Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / ChainOfDependencies.cs / 1 / ChainOfDependencies.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// A Generic that provides user with the ability to chain dependent objects // of a shared base type and perform actions on them in order of dependency. // // // History: // 08/28/2005: [....]: Initial implementation. //----------------------------------------------------------------------------- namespace MS.Internal.Documents.Application { ////// A Generic that provides user with the ability to chain dependent objects /// of a shared base type and perform actions on them in order of dependency. /// ////// This is different from the chain of responsiblity in the following ways: /// /// - Order of execution in the chain can be inversed by calling LastToFirst. /// - The same operation is performed on each member once. /// /// This class has many methods which are intentionaly recursive. There is /// currently no validation to prevent cyclic dependencies. As the chain is /// currently fixed at compile time there is no need; StackOverFlowException /// durring testing is fine. /// ///A type common to all in the chain. internal static class ChainOfDependencieswhere T : IChainOfDependenciesNode { #region Internal Methods //------------------------------------------------------------------------- // Internal Methods //------------------------------------------------------------------------- /// /// Gets the last member in the chain. (The one with no dependencies.) /// /// The current member. ///The last member in the chain. (The one with no dependencies.) /// internal static T GetLast(T member) { T last = member; if (member.Dependency != null) { last = GetLast(member.Dependency); } return last; } ////// Will perform the action from the member with no dependencies to the most /// dependent member. /// /// The member on which to perform the action. /// The action to perform on the member. ///Returns true if all the actions returned true. internal static bool OrderByLeastDependent( T member, ChainOfDependencies.Action action) { bool satisfied = true; T nextInChain = member.Dependency; if (nextInChain != null) { satisfied = OrderByLeastDependent(nextInChain, action); } if (satisfied) { satisfied = action(member); } else { Trace.SafeWrite( Trace.File, "Dependency for {0}#{1} was not satisfied skipping action.", member.GetType(), member.GetHashCode()); } return satisfied; } /// /// Will perform the action from most dependent to not dependent. /// /// The member on which to perform the action. /// The action to perform on the member. ///Returns true if the all the actions returned true. internal static bool OrderByMostDependent( T member, ChainOfDependencies.Action action) { bool satisfied = action(member); T nextInChain = member.Dependency; if (satisfied) { if (nextInChain != null) { satisfied = OrderByMostDependent(nextInChain, action); } } else { Trace.SafeWrite( Trace.File, "Dependency for {0}#{1} was not satisfied skipping action.", member.GetType(), member.GetHashCode()); } return satisfied; } #endregion Internal Methods #region Internal Delegates //-------------------------------------------------------------------------- // Internal Delegates //------------------------------------------------------------------------- /// /// An action to perform on a ChainOfDependencies member. /// /// The member on which to perform the action. ///True if the dependency was satisfied. internal delegate bool Action(T member); #endregion Internal Delegates } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
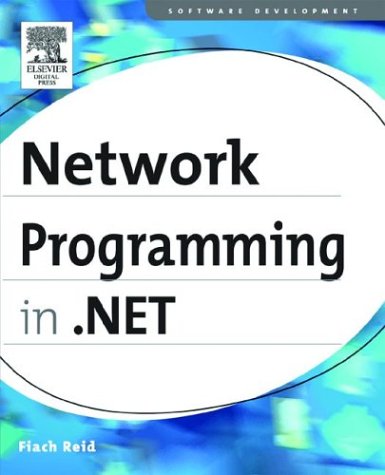
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KnownTypesHelper.cs
- SweepDirectionValidation.cs
- DateTime.cs
- DefaultAssemblyResolver.cs
- InvariantComparer.cs
- ServiceProviders.cs
- SqlDataSource.cs
- BulletDecorator.cs
- CallSiteBinder.cs
- Geometry3D.cs
- ChtmlTextWriter.cs
- ZoneLinkButton.cs
- TextServicesLoader.cs
- PtsCache.cs
- EventMap.cs
- DependencyPropertyAttribute.cs
- UriWriter.cs
- StylesEditorDialog.cs
- GroupAggregateExpr.cs
- SemanticResultValue.cs
- StrokeNodeEnumerator.cs
- TimeIntervalCollection.cs
- CreateUserWizardAutoFormat.cs
- CodeDomDecompiler.cs
- CodeAttributeDeclarationCollection.cs
- InvalidDataContractException.cs
- SqlCharStream.cs
- Calendar.cs
- ResourcePool.cs
- ChangeTracker.cs
- OdbcConnection.cs
- CodeAttributeArgument.cs
- ToolStripRendererSwitcher.cs
- DashStyle.cs
- ExtensionSimplifierMarkupObject.cs
- SqlDataReader.cs
- SubclassTypeValidatorAttribute.cs
- CharacterBuffer.cs
- PnrpPeerResolverBindingElement.cs
- OdbcConnectionFactory.cs
- BuildProviderUtils.cs
- BufferModeSettings.cs
- ExeConfigurationFileMap.cs
- RowBinding.cs
- UnionQueryOperator.cs
- ResXResourceReader.cs
- _RegBlobWebProxyDataBuilder.cs
- CharEnumerator.cs
- DataSourceControl.cs
- MetaForeignKeyColumn.cs
- InputBinder.cs
- CreateUserErrorEventArgs.cs
- ViewManagerAttribute.cs
- CodeNamespaceImportCollection.cs
- Point3DAnimation.cs
- DataGridViewRowCancelEventArgs.cs
- DnsPermission.cs
- SecondaryIndexList.cs
- TransformerInfoCollection.cs
- BaseConfigurationRecord.cs
- PersistChildrenAttribute.cs
- SQLInt32Storage.cs
- TickBar.cs
- RequestResizeEvent.cs
- HttpModuleActionCollection.cs
- FragmentNavigationEventArgs.cs
- ObjectDataSourceSelectingEventArgs.cs
- ActiveXContainer.cs
- WindowsTooltip.cs
- ScrollChrome.cs
- ObjectStateEntryDbDataRecord.cs
- ListViewSortEventArgs.cs
- MappingItemCollection.cs
- HttpWriter.cs
- PerformanceCounter.cs
- StyleTypedPropertyAttribute.cs
- NativeRightsManagementAPIsStructures.cs
- AssemblyBuilder.cs
- TableCellsCollectionEditor.cs
- ScriptControlManager.cs
- DateTimeValueSerializerContext.cs
- OperatingSystem.cs
- XmlQuerySequence.cs
- XamlFigureLengthSerializer.cs
- InfoCardProofToken.cs
- DataProtection.cs
- FeatureManager.cs
- TextTabProperties.cs
- DirectionalLight.cs
- HelpHtmlBuilder.cs
- ResourceDictionaryCollection.cs
- SizeConverter.cs
- ManipulationCompletedEventArgs.cs
- PermissionSetTriple.cs
- CharacterBufferReference.cs
- StringSorter.cs
- DataGridViewCell.cs
- XmlSerializerVersionAttribute.cs
- IdentifierService.cs
- SpinLock.cs