Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / IO / Zip / WriteTimeStream.cs / 1 / WriteTimeStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WriteTimeStream - wraps the ArchiveStream in Streaming generation scenarios so that we // can determine current archive stream offset even when working on a stream that is non-seekable // because the Position property is unusable on such streams. // // History: // 03/25/2002: BruceMac: Created. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows; namespace MS.Internal.IO.Zip { internal class WriteTimeStream : Stream { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// CanRead - never /// override public bool CanRead { get { return false; } } ////// CanSeek - never /// override public bool CanSeek{ get { return false; } } ////// CanWrite - only if we are not disposed /// override public bool CanWrite { get { return (_baseStream != null); } } ////// Same as Position /// override public long Length { get { CheckDisposed(); return _position; } } ////// Get is supported even on Write-only stream /// override public long Position { get { CheckDisposed(); return _position; } set { CheckDisposed(); IllegalAccess(); // throw exception } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- public override void SetLength(long newLength) { IllegalAccess(); // throw exception } override public long Seek(long offset, SeekOrigin origin) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public int Read(byte[] buffer, int offset, int count) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _baseStream.Write(buffer, offset, count); checked{_position += count;} } override public void Flush() { CheckDisposed(); _baseStream.Flush(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ internal WriteTimeStream(Stream baseStream) { if (baseStream == null) { throw new ArgumentNullException("baseStream"); } _baseStream = baseStream; // must be based on writable stream if (!_baseStream.CanWrite) throw new ArgumentException(SR.Get(SRID.WriteNotSupported), "baseStream"); } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && (_baseStream != null)) { _baseStream.Close(); } } finally { _baseStream = null; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private static void IllegalAccess() { throw new NotSupportedException(SR.Get(SRID.WriteOnlyStream)); } private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } // _baseStream doubles as our disposed indicator - it's null if we are disposed private Stream _baseStream; // stream we wrap - needs to only support Write private long _position; // current position } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WriteTimeStream - wraps the ArchiveStream in Streaming generation scenarios so that we // can determine current archive stream offset even when working on a stream that is non-seekable // because the Position property is unusable on such streams. // // History: // 03/25/2002: BruceMac: Created. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows; namespace MS.Internal.IO.Zip { internal class WriteTimeStream : Stream { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// CanRead - never /// override public bool CanRead { get { return false; } } ////// CanSeek - never /// override public bool CanSeek{ get { return false; } } ////// CanWrite - only if we are not disposed /// override public bool CanWrite { get { return (_baseStream != null); } } ////// Same as Position /// override public long Length { get { CheckDisposed(); return _position; } } ////// Get is supported even on Write-only stream /// override public long Position { get { CheckDisposed(); return _position; } set { CheckDisposed(); IllegalAccess(); // throw exception } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- public override void SetLength(long newLength) { IllegalAccess(); // throw exception } override public long Seek(long offset, SeekOrigin origin) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public