Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / LocalValueEnumerator.cs / 1305600 / LocalValueEnumerator.cs
using System; using System.Collections; using System.Diagnostics; using MS.Internal.WindowsBase; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// Local value enumeration object /// ////// Modifying local values (via SetValue or ClearValue) during enumeration /// is unsupported /// public struct LocalValueEnumerator : IEnumerator { ////// Overrides Object.GetHashCode /// ///An integer that represents the hashcode for this object public override int GetHashCode() { return base.GetHashCode(); } ////// Determine equality /// public override bool Equals(object obj) { if(obj is LocalValueEnumerator) { LocalValueEnumerator other = (LocalValueEnumerator) obj; return (_count == other._count && _index == other._index && _snapshot == other._snapshot); } else { // being compared against something that isn't a LocalValueEnumerator. return false; } } ////// Determine equality /// public static bool operator ==(LocalValueEnumerator obj1, LocalValueEnumerator obj2) { return obj1.Equals(obj2); } ////// Determine inequality /// public static bool operator !=(LocalValueEnumerator obj1, LocalValueEnumerator obj2) { return !(obj1 == obj2); } ////// Get current entry /// public LocalValueEntry Current { get { if(_index == -1 ) { #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.LocalValueEnumerationReset)); } if(_index >= Count ) { #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.LocalValueEnumerationOutOfBounds)); } return _snapshot[_index]; } } ////// Get current entry (object reference based) /// object IEnumerator.Current { get { return Current; } } ////// Move to the next item in the enumerator /// ///Success of the method public bool MoveNext() { _index++; return _index < Count; } ////// Reset enumeration /// public void Reset() { _index = -1; } ////// Return number of items represented in the collection /// public int Count { get { return _count; } } internal LocalValueEnumerator(LocalValueEntry[] snapshot, int count) { _index = -1; _count = count; _snapshot = snapshot; } private int _index; private LocalValueEntry[] _snapshot; private int _count; } ////// Represents a Property-Value pair for local value enumeration /// public struct LocalValueEntry { ////// Overrides Object.GetHashCode /// ///An integer that represents the hashcode for this object public override int GetHashCode() { return base.GetHashCode(); } ////// Determine equality /// public override bool Equals(object obj) { LocalValueEntry other = (LocalValueEntry) obj; return (_dp == other._dp && _value == other._value); } ////// Determine equality /// public static bool operator ==(LocalValueEntry obj1, LocalValueEntry obj2) { return obj1.Equals(obj2); } ////// Determine inequality /// public static bool operator !=(LocalValueEntry obj1, LocalValueEntry obj2) { return !(obj1 == obj2); } ////// Dependency property /// public DependencyProperty Property { get { return _dp; } } ////// Value of the property /// public object Value { get { return _value; } } internal LocalValueEntry(DependencyProperty dp, object value) { _dp = dp; _value = value; } // Internal here because we need to change these around when building // the snapshot for the LocalValueEnumerator, and we can't make internal // setters when we have public getters. internal DependencyProperty _dp; internal object _value; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
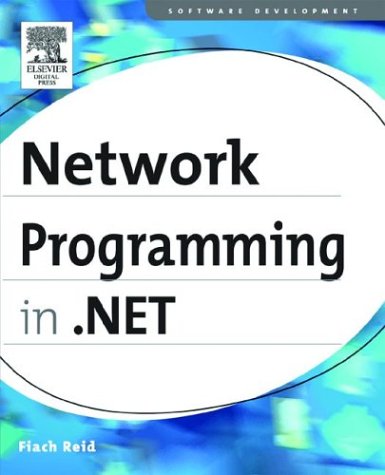
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- input.cs
- ScriptResourceHandler.cs
- DbgUtil.cs
- CommandHelper.cs
- AccessedThroughPropertyAttribute.cs
- WindowsTokenRoleProvider.cs
- tooltip.cs
- MethodBody.cs
- TagPrefixAttribute.cs
- PrintPreviewDialog.cs
- HScrollBar.cs
- TargetConverter.cs
- ItemContainerPattern.cs
- HttpModuleCollection.cs
- BulletChrome.cs
- UriTemplateEquivalenceComparer.cs
- InputBinder.cs
- FixedSOMTextRun.cs
- Journaling.cs
- ArgumentException.cs
- FlatButtonAppearance.cs
- followingquery.cs
- CheckableControlBaseAdapter.cs
- HtmlHistory.cs
- Utils.cs
- ComponentEditorPage.cs
- ProfileGroupSettingsCollection.cs
- ReadOnlyCollectionBase.cs
- StandardBindingReliableSessionElement.cs
- ChangeNode.cs
- ControlValuePropertyAttribute.cs
- CodeDelegateCreateExpression.cs
- NullRuntimeConfig.cs
- Stacktrace.cs
- SubMenuStyleCollection.cs
- ComboBox.cs
- SynthesizerStateChangedEventArgs.cs
- UpdatePanelTriggerCollection.cs
- DataControlCommands.cs
- TypeUtils.cs
- SecurityKeyIdentifier.cs
- WaitHandle.cs
- ProcessProtocolHandler.cs
- HostedNamedPipeTransportManager.cs
- MediaPlayerState.cs
- PeerToPeerException.cs
- AuthenticationModulesSection.cs
- ComponentSerializationService.cs
- Models.cs
- InkCanvasSelection.cs
- ToolStripContentPanelDesigner.cs
- DropAnimation.xaml.cs
- EntityClassGenerator.cs
- SettingsBase.cs
- LayoutEditorPart.cs
- X509Chain.cs
- PolicyImporterElement.cs
- Scene3D.cs
- TagPrefixAttribute.cs
- ConfigViewGenerator.cs
- MarginCollapsingState.cs
- XmlUtf8RawTextWriter.cs
- TraceData.cs
- tibetanshape.cs
- DataServiceException.cs
- TextProperties.cs
- GridLength.cs
- HttpHandlersSection.cs
- metadatamappinghashervisitor.cs
- OdbcConnectionStringbuilder.cs
- SocketManager.cs
- Soap.cs
- StructuredTypeInfo.cs
- HwndSource.cs
- ScrollProviderWrapper.cs
- BitmapEffectState.cs
- Math.cs
- SectionUpdates.cs
- Logging.cs
- LabelLiteral.cs
- PnrpPeerResolverBindingElement.cs
- EventSetterHandlerConverter.cs
- IdentifierService.cs
- SqlBooleanMismatchVisitor.cs
- BindingContext.cs
- MessageQueueException.cs
- EventToken.cs
- MSG.cs
- EventBuilder.cs
- SpnegoTokenAuthenticator.cs
- AutoResizedEvent.cs
- recordstatefactory.cs
- PlacementWorkspace.cs
- DataGrid.cs
- EntitySetBase.cs
- WindowsFormsSynchronizationContext.cs
- ZoneIdentityPermission.cs
- DragStartedEventArgs.cs
- WinFormsComponentEditor.cs
- StringCollectionMarkupSerializer.cs