowsToolbar.cs
- ObjectDataSourceEventArgs.cs
- SignatureHelper.cs
- RawKeyboardInputReport.cs
- ToolStripPanelRow.cs
- SoapFault.cs
- objectquery_tresulttype.cs
- XmlSchemaSequence.cs
- ImmutablePropertyDescriptorGridEntry.cs
- PropertyDescriptor.cs
- MatrixAnimationBase.cs