Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / StaticExtension.cs / 1 / StaticExtension.cs
/****************************************************************************\ * * File: StaticExtension.cs * * Class for Xaml markup extension for static field and property references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Windows; using System.Windows.Input; using System.Reflection; using MS.Utility; namespace System.Windows.Markup { ////// Class for Xaml markup extension for static field and property references. /// [TypeConverter(typeof(StaticExtensionConverter))] [MarkupExtensionReturnType(typeof(object))] public class StaticExtension : MarkupExtension { ////// Constructor that takes no parameters /// public StaticExtension() { } ////// Constructor that takes the member that this is a static reference to. /// This string is of the format /// Prefix:ClassName.FieldOrPropertyName. The Prefix is /// optional, and refers to the XML prefix in a Xaml file. /// public StaticExtension( string member) { if (member == null) { throw new ArgumentNullException("member"); } _member = member; } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For a StaticExtension this is a static field /// or property value. /// /// Object that can provide services for the markup extension. ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (_member == null) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionStaticMember)); } object value = null; Type type = MemberType; string fieldString = null; string memberFullName = null; if (type != null) { fieldString = _member; memberFullName = type.FullName + "." + _member; } else { memberFullName = _member; // optimize for some well known common resource keys defined by known controls. // if found just return the value, else use reflection to get the value. // This optimization can be removed from here in the future, if the Baml record // for resource keys is optimized to store this info. value = SystemResourceKey.GetSystemResourceKey(_member); if (value != null) { return value; } // Validate the _member int dotIndex = _member.IndexOf('.'); if (dotIndex < 0) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } // Pull out the type substring (this will include any XML prefix, e.g. "av:Button") string typeString = _member.Substring(0, dotIndex); if (typeString == string.Empty) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } // Get the IXamlTypeResolver from the service provider IXamlTypeResolver xamlTypeResolver = serviceProvider.GetService(typeof(IXamlTypeResolver)) as IXamlTypeResolver; if (xamlTypeResolver == null) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IXamlTypeResolver")); } // Use the type resolver to get a Type instance type = xamlTypeResolver.Resolve(typeString); // Get the member name substring fieldString = _member.Substring(dotIndex + 1, _member.Length - dotIndex - 1); if (fieldString == string.Empty) { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, _member)); } } // optimize for some well known common commands defined by known controls. // if found just return the value, else use reflection to get the value. // This optimization can be removed from here in the future, if each known // or registered command is mapped to a CommandId that is then stored in the // Baml record insated of the string for the command name. value = CommandConverter.GetKnownControlCommand(type, fieldString); if (value != null) { return value; } // Use the built-in parser for enum types if (type.IsEnum) { return Enum.Parse(type, fieldString); } // For other types, reflect bool found = false; object fieldOrProp = type.GetField(fieldString, BindingFlags.Public | BindingFlags.FlattenHierarchy | BindingFlags.Static); if (fieldOrProp == null) { fieldOrProp = type.GetProperty(fieldString, BindingFlags.Public | BindingFlags.FlattenHierarchy | BindingFlags.Static); if (fieldOrProp is PropertyInfo) { value = ((PropertyInfo)fieldOrProp).GetValue(null,null); found = true; } } else if (fieldOrProp is FieldInfo) { value = ((FieldInfo)fieldOrProp).GetValue(null); found = true; } if (found) { return value; } else { throw new ArgumentException(SR.Get(SRID.MarkupExtensionBadStatic, memberFullName)); } } ////// The static field or property represented by a string. This string is /// of the format Prefix:ClassName.FieldOrPropertyName. The Prefix is /// optional, and refers to the XML prefix in a Xaml file. /// [ConstructorArgument("member")] public string Member { get { return _member; } set { if (value == null) { throw new ArgumentNullException("value"); } _member = value; } } internal Type MemberType { get { return _memberType; } set { if (value == null) { throw new ArgumentNullException("value"); } _memberType = value; } } private string _member; private Type _memberType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
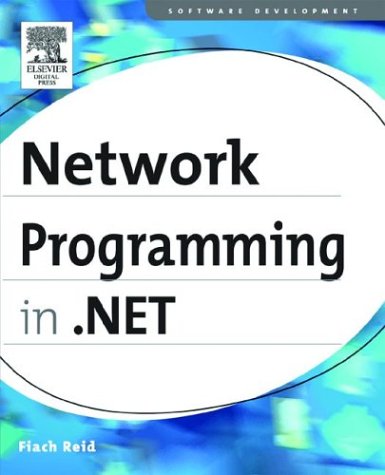
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Config.cs
- FontCollection.cs
- JournalEntry.cs
- WriteTimeStream.cs
- WebPartCatalogCloseVerb.cs
- DbConnectionPoolGroupProviderInfo.cs
- ScopelessEnumAttribute.cs
- WorkflowExecutor.cs
- Trigger.cs
- IBuiltInEvidence.cs
- HtmlToClrEventProxy.cs
- Win32SafeHandles.cs
- StubHelpers.cs
- GridViewDeletedEventArgs.cs
- FixedStringLookup.cs
- TextEditorDragDrop.cs
- XmlSchemaSimpleTypeUnion.cs
- StateMachine.cs
- SplineQuaternionKeyFrame.cs
- BrowserTree.cs
- Baml2006KnownTypes.cs
- SQLMembershipProvider.cs
- DbCommandDefinition.cs
- KeyManager.cs
- SafeEventHandle.cs
- EntityDataSourceSelectedEventArgs.cs
- DetailsViewActionList.cs
- dsa.cs
- CacheOutputQuery.cs
- TargetFrameworkUtil.cs
- COM2ColorConverter.cs
- OleDbInfoMessageEvent.cs
- XmlSchemaAnnotated.cs
- ComponentDispatcherThread.cs
- ReaderContextStackData.cs
- SignatureResourcePool.cs
- SamlAssertionKeyIdentifierClause.cs
- NavigatorInvalidBodyAccessException.cs
- QuaternionConverter.cs
- ImageList.cs
- querybuilder.cs
- sitestring.cs
- MetadataAssemblyHelper.cs
- CodeSnippetCompileUnit.cs
- RouteValueExpressionBuilder.cs
- isolationinterop.cs
- MaterialGroup.cs
- OdbcEnvironmentHandle.cs
- XmlAnyAttributeAttribute.cs
- ControlParser.cs
- SynchronizationLockException.cs
- EntityContainerRelationshipSetEnd.cs
- SmiContext.cs
- DataProviderNameConverter.cs
- CompilationUnit.cs
- ServiceSecurityAuditElement.cs
- GZipUtils.cs
- XomlCompilerError.cs
- SafeNativeMethods.cs
- COM2EnumConverter.cs
- _BufferOffsetSize.cs
- ScrollContentPresenter.cs
- RecognizedWordUnit.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- FocusChangedEventArgs.cs
- TextureBrush.cs
- odbcmetadatacolumnnames.cs
- CollectionViewGroup.cs
- SQLDecimal.cs
- FormatConvertedBitmap.cs
- XPathNodePointer.cs
- DecimalStorage.cs
- SqlUserDefinedTypeAttribute.cs
- ConstraintCollection.cs
- typedescriptorpermissionattribute.cs
- XmlAttribute.cs
- ReaderOutput.cs
- unsafenativemethodsother.cs
- BooleanFunctions.cs
- UpdatePanel.cs
- FacetChecker.cs
- TextSerializer.cs
- cookieexception.cs
- FixedTextBuilder.cs
- ArraySet.cs
- Oid.cs
- WebSysDescriptionAttribute.cs
- UIElementHelper.cs
- WebPartUserCapability.cs
- ImageBrush.cs
- HttpHandlerAction.cs
- RectAnimationClockResource.cs
- ProtectedConfigurationProviderCollection.cs
- JsonSerializer.cs
- HttpResponseHeader.cs
- CodeCatchClause.cs
- ToolStripSeparatorRenderEventArgs.cs
- SymLanguageType.cs
- TextCompositionManager.cs
- SeekStoryboard.cs