Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / ContextDataSourceView.cs / 1305376 / ContextDataSourceView.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls { #else namespace System.Web.UI.WebControls { #endif using System; using System.Collections; using System.Diagnostics.CodeAnalysis; using System.Reflection; using System.Security.Permissions; using System.Web; using System.Web.Compilation; using System.Web.UI; public abstract class ContextDataSourceView : QueryableDataSourceView { private string _entitySetName; private string _contextTypeName; private Type _contextType; private string _entityTypeName; private Type _entityType; private Type _entitySetType; private Control _owner; protected static readonly object EventContextCreating = new object(); protected static readonly object EventContextCreated = new object(); protected static readonly object EventContextDisposing = new object(); protected ContextDataSourceView(DataSourceControl owner, string viewName, HttpContext context) : base(owner, viewName, context) { _owner = owner; } internal ContextDataSourceView(DataSourceControl owner, string viewName, HttpContext context, IDynamicQueryable queryable) : base(owner, viewName, context, queryable) { } public string EntitySetName { get { return _entitySetName ?? String.Empty; } set { if (_entitySetName != value) { _entitySetName = value; _entitySetType = null; OnDataSourceViewChanged(EventArgs.Empty); } } } public string EntityTypeName { get { return _entityTypeName ?? String.Empty; } set { if (_entityTypeName != value) { _entityTypeName = value; _entityType = null; OnDataSourceViewChanged(EventArgs.Empty); } } } protected override Type EntityType { get { string typeName = EntityTypeName; if (_entityType == null) { _entityType = GetDataObjectTypeByName(typeName) ?? GetDataObjectType(EntitySetType); } return _entityType; } } public virtual string ContextTypeName { get { return _contextTypeName ?? String.Empty; } set { if (_contextTypeName != value) { _contextTypeName = value; _contextType = null; OnDataSourceViewChanged(EventArgs.Empty); } } } public virtual Type ContextType { get { if (_contextType == null && !String.IsNullOrEmpty(ContextTypeName)) { _contextType = DataSourceHelper.GetType(ContextTypeName); } return _contextType; } } ////// Current Context /// protected object Context { get; set; } ////// Current EntitySet /// protected object EntitySet { get; private set; } [SuppressMessage("Microsoft.Naming", "CA1721:PropertyNamesShouldNotMatchGetMethods", Justification = "The result of GetEntitySetType() is cached unless the EntitySetTypeName changes.")] protected Type EntitySetType { get { if (_entitySetType == null) { _entitySetType = GetEntitySetType(); } return _entitySetType; } } // Default implementation assumes the EntitySet is a property or field of the Context protected virtual Type GetEntitySetType() { MemberInfo mi = GetEntitySetMember(ContextType); if (mi.MemberType == MemberTypes.Property) { return ((PropertyInfo)mi).PropertyType; } else if (mi.MemberType == MemberTypes.Field) { return ((FieldInfo)mi).FieldType; } // throw new InvalidOperationException("EntitySet Type must be a field or property"); } private MemberInfo GetEntitySetMember(Type contextType) { string entitySetTypeName = EntitySetName; if (String.IsNullOrEmpty(entitySetTypeName)) { // return null; } MemberInfo[] members = contextType.FindMembers(MemberTypes.Field | MemberTypes.Property, BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static, /*filter*/null, /*filterCriteria*/null); for (int i = 0; i < members.Length; i++) { if (String.Equals(members[i].Name, entitySetTypeName, StringComparison.OrdinalIgnoreCase)) { return members[i]; } } return null; } private static Type GetDataObjectTypeByName(string typeName) { Type entityType = null; if (!String.IsNullOrEmpty(typeName)) { entityType = BuildManager.GetType(typeName, /*throwOnFail*/ false, /*ignoreCase*/ true); } return entityType; } protected virtual Type GetDataObjectType(Type type) { if (type.IsGenericType) { Type[] genericTypes = type.GetGenericArguments(); if (genericTypes.Length == 1) { return genericTypes[0]; } } // return typeof(object); } protected virtual ContextDataSourceContextData CreateContext(DataSourceOperation operation) { return null; } protected