Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / DataObjectEventArgs.cs / 1305600 / DataObjectEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Base class for DataObject events arguments // //--------------------------------------------------------------------------- using System; namespace System.Windows { ////// Base class for DataObject.Copying/Pasting events. /// These events are raised when an editor deals with /// a data object before putting it to clipboard on copy /// and before starting drag operation; /// or before Pasting its content into a selection /// on Paste/Drop operations. /// /// This class is abstract - it provides only common /// members for the events. Particular commands /// must use more specific event arguments - /// DataObjectCopyingEventArgs or DataObjectPastingEventArgs. /// public abstract class DataObjectEventArgs : RoutedEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a DataObjectCopyEvent. /// This object created by editors executing a Copy/Paste /// and Drag/Drop comands. /// /// /// An event id. One of: CopyingEvent or PastingEvent /// /// /// A flag indicating if this operation is part of drag/drop. /// Copying event fired on drag start, Pasting - on drop. /// Cancelling the command stops drag/drop process in /// an appropriate moment. /// internal DataObjectEventArgs(RoutedEvent routedEvent, bool isDragDrop) : base() { if (routedEvent != DataObject.CopyingEvent && routedEvent != DataObject.PastingEvent && routedEvent != DataObject.SettingDataEvent) { throw new ArgumentOutOfRangeException("routedEvent"); } RoutedEvent = routedEvent; _isDragDrop = isDragDrop; _commandCancelled = false; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// A flag indicating if this operation is part of drag/drop. /// Copying event fired on drag start, Pasting - on drop. /// Cancelling the command stops drag/drop process in /// an appropriate moment. /// public bool IsDragDrop { get { return _isDragDrop; } } ////// A current cancellation status of the event. /// When set to true, copy command is going to be cancelled. /// public bool CommandCancelled { get { return _commandCancelled; } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Public Methods ////// Sets cancelled status of a command to true. /// After calling this method the command will be /// stopped from calling. /// Applied to Drag (event="Copying", isDragDrop="true") /// this would stop the whole dragdrop process. /// ////// After an event has been cancelled it's impossible /// to re-enable it. /// public void CancelCommand() { _commandCancelled = true; } #endregion Public Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private bool _isDragDrop; private bool _commandCancelled; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
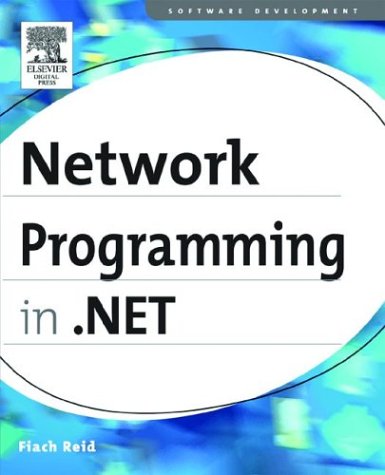
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- diagnosticsswitches.cs
- DataServiceSaveChangesEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- DataFieldConverter.cs
- SQLByte.cs
- SamlSerializer.cs
- ResourcesChangeInfo.cs
- DataGridViewDataConnection.cs
- TrustManager.cs
- CrossSiteScriptingValidation.cs
- PackagePart.cs
- FrameworkElement.cs
- HandleRef.cs
- XsdBuilder.cs
- AcceleratedTokenAuthenticator.cs
- PenThreadPool.cs
- CompiledQueryCacheKey.cs
- DataRecordObjectView.cs
- CorrelationToken.cs
- PlacementWorkspace.cs
- DataTableTypeConverter.cs
- DataGridViewComboBoxColumn.cs
- TdsParserHelperClasses.cs
- CardSpaceShim.cs
- CacheAxisQuery.cs
- Pens.cs
- ComponentDispatcherThread.cs
- NamespaceCollection.cs
- ThicknessAnimation.cs
- AsymmetricAlgorithm.cs
- FloaterParagraph.cs
- ManagedWndProcTracker.cs
- SettingsPropertyValue.cs
- SQLMoneyStorage.cs
- ContextStaticAttribute.cs
- CodeChecksumPragma.cs
- ExpressionBinding.cs
- RectIndependentAnimationStorage.cs
- AutomationFocusChangedEventArgs.cs
- BindingList.cs
- Cursor.cs
- FactoryGenerator.cs
- JournalEntry.cs
- EngineSiteSapi.cs
- PenThreadPool.cs
- CellQuery.cs
- Normalization.cs
- FixedDocument.cs
- VisualTreeUtils.cs
- MinMaxParagraphWidth.cs
- ActivityDelegate.cs
- InternalCompensate.cs
- SrgsElementFactoryCompiler.cs
- ConnectionPoint.cs
- PersonalizationDictionary.cs
- ParallelTimeline.cs
- RestHandler.cs
- LabelTarget.cs
- ShaderRenderModeValidation.cs
- ApplicationGesture.cs
- BuildProvider.cs
- PackWebRequestFactory.cs
- TemplatingOptionsDialog.cs
- CodeExporter.cs
- OLEDB_Enum.cs
- PreviousTrackingServiceAttribute.cs
- GeometryGroup.cs
- Message.cs
- IListConverters.cs
- AuthenticationSection.cs
- StylusPointPropertyUnit.cs
- TableLayoutColumnStyleCollection.cs
- ReadOnlyObservableCollection.cs
- TemplatedMailWebEventProvider.cs
- PersonalizationStateInfoCollection.cs
- OdbcErrorCollection.cs
- shaper.cs
- Automation.cs
- DLinqColumnProvider.cs
- EntityDescriptor.cs
- AnnotationService.cs
- GridEntry.cs
- RoutedPropertyChangedEventArgs.cs
- LayoutTable.cs
- DataGridRowDetailsEventArgs.cs
- milexports.cs
- RenderData.cs
- Int64.cs
- DependencyObjectProvider.cs
- Config.cs
- WindowsListViewScroll.cs
- LayoutInformation.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- XmlWriter.cs
- ConfigurationCollectionAttribute.cs
- Transform3D.cs
- ScriptManager.cs
- DataServiceClientException.cs
- HtmlElementEventArgs.cs
- TextParagraphView.cs