Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Hosting / SimpleWorkerRequest.cs / 1587057 / SimpleWorkerRequest.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Hosting { using System.Collections; using System.IO; using System.Runtime.InteropServices; using System.Security.Principal; using System.Security; using System.Security.Permissions; using System.Threading; using System.Web.Configuration; using System.Web.Util; // // Simple Worker Request provides a concrete implementation // of HttpWorkerRequest that writes the respone to the user // supplied writer. // ////// [ComVisible(false)] public class SimpleWorkerRequest : HttpWorkerRequest { private bool _hasRuntimeInfo; private String _appVirtPath; // "/foo" private String _appPhysPath; // "c:\foo\" private String _page; private String _pathInfo; private String _queryString; private TextWriter _output; private String _installDir; private void ExtractPagePathInfo() { int i = _page.IndexOf('/'); if (i >= 0) { _pathInfo = _page.Substring(i); _page = _page.Substring(0, i); } } private String GetPathInternal(bool includePathInfo) { String s = _appVirtPath.Equals("/") ? ("/" + _page) : (_appVirtPath + "/" + _page); if (includePathInfo && _pathInfo != null) return s + _pathInfo; else return s; } // // HttpWorkerRequest implementation // // "/foo/page.aspx/tail" ///[To be supplied.] ////// public override String GetUriPath() { return GetPathInternal(true); } // "param=bar" ///[To be supplied.] ////// public override String GetQueryString() { return _queryString; } // "/foo/page.aspx/tail?param=bar" ///[To be supplied.] ////// public override String GetRawUrl() { String qs = GetQueryString(); if (!String.IsNullOrEmpty(qs)) return GetPathInternal(true) + "?" + qs; else return GetPathInternal(true); } ///[To be supplied.] ////// public override String GetHttpVerbName() { return "GET"; } ///[To be supplied.] ////// public override String GetHttpVersion() { return "HTTP/1.0"; } ///[To be supplied.] ////// public override String GetRemoteAddress() { return "127.0.0.1"; } ///[To be supplied.] ////// public override int GetRemotePort() { return 0; } ///[To be supplied.] ////// public override String GetLocalAddress() { return "127.0.0.1"; } ///[To be supplied.] ////// public override int GetLocalPort() { return 80; } ///[To be supplied.] ////// public override IntPtr GetUserToken() { return IntPtr.Zero; } ///[To be supplied.] ////// public override String GetFilePath() { return GetPathInternal(false); } ///[To be supplied.] ////// public override String GetFilePathTranslated() { String path = _appPhysPath + _page.Replace('/', '\\'); InternalSecurityPermissions.PathDiscovery(path).Demand(); return path; } ///[To be supplied.] ////// public override String GetPathInfo() { return (_pathInfo != null) ? _pathInfo : String.Empty; } ///[To be supplied.] ////// public override String GetAppPath() { return _appVirtPath; } ///[To be supplied.] ////// public override String GetAppPathTranslated() { InternalSecurityPermissions.PathDiscovery(_appPhysPath).Demand(); return _appPhysPath; } ///[To be supplied.] ////// public override String GetServerVariable(String name) { return String.Empty; } ///[To be supplied.] ////// public override String MapPath(String path) { if (!