Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Remoting / Channels / IPC / PortCache.cs / 1305376 / PortCache.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //============================================================================ // File: PortCache.cs // Author: [....]@Microsoft.Com // Summary: Implements a cache for port handles // //========================================================================= using System; using System.Collections; using System.Threading; using System.Security.Principal; namespace System.Runtime.Remoting.Channels.Ipc { internal class PortConnection { private IpcPort _port; private DateTime _socketLastUsed; internal PortConnection(IpcPort port) { _port = port; _socketLastUsed = DateTime.Now; } internal IpcPort Port { get { return _port; } } internal DateTime LastUsed { get { return _socketLastUsed; } } } internal class ConnectionCache { // collection of RemoteConnection's. private static Hashtable _connections = new Hashtable(); // socket timeout data private static RegisteredWaitHandle _registeredWaitHandle; private static WaitOrTimerCallback _socketTimeoutDelegate; private static AutoResetEvent _socketTimeoutWaitHandle; private static TimeSpan _socketTimeoutPollTime = TimeSpan.FromSeconds(10); private static TimeSpan _portLifetime = TimeSpan.FromSeconds(10); static ConnectionCache() { InitializeConnectionTimeoutHandler(); } private static void InitializeConnectionTimeoutHandler() { _socketTimeoutDelegate = new WaitOrTimerCallback(TimeoutConnections); _socketTimeoutWaitHandle = new AutoResetEvent(false); _registeredWaitHandle = ThreadPool.UnsafeRegisterWaitForSingleObject( _socketTimeoutWaitHandle, _socketTimeoutDelegate, "IpcConnectionTimeout", _socketTimeoutPollTime, true); // execute only once } // InitializeSocketTimeoutHandler private static void TimeoutConnections(Object state, Boolean wasSignalled) { DateTime currentTime = DateTime.UtcNow; lock (_connections) { foreach (DictionaryEntry entry in _connections) { PortConnection connection = (PortConnection)entry.Value; if (DateTime.Now - connection.LastUsed > _portLifetime) connection.Port.Dispose(); } } _registeredWaitHandle.Unregister(null); _registeredWaitHandle = ThreadPool.UnsafeRegisterWaitForSingleObject( _socketTimeoutWaitHandle, _socketTimeoutDelegate, "IpcConnectionTimeout", _socketTimeoutPollTime, true); // execute only once } // TimeoutConnections // The key is expected to of the form portName public IpcPort GetConnection(String portName, bool secure, TokenImpersonationLevel level, int timeout) { PortConnection connection = null; lock (_connections) { bool cacheable = true; if (secure) { try { WindowsIdentity currentId = WindowsIdentity.GetCurrent(true/*ifImpersonating*/); if (currentId != null) { cacheable = false; currentId.Dispose(); } } catch(Exception) { cacheable = false; } } if (cacheable) { connection = (PortConnection)_connections[portName]; } if (connection == null || connection.Port.IsDisposed) { connection = new PortConnection(IpcPort.Connect(portName, secure, level, timeout)); connection.Port.Cacheable = cacheable; } else { // Remove the connection