Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / JoinCqlBlock.cs / 1305376 / JoinCqlBlock.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Structures; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // This class corresponds to the various Join nodes in the view, i.e., // IJ, LOJ, FOJ internal class JoinCqlBlock : CqlBlock { #region Constructor // effects: Creates a Join CqlBlock (type given by opType) with // SELECT (slotinfos), FROM (children), ON (onClauses - one for each child except 0th), // WHERE (true), AS (blockAliasNum) internal JoinCqlBlock(CellTreeOpType opType, SlotInfo[] slotInfos, Listchildren, List onClauses, CqlIdentifiers identifiers, int blockAliasNum) : base(slotInfos, children, BoolExpression.True, identifiers, blockAliasNum) { m_opType = opType; m_onClauses = onClauses; } #endregion #region Fields private CellTreeOpType m_opType; // LOJ or IJ etc private List m_onClauses; #endregion #region Methods // effects: See CqlBlock.AsCql internal override StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel) { // The SELECT part GenerateProjectedtList(builder, indentLevel, CqlAlias, true); // The FROM part by joining all the children using ON Clauses builder.Append("FROM "); int i = 0; foreach (CqlBlock child in Children) { if (i > 0) { StringUtil.IndentNewLine(builder, indentLevel + 1); builder.Append(OpCellTreeNode.OpToCql(m_opType)); } builder.Append(" ("); child.AsCql(builder, false, indentLevel + 1); builder.Append(") AS ") .Append(child.CqlAlias); // The ON part if (i > 0) { StringUtil.IndentNewLine(builder, indentLevel + 1); builder.Append("ON "); m_onClauses[i - 1].AsCql(builder); } i++; } return builder; } #endregion // A class that represents "slot1 == slot2 AND "slot3 == slot4" ... // for two join blocks. That is, it is complete ON clause internal class OnClause : InternalBase { #region Constructor internal OnClause() { m_singleClauses = new List (); } #endregion #region Fields private List m_singleClauses; #endregion #region Methods // effects: Modified builder to contain a Cql string of the form "Slot1 = Slot2 AND // slot3 = slot4 AND ... Returns the builder internal StringBuilder AsCql(StringBuilder builder) { bool isFirst = true; foreach (SingleClause singleClause in m_singleClauses) { if (false == isFirst) { builder.Append(" AND "); } singleClause.AsCql(builder); isFirst = false; } return builder; } // effects: Adds an OnClause element for a join of the form "firstSlot = secondSlot" internal void Add(AliasedSlot firstSlot, AliasedSlot secondSlot) { SingleClause singleClause = new SingleClause(firstSlot, secondSlot); m_singleClauses.Add(singleClause); } internal override void ToCompactString(StringBuilder builder) { builder.Append("ON "); StringUtil.ToSeparatedString(builder, m_singleClauses, " AND "); } #endregion #region Struct // Denotes an expression between slots of the form: FirstSlot == SecondSlot private class SingleClause : InternalBase { internal SingleClause(AliasedSlot firstSlot, AliasedSlot secondSlot) { m_firstSlot = firstSlot; m_secondSlot = secondSlot; } #region Fields private AliasedSlot m_firstSlot; private AliasedSlot m_secondSlot; #endregion #region Methods // effects: Modifies builder to constain the Cql string of the form "firstSlot = secondSlot" // Returns the modified builder internal StringBuilder AsCql(StringBuilder builder) { builder.Append(m_firstSlot.FullCqlAlias()) .Append(" = ") .Append(m_secondSlot.FullCqlAlias()); return builder; } internal override void ToCompactString(StringBuilder builder) { m_firstSlot.ToCompactString(builder); builder.Append(" = "); m_secondSlot.ToCompactString(builder); } #endregion } #endregion } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
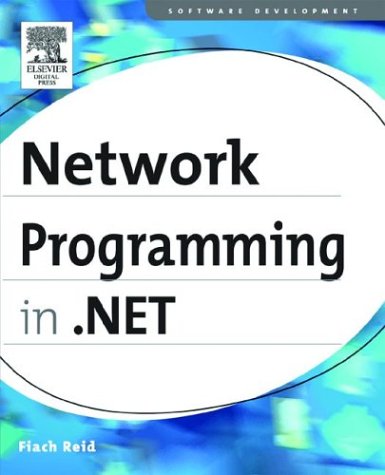
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComponentEvent.cs
- SystemColorTracker.cs
- FragmentQueryKB.cs
- MediaTimeline.cs
- InputScope.cs
- ScriptRef.cs
- EncryptedXml.cs
- ComMethodElement.cs
- HttpHandlerActionCollection.cs
- XamlSerializer.cs
- Win32Native.cs
- DataGridTable.cs
- WorkflowServiceInstance.cs
- WorkflowView.cs
- Literal.cs
- ProtocolsSection.cs
- RequiredFieldValidator.cs
- OracleCommandSet.cs
- CatalogZoneBase.cs
- TypefaceMetricsCache.cs
- LayoutTable.cs
- MailDefinition.cs
- TailCallAnalyzer.cs
- DBConnection.cs
- AuthenticationSection.cs
- HMACSHA384.cs
- ErrorHandler.cs
- TreeViewItem.cs
- HuffCodec.cs
- TableItemStyle.cs
- RotateTransform3D.cs
- WebConfigurationManager.cs
- DynamicMetaObject.cs
- SelectionEditingBehavior.cs
- AuthenticatedStream.cs
- ControlBindingsCollection.cs
- _SingleItemRequestCache.cs
- MULTI_QI.cs
- TeredoHelper.cs
- PageParserFilter.cs
- CharacterBufferReference.cs
- MessageSmuggler.cs
- ComplexTypeEmitter.cs
- Sql8ExpressionRewriter.cs
- AudioFileOut.cs
- XPathConvert.cs
- FrameworkPropertyMetadata.cs
- ReadContentAsBinaryHelper.cs
- ListMarkerSourceInfo.cs
- CodeActivity.cs
- SingleAnimationUsingKeyFrames.cs
- Endpoint.cs
- WebHttpSecurityElement.cs
- AttachedPropertiesService.cs
- DataGridViewComboBoxEditingControl.cs
- TextSpanModifier.cs
- ZoneLinkButton.cs
- ProjectionPathBuilder.cs
- CollectionViewGroupRoot.cs
- ComponentCache.cs
- StylusButton.cs
- MatchAllMessageFilter.cs
- TextTreeRootTextBlock.cs
- ConfigXmlComment.cs
- XmlSchemaValidationException.cs
- basecomparevalidator.cs
- ADMembershipUser.cs
- PackageFilter.cs
- XmlSchemaFacet.cs
- HashCodeCombiner.cs
- XmlSchemaSequence.cs
- XmlNullResolver.cs
- TabRenderer.cs
- SecurityTokenResolver.cs
- UInt16Converter.cs
- COM2PictureConverter.cs
- ResourceDescriptionAttribute.cs
- EdmEntityTypeAttribute.cs
- ShapingWorkspace.cs
- KeyboardNavigation.cs
- EventSinkHelperWriter.cs
- FormClosedEvent.cs
- CqlGenerator.cs
- MessageAction.cs
- AsnEncodedData.cs
- BindingNavigator.cs
- ProtocolsConfiguration.cs
- LogWriteRestartAreaState.cs
- FileInfo.cs
- ObjectMemberMapping.cs
- DbInsertCommandTree.cs
- ToolBar.cs
- HtmlAnchor.cs
- DebugView.cs
- DefaultHttpHandler.cs
- EntityDataSourceValidationException.cs
- WeakReferenceList.cs
- BitSet.cs
- DataBoundControlActionList.cs
- X509ImageLogo.cs