Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewLinkColumn.cs / 1305376 / DataGridViewLinkColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Text; using System.Drawing; using System.ComponentModel; using System.Windows.Forms; using System.Globalization; ///[ToolboxBitmapAttribute(typeof(DataGridViewLinkColumn), "DataGridViewLinkColumn.bmp")] public class DataGridViewLinkColumn : DataGridViewColumn { private static Type columnType = typeof(DataGridViewLinkColumn); private string text; /// public DataGridViewLinkColumn() : base(new DataGridViewLinkCell()) { } /// [ SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_LinkColumnActiveLinkColorDescr) ] public Color ActiveLinkColor { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewLinkCell)this.CellTemplate).ActiveLinkColor; } set { if (!this.ActiveLinkColor.Equals(value)) { ((DataGridViewLinkCell)this.CellTemplate).ActiveLinkColorInternal = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null) { dataGridViewCell.ActiveLinkColorInternal = value; } } this.DataGridView.InvalidateColumn(this.Index); } } } } private bool ShouldSerializeActiveLinkColor() { return !this.ActiveLinkColor.Equals(LinkUtilities.IEActiveLinkColor); } /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override DataGridViewCell CellTemplate { get { return base.CellTemplate; } set { if (value != null && !(value is System.Windows.Forms.DataGridViewLinkCell)) { throw new InvalidCastException(SR.GetString(SR.DataGridViewTypeColumn_WrongCellTemplateType, "System.Windows.Forms.DataGridViewLinkCell")); } base.CellTemplate = value; } } /// [ DefaultValue(LinkBehavior.SystemDefault), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_LinkColumnLinkBehaviorDescr) ] public LinkBehavior LinkBehavior { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewLinkCell)this.CellTemplate).LinkBehavior; } set { if (!this.LinkBehavior.Equals(value)) { ((DataGridViewLinkCell)this.CellTemplate).LinkBehavior = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null) { dataGridViewCell.LinkBehaviorInternal = value; } } this.DataGridView.InvalidateColumn(this.Index); } } } } /// [ SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_LinkColumnLinkColorDescr) ] public Color LinkColor { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewLinkCell)this.CellTemplate).LinkColor; } set { if (!this.LinkColor.Equals(value)) { ((DataGridViewLinkCell)this.CellTemplate).LinkColorInternal = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null) { dataGridViewCell.LinkColorInternal = value; } } this.DataGridView.InvalidateColumn(this.Index); } } } } private bool ShouldSerializeLinkColor() { return !this.LinkColor.Equals(LinkUtilities.IELinkColor); } /// [ DefaultValue(null), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_LinkColumnTextDescr) ] public string Text { get { return this.text; } set { if (!string.Equals(value, this.text, StringComparison.Ordinal)) { this.text = value; if (this.DataGridView != null) { if (this.UseColumnTextForLinkValue) { this.DataGridView.OnColumnCommonChange(this.Index); } else { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null && dataGridViewCell.UseColumnTextForLinkValue) { this.DataGridView.OnColumnCommonChange(this.Index); return; } } this.DataGridView.InvalidateColumn(this.Index); } } } } } /// [ DefaultValue(true), SRCategory(SR.CatBehavior), SRDescription(SR.DataGridView_LinkColumnTrackVisitedStateDescr) ] public bool TrackVisitedState { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewLinkCell)this.CellTemplate).TrackVisitedState; } set { if (this.TrackVisitedState != value) { ((DataGridViewLinkCell)this.CellTemplate).TrackVisitedStateInternal = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null) { dataGridViewCell.TrackVisitedStateInternal = value; } } this.DataGridView.InvalidateColumn(this.Index); } } } } /// [ DefaultValue(false), SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_LinkColumnUseColumnTextForLinkValueDescr) ] public bool UseColumnTextForLinkValue { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewLinkCell)this.CellTemplate).UseColumnTextForLinkValue; } set { if (this.UseColumnTextForLinkValue != value) { ((DataGridViewLinkCell)this.CellTemplate).UseColumnTextForLinkValueInternal = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null) { dataGridViewCell.UseColumnTextForLinkValueInternal = value; } } this.DataGridView.OnColumnCommonChange(this.Index); } } } } /// [ SRCategory(SR.CatAppearance), SRDescription(SR.DataGridView_LinkColumnVisitedLinkColorDescr) ] public Color VisitedLinkColor { get { if (this.CellTemplate == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewColumn_CellTemplateRequired)); } return ((DataGridViewLinkCell)this.CellTemplate).VisitedLinkColor; } set { if (!this.VisitedLinkColor.Equals(value)) { ((DataGridViewLinkCell)this.CellTemplate).VisitedLinkColorInternal = value; if (this.DataGridView != null) { DataGridViewRowCollection dataGridViewRows = this.DataGridView.Rows; int rowCount = dataGridViewRows.Count; for (int rowIndex = 0; rowIndex < rowCount; rowIndex++) { DataGridViewRow dataGridViewRow = dataGridViewRows.SharedRow(rowIndex); DataGridViewLinkCell dataGridViewCell = dataGridViewRow.Cells[this.Index] as DataGridViewLinkCell; if (dataGridViewCell != null) { dataGridViewCell.VisitedLinkColorInternal = value; } } this.DataGridView.InvalidateColumn(this.Index); } } } } private bool ShouldSerializeVisitedLinkColor() { return !this.VisitedLinkColor.Equals(LinkUtilities.IEVisitedLinkColor); } /// public override object Clone() { DataGridViewLinkColumn dataGridViewColumn; Type thisType = this.GetType(); if (thisType == columnType) //performance improvement { dataGridViewColumn = new DataGridViewLinkColumn(); } else { // SECREVIEW : Late-binding does not represent a security threat, see bug#411899 for more info.. // dataGridViewColumn = (DataGridViewLinkColumn)System.Activator.CreateInstance(thisType); } if (dataGridViewColumn != null) { base.CloneInternal(dataGridViewColumn); dataGridViewColumn.Text = this.text; } return dataGridViewColumn; } /// public override string ToString() { StringBuilder sb = new StringBuilder(64); sb.Append("DataGridViewLinkColumn { Name="); sb.Append(this.Name); sb.Append(", Index="); sb.Append(this.Index.ToString(CultureInfo.CurrentCulture)); sb.Append(" }"); return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
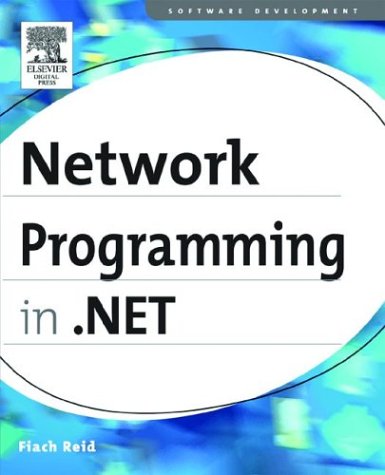
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsListViewGroup.cs
- StringUtil.cs
- SafeCryptHandles.cs
- EntityDataSourceDataSelection.cs
- MetaChildrenColumn.cs
- SHA512Managed.cs
- BuildManagerHost.cs
- DataGridGeneralPage.cs
- DataKey.cs
- Base64Encoder.cs
- Int64AnimationBase.cs
- HttpBrowserCapabilitiesWrapper.cs
- FlowDocument.cs
- ExpressionBindings.cs
- ElapsedEventArgs.cs
- SmiGettersStream.cs
- SiteMapDataSource.cs
- ArcSegment.cs
- CopyNodeSetAction.cs
- InputBinding.cs
- DocumentXPathNavigator.cs
- ClassDataContract.cs
- NullableConverter.cs
- SettingsAttributeDictionary.cs
- PolicyLevel.cs
- ApplicationServiceManager.cs
- MatrixTransform.cs
- Container.cs
- ParserExtension.cs
- MSG.cs
- ApplicationContext.cs
- DSASignatureFormatter.cs
- TitleStyle.cs
- Operators.cs
- RegistryKey.cs
- OdbcEnvironmentHandle.cs
- CodeVariableReferenceExpression.cs
- RegexCode.cs
- ISessionStateStore.cs
- EmbeddedMailObject.cs
- AsyncDataRequest.cs
- FileDialog_Vista.cs
- SerialPinChanges.cs
- MsmqChannelFactory.cs
- HwndTarget.cs
- FileLevelControlBuilderAttribute.cs
- DTCTransactionManager.cs
- ObjectTypeMapping.cs
- SecureUICommand.cs
- BrushMappingModeValidation.cs
- ReversePositionQuery.cs
- RtfControls.cs
- OdbcInfoMessageEvent.cs
- Rule.cs
- SystemException.cs
- InheritanceContextChangedEventManager.cs
- ProfileService.cs
- SqlUserDefinedTypeAttribute.cs
- CatalogZoneBase.cs
- FilePrompt.cs
- Matrix.cs
- ModelService.cs
- AttachInfo.cs
- SmtpFailedRecipientException.cs
- Baml2006KnownTypes.cs
- IdnElement.cs
- MasterPageCodeDomTreeGenerator.cs
- ConfigurationSettings.cs
- ObjectIDGenerator.cs
- SessionSwitchEventArgs.cs
- DecimalConverter.cs
- ReaderWriterLock.cs
- Int64KeyFrameCollection.cs
- QilFactory.cs
- MiniLockedBorderGlyph.cs
- CopyOnWriteList.cs
- FixedSOMTableRow.cs
- SerTrace.cs
- cookiecontainer.cs
- ReferencedAssemblyResolver.cs
- StrongNameSignatureInformation.cs
- WindowsRebar.cs
- NativeMethods.cs
- BuildProvidersCompiler.cs
- DataGridViewCellCollection.cs
- StructuralComparisons.cs
- FormattedText.cs
- cookiecollection.cs
- MsmqOutputChannel.cs
- RoutedEventConverter.cs
- EntityViewGenerationConstants.cs
- PathFigure.cs
- XmlValidatingReader.cs
- RIPEMD160Managed.cs
- Comparer.cs
- ProcessProtocolHandler.cs
- ConfigXmlAttribute.cs
- EdmTypeAttribute.cs
- EntityModelSchemaGenerator.cs
- SoapFault.cs