Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / QualifierSet.cs / 1305376 / QualifierSet.cs
using System; using System.Collections; using System.Runtime.InteropServices; using WbemClient_v1; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// ///Represents a collection of ///objects. /// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class QualifierDataCollection : ICollection, IEnumerable { private ManagementBaseObject parent; private string propertyOrMethodName; private QualifierType qualifierSetType; internal QualifierDataCollection(ManagementBaseObject parent) : base() { this.parent = parent; this.qualifierSetType = QualifierType.ObjectQualifier; this.propertyOrMethodName = null; } internal QualifierDataCollection(ManagementBaseObject parent, string propertyOrMethodName, QualifierType type) : base() { this.parent = parent; this.propertyOrMethodName = propertyOrMethodName; this.qualifierSetType = type; } ///using System; /// using System.Management; /// /// // This sample demonstrates how to list all qualifiers including amended /// // qualifiers of a ManagementClass object. /// class Sample_QualifierDataCollection /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("Win32_LogicalDisk"); /// diskClass.Options.UseAmendedQualifiers = true; /// QualifierDataCollection qualifierCollection = diskClass.Qualifiers; /// foreach (QualifierData q in qualifierCollection) { /// Console.WriteLine(q.Name + " = " + q.Value); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// ' This sample demonstrates how to list all qualifiers including amended /// ' qualifiers of a ManagementClass object. /// Class Sample_QualifierDataCollection /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("Win32_LogicalDisk") /// diskClass.Options.UseAmendedQualifiers = true /// Dim qualifierCollection As QualifierDataCollection = diskClass.Qualifiers /// Dim q As QualifierData /// For Each q In qualifierCollection /// Console.WriteLine(q.Name & " = " & q.Value) /// Next q /// Return 0 /// End Function /// End Class ///
////// Return the qualifier set associated with its type /// Overload with use of private data member, qualifierType /// private IWbemQualifierSetFreeThreaded GetTypeQualifierSet() { return GetTypeQualifierSet(qualifierSetType); } ////// Return the qualifier set associated with its type /// private IWbemQualifierSetFreeThreaded GetTypeQualifierSet(QualifierType qualifierSetType) { IWbemQualifierSetFreeThreaded qualifierSet = null; int status = (int)ManagementStatus.NoError; switch (qualifierSetType) { case QualifierType.ObjectQualifier : status = parent.wbemObject.GetQualifierSet_(out qualifierSet); break; case QualifierType.PropertyQualifier : status = parent.wbemObject.GetPropertyQualifierSet_(propertyOrMethodName, out qualifierSet); break; case QualifierType.MethodQualifier : status = parent.wbemObject.GetMethodQualifierSet_(propertyOrMethodName, out qualifierSet); break; default : throw new ManagementException(ManagementStatus.Unexpected, null, null); // Is this the best fit error ?? } if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return qualifierSet; } // //ICollection // ////// ///Gets or sets the number of ///objects in the . /// public int Count { get { string[] qualifierNames = null; IWbemQualifierSetFreeThreaded quals; try { quals = GetTypeQualifierSet(); } catch(ManagementException e) { // If we ask for the number of qualifiers on a system property, we return '0' if(qualifierSetType == QualifierType.PropertyQualifier && e.ErrorCode == ManagementStatus.SystemProperty) return 0; else throw; } int status = quals.GetNames_(0, out qualifierNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } return qualifierNames.Length; } } ///The number of objects in the collection. ////// ///Gets or sets a value indicating whether the object is synchronized. ////// public bool IsSynchronized { get { return false; } } ////// if the object is synchronized; /// otherwise, . /// ///Gets or sets the object to be used for synchronization. ////// public object SyncRoot { get { return this; } } ///The object to be used for synchronization. ////// ///Copies the ///into an array. /// /// The array to which to copy theCopies the ///into an array. . /// The index from which to start copying. public void CopyTo(Array array, int index) { if (null == array) throw new ArgumentNullException("array"); if ((index < array.GetLowerBound(0)) || (index > array.GetUpperBound(0))) throw new ArgumentOutOfRangeException("index"); // Get the names of the qualifiers string[] qualifierNames = null; IWbemQualifierSetFreeThreaded quals; try { quals = GetTypeQualifierSet(); } catch(ManagementException e) { // There are NO qualifiers on system properties, so we just return if(qualifierSetType == QualifierType.PropertyQualifier && e.ErrorCode == ManagementStatus.SystemProperty) return; else throw; } int status = quals.GetNames_(0, out qualifierNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } if ((index + qualifierNames.Length) > array.Length) throw new ArgumentException(null, "index"); foreach (string qualifierName in qualifierNames) array.SetValue(new QualifierData(parent, propertyOrMethodName, qualifierName, qualifierSetType), index++); return; } /// /// ///Copies the ///into a specialized /// /// array. The specialized array of /// The index from which to start copying. public void CopyTo(QualifierData[] qualifierArray, int index) { CopyTo((Array)qualifierArray, index); } // // IEnumerable // IEnumerator IEnumerable.GetEnumerator() { return (IEnumerator)(new QualifierDataEnumerator(parent, propertyOrMethodName, qualifierSetType)); } ///objects /// to which to copy the . /// ///Returns an enumerator for the ///. This method is strongly typed. /// public QualifierDataEnumerator GetEnumerator() { return new QualifierDataEnumerator(parent, propertyOrMethodName, qualifierSetType); } ///An ///that can be used to iterate through the /// collection. /// ///Represents the enumerator for ////// objects in the . /// public class QualifierDataEnumerator : IEnumerator { private ManagementBaseObject parent; private string propertyOrMethodName; private QualifierType qualifierType; private string[] qualifierNames; private int index = -1; //Internal constructor internal QualifierDataEnumerator(ManagementBaseObject parent, string propertyOrMethodName, QualifierType qualifierType) { this.parent = parent; this.propertyOrMethodName = propertyOrMethodName; this.qualifierType = qualifierType; this.qualifierNames = null; IWbemQualifierSetFreeThreaded qualifierSet = null; int status = (int)ManagementStatus.NoError; switch (qualifierType) { case QualifierType.ObjectQualifier : status = parent.wbemObject.GetQualifierSet_(out qualifierSet); break; case QualifierType.PropertyQualifier : status = parent.wbemObject.GetPropertyQualifierSet_(propertyOrMethodName, out qualifierSet); break; case QualifierType.MethodQualifier : status = parent.wbemObject.GetMethodQualifierSet_(propertyOrMethodName, out qualifierSet); break; default : throw new ManagementException(ManagementStatus.Unexpected, null, null); // Is this the best fit error ?? } // If we got an error code back, assume there are NO qualifiers for this object/property/method if(status < 0) { // qualifierNames = new String[]{}; } else { status = qualifierSet.GetNames_(0, out qualifierNames); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } } //Standard "Current" variant ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate qualifiers of a ManagementClass /// // using QualifierDataEnumerator object. /// class Sample_QualifierDataEnumerator /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("Win32_LogicalDisk"); /// diskClass.Options.UseAmendedQualifiers = true; /// QualifierDataCollection diskQualifier = diskClass.Qualifiers; /// QualifierDataCollection.QualifierDataEnumerator /// qualifierEnumerator = diskQualifier.GetEnumerator(); /// while(qualifierEnumerator.MoveNext()) { /// Console.WriteLine(qualifierEnumerator.Current.Name + " = " + /// qualifierEnumerator.Current.Value); /// } /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate qualifiers of a ManagementClass /// ' using QualifierDataEnumerator object. /// Class Sample_QualifierDataEnumerator /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("win32_logicaldisk") /// diskClass.Options.UseAmendedQualifiers = True /// Dim diskQualifier As QualifierDataCollection = diskClass.Qualifiers /// Dim qualifierEnumerator As _ /// QualifierDataCollection.QualifierDataEnumerator = _ /// diskQualifier.GetEnumerator() /// While qualifierEnumerator.MoveNext() /// Console.WriteLine(qualifierEnumerator.Current.Name & _ /// " = " & qualifierEnumerator.Current.Value) /// End While /// Return 0 /// End Function /// End Class ///
///object IEnumerator.Current { get { return (object)this.Current; } } /// /// ///Gets or sets the current ///in the enumeration. /// public QualifierData Current { get { if ((index == -1) || (index == qualifierNames.Length)) throw new InvalidOperationException(); else return new QualifierData(parent, propertyOrMethodName, qualifierNames[index], qualifierType); } } ///The current ///element in the collection. /// ///Moves to the next element in the ///enumeration. /// public bool MoveNext() { if (index == qualifierNames.Length) //passed the end of the array return false; //don't advance the index any more index++; return (index == qualifierNames.Length) ? false : true; } ////// if the enumerator was successfully advanced to the next /// element; if the enumerator has passed the end of the /// collection. /// public void Reset() { index = -1; } }//QualifierDataEnumerator // //Methods // ///Resets the enumerator to the beginning of the ///enumeration. /// /// The name of theGets the specified ///from the . to access in the . /// /// public