override object GetSource(QueryContext context) { ContextDataSourceContextData contextData = CreateContext(DataSourceOperation.Select); if (contextData != null) { // Set the current context Context = contextData.Context; EntitySet = contextData.EntitySet; return EntitySet; } return null; } protected override int ExecuteUpdate(IDictionary keys, IDictionary values, IDictionary oldValues) { ContextDataSourceContextData contextData = null; try { contextData = CreateContext(DataSourceOperation.Update); if (contextData != null) { // Set the current context Context = contextData.Context; EntitySet = contextData.EntitySet; return base.ExecuteUpdate(keys, values, oldValues); } } finally { DisposeContext(); } return -1; } protected override int ExecuteDelete(IDictionary keys, IDictionary oldValues) { ContextDataSourceContextData contextData = null; try { contextData = CreateContext(DataSourceOperation.Delete); if (contextData != null) { // Set the current context Context = contextData.Context; EntitySet = contextData.EntitySet; return base.ExecuteDelete(keys, oldValues); } } finally { DisposeContext(); } return -1; } protected override int ExecuteInsert(IDictionary values) { ContextDataSourceContextData contextData = null; try { contextData = CreateContext(DataSourceOperation.Insert); if (contextData != null) { // Set the current context Context = contextData.Context; EntitySet = contextData.EntitySet; return base.ExecuteInsert(values); } } finally { DisposeContext(); } return -1; } protected virtual void DisposeContext(object dataContext) { if (dataContext != null) { IDisposable disposableObject = dataContext as IDisposable; if (disposableObject != null) { disposableObject.Dispose(); } dataContext = null; } } protected void DisposeContext() { DisposeContext(Context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
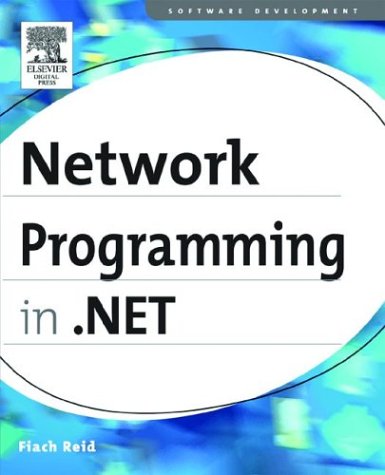
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIServiceHelper.cs
- TypeReference.cs
- SafeSecurityHandles.cs
- SuppressIldasmAttribute.cs
- RSAPKCS1KeyExchangeFormatter.cs
- TextBoxRenderer.cs
- VectorCollectionValueSerializer.cs
- DataException.cs
- IUnknownConstantAttribute.cs
- ConfigurationManager.cs
- UndoManager.cs
- CaseInsensitiveHashCodeProvider.cs
- ContextProperty.cs
- CustomPopupPlacement.cs
- RegisteredExpandoAttribute.cs
- CollectionAdapters.cs
- DataGridViewCell.cs
- ButtonRenderer.cs
- TraceListener.cs
- AudioBase.cs
- Int32EqualityComparer.cs
- ActiveXSite.cs
- SyndicationSerializer.cs
- ConsoleCancelEventArgs.cs
- DataListGeneralPage.cs
- DiscoveryDocument.cs
- ProfileSection.cs
- DesignerTransactionCloseEvent.cs
- DomNameTable.cs
- RawMouseInputReport.cs
- DbSetClause.cs
- StyleTypedPropertyAttribute.cs
- Debug.cs
- ToolStripDropDownButton.cs
- DocumentSequenceHighlightLayer.cs
- RtfToXamlLexer.cs
- ToolStripContainer.cs
- FixedTextBuilder.cs
- EnumValAlphaComparer.cs
- TypeConverterHelper.cs
- _NegoStream.cs
- ConfigXmlWhitespace.cs
- AuthenticationModulesSection.cs
- DefaultPropertyAttribute.cs
- SerializationAttributes.cs
- ListView.cs
- XmlResolver.cs
- WebResponse.cs
- EnumBuilder.cs
- ObjectHelper.cs
- MeshGeometry3D.cs
- DataGridDesigner.cs
- BinaryQueryOperator.cs
- EngineSiteSapi.cs
- FieldBuilder.cs
- ImageListDesigner.cs
- SQLInt32.cs
- FocusChangedEventArgs.cs
- PresentationTraceSources.cs
- TemplateField.cs
- BitConverter.cs
- codemethodreferenceexpression.cs
- ValidationVisibilityAttribute.cs
- RecordManager.cs
- IntegerValidatorAttribute.cs
- TransformerInfo.cs
- Query.cs
- _LazyAsyncResult.cs
- Object.cs
- SystemColors.cs
- TransformerInfoCollection.cs
- StreamReader.cs
- PersonalizableAttribute.cs
- CultureData.cs
- FormViewDesigner.cs
- _SSPIWrapper.cs
- ArrayConverter.cs
- TextShapeableCharacters.cs
- DrawingContextWalker.cs
- CompositeKey.cs
- OutputScopeManager.cs
- ProfileGroupSettingsCollection.cs
- QilSortKey.cs
- PasswordPropertyTextAttribute.cs
- ProcessInfo.cs
- BinaryWriter.cs
- iisPickupDirectory.cs
- GenericEnumerator.cs
- ComboBoxRenderer.cs
- SessionEndedEventArgs.cs
- LongCountAggregationOperator.cs
- GradientBrush.cs
- SynchronizedReadOnlyCollection.cs
- DbProviderFactoriesConfigurationHandler.cs
- DataSetSchema.cs
- XmlTypeMapping.cs
- ClientSponsor.cs
- Queue.cs
- VoiceObjectToken.cs
- ContextStack.cs