Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / TransactionInformation.cs / 1305376 / TransactionInformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Transactions { using System; using System.Transactions.Diagnostics; public class TransactionInformation { private InternalTransaction internalTransaction; internal TransactionInformation( InternalTransaction internalTransaction ) { this.internalTransaction = internalTransaction; } public string LocalIdentifier { get { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "TransactionInformation.get_LocalIdentifier" ); } try { return this.internalTransaction.TransactionTraceId.TransactionIdentifier; } finally { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "TransactionInformation.get_LocalIdentifier" ); } } } } public Guid DistributedIdentifier { get { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "TransactionInformation.get_DistributedIdentifier" ); } try { // syncronize to avoid potential ---- between accessing the DistributerIdentifier // and getting the transaction information entry populated... lock(this.internalTransaction) { return this.internalTransaction.State.get_Identifier( this.internalTransaction ); } } finally { if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "TransactionInformation.get_DistributedIdentifier" ); } } } } public DateTime CreationTime { get { return new DateTime( this.internalTransaction.CreationTime ); } } public TransactionStatus Status { get { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "TransactionInformation.get_Status" ); } try { return this.internalTransaction.State.get_Status( this.internalTransaction ); } finally { if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceLtm ), "TransactionInformation.get_Status" ); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
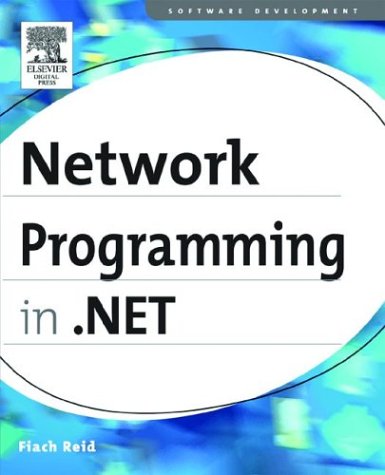
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RightsManagementInformation.cs
- LoginUtil.cs
- WebPartUtil.cs
- ClientTarget.cs
- UnauthorizedWebPart.cs
- TextProviderWrapper.cs
- DataGridCommandEventArgs.cs
- BooleanAnimationBase.cs
- SqlConnectionFactory.cs
- MaxMessageSizeStream.cs
- Calendar.cs
- XNodeNavigator.cs
- WSSecurityTokenSerializer.cs
- Publisher.cs
- CodeGenHelper.cs
- TimelineClockCollection.cs
- XmlBoundElement.cs
- PositiveTimeSpanValidatorAttribute.cs
- Expressions.cs
- VirtualPathUtility.cs
- EdmSchemaAttribute.cs
- XPathDocumentBuilder.cs
- DefaultValueTypeConverter.cs
- ClientType.cs
- DetailsViewDeleteEventArgs.cs
- DragStartedEventArgs.cs
- MaxValueConverter.cs
- XpsColorContext.cs
- LightweightEntityWrapper.cs
- GradientBrush.cs
- PropertyDescriptorCollection.cs
- ApplicationDirectory.cs
- SupportedAddressingMode.cs
- StringValidator.cs
- TreeIterators.cs
- IteratorAsyncResult.cs
- DrawingImage.cs
- ToolStripMenuItem.cs
- SQLMembershipProvider.cs
- DataGridViewCheckBoxColumn.cs
- ColumnMapProcessor.cs
- ExpressionPrefixAttribute.cs
- RequestDescription.cs
- InstanceDataCollection.cs
- Pen.cs
- Semaphore.cs
- ControlEvent.cs
- recordstate.cs
- VersionedStreamOwner.cs
- SerializableAttribute.cs
- HtmlInputButton.cs
- CompositeFontInfo.cs
- DataGridViewSelectedCellCollection.cs
- ConfigurationFileMap.cs
- ReflectTypeDescriptionProvider.cs
- RegisterInfo.cs
- BitmapScalingModeValidation.cs
- httpserverutility.cs
- ListComponentEditorPage.cs
- DataViewManager.cs
- StatusBarPanel.cs
- ReachDocumentSequenceSerializerAsync.cs
- SerializerWriterEventHandlers.cs
- WpfPayload.cs
- ReliabilityContractAttribute.cs
- CodeChecksumPragma.cs
- WebZone.cs
- DesignerVerbCollection.cs
- PauseStoryboard.cs
- PointUtil.cs
- BindingExpressionBase.cs
- OracleCommandBuilder.cs
- XMLSchema.cs
- DataGridToolTip.cs
- IDReferencePropertyAttribute.cs
- Attachment.cs
- RadioButtonPopupAdapter.cs
- ConnectionInterfaceCollection.cs
- MimeParameters.cs
- PropertyValueChangedEvent.cs
- ObjectContextServiceProvider.cs
- SqlInternalConnection.cs
- BufferBuilder.cs
- GeneratedCodeAttribute.cs
- TypographyProperties.cs
- Help.cs
- DesigntimeLicenseContextSerializer.cs
- SqlUtils.cs
- PasswordBoxAutomationPeer.cs
- HttpListenerPrefixCollection.cs
- CompositionTarget.cs
- DataGridColumnHeaderAutomationPeer.cs
- ClientCredentials.cs
- LinkLabel.cs
- PagedDataSource.cs
- ClientUrlResolverWrapper.cs
- BaseTemplateBuildProvider.cs
- AttachmentCollection.cs
- DataGridColumnHeaderAutomationPeer.cs
- WebPartRestoreVerb.cs