_hasRuntimeInfo) return null; String mappedPath = null; String appPath = _appPhysPath.Substring(0, _appPhysPath.Length-1); // without trailing "\" if (String.IsNullOrEmpty(path) || path.Equals("/")) { mappedPath = appPath; } if (StringUtil.StringStartsWith(path, _appVirtPath)) { mappedPath = appPath + path.Substring(_appVirtPath.Length).Replace('/', '\\'); } InternalSecurityPermissions.PathDiscovery(mappedPath).Demand(); return mappedPath; } ///[To be supplied.] ////// public override string MachineConfigPath { get { if (_hasRuntimeInfo) { string path = HttpConfigurationSystem.MachineConfigurationFilePath; InternalSecurityPermissions.PathDiscovery(path).Demand(); return path; } else return null; } } ///[To be supplied.] ////// public override string RootWebConfigPath { get { if (_hasRuntimeInfo) { string path = HttpConfigurationSystem.RootWebConfigurationFilePath; InternalSecurityPermissions.PathDiscovery(path).Demand(); return path; } else return null; } } ///[To be supplied.] ////// public override String MachineInstallDirectory { get { if (_hasRuntimeInfo) { InternalSecurityPermissions.PathDiscovery(_installDir).Demand(); return _installDir; } return null; } } ///[To be supplied.] ////// public override void SendStatus(int statusCode, String statusDescription) { } ///[To be supplied.] ////// public override void SendKnownResponseHeader(int index, String value) { } ///[To be supplied.] ////// public override void SendUnknownResponseHeader(String name, String value) { } ///[To be supplied.] ////// public override void SendResponseFromMemory(byte[] data, int length) { _output.Write(System.Text.Encoding.Default.GetChars(data, 0, length)); } ///[To be supplied.] ////// public override void SendResponseFromFile(String filename, long offset, long length) { } ///[To be supplied.] ////// public override void SendResponseFromFile(IntPtr handle, long offset, long length) { } ///[To be supplied.] ////// public override void FlushResponse(bool finalFlush) { } ///[To be supplied.] ////// public override void EndOfRequest() { } internal override void UpdateInitialCounters() { PerfCounters.IncrementGlobalCounter(GlobalPerfCounter.REQUESTS_CURRENT); PerfCounters.IncrementCounter(AppPerfCounter.REQUESTS_TOTAL); } // // Internal support // internal override void UpdateResponseCounters(bool finalFlush, int bytesOut) { // Integrated mode uses a fake simple worker request to initialize (Dev10 Bugs 466973) if (HttpRuntime.UseIntegratedPipeline) { return; } if (finalFlush) { PerfCounters.DecrementGlobalCounter(GlobalPerfCounter.REQUESTS_CURRENT); PerfCounters.DecrementCounter(AppPerfCounter.REQUESTS_EXECUTING); } if (bytesOut > 0) { PerfCounters.IncrementCounterEx(AppPerfCounter.REQUEST_BYTES_OUT, bytesOut); } } internal override void UpdateRequestCounters(int bytesIn) { // Integrated mode uses a fake simple worker request to initialize (Dev10 Bugs 466973) if (HttpRuntime.UseIntegratedPipeline) { return; } if (bytesIn > 0) { PerfCounters.IncrementCounterEx(AppPerfCounter.REQUEST_BYTES_IN, bytesIn); } } // // Ctors // private SimpleWorkerRequest() { } /* * Ctor that gets application data from HttpRuntime, assuming * HttpRuntime has been set up (app domain specially created, etc.) */ ///[To be supplied.] ////// public SimpleWorkerRequest(String page, String query, TextWriter output): this() { _queryString = query; _output = output; _page = page; ExtractPagePathInfo(); _appPhysPath = Thread.GetDomain().GetData(".appPath").ToString(); _appVirtPath = Thread.GetDomain().GetData(".appVPath").ToString(); _installDir = HttpRuntime.AspInstallDirectoryInternal; _hasRuntimeInfo = true; } /* * Ctor that gets application data as arguments,assuming HttpRuntime * has not been set up. * * This allows for limited functionality to execute handlers. */ ///[To be supplied.] ////// public SimpleWorkerRequest(String appVirtualDir, String appPhysicalDir, String page, String query, TextWriter output): this() { if (Thread.GetDomain().GetData(".appPath") != null) { throw new HttpException(SR.GetString(SR.Wrong_SimpleWorkerRequest)); } _appVirtPath = appVirtualDir; _appPhysPath = appPhysicalDir; _queryString = query; _output = output; _page = page; ExtractPagePathInfo(); if (!StringUtil.StringEndsWith(_appPhysPath, '\\')) _appPhysPath += "\\"; _hasRuntimeInfo = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
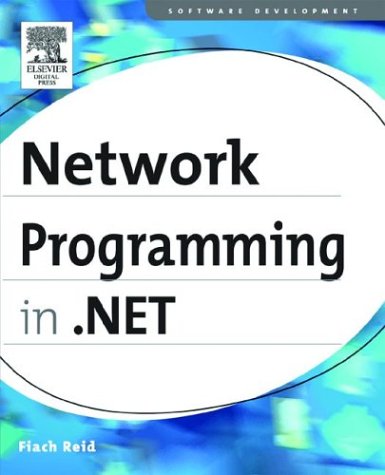
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormViewPageEventArgs.cs
- ShapingWorkspace.cs
- HtmlWindow.cs
- PageTheme.cs
- ListManagerBindingsCollection.cs
- InteropAutomationProvider.cs
- ItemContainerProviderWrapper.cs
- SqlParameterCollection.cs
- X509Extension.cs
- CharEnumerator.cs
- CheckBoxField.cs
- XPathDocumentBuilder.cs
- MessageVersion.cs
- ModifierKeysValueSerializer.cs
- MimeFormImporter.cs
- MappingModelBuildProvider.cs
- VectorAnimation.cs
- ConfigXmlDocument.cs
- ReflectionPermission.cs
- HtmlInputButton.cs
- CollectionViewGroup.cs
- CapabilitiesPattern.cs
- XmlSchemaDatatype.cs
- PointLightBase.cs
- MatrixConverter.cs
- PeerCustomResolverSettings.cs
- OutOfMemoryException.cs
- CodeMemberEvent.cs
- KeyValuePairs.cs
- FontClient.cs
- XmlAutoDetectWriter.cs
- EventHandlerList.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- MenuBase.cs
- X509CertificateValidator.cs
- Point3DAnimationBase.cs
- SqlRowUpdatedEvent.cs
- AdapterUtil.cs
- Vector3dCollection.cs
- AsyncCompletedEventArgs.cs
- WinInet.cs
- DataColumnPropertyDescriptor.cs
- DataGridTextBox.cs
- ControlDesigner.cs
- Stack.cs
- WindowsFormsHostAutomationPeer.cs
- PersonalizationProvider.cs
- ResXResourceReader.cs
- ColorKeyFrameCollection.cs
- FontStretch.cs
- FloaterBaseParagraph.cs
- HttpServerUtilityWrapper.cs
- MemberRelationshipService.cs
- SqlDependency.cs
- DbExpressionVisitor.cs
- FileUtil.cs
- UdpSocket.cs
- WinEventTracker.cs
- PrimitiveSchema.cs
- ToolboxItemCollection.cs
- PersonalizablePropertyEntry.cs
- CompressStream.cs
- DummyDataSource.cs
- ListViewSortEventArgs.cs
- DbParameterCollection.cs
- MenuItemCollectionEditor.cs
- StreamGeometryContext.cs
- ISFTagAndGuidCache.cs
- PeerEndPoint.cs
- FontConverter.cs
- StrongNameUtility.cs
- ListView.cs
- PopOutPanel.cs
- EncodedStreamFactory.cs
- RootBuilder.cs
- FileSystemEventArgs.cs
- RoleService.cs
- ParallelTimeline.cs
- XmlSchemaSimpleContentRestriction.cs
- Crypto.cs
- BoundField.cs
- Label.cs
- ButtonPopupAdapter.cs
- SpellerError.cs
- XmlQueryStaticData.cs
- EdmTypeAttribute.cs
- HttpHeaderCollection.cs
- Vector3D.cs
- ObjectItemCachedAssemblyLoader.cs
- Socket.cs
- InvokeGenerator.cs
- XmlSchemaExporter.cs
- ProgressBarRenderer.cs
- FragmentQuery.cs
- AutomationIdentifierGuids.cs
- Bits.cs
- Rect3DValueSerializer.cs
- mansign.cs
- ColorConverter.cs
- XmlDataImplementation.cs