Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StorageMappingFragment.cs / 1305376 / StorageMappingFragment.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Represents the metadata for mapping fragment. /// A set of mapping fragments makes up the Set mappings( EntitySet, AssociationSet or CompositionSet ) /// Each MappingFragment provides mapping for those properties of a type that map to a single table. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ComplexPropertyMap /// --ComplexTypeMapping /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarProperyMap ( CMemberMetadata-->SMemberMetadata ) /// --DiscriminatorProperyMap ( constant value-->SMemberMetadata ) /// --ComplexTypeMapping /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarProperyMap ( CMemberMetadata-->SMemberMetadata ) /// --DiscriminatorProperyMap ( constant value-->SMemberMetadata ) /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarProperyMap ( CMemberMetadata-->SMemberMetadata ) /// --EndPropertyMap /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --EntityKey /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarPropertyMap ( CMemberMetadata-->Constant value ) /// --ComplexPropertyMap /// --ComplexTypeMapping /// --ScalarPropertyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarProperyMap ( CMemberMetadata-->SMemberMetadata ) /// --ScalarPropertyMap ( CMemberMetadata-->Constant value ) /// This class represents the metadata for all the mapping fragment elements in the /// above example. Users can access all the top level constructs of /// MappingFragment element like EntityKey map, Property Maps, Discriminator /// property through this mapping fragment class. /// internal class StorageMappingFragment { #region Constructors ////// Construct a new Mapping Fragment object /// /// /// internal StorageMappingFragment(EntitySet tableExtent, StorageTypeMapping typeMapping, bool distinctFlag) { Debug.Assert(tableExtent != null, "Table should not be null when constructing a Mapping Fragment"); Debug.Assert(typeMapping != null, "TypeMapping should not be null when constructing a Mapping Fragment"); this.m_tableExtent = tableExtent; this.m_typeMapping = typeMapping; this.m_isSQueryDistinct = distinctFlag; } #endregion #region Fields EntitySet m_tableExtent; //table extent from which the properties are mapped under this fragment StorageTypeMapping m_typeMapping; //Type mapping under which this mapping fragment exists Dictionarym_conditionProperties = new Dictionary (EqualityComparer .Default); //Condition property mappings for this mapping fragment List m_properties = new List (); //All the other properties int m_startLineNumber; //Line Number for MappingFragment element start tag int m_startLinePosition; //Line position for MappingFragment element start tag int m_endLineNumber; //Line Number for MappingFragment element end tag int m_endLinePosition; //Line position for MappingFragment element end tag bool m_isSQueryDistinct; #endregion #region Properties /// /// The table from which the properties are mapped in this fragment /// internal EntitySet TableSet { get { return this.m_tableExtent; } } internal bool IsSQueryDistinct { get { return m_isSQueryDistinct; } } ////// Returns all the property mappings defined in the complex type mapping /// including Properties and Condition Properties /// internal ReadOnlyCollectionAllProperties { get { List properties = new List (); properties.AddRange(m_properties); properties.AddRange(m_conditionProperties.Values); return properties.AsReadOnly(); } } /// /// Returns all the property mappings defined in the complex type mapping /// including Properties and Condition Properties /// internal ReadOnlyCollectionProperties { get { return m_properties.AsReadOnly(); } } ///// ///// The Extent Mapping that contains this Mapping Fragment. ///// //internal StorageSetMapping ExtentMapping { // get { // return this.m_typeMapping.SetMapping; // } //} ////////// The Type Mapping that contains this Mapping Fragment. ///// //internal StorageTypeMapping TypeMapping { // get { // return this.m_typeMapping; // } //} ////// Line Number in MSL file where the Mapping Fragment Element's Start Tag is present. /// internal int StartLineNumber { get { return m_startLineNumber; } set { m_startLineNumber = value; } } ////// Line Position in MSL file where the Mapping Fragment Element's Start Tag is present. /// internal int StartLinePosition { get { return m_startLinePosition; } set { m_startLinePosition = value; } } ////// Line Number in MSL file where the Mapping Fragment Element's End Tag is present. /// internal int EndLineNumber { //get { // return m_endLineNumber; //} set { m_endLineNumber = value; } } ////// Line Position in MSL file where the Mapping Fragment Element's End Tag is present. /// internal int EndLinePosition { //get { // return m_endLinePosition; //} set { m_endLinePosition = value; } } ////// File URI of the MSL file /// //This should not be stored on the Fragment. Probably it should go on schema. //But this requires some thinking before we can finally decide where it should go. internal string SourceLocation { get { return this.m_typeMapping.SetMapping.EntityContainerMapping.SourceLocation; } } #endregion #region Methods ////// Add a property mapping as a child of this mapping fragment /// /// child property mapping to be added internal void AddProperty(StoragePropertyMapping prop) { this.m_properties.Add(prop); } ////// Add a condition property mapping as a child of this complex property mapping /// Condition Property Mapping specifies a Condition either on the C side property or S side property. /// /// The mapping that needs to be added internal bool AddConditionProperty(StorageConditionPropertyMapping conditionPropertyMap) { //Same Member can not have more than one Condition with in the //same Mapping Fragment. EdmProperty conditionMember = (conditionPropertyMap.EdmProperty != null) ? conditionPropertyMap.EdmProperty : conditionPropertyMap.ColumnProperty; Debug.Assert(conditionMember != null); if (m_conditionProperties.ContainsKey(conditionMember)) { return false; } m_conditionProperties.Add(conditionMember, conditionPropertyMap); return true; } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal virtual void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("MappingFragment"); sb.Append(" "); sb.Append("Table Name:"); sb.Append(this.m_tableExtent.Name); Console.WriteLine(sb.ToString()); foreach (StorageConditionPropertyMapping conditionMap in m_conditionProperties.Values) (conditionMap).Print(index + 5); foreach (StoragePropertyMapping propertyMapping in m_properties) { propertyMapping.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
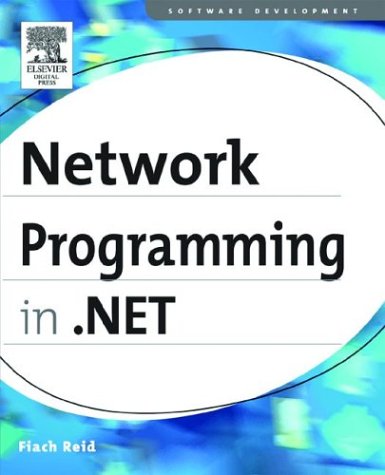
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileParameter.cs
- IndentedWriter.cs
- TextEditorMouse.cs
- WebPartAuthorizationEventArgs.cs
- ProgressPage.cs
- COM2TypeInfoProcessor.cs
- ColumnHeaderConverter.cs
- ConfigPathUtility.cs
- SoapEnumAttribute.cs
- SRGSCompiler.cs
- EntryPointNotFoundException.cs
- ImageField.cs
- Rect3D.cs
- SafePEFileHandle.cs
- StaticSiteMapProvider.cs
- Rotation3DAnimationBase.cs
- XamlStyleSerializer.cs
- NameTable.cs
- InfocardInteractiveChannelInitializer.cs
- SafeNativeMethods.cs
- HttpListenerContext.cs
- KnownTypesProvider.cs
- XmlDomTextWriter.cs
- CodeAttachEventStatement.cs
- XhtmlBasicObjectListAdapter.cs
- CapabilitiesState.cs
- NonPrimarySelectionGlyph.cs
- PerspectiveCamera.cs
- DataRowCollection.cs
- XmlSchemaCollection.cs
- QilXmlReader.cs
- MouseWheelEventArgs.cs
- GPRECT.cs
- TrueReadOnlyCollection.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- Panel.cs
- OlePropertyStructs.cs
- SocketInformation.cs
- MouseOverProperty.cs
- QilGenerator.cs
- WCFBuildProvider.cs
- DataGridViewTopLeftHeaderCell.cs
- TemplateInstanceAttribute.cs
- XmlAnyElementAttribute.cs
- datacache.cs
- KeyInterop.cs
- PerformanceCounterPermission.cs
- WindowsHyperlink.cs
- LogoValidationException.cs
- CommandHelper.cs
- WebServiceClientProxyGenerator.cs
- TreeNodeBindingCollection.cs
- ValidatedControlConverter.cs
- SmtpReplyReader.cs
- UIElement3D.cs
- StickyNoteAnnotations.cs
- ToolStripPanelSelectionBehavior.cs
- XmlAttributeCollection.cs
- GridLength.cs
- DataServiceHost.cs
- BorderGapMaskConverter.cs
- ComponentChangingEvent.cs
- IResourceProvider.cs
- AddInSegmentDirectoryNotFoundException.cs
- FreezableDefaultValueFactory.cs
- DbDataReader.cs
- ExtensibleClassFactory.cs
- ErrorFormatterPage.cs
- MailHeaderInfo.cs
- UndoEngine.cs
- CodeIdentifier.cs
- BoolExpressionVisitors.cs
- DataGridRow.cs
- SingleStorage.cs
- MenuRendererStandards.cs
- Vector.cs
- DataControlExtensions.cs
- dbenumerator.cs
- PropertyPathWorker.cs
- AbandonedMutexException.cs
- XamlStackWriter.cs
- SystemIPInterfaceStatistics.cs
- DrawingBrush.cs
- ObjectPersistData.cs
- AnnouncementDispatcherAsyncResult.cs
- ResXBuildProvider.cs
- CodeDomSerializer.cs
- QilStrConcat.cs
- Model3D.cs
- WindowsGraphicsCacheManager.cs
- PointLight.cs
- CellParaClient.cs
- FormsAuthenticationEventArgs.cs
- HashSetEqualityComparer.cs
- UserPreferenceChangedEventArgs.cs
- DSASignatureDeformatter.cs
- XmlSchemas.cs
- LockCookie.cs
- OracleConnectionStringBuilder.cs
- GraphicsState.cs