virtual QualifierData this[string qualifierName] { get { if (null == qualifierName) throw new ArgumentNullException("qualifierName"); return new QualifierData(parent, propertyOrMethodName, qualifierName, qualifierSetType); } } ///A ///, based on the name specified. /// /// The name of theRemoves a ///from the by name. to remove. public virtual void Remove(string qualifierName) { int status = GetTypeQualifierSet().Delete_(qualifierName); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } /// /// ///Adds a ///to the . /// /// The name of theAdds a ///to the . This overload specifies the qualifier name and value. to be added to the . /// The value for the new qualifier. public virtual void Add(string qualifierName, object qualifierValue) { Add(qualifierName, qualifierValue, false, false, false, true); } /// /// /// The qualifier name. /// The qualifier value. ///Adds a ///to the . This overload /// specifies all property values for a object. to specify that this qualifier is amended (flavor); otherwise, . /// to propagate this qualifier to instances; otherwise, . /// to propagate this qualifier to subclasses; otherwise, . /// to specify that this qualifier's value is overridable in instances of subclasses; otherwise, . public virtual void Add(string qualifierName, object qualifierValue, bool isAmended, bool propagatesToInstance, bool propagatesToSubclass, bool isOverridable) { //Build the flavors bitmask and call the internal Add that takes a bitmask int qualFlavor = 0; if (isAmended) qualFlavor = (qualFlavor | (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED); if (propagatesToInstance) qualFlavor = (qualFlavor | (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE); if (propagatesToSubclass) qualFlavor = (qualFlavor | (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS); // Note we use the NOT condition here since WBEM_FLAVOR_OVERRIDABLE == 0 if (!isOverridable) qualFlavor = (qualFlavor | (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_NOT_OVERRIDABLE); //Try to add the qualifier to the WMI object int status = GetTypeQualifierSet().Put_(qualifierName, ref qualifierValue, qualFlavor); if (status < 0) { if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else Marshal.ThrowExceptionForHR(status); } } }//QualifierDataCollection } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
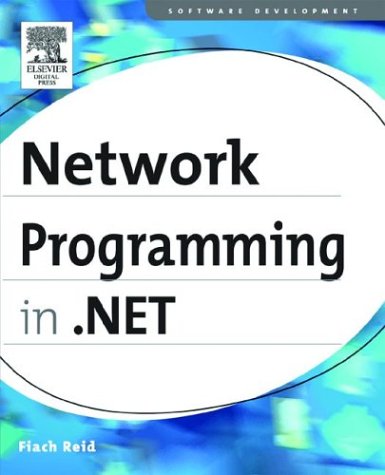
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComponentConverter.cs
- securitycriticaldataformultiplegetandset.cs
- VectorCollectionConverter.cs
- ServicesExceptionNotHandledEventArgs.cs
- SqlRecordBuffer.cs
- EndPoint.cs
- RtfToXamlLexer.cs
- ButtonFieldBase.cs
- dtdvalidator.cs
- BufferedGraphicsManager.cs
- ColorBlend.cs
- Odbc32.cs
- Expressions.cs
- XmlTextReader.cs
- CodeGroup.cs
- QueueProcessor.cs
- AffineTransform3D.cs
- AbandonedMutexException.cs
- ProgressBarHighlightConverter.cs
- RadioButtonBaseAdapter.cs
- WhiteSpaceTrimStringConverter.cs
- ExtendedPropertyInfo.cs
- BamlWriter.cs
- ScriptingProfileServiceSection.cs
- followingquery.cs
- RegistryKey.cs
- DataGridViewTopLeftHeaderCell.cs
- GenericTextProperties.cs
- WorkflowDurableInstance.cs
- SecurityManager.cs
- CodeArrayIndexerExpression.cs
- GC.cs
- CalendarDesigner.cs
- WebServiceData.cs
- StringAnimationBase.cs
- XhtmlBasicValidatorAdapter.cs
- ClickablePoint.cs
- ParamArrayAttribute.cs
- DateTimeSerializationSection.cs
- CodeGenerationManager.cs
- DynamicFilterExpression.cs
- SqlClientPermission.cs
- DeferredTextReference.cs
- UntrustedRecipientException.cs
- LabelLiteral.cs
- DataObject.cs
- MultipleFilterMatchesException.cs
- SendMessageRecord.cs
- WmlPhoneCallAdapter.cs
- SqlDataSource.cs
- DPTypeDescriptorContext.cs
- BaseValidatorDesigner.cs
- CanonicalXml.cs
- WebServiceEndpoint.cs
- DesignTimeParseData.cs
- PenThreadWorker.cs
- DataGridViewColumn.cs
- DependencyObjectProvider.cs
- SpeechSeg.cs
- PingReply.cs
- BeginStoryboard.cs
- StringFreezingAttribute.cs
- BasicAsyncResult.cs
- DataBindingHandlerAttribute.cs
- LongTypeConverter.cs
- XmlFormatWriterGenerator.cs
- TeredoHelper.cs
- SqlTypeSystemProvider.cs
- ContentAlignmentEditor.cs
- ZipFileInfo.cs
- ByteAnimationUsingKeyFrames.cs
- ColumnReorderedEventArgs.cs
- TransactionScope.cs
- AuthorizationRule.cs
- MaskedTextBox.cs
- GlyphRunDrawing.cs
- CrossSiteScriptingValidation.cs
- DataControlButton.cs
- ParenExpr.cs
- WebBrowser.cs
- PropertyConverter.cs
- InstanceHandleConflictException.cs
- ListViewGroupCollectionEditor.cs
- NativeActivityTransactionContext.cs
- XmlWriterTraceListener.cs
- DataObjectCopyingEventArgs.cs
- SettingsSavedEventArgs.cs
- ByeOperationAsyncResult.cs
- BaseCodeDomTreeGenerator.cs
- GridViewHeaderRowPresenter.cs
- PrintPreviewControl.cs
- VBIdentifierDesigner.xaml.cs
- DataGridTableCollection.cs
- AdRotator.cs
- TableRow.cs
- TextParentUndoUnit.cs
- FillBehavior.cs
- arc.cs
- dbdatarecord.cs
- CapabilitiesPattern.cs