int Read(byte[] buffer, int offset, int count) { IllegalAccess(); // throw exception return -1; // keep compiler happy } override public void Write(byte[] buffer, int offset, int count) { CheckDisposed(); _baseStream.Write(buffer, offset, count); checked{_position += count;} } override public void Flush() { CheckDisposed(); _baseStream.Flush(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ internal WriteTimeStream(Stream baseStream) { if (baseStream == null) { throw new ArgumentNullException("baseStream"); } _baseStream = baseStream; // must be based on writable stream if (!_baseStream.CanWrite) throw new ArgumentException(SR.Get(SRID.WriteNotSupported), "baseStream"); } //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ protected override void Dispose(bool disposing) { try { if (disposing && (_baseStream != null)) { _baseStream.Close(); } } finally { _baseStream = null; base.Dispose(disposing); } } //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- private static void IllegalAccess() { throw new NotSupportedException(SR.Get(SRID.WriteOnlyStream)); } private void CheckDisposed() { if (_baseStream == null) throw new ObjectDisposedException("Stream"); } // _baseStream doubles as our disposed indicator - it's null if we are disposed private Stream _baseStream; // stream we wrap - needs to only support Write private long _position; // current position } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
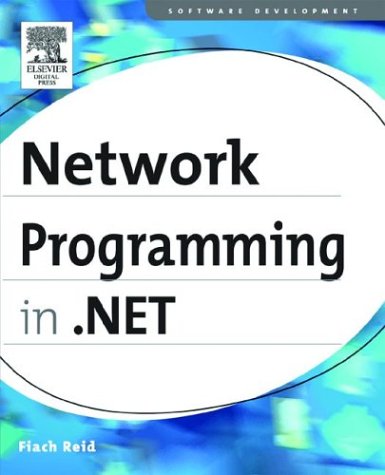
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IDQuery.cs
- BindingSource.cs
- Authorization.cs
- Form.cs
- ImageCodecInfoPrivate.cs
- PathSegmentCollection.cs
- MaterialGroup.cs
- BoundingRectTracker.cs
- Function.cs
- PreservationFileReader.cs
- parserscommon.cs
- SqlStream.cs
- GeneralTransform2DTo3D.cs
- XPathNodeIterator.cs
- HtmlInputPassword.cs
- ConfigurationManager.cs
- FrameworkContentElement.cs
- TraceHwndHost.cs
- DataViewSetting.cs
- GcHandle.cs
- DataContract.cs
- TableLayoutPanelCodeDomSerializer.cs
- ActivityUtilities.cs
- KerberosSecurityTokenAuthenticator.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- XmlSortKey.cs
- KeyGestureConverter.cs
- AstNode.cs
- ExecutionScope.cs
- DetailsViewRow.cs
- CallbackHandler.cs
- RedirectionProxy.cs
- InfoCardBinaryReader.cs
- SqlFactory.cs
- TrackingConditionCollection.cs
- Drawing.cs
- RouteCollection.cs
- Hashtable.cs
- X509Certificate2.cs
- LineSegment.cs
- ZoneLinkButton.cs
- EntityKeyElement.cs
- FtpWebRequest.cs
- SqlConnectionPoolGroupProviderInfo.cs
- TextTreePropertyUndoUnit.cs
- ReplyChannel.cs
- ErrorHandlerModule.cs
- X509ThumbprintKeyIdentifierClause.cs
- DataError.cs
- SqlClientPermission.cs
- ObjectTag.cs
- EntityDataSourceViewSchema.cs
- XPathSelectionIterator.cs
- TextEditorThreadLocalStore.cs
- NavigationExpr.cs
- AutomationPatternInfo.cs
- AsymmetricSignatureDeformatter.cs
- FrameworkPropertyMetadata.cs
- BitArray.cs
- _FixedSizeReader.cs
- ResolvePPIDRequest.cs
- ByValueEqualityComparer.cs
- SmtpTransport.cs
- ScriptingProfileServiceSection.cs
- ReferenceTypeElement.cs
- FrameworkContentElementAutomationPeer.cs
- KeyValueConfigurationElement.cs
- SafeRightsManagementPubHandle.cs
- WebPartUserCapability.cs
- ClientSettingsSection.cs
- StyleModeStack.cs
- PageCatalogPart.cs
- safex509handles.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ObjectSecurity.cs
- Trace.cs
- SourceFileBuildProvider.cs
- NameValueSectionHandler.cs
- AmbiguousMatchException.cs
- IndexedString.cs
- SHA512.cs
- SpecialFolderEnumConverter.cs
- XmlArrayAttribute.cs
- GradientStop.cs
- AttributeData.cs
- RequestCachePolicy.cs
- DataColumnChangeEvent.cs
- XmlHierarchicalDataSourceView.cs
- SQLBytes.cs
- SqlBooleanizer.cs
- ListViewTableCell.cs
- RectAnimation.cs
- ToReply.cs
- XmlSchemaAnnotation.cs
- TabletDevice.cs
- NumberFormatter.cs
- XmlAttributeOverrides.cs
- HotSpot.cs
- ContentIterators.cs
- WsdlInspector.cs