Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Design / PhoneCallDesigner.cs / 1305376 / PhoneCallDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.IO; using System.Web.UI; using System.Web.UI.Design; using System.Web.UI.MobileControls.Adapters; using System.Web.UI.Design.MobileControls.Adapters; using System.Web.UI.Design.MobileControls.Converters; ////// ////// Provides a designer for the ////// control. /// [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal class PhoneCallDesigner : MobileControlDesigner { private System.Web.UI.MobileControls.PhoneCall _call; /// /// /// /// The control element for design. /// ////// Initializes the designer with the component for design. /// ////// ////// This is called by the designer host to establish the component for /// design. /// ///public override void Initialize(IComponent component) { Debug.Assert(component is System.Web.UI.MobileControls.PhoneCall, "PhoneCallDesigner.Initialize - Invalid PhoneCall Control"); _call = (System.Web.UI.MobileControls.PhoneCall) component; base.Initialize(component); } /// /// ////// Returns the design-time HTML of the ////// mobile control /// /// ////// The HTML of the control. /// ///protected override String GetDesignTimeNormalHtml() { Debug.Assert(_call.Text != null); DesignerTextWriter tw; Control[] children = null; String originalText = _call.Text; bool blankText = (originalText.Trim().Length == 0); bool hasControls = _call.HasControls(); if (blankText) { if (hasControls) { children = new Control[_call.Controls.Count]; _call.Controls.CopyTo(children, 0); } _call.Text = "[" + _call.ID + "]"; } try { tw = new DesignerTextWriter(); _call.Adapter.Render(tw); } finally { if (blankText) { _call.Text = originalText; if (hasControls) { foreach (Control c in children) { _call.Controls.Add(c); } } } } return tw.ToString(); } public override void OnComponentChanged(Object sender, ComponentChangedEventArgs e) { if ((e.Member != null) && e.Member.Name.Equals("AlternateUrl")) { _call.AlternateUrl = NavigateUrlConverter.GetUrl( _call, e.NewValue.ToString(), e.OldValue.ToString() ); e = new ComponentChangedEventArgs(e.Component, e.Member, e.OldValue, _call.AlternateUrl); } base.OnComponentChanged(sender, e); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
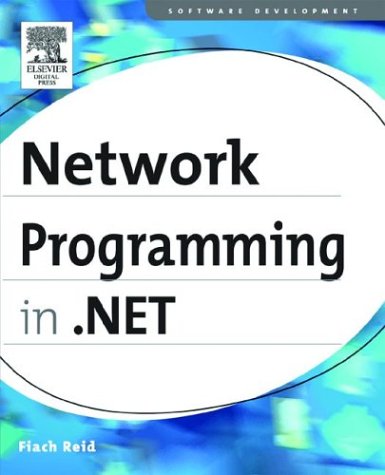
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA1.cs
- GridLengthConverter.cs
- MethodBuilder.cs
- SafeFileHandle.cs
- TypeSystem.cs
- Identifier.cs
- AppDomain.cs
- BitmapCodecInfoInternal.cs
- ListViewGroup.cs
- ToolboxService.cs
- BitmapMetadata.cs
- WMIInterop.cs
- IdentifierService.cs
- StringHelper.cs
- TextTreeTextBlock.cs
- SimpleModelProvider.cs
- PasswordTextContainer.cs
- PerspectiveCamera.cs
- Sorting.cs
- DoubleAnimationUsingPath.cs
- XmlLoader.cs
- TimeoutException.cs
- HtmlInputHidden.cs
- ConstructorNeedsTagAttribute.cs
- IndexedEnumerable.cs
- GacUtil.cs
- EntitySqlQueryCacheEntry.cs
- TabControlCancelEvent.cs
- SQLRoleProvider.cs
- SmuggledIUnknown.cs
- DataSourceCacheDurationConverter.cs
- XmlLanguageConverter.cs
- SharedUtils.cs
- DesignerTextBoxAdapter.cs
- CrossAppDomainChannel.cs
- ServerValidateEventArgs.cs
- CalendarButton.cs
- TaskHelper.cs
- DiagnosticsConfigurationHandler.cs
- SubstitutionList.cs
- AdornerLayer.cs
- MulticastNotSupportedException.cs
- MemoryRecordBuffer.cs
- TransactionManager.cs
- DeclaredTypeValidatorAttribute.cs
- Vector3DCollectionConverter.cs
- _SSPIWrapper.cs
- ExpressionsCollectionConverter.cs
- XmlWrappingReader.cs
- GridViewPageEventArgs.cs
- LinqDataSourceValidationException.cs
- MetadataUtilsSmi.cs
- AnnotationResourceCollection.cs
- ChainOfDependencies.cs
- DbModificationClause.cs
- StylusPointPropertyInfo.cs
- QueueProcessor.cs
- WebPartActionVerb.cs
- DynamicEndpoint.cs
- SqlDataSourceEnumerator.cs
- EmptyCollection.cs
- CallSiteOps.cs
- List.cs
- SystemIPv4InterfaceProperties.cs
- MulticastIPAddressInformationCollection.cs
- ParentUndoUnit.cs
- Rect.cs
- SurrogateEncoder.cs
- ScrollEvent.cs
- TextParagraphCache.cs
- Int32Converter.cs
- _AcceptOverlappedAsyncResult.cs
- WindowsImpersonationContext.cs
- InputScopeConverter.cs
- FunctionGenerator.cs
- InfoCardSymmetricCrypto.cs
- SchemaImporterExtensionElement.cs
- ObjectDataSourceMethodEditor.cs
- ProcessThreadCollection.cs
- CdpEqualityComparer.cs
- HttpServerVarsCollection.cs
- ValidationResult.cs
- AxisAngleRotation3D.cs
- Sql8ExpressionRewriter.cs
- FilterEventArgs.cs
- ListItemParagraph.cs
- BuildProviderCollection.cs
- LocalizationParserHooks.cs
- CompilerHelpers.cs
- UserPreferenceChangingEventArgs.cs
- SqlVersion.cs
- XPathEmptyIterator.cs
- WebBrowserContainer.cs
- UserNamePasswordClientCredential.cs
- XPathBuilder.cs
- DesignerWidgets.cs
- TextTreeInsertElementUndoUnit.cs
- SimpleLine.cs
- PowerModeChangedEventArgs.cs
- SecurityKeyIdentifier.cs