Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlSerializer.cs / 1305376 / SamlSerializer.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IdentityModel; using System.IdentityModel.Selectors; using System.Runtime.Serialization; using System.Security; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; using System.Text; using System.Xml; public class SamlSerializer { DictionaryManager dictionaryManager; public SamlSerializer() { } // Interface to plug in external Dictionaries. The external // dictionary should already be populated with all strings // required by this assembly. public void PopulateDictionary(IXmlDictionary dictionary) { if (dictionary == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("dictionary"); this.dictionaryManager = new DictionaryManager(dictionary); } internal DictionaryManager DictionaryManager { get { if (this.dictionaryManager == null) this.dictionaryManager = new DictionaryManager(); return this.dictionaryManager; } } public virtual SamlSecurityToken ReadToken(XmlReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); XmlDictionaryReader dictionaryReader = XmlDictionaryReader.CreateDictionaryReader(reader); WrappedReader wrappedReader = new WrappedReader(dictionaryReader); SamlAssertion assertion = LoadAssertion(wrappedReader, keyInfoSerializer, outOfBandTokenResolver); if (assertion == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLUnableToLoadAssertion))); //if (assertion.Signature == null) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SamlTokenMissingSignature))); return new SamlSecurityToken(assertion); } public virtual void WriteToken(SamlSecurityToken token, XmlWriter writer, SecurityTokenSerializer keyInfoSerializer) { if (token == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); #pragma warning suppress 56506 // token.Assertion is never null. token.Assertion.WriteTo(writer, this, keyInfoSerializer); } public virtual SamlAssertion LoadAssertion(XmlDictionaryReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); SamlAssertion assertion = new SamlAssertion(); assertion.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return assertion; } public virtual SamlCondition LoadCondition(XmlDictionaryReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); if (reader.IsStartElement(DictionaryManager.SamlDictionary.AudienceRestrictionCondition, DictionaryManager.SamlDictionary.Namespace)) { SamlAudienceRestrictionCondition audienceRestriction = new SamlAudienceRestrictionCondition(); audienceRestriction.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return audienceRestriction; } else if (reader.IsStartElement(DictionaryManager.SamlDictionary.DoNotCacheCondition, DictionaryManager.SamlDictionary.Namespace)) { SamlDoNotCacheCondition doNotCacheCondition = new SamlDoNotCacheCondition(); doNotCacheCondition.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return doNotCacheCondition; } else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.SAMLUnableToLoadUnknownElement, reader.LocalName))); } public virtual SamlConditions LoadConditions(XmlDictionaryReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); SamlConditions conditions = new SamlConditions(); conditions.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return conditions; } public virtual SamlAdvice LoadAdvice(XmlDictionaryReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); SamlAdvice advice = new SamlAdvice(); advice.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return advice; } public virtual SamlStatement LoadStatement(XmlDictionaryReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); if (reader.IsStartElement(DictionaryManager.SamlDictionary.AuthenticationStatement, DictionaryManager.SamlDictionary.Namespace)) { SamlAuthenticationStatement authStatement = new SamlAuthenticationStatement(); authStatement.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return authStatement; } else if (reader.IsStartElement(DictionaryManager.SamlDictionary.AttributeStatement, DictionaryManager.SamlDictionary.Namespace)) { SamlAttributeStatement attrStatement = new SamlAttributeStatement(); attrStatement.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return attrStatement; } else if (reader.IsStartElement(DictionaryManager.SamlDictionary.AuthorizationDecisionStatement, DictionaryManager.SamlDictionary.Namespace)) { SamlAuthorizationDecisionStatement authDecisionStatement = new SamlAuthorizationDecisionStatement(); authDecisionStatement.