from the cache _connections.Remove(portName); } } return connection.Port; } // GetSocket public void ReleaseConnection(IpcPort port) { string portName = port.Name; PortConnection connection = (PortConnection)_connections[portName]; if (port.Cacheable && (connection == null || connection.Port.IsDisposed)) { lock(_connections) { _connections[portName] = new PortConnection(port); } } else { // there should have been a connection, so let's just close // this socket. port.Dispose(); } } // ReleasePort } // ConnectionCache } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
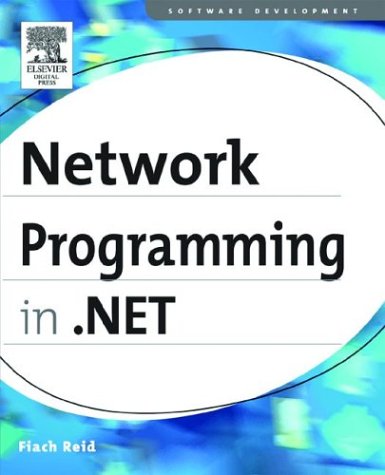
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DialogBaseForm.cs
- HttpGetServerProtocol.cs
- SmtpDigestAuthenticationModule.cs
- MasterPageBuildProvider.cs
- AppDomainAttributes.cs
- PageOrientation.cs
- SizeChangedInfo.cs
- PerfCounterSection.cs
- SchemaElement.cs
- Link.cs
- MapPathBasedVirtualPathProvider.cs
- Vector3DConverter.cs
- SecurityState.cs
- ToolStripDropDownMenu.cs
- WinFormsSecurity.cs
- UserControlBuildProvider.cs
- RectAnimationBase.cs
- BindingsSection.cs
- WebPartEventArgs.cs
- SpeechSynthesizer.cs
- WebPartConnectionsConnectVerb.cs
- MappingSource.cs
- BasicCommandTreeVisitor.cs
- ToolStripDropDownItem.cs
- ReadOnlyTernaryTree.cs
- EntityKeyElement.cs
- XPathArrayIterator.cs
- StringFreezingAttribute.cs
- DataGridViewAccessibleObject.cs
- ChannelSinkStacks.cs
- ObjectCacheSettings.cs
- GcSettings.cs
- MissingSatelliteAssemblyException.cs
- ToolBarButton.cs
- Animatable.cs
- DataControlPagerLinkButton.cs
- SettingsAttributes.cs
- XmlUrlEditor.cs
- ApplicationGesture.cs
- BitmapFrameDecode.cs
- DocumentsTrace.cs
- _Connection.cs
- WriteableBitmap.cs
- DataSet.cs
- DragDeltaEventArgs.cs
- FtpWebRequest.cs
- CellConstantDomain.cs
- FrameworkContentElement.cs
- ReferenceEqualityComparer.cs
- DefaultValueConverter.cs
- ToolStripDropDownItem.cs
- DynamicActionMessageFilter.cs
- SurrogateChar.cs
- x509store.cs
- RequestQueryProcessor.cs
- RegexWorker.cs
- SqlWorkflowInstanceStore.cs
- PropertyMapper.cs
- HttpProxyTransportBindingElement.cs
- MessageDesigner.cs
- Geometry3D.cs
- GlyphRun.cs
- GlyphRunDrawing.cs
- CodeAccessSecurityEngine.cs
- CodeParameterDeclarationExpression.cs
- PointAnimationUsingKeyFrames.cs
- SerializationInfo.cs
- HoistedLocals.cs
- CodeParameterDeclarationExpression.cs
- WizardForm.cs
- TypeValidationEventArgs.cs
- __ConsoleStream.cs
- AsymmetricKeyExchangeDeformatter.cs
- EntityStoreSchemaGenerator.cs
- ProvidersHelper.cs
- DataServiceEntityAttribute.cs
- Models.cs
- DiscriminatorMap.cs
- File.cs
- DataGridViewCheckBoxCell.cs
- ColorBlend.cs
- MissingSatelliteAssemblyException.cs
- XmlWhitespace.cs
- Rotation3D.cs
- X509CertificateCollection.cs
- WebConfigurationFileMap.cs
- FontFaceLayoutInfo.cs
- RtfControlWordInfo.cs
- CompositeCollection.cs
- PrePostDescendentsWalker.cs
- FileDialog_Vista_Interop.cs
- ByteRangeDownloader.cs
- LoadedEvent.cs
- KeyedCollection.cs
- HttpValueCollection.cs
- DBCommandBuilder.cs
- ColumnResult.cs
- DatagridviewDisplayedBandsData.cs
- IisTraceWebEventProvider.cs
- TextDecorationUnitValidation.cs