Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / Diagnostics / TraceEventCache.cs / 1305376 / TraceEventCache.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Threading; using System.Security.Permissions; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.Versioning; namespace System.Diagnostics { public class TraceEventCache { private static int processId; private static string processName; private long timeStamp = -1; private DateTime dateTime = DateTime.MinValue; private string stackTrace = null; internal Guid ActivityId { get { return Trace.CorrelationManager.ActivityId; } } public string Callstack { get { if (stackTrace == null) stackTrace = Environment.StackTrace; else new EnvironmentPermission(PermissionState.Unrestricted).Demand(); return stackTrace; } } public Stack LogicalOperationStack { get { return Trace.CorrelationManager.LogicalOperationStack; } } public DateTime DateTime { get { if (dateTime == DateTime.MinValue) dateTime = DateTime.UtcNow; return dateTime; } } public int ProcessId { [ResourceExposure(ResourceScope.Process)] // Returns the current process's pid [ResourceConsumption(ResourceScope.Process)] get { return GetProcessId(); } } public string ThreadId { get { return GetThreadId().ToString(CultureInfo.InvariantCulture); } } public long Timestamp { get { if (timeStamp == -1) timeStamp = Stopwatch.GetTimestamp(); return timeStamp ; } } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] private static void InitProcessInfo() { // Demand unmanaged code permission new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); if (processName == null) { Process p = Process.GetCurrentProcess(); try { processId = p.Id; processName = p.ProcessName; } finally { p.Dispose(); } } } [ResourceExposure(ResourceScope.Process)] internal static int GetProcessId() { InitProcessInfo(); return processId; } internal static string GetProcessName() { InitProcessInfo(); return processName; } internal static int GetThreadId() { return Thread.CurrentThread.ManagedThreadId; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
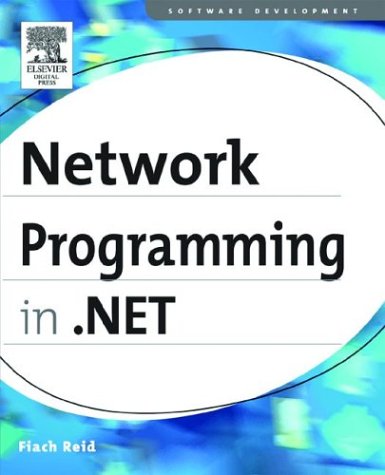
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventMap.cs
- CollectionsUtil.cs
- ProviderMetadata.cs
- NetworkStream.cs
- SvcMapFileSerializer.cs
- StringSource.cs
- AncestorChangedEventArgs.cs
- PathFigure.cs
- Hashtable.cs
- XmlIlTypeHelper.cs
- DesignSurfaceServiceContainer.cs
- OutputScopeManager.cs
- ConstraintStruct.cs
- ListViewTableCell.cs
- MsmqNonTransactedPoisonHandler.cs
- ClosureBinding.cs
- AxHost.cs
- Empty.cs
- ClientSession.cs
- EmbeddedMailObject.cs
- IfAction.cs
- MeasureItemEvent.cs
- BatchServiceHost.cs
- AsyncOperationManager.cs
- Sentence.cs
- PerfCounters.cs
- Activity.cs
- ManipulationStartingEventArgs.cs
- Pts.cs
- CodeConstructor.cs
- Rect3D.cs
- ModifierKeysConverter.cs
- FixedTextContainer.cs
- ContextMenuStrip.cs
- ToolStripHighContrastRenderer.cs
- RtfNavigator.cs
- HttpPostedFile.cs
- LinkUtilities.cs
- ChineseLunisolarCalendar.cs
- FixedPageStructure.cs
- MemberCollection.cs
- HttpContext.cs
- ComponentEditorForm.cs
- BaseDataListDesigner.cs
- OdbcUtils.cs
- ResolveNameEventArgs.cs
- DragStartedEventArgs.cs
- TrustSection.cs
- SchemaElementDecl.cs
- TrackingDataItem.cs
- Signature.cs
- ValidationEventArgs.cs
- HostingPreferredMapPath.cs
- entityreference_tresulttype.cs
- RichTextBoxAutomationPeer.cs
- EntryIndex.cs
- SHA512Managed.cs
- CompilationUtil.cs
- PerformanceCounterScope.cs
- NativeCompoundFileAPIs.cs
- TaskFormBase.cs
- OperandQuery.cs
- RegexRunnerFactory.cs
- CustomValidator.cs
- SQLString.cs
- ValidatingPropertiesEventArgs.cs
- WriterOutput.cs
- AnimatedTypeHelpers.cs
- formatter.cs
- CodeGen.cs
- LicFileLicenseProvider.cs
- WebPartHeaderCloseVerb.cs
- ObjectTokenCategory.cs
- AsyncOperationManager.cs
- SharedPersonalizationStateInfo.cs
- UserControlParser.cs
- PackagePartCollection.cs
- XmlSchemaAttribute.cs
- ObjectStateFormatter.cs
- Single.cs
- ControlTemplate.cs
- ConstrainedDataObject.cs
- XmlSchemaSimpleContentExtension.cs
- XdrBuilder.cs
- ZoomPercentageConverter.cs
- PrefixQName.cs
- SignerInfo.cs
- WinEventHandler.cs
- LocalizableAttribute.cs
- CompilerScopeManager.cs
- GridViewColumnHeader.cs
- XmlAttributeHolder.cs
- DoWorkEventArgs.cs
- DesignerDataSourceView.cs
- SQLUtility.cs
- ProtocolsConfigurationHandler.cs
- RenderDataDrawingContext.cs
- BufferedWebEventProvider.cs
- BeginGetFileNameFromUserRequest.cs
- RecipientInfo.cs