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return authDecisionStatement; } else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.SAMLUnableToLoadUnknownElement, reader.LocalName))); } public virtual SamlAttribute LoadAttribute(XmlDictionaryReader reader, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { // We will load all attributes as string values. SamlAttribute attribute = new SamlAttribute(); attribute.ReadXml(reader, this, keyInfoSerializer, outOfBandTokenResolver); return attribute; } // Helper metods to read and write SecurityKeyIdentifiers. internal static SecurityKeyIdentifier ReadSecurityKeyIdentifier(XmlReader reader, SecurityTokenSerializer tokenSerializer) { if (tokenSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("tokenSerializer", SR.GetString(SR.SamlSerializerRequiresExternalSerializers)); if (tokenSerializer.CanReadKeyIdentifier(reader)) { return tokenSerializer.ReadKeyIdentifier(reader); } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SamlSerializerUnableToReadSecurityKeyIdentifier))); } internal static void WriteSecurityKeyIdentifier(XmlWriter writer, SecurityKeyIdentifier ski, SecurityTokenSerializer tokenSerializer) { if (tokenSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("tokenSerializer", SR.GetString(SR.SamlSerializerRequiresExternalSerializers)); bool keyWritten = false; if (tokenSerializer.CanWriteKeyIdentifier(ski)) { tokenSerializer.WriteKeyIdentifier(writer, ski); keyWritten = true; } if (!keyWritten) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SamlSerializerUnableToWriteSecurityKeyIdentifier, ski.ToString()))); } internal static SecurityKey ResolveSecurityKey(SecurityKeyIdentifier ski, SecurityTokenResolver tokenResolver) { if (ski == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("ski"); if (tokenResolver != null) { for (int i = 0; i < ski.Count; ++i) { SecurityKey key = null; if (tokenResolver.TryResolveSecurityKey(ski[i], out key)) return key; } } if (ski.CanCreateKey) return ski.CreateKey(); return null; } internal static SecurityToken ResolveSecurityToken(SecurityKeyIdentifier ski, SecurityTokenResolver tokenResolver) { SecurityToken token = null; if (tokenResolver != null) { tokenResolver.TryResolveToken(ski, out token); } if (token == null) { // Check if this is a RSA key. RsaKeyIdentifierClause rsaClause; if (ski.TryFind(out rsaClause)) token = new RsaSecurityToken(rsaClause.Rsa); } if (token == null) { // Check if this is a X509RawDataKeyIdentifier Clause. X509RawDataKeyIdentifierClause rawDataKeyIdentifierClause; if (ski.TryFind (out rawDataKeyIdentifierClause)) token = new X509SecurityToken(new X509Certificate2(rawDataKeyIdentifierClause.GetX509RawData())); } return token; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
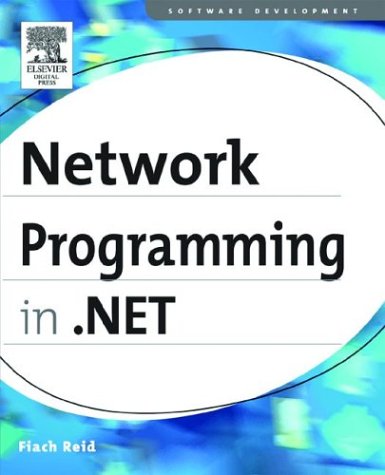
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolboxComponentsCreatedEventArgs.cs
- ImageProxy.cs
- WebPartCatalogAddVerb.cs
- Geometry.cs
- UntypedNullExpression.cs
- Pkcs9Attribute.cs
- EmptyWorkItem.cs
- PkcsUtils.cs
- HtmlTitle.cs
- SiteMapPath.cs
- Operator.cs
- TimeoutValidationAttribute.cs
- SessionPageStateSection.cs
- ConditionedDesigner.cs
- Parser.cs
- Label.cs
- DataGridViewElement.cs
- DeviceSpecificChoice.cs
- ClientCredentials.cs
- X509AsymmetricSecurityKey.cs
- DataControlCommands.cs
- ToolStripManager.cs
- UserNamePasswordValidationMode.cs
- FormView.cs
- BooleanStorage.cs
- SiteOfOriginContainer.cs
- SoapInteropTypes.cs
- CatalogZoneAutoFormat.cs
- LinkLabelLinkClickedEvent.cs
- EndpointAddressMessageFilterTable.cs
- SafeUserTokenHandle.cs
- ToolStripOverflowButton.cs
- Semaphore.cs
- SelectionItemPattern.cs
- WebServiceClientProxyGenerator.cs
- ModelTreeEnumerator.cs
- Roles.cs
- ConnectionProviderAttribute.cs
- BezierSegment.cs
- OpenTypeLayout.cs
- HtmlInputReset.cs
- RepeaterItemCollection.cs
- FileDataSourceCache.cs
- XmlEncodedRawTextWriter.cs
- IndexerReference.cs
- FixedTextSelectionProcessor.cs
- ControlBuilderAttribute.cs
- X509SecurityToken.cs
- SessionStateUtil.cs
- WebPartConnectionsConfigureVerb.cs
- UnsafeNativeMethods.cs
- TreeViewImageIndexConverter.cs
- XmlSchemaAppInfo.cs
- SqlXmlStorage.cs
- IPPacketInformation.cs
- DBProviderConfigurationHandler.cs
- tibetanshape.cs
- SerialStream.cs
- ShutDownListener.cs
- RecordsAffectedEventArgs.cs
- RemoveStoryboard.cs
- BypassElement.cs
- ProtocolsConfigurationHandler.cs
- COM2Properties.cs
- MessageQueueException.cs
- ADMembershipUser.cs
- FontStyleConverter.cs
- MarshalByRefObject.cs
- DocumentCollection.cs
- ProbeMatchesMessage11.cs
- DataPagerFieldCollection.cs
- Message.cs
- ControlBuilderAttribute.cs
- BorderGapMaskConverter.cs
- DataGridColumnHeaderItemAutomationPeer.cs
- SqlProcedureAttribute.cs
- PasswordBoxAutomationPeer.cs
- WebServicesDescriptionAttribute.cs
- Queue.cs
- WorkflowFileItem.cs
- ChtmlTextWriter.cs
- PresentationTraceSources.cs
- InstanceDataCollection.cs
- GZipStream.cs
- FileStream.cs
- InvalidateEvent.cs
- ImmutableObjectAttribute.cs
- Point4DValueSerializer.cs
- ParserExtension.cs
- SetterBase.cs
- Attachment.cs
- DiscreteKeyFrames.cs
- DesignerForm.cs
- HMACMD5.cs
- CqlIdentifiers.cs
- ScopedMessagePartSpecification.cs
- COM2Enum.cs
- Matrix.cs
- FileDialog_Vista.cs
- compensatingcollection.cs